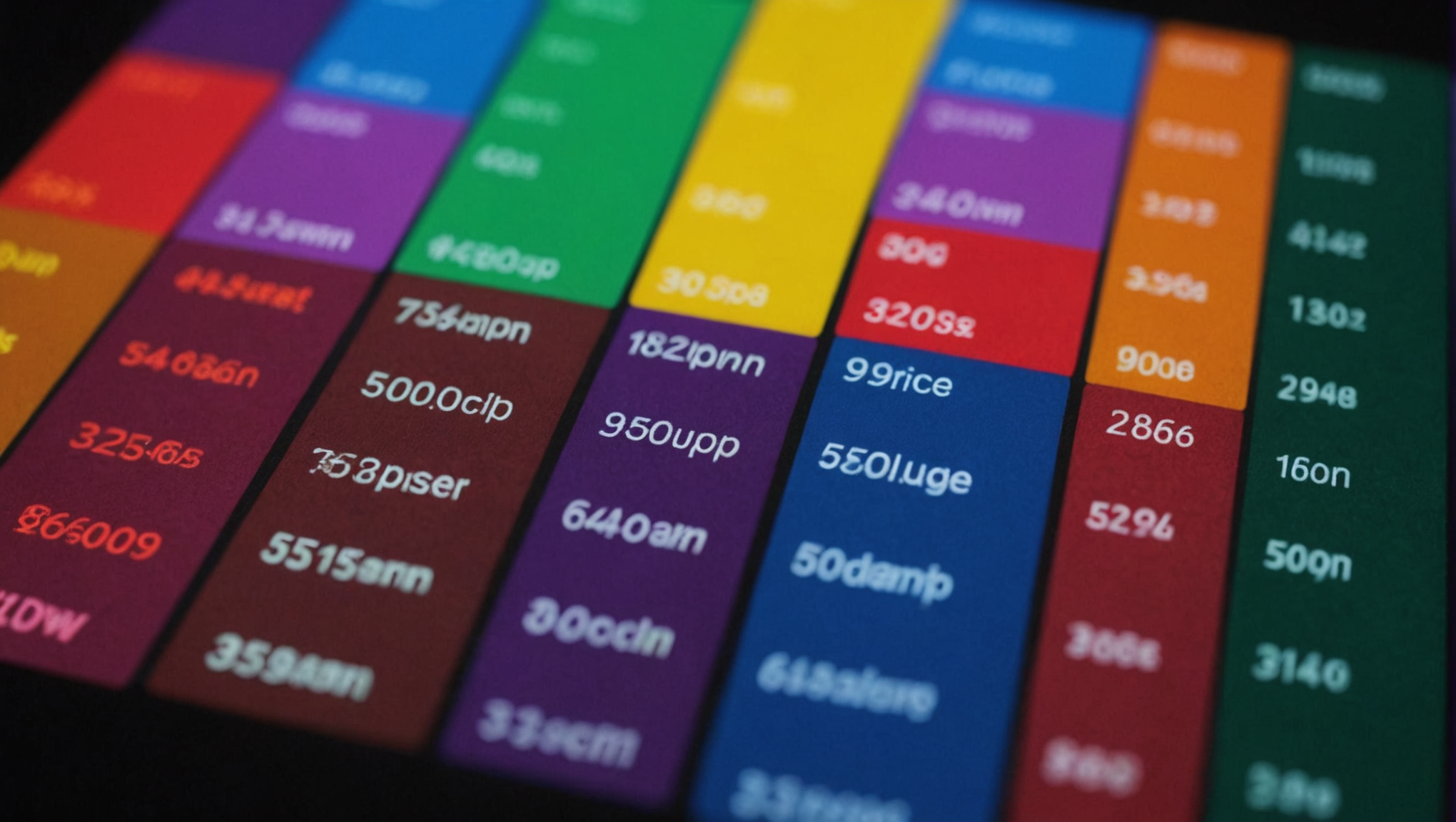
JavaScript and Data Visualization
Data visualization is an essential skill in the realm of data science and web development, enabling developers to transform complex datasets into accessible and understandable visual formats. At its core, data visualization involves the use of graphical representations to communicate information clearly and efficiently. This process not only helps in identifying trends, patterns, and outliers within the data but also facilitates a more intuitive understanding of underlying truths.
To grasp the fundamentals of data visualization, one must consider the following key principles:
1. Clarity and Simplicity: Visualizations should be simpler, avoiding unnecessary embellishments that may distract from the data’s message. This means choosing the right type of chart or graph that conveys the data effectively.
2. Choosing the Right Graph: Each type of graph serves a different purpose. For example, line charts are perfect for showing trends over time, while bar charts are better suited for comparing quantities across categories. Understanding these distinctions is important for effective data representation.
3. Use of Color: Color can play a significant role in data visualization, helping to differentiate between data sets or emphasizing key points. However, one must be cautious to use colors that are accessible and do not mislead the viewer.
4. Contextual Information: Providing context helps viewers interpret the data accurately. Titles, labels, and legends are essential components that offer crucial information about what the viewer is observing.
5. Interactivity: State-of-the-art data visualizations increasingly incorporate interactivity, allowing users to explore the data more thoroughly. Interactivity can range from simple hover effects that reveal additional data points to complex filtering systems that enable users to customize what they see.
To illustrate these principles, think the following example using JavaScript to create a simple bar chart with the canvas
element:
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); const data = [12, 19, 3, 5, 2, 3]; const labels = ['Red', 'Blue', 'Yellow', 'Green', 'Purple', 'Orange']; const barWidth = 40; const gap = 10; data.forEach((value, index) => { const x = index * (barWidth + gap); const y = canvas.height - value * 10; // Scaling the value for visualization ctx.fillStyle = labels[index]; // Using color based on label ctx.fillRect(x, y, barWidth, value * 10); // Drawing the bar });
In this example, we create a simple bar chart on an HTML canvas. The data array holds the values to be represented, while the labels array corresponds to the colors. Each bar’s height is scaled appropriately to visualize relative differences in data values.
Understanding these fundamentals of data visualization is critical for anyone looking to effectively communicate insights through data. Mastering these principles will lay a solid foundation for using more advanced JavaScript libraries and techniques in data visualization.
Popular JavaScript Libraries for Data Visualization
When it comes to bringing data visualizations to life in JavaScript, a myriad of libraries exist to facilitate the process. Each library has its strengths, catering to various needs and preferences of developers. Below, we explore some of the most popular JavaScript libraries for data visualization, each offering unique capabilities and advantages.
D3.js (Data-Driven Documents) is one of the most powerful and flexible libraries for creating complex data visualizations. D3 allows developers to bind arbitrary data to a Document Object Model (DOM) and then apply data-driven transformations to the document. This approach provides a high degree of control over the final output, enabling the creation of intricate visualizations such as custom graphs, interactive charts, and even dynamic data-driven interfaces. Here’s a simple example of creating a bar chart using D3.js:
const data = [10, 15, 20, 25, 30, 35]; const width = 400; const height = 200; const svg = d3.select("body").append("svg") .attr("width", width) .attr("height", height); const xScale = d3.scaleBand() .domain(data.map((d, i) => i)) .range([0, width]) .padding(0.1); const yScale = d3.scaleLinear() .domain([0, d3.max(data)]) .range([height, 0]); svg.selectAll(".bar") .data(data) .enter() .append("rect") .attr("class", "bar") .attr("x", (d, i) => xScale(i)) .attr("y", d => yScale(d)) .attr("width", xScale.bandwidth()) .attr("height", d => height - yScale(d)) .attr("fill", "steelblue");
Another noteworthy library is Chart.js, which is particularly well-suited for developers who need to implement quick and simpler data representation without complex configurations. Chart.js provides a simple API to create beautiful charts with minimal effort. With built-in support for various chart types, including line, bar, radar, and pie charts, it’s an excellent choice for developers who prioritize ease of use and speed. Here’s how to create a line chart using Chart.js:
const ctx = document.getElementById('myChart').getContext('2d'); const myChart = new Chart(ctx, { type: 'line', data: { labels: ['January', 'February', 'March', 'April', 'May', 'June'], datasets: [{ label: 'Sales', data: [120, 190, 30, 50, 20, 30], borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1, fill: false }] }, options: { scales: { y: { beginAtZero: true } } } });
For developers looking to produce visually appealing and interactive charts quickly, both D3.js and Chart.js stand out as leading libraries in the JavaScript ecosystem. While D3.js excels in flexibility and customizability, Chart.js shines in its simplicity and ease of integration. Choosing the right library ultimately depends on the specific requirements of the project and the developer’s familiarity with the tools.
Lastly, Plotly.js deserves mention for its capability to create both 2D and 3D visualizations with ease. It offers built-in interactivity and a wide range of chart types, making it suitable for data analysis and robust visual storytelling. Additionally, libraries like Highcharts and Vis.js are often used for their rich feature sets, catering to diverse visualization needs from simple graphs to complex network diagrams.
As you explore the realm of data visualization with JavaScript, the choice of library can significantly impact your workflow and the final output. Each library has its unique attributes, and understanding these will help you select the best fit for your data visualization needs.
Creating Interactive Graphs with D3.js
const data = [10, 15, 20, 25, 30, 35]; const width = 400; const height = 200; const svg = d3.select("body").append("svg") .attr("width", width) .attr("height", height); const xScale = d3.scaleBand() .domain(data.map((d, i) => i)) .range([0, width]) .padding(0.1); const yScale = d3.scaleLinear() .domain([0, d3.max(data)]) .range([height, 0]); svg.selectAll(".bar") .data(data) .enter() .append("rect") .attr("class", "bar") .attr("x", (d, i) => xScale(i)) .attr("y", d => yScale(d)) .attr("width", xScale.bandwidth()) .attr("height", d => height - yScale(d)) .attr("fill", "steelblue");
D3.js stands out not just for its capabilities but also for its versatility in crafting interactive visualizations. The power of D3.js lies in its ability to manipulate the Document Object Model (DOM) based on data. It allows developers to bind data to elements, enabling transformations that can animate, transition, and respond to user interactions. As you explore D3.js, consider how it leverages selections, data binding, and enter-update-exit patterns to create rich, interactive visual experiences.
Consider a more interactive example where we can respond to user actions, such as hovering over bars to display their values dynamically. Here’s how to incorporate interactivity:
svg.selectAll(".bar") .data(data) .enter() .append("rect") .attr("class", "bar") .attr("x", (d, i) => xScale(i)) .attr("y", d => yScale(d)) .attr("width", xScale.bandwidth()) .attr("height", d => height - yScale(d)) .attr("fill", "steelblue") .on("mouseover", function(event, d) { d3.select(this).transition() .duration(200) .attr("fill", "orange"); svg.append("text") .attr("class", "label") .attr("x", parseFloat(d3.select(this).attr("x")) + xScale.bandwidth() / 2) .attr("y", parseFloat(d3.select(this).attr("y")) - 5) .attr("text-anchor", "middle") .text(d); }) .on("mouseout", function() { d3.select(this).transition() .duration(200) .attr("fill", "steelblue"); svg.selectAll(".label").remove(); });
In this enhanced example, we listen for mouse events on each bar. Upon hovering, we change the bar’s color and display its value above the bar. The transition effects provide a smooth visual cue, making the interaction feel more engaging.
The opportunity for interactivity extends to more complex visualizations where you might include features like filters, tooltips, or zooming capabilities. D3.js provides a robust API for such functionalities, enabling you to create a seamless experience for users as they explore the data.
As you become more adept with D3.js, you’ll find yourself not just creating static representations of data but rather engaging narratives that can transform raw numbers into compelling stories. The key is to embrace the capabilities of D3.js to create not only informative but also interactive experiences that captivate your audience and convey insights effectively.
Using Chart.js for Quick Data Representation
const ctx = document.getElementById('myChart').getContext('2d'); const myChart = new Chart(ctx, { type: 'bar', data: { labels: ['January', 'February', 'March', 'April', 'May', 'June'], datasets: [{ label: 'Monthly Sales', data: [120, 190, 30, 50, 20, 30], backgroundColor: [ 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', 'rgba(75, 192, 192, 0.2)', 'rgba(153, 102, 255, 0.2)', 'rgba(255, 159, 64, 0.2)', ], borderColor: [ 'rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', 'rgba(75, 192, 192, 1)', 'rgba(153, 102, 255, 1)', 'rgba(255, 159, 64, 1)', ], borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } }, responsive: true, maintainAspectRatio: false } });
Chart.js emerges as a formidable ally for developers looking to implement quick data representation without the steep learning curve associated with some of the more complex libraries. This library operates on the principle of simplicity, allowing developers to create aesthetically pleasing charts with minimal configuration and effort.
To illustrate the capabilities of Chart.js, let’s delve into another common scenario: creating a pie chart that provides an overview of data distribution across categories. The following example demonstrates how to set up and render a pie chart using Chart.js:
const ctx = document.getElementById('myPieChart').getContext('2d'); const myPieChart = new Chart(ctx, { type: 'pie', data: { labels: ['Red', 'Blue', 'Yellow'], datasets: [{ label: 'Votes', data: [12, 19, 3], backgroundColor: [ 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', ], borderColor: [ 'rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', ], borderWidth: 1 }] }, options: { responsive: true, maintainAspectRatio: false } });
This pie chart example succinctly demonstrates how to visualize categorical data. By simply defining the data and its corresponding labels, developers can quickly generate a visual representation that conveys the distribution of votes across different categories.
Moreover, Chart.js incorporates responsive design principles out of the box, ensuring that charts adapt seamlessly to various screen sizes. This feature is particularly beneficial in today’s multi-device landscape, where accessibility and usability are paramount.
For developers who require more than just static displays, Chart.js supports a high number of options for interactivity and animation, allowing for a richer user experience. You can easily configure animations to enhance the visual impact of your charts, further engaging users with dynamic transitions and effects.
const myAnimatedChart = new Chart(ctx, { type: 'bar', data: { labels: ['Red', 'Blue', 'Yellow'], datasets: [{ label: 'Votes', data: [12, 19, 3], backgroundColor: ['rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)'], borderColor: ['rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)'], borderWidth: 1 }] }, options: { animation: { duration: 1000, easing: 'easeInOutQuad' } } });
In this animated chart example, we can see how Chart.js facilitates user engagement through smooth transitions that occur when the chart is rendered. This type of feature not only makes the visualizations more attractive but also improves the overall user experience by adding a layer of dynamism.
Ultimately, Chart.js serves as an excellent choice for developers aiming to achieve a balance between ease of use and visual appeal. By using this library, you can quickly bring your data to life, allowing for effective communication of insights with minimal effort.
Integrating Data Sources for Dynamic Visualizations
Integrating data sources for dynamic visualizations is a pivotal step in creating responsive and interactive data experiences. With the increasing availability of APIs and data endpoints, developers can seamlessly fetch data and update visualizations in real time. This capability not only enhances the user experience but also adds significant value by ensuring that the visualizations reflect the most current data available.
One of the most simpler methods to integrate dynamic data into your visualizations is by using the fetch API for asynchronous data retrieval. Ponder a scenario where you want to visualize sales data pulled from a remote server. Here’s how you might implement this using JavaScript and D3.js:
fetch('https://api.example.com/salesdata') .then(response => response.json()) .then(data => { const svg = d3.select("body").append("svg") .attr("width", 400) .attr("height", 200); const xScale = d3.scaleBand() .domain(data.map((d, i) => i)) .range([0, 400]) .padding(0.1); const yScale = d3.scaleLinear() .domain([0, d3.max(data, d => d.sales)]) .range([200, 0]); svg.selectAll(".bar") .data(data) .enter() .append("rect") .attr("class", "bar") .attr("x", (d, i) => xScale(i)) .attr("y", d => yScale(d.sales)) .attr("width", xScale.bandwidth()) .attr("height", d => 200 - yScale(d.sales)) .attr("fill", "steelblue"); }) .catch(error => console.error('Error fetching data:', error));
In this example, we initiate a fetch request to an API endpoint that returns sales data in JSON format. Upon receiving the data, we create an SVG canvas and draw a bar chart using the values obtained from the API. The dynamic aspect comes from the fact that the chart will update according to the latest data provided by the server.
Another method for integrating data sources is through the use of libraries like Chart.js, which also supports dynamic updates. Here’s an example of how you can update a chart with new data at regular intervals:
const ctx = document.getElementById('myChart').getContext('2d'); const myChart = new Chart(ctx, { type: 'bar', data: { labels: ['January', 'February', 'March', 'April', 'May', 'June'], datasets: [{ label: 'Sales', data: [120, 190, 30, 50, 20, 30], backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } } } }); // Function to update chart data dynamically function updateChart() { fetch('https://api.example.com/dynamicdata') .then(response => response.json()) .then(newData => { myChart.data.datasets[0].data = newData; myChart.update(); }) .catch(error => console.error('Error fetching new data:', error)); } // Update chart every 5 seconds setInterval(updateChart, 5000);
Here, we define a function updateChart that fetches new data from an API and updates the Chart.js instance accordingly. This allows the chart to reflect the latest information without requiring a full page reload, creating a more engaging experience for users.
Integrating data sources can also be extended to various formats, such as CSV files or even streaming data from WebSockets. The key takeaway is that the ability to dynamically update visualizations using real-time data not only enhances interactivity but also increases the relevance and impact of the insights being presented. As you explore these integration techniques, consider the implications of data accuracy, loading times, and user experience to create seamless and informative data visualizations.
Best Practices for Effective Data Visualization in JavaScript
When it comes to creating effective data visualizations in JavaScript, adhering to best practices is essential for ensuring that your visualizations not only convey the intended message but also engage and inform your audience. Here are some key principles to consider:
1. Know Your Audience: Understanding who will be viewing your visualizations very important. Think their level of expertise, interests, and what they hope to gain from the data presented. Tailoring your visualizations to the audience can greatly enhance comprehension and engagement.
2. Use Appropriate Visual Representations: Different types of data require different visualization techniques. A pie chart may suffice for simple proportions, but a line chart is more effective for showing trends over time. It is essential to choose the right chart type that aligns with the story you want to tell through your data.
3. Maintain Visual Balance: A well-balanced visualization is more appealing and easier to interpret. Ensure that your visual elements are distributed evenly and that no single aspect dominates the visualization. This includes sizing, spacing, and the color palette used.
4. Limit the Data Presented: Presenting too much data concurrently can overwhelm viewers. Instead, focus on key insights and limit the amount of information shown in a single visualization. This approach aids clarity and helps the audience grasp the essential points more quickly.
5. Enhance Accessibility: Ensure your visualizations are accessible to all users, including those with disabilities. Ponder color contrast, use of text labels, and alternative text for images. Tools like ARIA (Accessible Rich Internet Applications) can help make your visualizations more inclusive.
6. Incorporate Interactive Elements Wisely: While interactivity can enhance user engagement, it should not come at the expense of clarity. Features like tooltips, zooming, and filtering should enhance the user experience without overwhelming the viewer with too many options.
To illustrate some of these best practices in action, let’s ponder a simple scatter plot using D3.js that demonstrates the correlation between two variables:
const data = [ {x: 5, y: 10}, {x: 15, y: 30}, {x: 25, y: 20}, {x: 35, y: 40}, {x: 45, y: 60}, {x: 55, y: 35} ]; const width = 500, height = 300; const svg = d3.select("body").append("svg") .attr("width", width) .attr("height", height); const xScale = d3.scaleLinear() .domain([0, d3.max(data, d => d.x)]) .range([0, width]); const yScale = d3.scaleLinear() .domain([0, d3.max(data, d => d.y)]) .range([height, 0]); svg.selectAll("circle") .data(data) .enter() .append("circle") .attr("cx", d => xScale(d.x)) .attr("cy", d => yScale(d.y)) .attr("r", 5) .attr("fill", "steelblue") .on("mouseover", function(event, d) { d3.select(this).attr("fill", "orange"); svg.append("text") .attr("x", xScale(d.x)) .attr("y", yScale(d.y) - 10) .attr("text-anchor", "middle") .text(`(${d.x}, ${d.y})`); }) .on("mouseout", function() { d3.select(this).attr("fill", "steelblue"); svg.selectAll("text").remove(); });
This example demonstrates several best practices: it utilizes clear and simple circles to represent data points, interactive tooltips to display information on hover, and a limited number of data points to maintain clarity. Additionally, by using appropriate scales and axes, the scatter plot effectively communicates the relationship between the two variables.
Ultimately, effective data visualization in JavaScript hinges on a combination of technical skills and a deep understanding of the principles that underpin good design. By following these best practices, developers can create compelling visual narratives that not only inform but also inspire action among their audiences.
Exploring data visualization opens up new dimensions for storytelling. I’d like to add that while discussing popular libraries like D3.js and Chart.js, considering their performance in handling large datasets especially important. As data grows in size and complexity, performance optimizations—like using Canvas for rendering in D3.js or lazy loading data in Chart.js—can significantly enhance user experience. This practical aspect ensures that visualizations maintain responsiveness and clarity even when scaled up, empowering developers to create dynamic and fluid visual experiences.