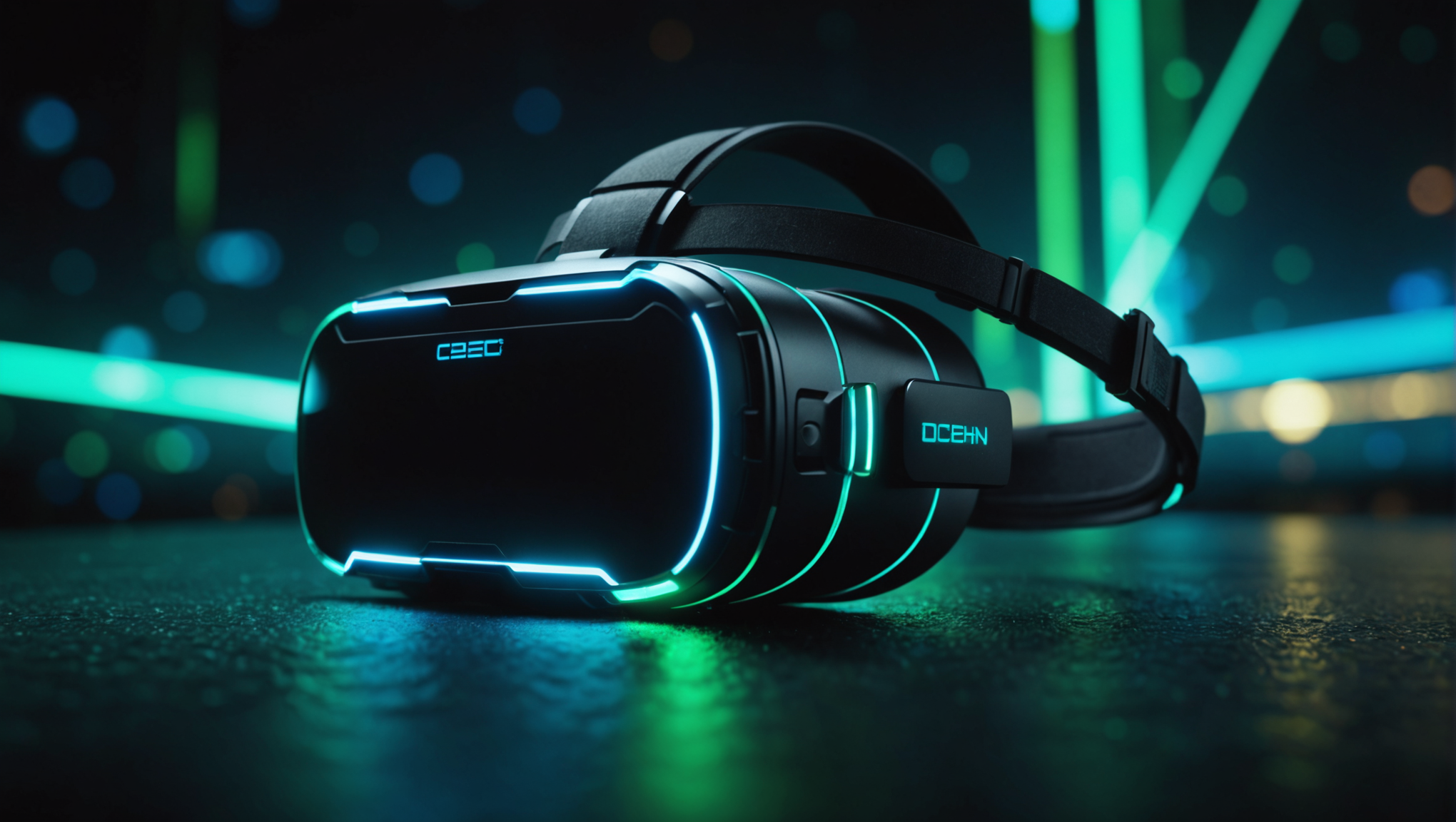
Python and Virtual Reality: Getting Started
Virtual reality (VR) is an immersive technology that simulates a user’s physical presence in a virtual environment. By using VR hardware such as headsets and motion controllers, users can interact with 3D spaces in a way that feels real. Understanding the core concepts of VR is essential for anyone looking to develop VR applications using Python.
- VR provides a sense of presence, allowing users to feel as though they are actually inside a digital world. This experience can be enhanced through sensory feedback, including visual, auditory, and haptic responses.
- Tracking: Movement tracking very important for VR. It allows the system to detect the user’s position and orientation within the virtual space. Tracking can be achieved via various technologies such as:
- Inertial Measurement Units (IMUs)
- Optical tracking using cameras
- Ultrasonic and radio frequency systems
- Users interact with the virtual environment through controllers or hand tracking. Actions such as grabbing, throwing, or manipulating objects must be translated into the virtual space, which requires careful programming to ensure intuitive user experience.
- The process of creating visual content in a VR application is known as 3D rendering. It involves rendering 3D models, textures, lighting, and shadows to create realistic environments.
- The extent of the observable world seen at any given moment is determined by the field of view. A wider FoV enhances immersion but can also increase the complexity of rendering.
- Low latency is critical in VR to prevent motion sickness and provide a smooth user experience. Any delay between a user’s movement and the corresponding change in the virtual environment can disrupt immersion.
As you begin your journey into VR with Python, it’s important to grasp these foundational concepts. They lay the groundwork for effectively developing engaging and immersive virtual experiences.
Setting Up Your Python Environment for VR
To embark on your VR development journey using Python, you first need to set up a suitable development environment. This involves ensuring that you have the necessary tools and libraries installed, as well as configuring your system to work with VR hardware. Below are the steps to guide you through this process.
- Make sure you have Python installed on your system. You can download the latest version from the official Python website.
- It’s a good practice to create a virtual environment for your VR projects to avoid dependency conflicts with other Python packages. Here’s how to create and activate a virtual environment:
# Install venv module if not already installed pip install virtualenv # Create a new virtual environment named vr_env python -m venv vr_env # Activate the virtual environment (use 'vr_envScriptsactivate' on Windows) source vr_env/bin/activate
- Depending on the VR framework you choose, you will need to install various packages. Here are some common libraries used for VR development in Python:
# Install the Pygame library for game development pip install pygame # Install OpenVR for accessing VR hardware pip install openvr # Install PyOpenGL for rendering 3D graphics pip install PyOpenGL PyOpenGL_accelerate
After installing the libraries, you can verify their installation by running a simple script to check if they import correctly:
try: import pygame import openvr from OpenGL import GL print("All libraries imported successfully!") except ImportError as e: print(f"Error importing libraries: {e}")
Ensure your VR hardware is properly set up and recognized by your operating system. For most common VR headsets, installing the manufacturer’s drivers and software will help in this regard. You can verify if your hardware is detected using the OpenVR library:
import openvr def check_vr_hardware(): openvr.init(openvr.VRApplication_Scene) system = openvr.VRSystem() if system: print("VR hardware detected:") print(f" HMD Type: {system.getStringTrackedDeviceProperty(openvr.k_unTrackedDeviceIndex_Hmd, openvr.Prop_TrackingSystemName_String)}") else: print("No VR hardware detected.") check_vr_hardware()
Once your environment is set up and hardware verified, you are ready to explore VR programming using Python. This foundational setup especially important for developing effective VR applications, as it ensures that you have control over the development process and a working platform to experiment with your ideas.
Exploring VR Frameworks and Libraries
As you progress in your VR development journey, you’ll find that selecting the right frameworks and libraries especially important. There are several popular options available for Python developers, each offering unique features and capabilities tailored for different aspects of VR development. Below are some of the most commonly used frameworks and libraries in the Python ecosystem for creating immersive VR experiences:
- While primarily a game development library, Pygame can be used for VR applications, especially for handling input and creating basic windowing systems. It can serve as a good starting point if you are also interested in 2D game development.
- Developed by Valve, OpenVR is a powerful library for accessing virtual reality hardware. It provides an interface for working with VR headsets and controllers, making it easier to manage interactions and tracking.
- This library allows you to leverage OpenGL, which is essential for rendering 3D graphics in VR. PyOpenGL provides a Pythonic way to interact with OpenGL, making it easier to create complex visual scenes.
- Vizard is a commercial VR software platform that includes a Python API. It’s highly optimized for VR development and provides a high number of built-in functions for creating interactive environments. Although it is commercial, it is favored for its comprehensive features and ease of use.
- Although primarily a game engine, Godot supports scripting in Python and has built-in support for VR. It provides a robust set of tools for designing games and simulations, including physics, 3D modeling, and animations.
- Blender is not just a 3D modeling tool; it has a Python API that allows scripting for VR applications. While it’s mainly used for creating assets, its support for VR rendering means you can create immersive experiences directly from your models.
When choosing a framework, consider your development goals, the complexity of your application, and your familiarity with the tools. For instance, if you are building a simple VR game, Pygame combined with OpenVR and PyOpenGL might suffice. However, for a more complex application requiring advanced rendering, you might want to look into Vizard or Godot.
Here’s how you can start a simple VR environment using OpenVR and PyOpenGL:
import openvr from OpenGL.GL import * from OpenGL.GL.shaders import compileProgram, compileShader def setup_vr(): openvr.init(openvr.VRApplication_Scene) vr_system = openvr.VRSystem() return vr_system def main(): vr_system = setup_vr() print("VR system initialized.") # Load shaders, set up OpenGL, etc. # That's just the setup part; rendering and VR interactions would follow. if __name__ == "__main__": main()
Using these frameworks and libraries, you can start building engaging virtual environments that harness the full potential of VR. Experimenting with different libraries will help you understand their strengths and weaknesses, ultimately leading to better VR application design in Python.
Building Your First Virtual Reality Application in Python
import openvr from OpenGL.GL import * from OpenGL.GL.shaders import compileProgram, compileShader def setup_vr(): openvr.init(openvr.VRApplication_Scene) vr_system = openvr.VRSystem() return vr_system def main(): vr_system = setup_vr() print("VR system initialized.") # Load shaders, set up OpenGL, etc. # That's just the setup part; rendering and VR interactions would follow. if __name__ == "__main__": main()
Once you have your development environment in place and are familiar with the frameworks available, it’s time to build your first virtual reality application in Python. This process can be broken down into several key steps, including setting up your VR scene, managing user input, and rendering 3D graphics.
First, let’s create a basic VR scene. The scene can consist of a simple 3D object, such as a cube or sphere, that we will render within the virtual environment. To do this, you will need to set up the rendering context and create a display loop that handles the rendering of the 3D objects.
Below is an example of setting up a simple VR scene with a cube:
def create_cube(): vertices = [ -1, -1, -1, # Back bottom left 1, -1, -1, # Back bottom right 1, 1, -1, # Back top right -1, 1, -1, # Back top left -1, -1, 1, # Front bottom left 1, -1, 1, # Front bottom right 1, 1, 1, # Front top right -1, 1, 1 # Front top left ] indices = [ 0, 1, 2, 2, 3, 0, # Back face 4, 5, 6, 6, 7, 4, # Front face 0, 1, 5, 5, 4, 0, # Bottom face 2, 3, 7, 7, 6, 2, # Top face 0, 3, 7, 7, 4, 0, # Left face 1, 2, 6, 6, 5, 1 # Right face ] # Create OpenGL buffers and load vertices # Note: You would need to add the OpenGL setup code here for the buffers. def render_loop(): while True: # Handle VR rendering loop here glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT) create_cube() # Add rendering code to draw the cube # Swap buffers, handle events, etc. if __name__ == "__main__": main()
In the example above, we define a `create_cube` function that specifies the vertices and indices for a cube, which will be rendered in our VR environment. The `render_loop` function manages the rendering process, ensuring that the cube is displayed each frame.
Next, user input and interaction are essential for creating an engaging VR experience. You will use the OpenVR library to track the user’s position and orientation, as well as to handle input from VR controllers. Here’s a simple example of how to track controller input:
def handle_input(): # Track controller states controller_state = openvr.VRInput().getControllerState() if controller_state.unButtonPressed & openvr.k_EButton_SteamVR_Trigger: print("Trigger pressed!") # Add interaction code here def render_loop(): while True: handle_input() # Continue with the rest of the rendering code
In this case, the `handle_input` function checks if the VR controller’s trigger button has been pressed. You can expand this to include actions such as grabbing or throwing objects, which greatly enhance the interactive nature of the VR experience.
Combining these elements—scene rendering, user interaction, and input handling—will allow you to create a basic yet functional VR application in Python. From here, you can expand your application by adding more complex objects, enhancing graphics, implementing physics, or even creating a storyline for users to engage with. Experimentation and iteration are key to developing a robust VR application, so feel free to explore different designs and functionalities as you continue your VR development journey in Python.
It would be beneficial to include a section on optimizing performance in VR applications, as achieving smooth frame rates is critical for a comfortable user experience. Discussing techniques such as level of detail (LOD) rendering, culling, and the use of batching can help developers create more efficient applications. Providing information on how to profile VR applications for performance bottlenecks would also enhance the article—it’s essential for developers to recognize and address performance issues early in the development process.