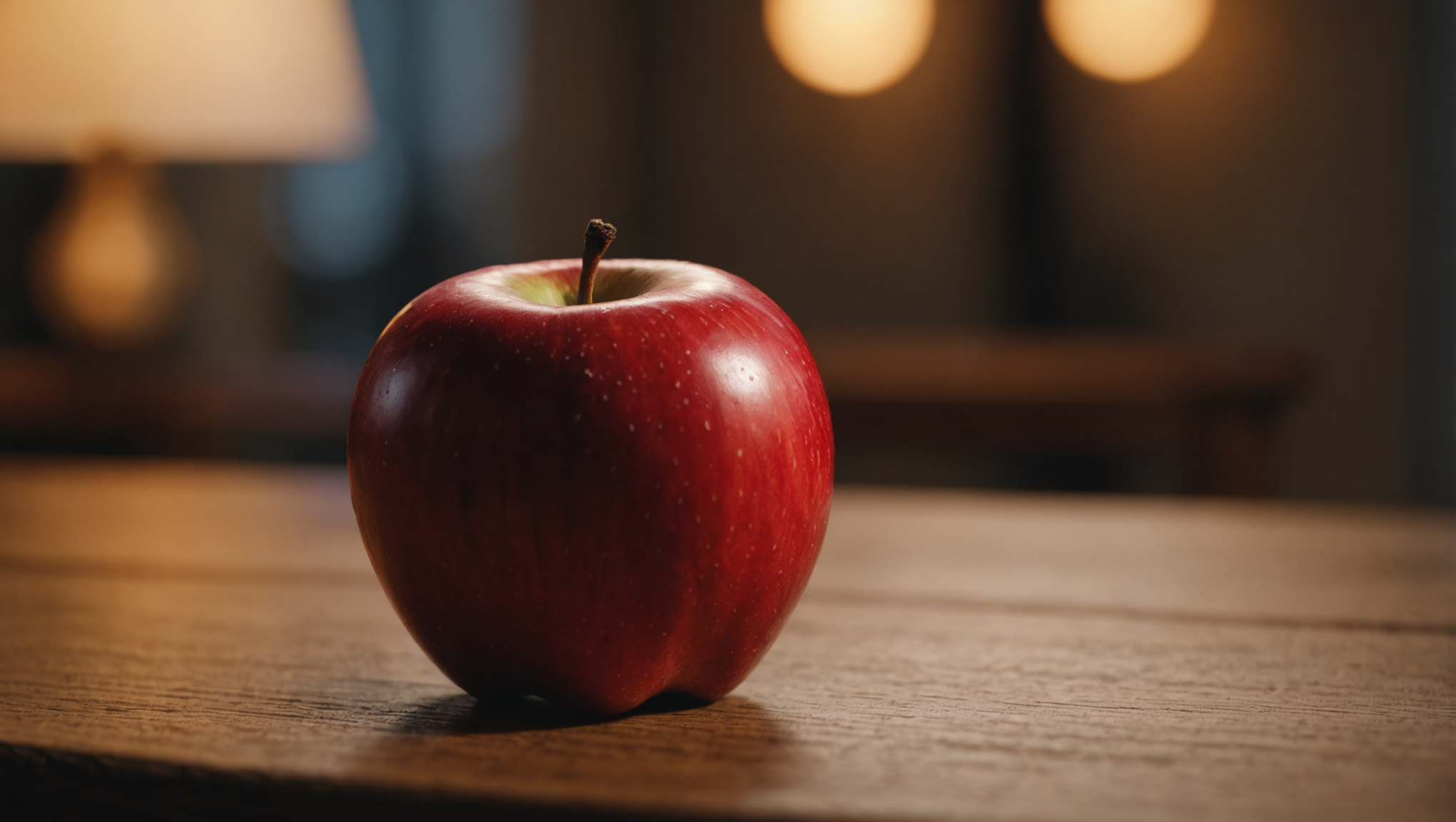
Data Types in Swift
Swift is a powerful programming language that offers a rich set of basic data types designed to handle a vast range of tasks with efficiency and clarity. At the core of Swift’s type system are the fundamental data types, which include Int, Double, Float, Bool, String, and Character. Understanding these types is essential for writing effective Swift code.
Int represents integer values, and it can store both positive and negative whole numbers. The size of an Int is determined by the platform, being either 32-bit or 64-bit. Here is a simple example:
let age: Int = 30
For floating-point numbers, Swift provides Double and Float. Double is a double-precision 64-bit floating-point type, suitable for most calculations requiring decimal precision. Meanwhile, Float is a single-precision 32-bit type, which can conserve memory in large arrays of floating-point numbers. Here’s how to declare these:
let pi: Double = 3.14159 let smallNumber: Float = 1.23
Bool is a simple yet crucial data type that represents a value that can either be true or false. This type is frequently used in conditional statements:
let isRaining: Bool = false
The String type in Swift is designed to hold text. It’s a collection of characters and supports Unicode, making it a versatile choice for international applications. Here’s an example of string manipulation:
let greeting: String = "Hello, World!" let greetingLength: Int = greeting.count
Moreover, Swift introduces the Character type, which represents a single character. That’s especially useful when you need to manipulate or display individual characters:
let firstLetter: Character = "H"
Swift also supports type inference, allowing the compiler to deduce the type of a variable from its initial value. This makes for cleaner, more concise code. For instance:
let inferredInt = 42 // inferred as Int let inferredDouble = 3.14 // inferred as Double
Swift’s basic data types are foundational elements that contribute to its expressive power and safety in handling data. By using these types, developers can create robust applications that perform effectively across a wide array of scenarios.
Collections: Arrays, Dictionaries, and Sets
In Swift, collections play an important role in managing groups of related values. The primary collection types are Arrays, Dictionaries, and Sets, each designed to serve different purposes while providing a high level of efficiency and type safety.
Arrays are ordered collections that store multiple values of the same type. They can be mutable or immutable, depending on how they are declared. You can easily add, remove, or modify values within an array. Here’s how you can work with arrays:
var numbers: [Int] = [1, 2, 3, 4, 5] // An array of integers numbers.append(6) // Add an element let firstNumber = numbers[0] // Accessing the first element numbers[1] = 10 // Modifying the second element
Swift’s Dictionaries provide a structure to store key-value pairs. Each key must be unique, and it is used to access its corresponding value. This allows for efficient lookups, as the dictionary is optimized for performance. Here’s a quick example:
var capitals: [String: String] = ["USA": "Washington, D.C.", "France": "Paris"] let usaCapital = capitals["USA"] // Retrieve the capital of the USA capitals["Germany"] = "Berlin" // Adding a new key-value pair
Sets offer a unique collection type that stores unordered values, ensuring that each value is distinct. This makes sets particularly useful when you need to eliminate duplicates or check for membership. Here’s how to create and use a set:
var uniqueNumbers: Set = [1, 2, 3, 4, 4, 5] // Dupe 4 will be removed uniqueNumbers.insert(6) // Add an element let containsThree = uniqueNumbers.contains(3) // Check for presence
An essential characteristic of all these collections is their type safety. Swift enforces type constraints on collections, ensuring that all values stored within them are of the specified type. This leads to fewer runtime errors and greater predictability in your code.
Additionally, collections in Swift come features an variety of methods and properties that facilitate operations such as iteration, filtering, and transformation. For example, you can easily iterate through an array using a for loop:
for number in numbers { print(number) }
Swift’s collections—Arrays, Dictionaries, and Sets—provide developers with robust tools for managing groups of related values. Their efficiency, type safety, and ease of use make them indispensable for creating effective Swift applications.
Optionals: Understanding the Absence of Value
In Swift, optionals play an important role in handling the absence of a value. An optional is a type that can hold either a value or `nil`, indicating that a value is missing. This powerful feature enables developers to represent the absence of data explicitly, thereby avoiding common pitfalls associated with null references that can lead to runtime crashes in other programming languages.
To declare an optional, you append a question mark `?` to the type. For instance, if you want to define a variable that can hold an integer or be `nil`, you would write:
var optionalAge: Int? = nil
In this example, `optionalAge` is an optional integer that is initially set to `nil`. You can later assign a valid integer value to it:
optionalAge = 25
To work with optionals safely, Swift provides a feature called optional binding, which allows you to check if an optional contains a value and safely unwrap it. Here’s how you can use optional binding with an `if let` statement:
if let age = optionalAge { print("The age is (age)") } else { print("Age is not available.") }
If `optionalAge` contains a value, it is safely unwrapped and assigned to the constant `age`. Otherwise, the code within the `else` block is executed.
Another way to unwrap an optional is using forced unwrapping, which is done by placing an exclamation mark `!` after the optional variable. However, this should be used with caution, as it will cause a runtime error if the optional is `nil`:
let forcedAge = optionalAge! // Risky! Will crash if optionalAge is nil print("The age is (forcedAge)")
Swift also provides optional chaining, so that you can call properties, methods, and subscripts on optional that might currently be `nil`. If the optional is `nil`, the entire chain returns `nil` without crashing. Here’s an example:
struct Person { var name: String var age: Int? } let person: Person? = Person(name: "John", age: nil) let personAge = person?.age // Optional Int? will be nil if person or person.age is nil
In this case, `personAge` will be `nil` if either `person` is `nil` or `age` is not assigned a value.
Swift’s optionals are not just a safety feature; they are a fundamental part of the language’s design philosophy, promoting safer code by ensuring that developers explicitly handle the potential absence of values. This reduces the likelihood of unexpected behavior in applications and enhances overall code quality.
Type Casting and Type Safety
Type casting in Swift is a mechanism that allows you to treat an instance of one type as another type. That’s particularly useful when working with class hierarchies or protocols, where you may need to verify and convert types at runtime. Swift provides two primary casting operators: the optional cast operator (`as?`) and the forced cast operator (`as!`). Understanding when to use these operators is essential for maintaining type safety in your applications.
The optional cast operator (`as?`) attempts to cast a value to a specified type and returns an optional result. If the cast is successful, it returns the value wrapped in an optional; if it fails, it returns `nil`. This allows you to handle potential casting failures gracefully. Here’s an example:
class Animal {} class Dog: Animal { func bark() { print("Woof!") } } let myPet: Animal = Dog() // myPet is of type Animal if let myDog = myPet as? Dog { myDog.bark() // Successfully casts and calls bark() } else { print("It's not a dog.") }
In this example, `myPet` is declared as an `Animal` type but is actually an instance of `Dog`. By using `as?`, we safely attempt to cast `myPet` to `Dog`, allowing us to call the `bark()` method only if the cast is successful.
On the other hand, the forced cast operator (`as!`) is used when you’re certain that the cast will succeed, and you want to obtain the value directly. However, using `as!` can lead to runtime crashes if the cast fails, making it vital to ensure the types match beforehand. Here’s how to use it:
let anotherPet: Animal = Dog() // anotherPet is of type Animal let myDogForced = anotherPet as! Dog // Forced cast myDogForced.bark() // Safe to call bark() here since we know it's a Dog
In the above example, we confidently use `as!` because we know that `anotherPet` is indeed a `Dog`. However, if `anotherPet` were not an instance of `Dog`, this would lead to a crash.
Swift’s type safety extends beyond just casting. The language enforces strict rules about data types, ensuring that you cannot inadvertently mix incompatible types. This characteristic significantly reduces the chances of runtime errors that can occur in less strictly-typed languages. For instance, trying to assign a `String` to an `Int` variable will result in a compile-time error:
let number: Int = "100" // Error: Cannot convert value of type 'String' to 'Int'
This strict type checking encourages developers to ponder critically about the types they’re using, leading to clearer and more maintainable code.
Additionally, type casting is often used with protocols. When a class conforms to a protocol, it can be cast to that protocol type. This allows for polymorphic behavior, where the same method can behave differently depending on the actual type of the object. Here’s an example:
protocol Speakable { func speak() } class Cat: Animal, Speakable { func speak() { print("Meow!") } } let myAnimal: Animal = Cat() if let speakableAnimal = myAnimal as? Speakable { speakableAnimal.speak() // Calls the speak() method }
In this case, we check if `myAnimal` conforms to the `Speakable` protocol. If it does, we call the `speak()` method, demonstrating how type casting can lead to flexible and reusable code.
Swift’s approach to type casting and type safety allows developers to write robust and predictable code. By understanding and effectively using `as?` and `as!`, you can navigate complex class hierarchies and protocols safely, enhancing the overall quality of your Swift applications.
Custom Data Types: Structs and Classes
In Swift, custom data types play a significant role in organizing and structuring code, enabling developers to create more complex and meaningful representations of data. The two primary constructs for creating custom data types are structs and classes. Both allow encapsulation of data and behavior, but they come with distinct characteristics that influence their usage in different scenarios.
Structs are value types, which means that when you assign or pass them around in your code, you are working with copies rather than references. This characteristic provides a certain level of safety, as modifying one instance does not affect another. Structs are particularly useful for modeling simple data structures or when you want to ensure immutability. Here’s an example of a struct in Swift:
struct Point { var x: Double var y: Double func distance(to other: Point) -> Double { return ((x - other.x) * (x - other.x) + (y - other.y) * (y - other.y)).squareRoot() } } let pointA = Point(x: 3.0, y: 4.0) let pointB = Point(x: 6.0, y: 8.0) let distance = pointA.distance(to: pointB) print("Distance: (distance)") // Output: Distance: 5.0
In the above example, the Point
struct encapsulates two properties, x
and y
, representing coordinates. The method distance(to:)
computes the distance to another point. This showcases the encapsulation of functionality within the data structure itself.
Classes, on the other hand, are reference types. When you create an instance of a class and assign it to a variable, you are dealing with a reference to the original instance. This means that multiple variables can refer to the same instance, and modifications through one reference will reflect in all others. This behavior is advantageous for complex systems where you want shared mutable state. Here’s a simple example of a class:
class Circle { var radius: Double init(radius: Double) { self.radius = radius } func area() -> Double { return .pi * radius * radius } } let circleA = Circle(radius: 5.0) let circleB = circleA // circleB references the same instance as circleA circleB.radius = 10.0 // Modifying circleB also affects circleA print("Area of Circle A: (circleA.area())") // Output: Area of Circle A: 314.159
In this example, the Circle
class has a property radius
and a method to calculate the area. When circleB
is assigned to circleA
, both names refer to the same object in memory. Changes to circleB
affect circleA
, demonstrating the reference type behavior of classes.
Another important distinction between structs and classes in Swift is that structs cannot inherit from other structs, while classes can inherit from other classes, allowing for a more extensive hierarchy of behavior and properties. This inheritance enables polymorphism, where different subclasses can override methods to provide specific implementations.
Choosing between structs and classes often comes down to the specific requirements of your application. If you need to model simple data types that are primarily immutable, structs might be the better choice. Conversely, for more complex data structures that require inheritance or shared state, classes are typically more suitable. Swift’s design encourages developers to think critically about these choices, leading to clearer and more maintainable code.
Ultimately, both structs and classes play integral roles in Swift programming, allowing developers to tailor data representation to their specific needs while using Swift’s robust type system to ensure safety and efficiency.