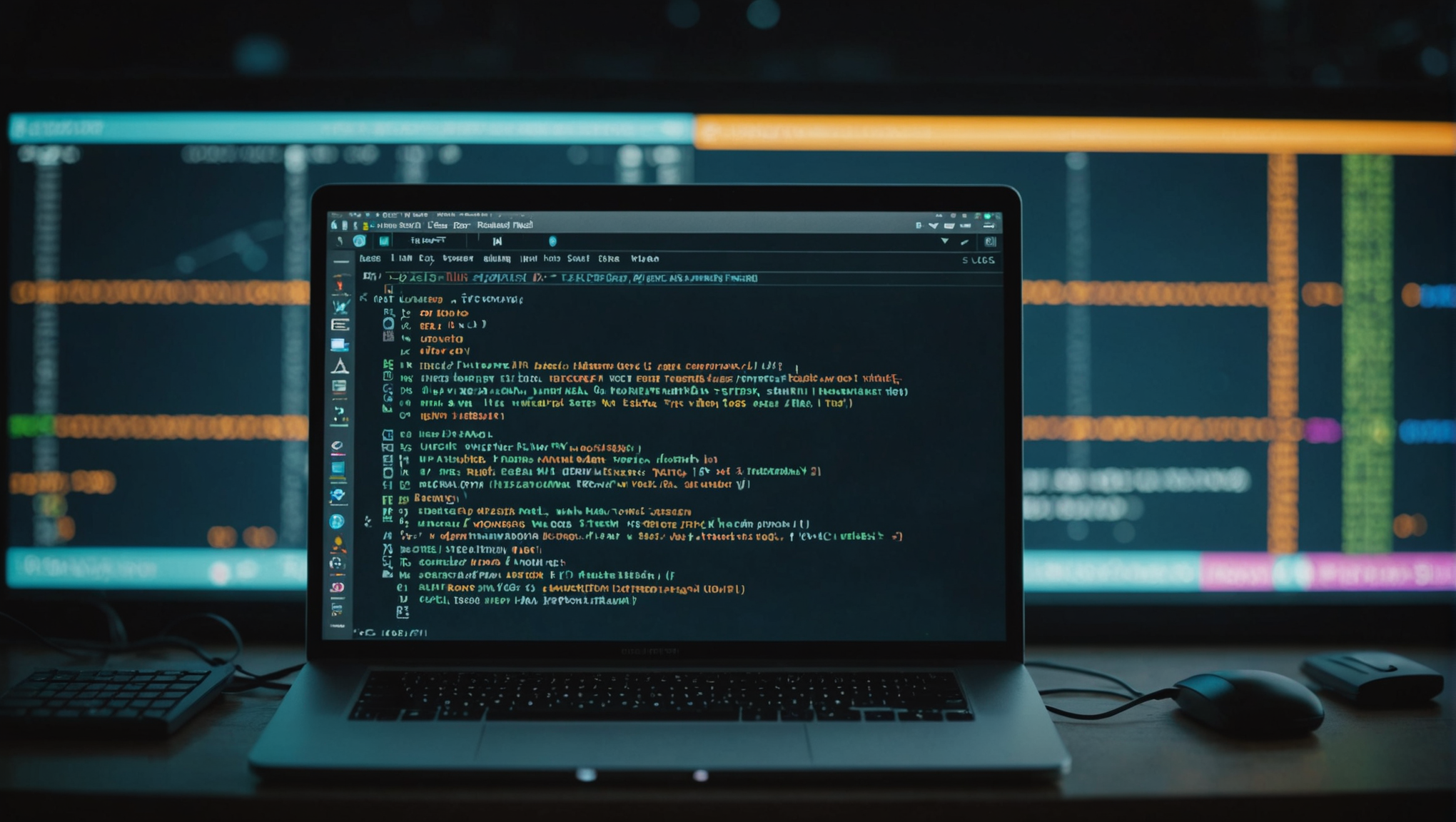
Cross-Platform Bash Scripting
Bash (Bourne Again SHell) is a widely used command interpreter that runs on various platforms, including Linux, macOS, and Windows (via tools like WSL or Cygwin). However, despite its popularity, Bash exhibits differences across these platforms that can impact script portability and functionality. Understanding these compatibility issues is essential for writing scripts that can run seamlessly across different operating systems.
Shell Versions and Implementations: Different environments may have different versions of Bash or may not even support Bash at all. For instance, macOS ships with a modified version of Bash, and Windows traditionally uses cmd.exe or PowerShell. Each environment may have unique features, syntax, or built-in commands missing in others. To write compatible scripts, it’s advisable to target the common features and syntax supported by all environments.
Line Endings: One crucial aspect of cross-platform compatibility is the handling of line endings. Unix/Linux uses a line feed (LF, n
), while Windows uses a carriage return followed by a line feed (CRLF, rn
). This discrepancy can cause issues when scripts are transferred between these systems. To ensure compatibility, it’s recommended to create your scripts using Unix line endings. You can enforce this by converting line endings before executing your scripts:
# Convert CRLF to LF in a file dos2unix myscript.sh
Environment Variables: Environment variable definitions may also differ across systems. Some variables might be available in one environment but absent in another, leading to unexpected results. As a best practice, always check for the existence of required variables and assign defaults if needed:
# Check for a variable and assign a default value : ${MY_VAR:=default_value}
Command Availability: Not all commands are guaranteed to be available on every system. For example, the grep
command is commonly available, but not all options may function identically across systems. It is important to stick to widely supported commands and be cautious when using flags. Always consult the system’s manual pages (e.g., man command
) or use command -v
to check command availability:
# Test for command availability if command -v mycommand > /dev/null; then echo "mycommand is available" else echo "mycommand is not available" fi
Path and Filename Considerations: File paths differ significantly between Unix-like systems and Windows. Unix uses forward slashes (/
) while Windows uses backslashes (). When writing scripts intended to run on multiple platforms, think using relative paths or built-in Bash features like
dirname
or basename
to manipulate paths correctly:
# Using base name and directory name for cross-platform paths SCRIPT_PATH="$(dirname "$0")" FILENAME="$(basename "$0")"
Writing Portable Scripts: Best Practices
When writing portable Bash scripts, following best practices is important to ensure that your scripts work seamlessly across different platforms. Here are several key practices to consider:
- Start your scripts with a shebang line specifying Bash, ensuring that the script uses the right interpreter:
#!/bin/bash
result=$(ls -l)
echo "File: $filename"
my_function() { echo "This is a portable function" }
set -e cp source.txt destination.txt
script_dir="$(cd "$(dirname "${BASH_SOURCE[0]}")" >> /dev/null && pwd)"
# This script requires 'curl' to be installed on the system.
Handling Platform-Specific Features and Limitations
When developing cross-platform Bash scripts, it’s vital to address platform-specific features and limitations. Each environment can have unique characteristics that, if not properly handled, may lead to errors or unexpected behavior in your scripts. Below are some key considerations for managing these differences effectively:
- The structure of the file system may vary significantly between platforms, especially regarding case sensitivity. Unix-based systems are generally case-sensitive, while Windows file systems (like NTFS) are not. As a result, references to files and directories should maintain consistent casing to avoid access issues.
- The implementation of regular expressions may differ slightly among tools and platforms. When using utilities like grep or sed, ensure you’re aware of the nuances in the regex syntax. Stick to basic patterns that are most likely to be supported across all platforms. For example, using basic regex without relying on extended options can enhance compatibility:
grep "pattern" filename
trap 'echo "Script interrupted"; exit' SIGINT
export LC_ALL=C
if ! command -v jq > /dev/null; then echo "'jq' is not available, falling back to alternatives." fi
Testing and Debugging Cross-Platform Bash Scripts
When it comes to testing and debugging cross-platform Bash scripts, a systematic approach is essential to identify and resolve issues effectively. Here are several strategies and tools that can assist in ensuring your scripts run smoothly across different environments:
- Setting up virtual machines or using container tools like Docker allows you to create isolated environments that simulate various platforms. This enables thorough testing of your scripts in conditions similar to your end users’. For example, you can create a Linux environment on Windows using WSL or run different versions of Bash in Docker containers.
- To catch syntax errors before running your script, ponder using a syntax checker. Bash provides the `-n` flag to check for syntax errors without executing the script:
bash -n myscript.sh
bash -x myscript.sh
my_command if [ $? -ne 0 ]; then echo "Command failed" fi
echo "Running script..." >> script.log
# Example Bats test @test "check command output" { run ./myscript.sh [ "$status" -eq 0 ] [ "${output}" = "Expected Output" ] }