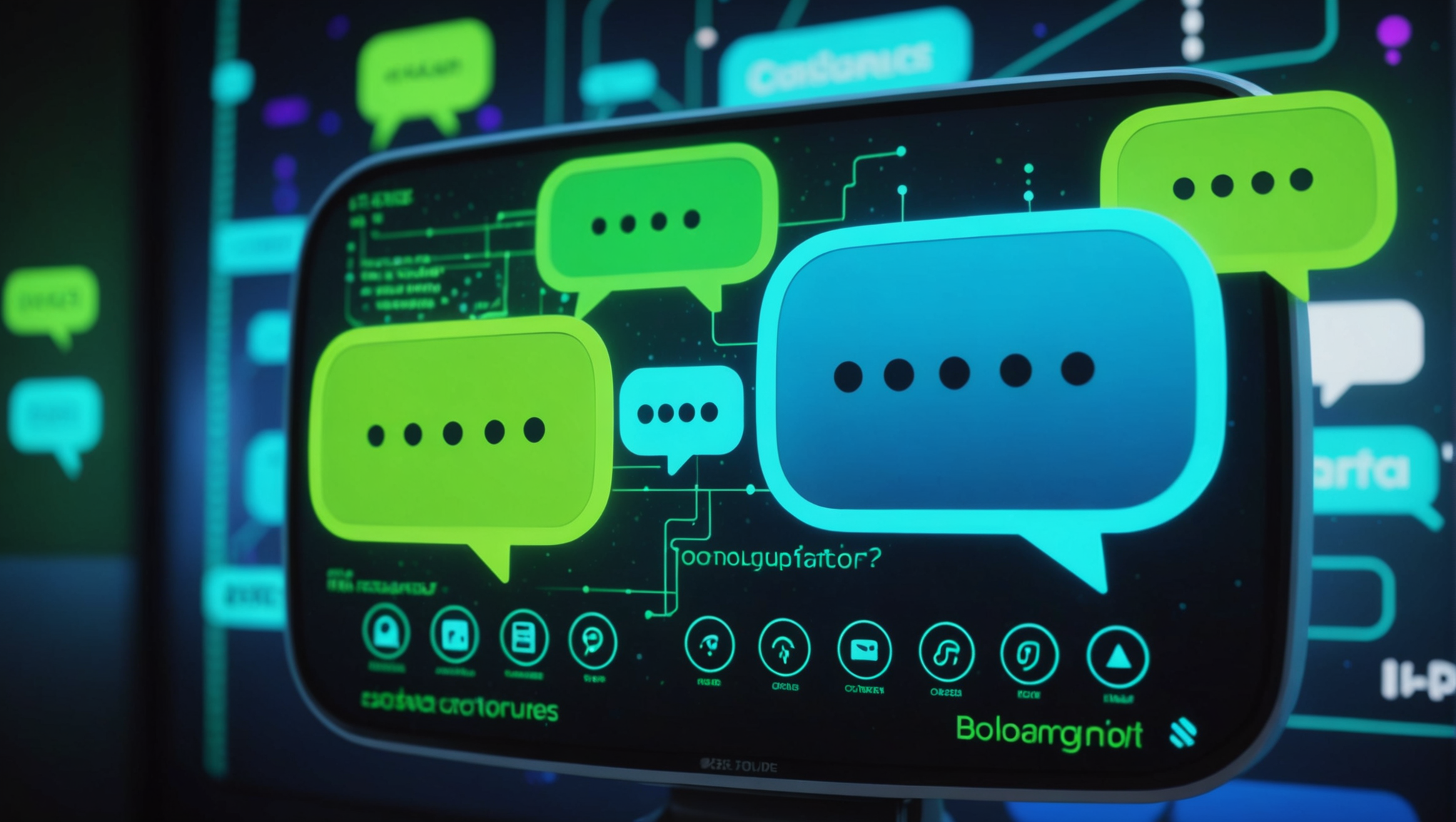
Python and Natural Language Processing
Natural Language Processing (NLP) is a fascinating intersection of computer science, artificial intelligence, and linguistics. At its core, NLP enables machines to understand, interpret, and respond to human language in a valuable way. It bridges the gap between human communication and computer understanding, turning the complexities of natural language—with its nuances and variability—into forms that machines can process. The challenges are manifold: from parsing text and identifying sentiment to generating coherent responses and extracting meaningful information from large datasets.
To truly grasp NLP, one must appreciate how language encompasses various levels of structure, including phonetics, syntax, semantics, and pragmatics. Each level presents its own set of challenges. For instance, consider the sentence: “I can’t wait to see her!”. The task of determining the sentiment behind this expression—whether positive or negative—requires an understanding not just of the words but of their context.
Python has emerged as the go-to language for many NLP tasks, thanks to its simplicity and the rich ecosystem of libraries that support various NLP functionalities. The beauty of Python lies not just in its syntax but also in the powerful libraries that make sophisticated tasks accessible to developers of all skill levels.
When processing natural language, one of the fundamental requirements is tokenization—the process of breaking down text into discrete units, such as words or sentences. For example, the sentence “Natural Language Processing is amazing!” can be split into tokens: [“Natural”, “Language”, “Processing”, “is”, “amazing”].
from nltk.tokenize import word_tokenize text = "Natural Language Processing is amazing!" tokens = word_tokenize(text) print(tokens) # Output: ['Natural', 'Language', 'Processing', 'is', 'amazing', '!']
Beyond tokenization, understanding the grammar of language is essential. Syntax trees can help visualize the structure of sentences, identifying subjects, verbs, and objects. This structural understanding is vital for tasks like translation and summarization.
Another critical aspect is the representation of words. Techniques such as Word Embeddings allow words to be represented as high-dimensional vectors, capturing semantic meanings and relationships. For instance, the words “king” and “queen” would be close to each other in this vector space, reflecting their related meanings.
from gensim.models import Word2Vec sentences = [["natural", "language", "processing"], ["machine", "learning"], ["deep", "learning"]] model = Word2Vec(sentences, vector_size=100, window=5, min_count=1, workers=4) vector_king = model.wv['king'] vector_queen = model.wv['queen'] print(vector_king, vector_queen)
NLP is a rich and complex field, with Python serving as a powerful tool for tackling its challenges. As we delve deeper into this domain, we will explore the libraries and techniques that make Python an indispensable ally in the quest for machines that can understand and generate human language.
Key Python Libraries for NLP
When it comes to Natural Language Processing in Python, several key libraries stand out for their functionality, flexibility, and ease of use. Each of these libraries offers unique features that cater to different aspects of NLP, enabling developers to choose the best tools for their specific tasks. Here, we will explore some of the most widely used libraries in this domain.
NLTK (Natural Language Toolkit) is one of the oldest and most comprehensive libraries for NLP in Python. It provides tools for text processing tasks, including classification, tokenization, stemming, tagging, parsing, and semantic reasoning. NLTK is particularly useful for educational purposes due to its extensive documentation and tutorials. For example, you can easily create a simple frequency distribution of words in a text as follows:
from nltk import FreqDist from nltk.tokenize import word_tokenize text = "Natural Language Processing is amazing. Natural language is a fascinating field." tokens = word_tokenize(text) fdist = FreqDist(tokens) print(fdist.most_common(5)) # Output: [('Natural', 2), ('Language', 2), ('Processing', 1), ('is', 2), ('amazing.', 1)]
spaCy is another powerful NLP library designed for performance and ease of use. It’s built specifically for production use cases and offers state-of-the-art pre-trained models for various languages. One of its standout features is its ability to handle large volumes of text efficiently. Here’s how you can use spaCy to perform named entity recognition:
import spacy nlp = spacy.load("en_core_web_sm") doc = nlp("Apple is looking at buying U.K. startup for $1 billion.") for entity in doc.ents: print(entity.text, entity.label_) # Output: Apple ORG, U.K. GPE, $1 billion MONEY
gensim focuses on topic modeling and document similarity analysis. Its capability to work with large text corpora and perform unsupervised learning makes it a go-to library for tasks such as building word vectors and training topic models. Here’s how to create a simple TF-IDF model using gensim:
from gensim import corpora, models documents = ["Human machine interaction", "Natural language processing in machines"] text_data = [[word for word in doc.lower().split()] for doc in documents] dictionary = corpora.Dictionary(text_data) corpus = [dictionary.doc2bow(text) for text in text_data] tfidf = models.TfidfModel(corpus) for doc in tfidf[corpus]: print(doc) # Output: List of TF-IDF tuples for each document
Transformers, developed by Hugging Face, allows for the use of cutting-edge deep learning models for NLP, such as BERT and GPT. The library provides a simple interface for using these models for various tasks, including text classification, summarization, and question answering. Here’s how you can quickly use a pre-trained model for text classification:
from transformers import pipeline classifier = pipeline("sentiment-analysis") result = classifier("I love programming in Python!") print(result) # Output: [{'label': 'POSITIVE', 'score': 0.9998}]
Each of these libraries plays a vital role in the NLP landscape, offering diverse functionalities that enable developers to tackle various challenges in processing and understanding human language. By using these tools, you can build robust NLP applications that can interpret and generate text effectively.
Text Preprocessing Techniques
Text preprocessing is an important step in the Natural Language Processing (NLP) pipeline. Before diving into more complex analyses and model building, the raw text data must be cleaned and prepared appropriately. This process involves several techniques aimed at transforming text into a format that’s easier for machine learning algorithms to work with.
One of the most common preprocessing techniques is lowercasing, where all text is converted to lowercase to ensure uniformity. This step helps in reducing the vocabulary size, making it easier to analyze the data. Here’s how you can achieve this using Python:
text = "Natural Language Processing Is Amazing!" cleaned_text = text.lower() print(cleaned_text) # Output: natural language processing is amazing!
Another essential preprocessing technique is removing punctuation. Punctuation marks can often be irrelevant in the context of text analysis, especially in tasks like sentiment analysis or topic modeling. You can easily remove punctuation using Python’s string library:
import string text = "Natural Language Processing, is amazing! Isn't it?" cleaned_text = text.translate(str.maketrans('', '', string.punctuation)) print(cleaned_text) # Output: Natural Language Processing is amazing Isnt it
Following punctuation removal, tokenization comes into play, where the cleaned text is split into individual words or tokens. This is foundational for most NLP tasks and can be accomplished using NLTK or other libraries:
from nltk.tokenize import word_tokenize text = "Natural Language Processing is amazing!" tokens = word_tokenize(text) print(tokens) # Output: ['Natural', 'Language', 'Processing', 'is', 'amazing', '!']
Next, stop words removal aids in eliminating common words that typically add little meaning to the context, such as “and,” “the,” and “is.” Removing these words can significantly enhance the performance of NLP models. Here’s how you can remove stop words using NLTK:
from nltk.corpus import stopwords stop_words = set(stopwords.words('english')) filtered_tokens = [word for word in tokens if word.lower() not in stop_words] print(filtered_tokens) # Output: ['Natural', 'Language', 'Processing', 'amazing', '!']
Another valuable technique is stemming or lemmatization, which reduces words to their root forms. Stemming is a more aggressive approach that may not always yield real words, while lemmatization considers the context and converts words to their base or dictionary form. Here’s an example of lemmatization using NLTK:
from nltk.stem import WordNetLemmatizer lemmatizer = WordNetLemmatizer() lemmatized_tokens = [lemmatizer.lemmatize(token) for token in filtered_tokens] print(lemmatized_tokens) # Output: ['Natural', 'Language', 'Processing', 'amazing', '!']
Finally, vectorization transforms textual data into numerical format, making it compatible with machine learning algorithms. Common techniques for vectorization include Bag of Words (BoW) and Term Frequency-Inverse Document Frequency (TF-IDF). Here’s a simple implementation of TF-IDF using scikit-learn:
from sklearn.feature_extraction.text import TfidfVectorizer documents = ["Natural Language Processing is amazing.", "I love programming in Python."] vectorizer = TfidfVectorizer() tfidf_matrix = vectorizer.fit_transform(documents) print(tfidf_matrix.toarray()) # Output: TF-IDF matrix representation
By applying these preprocessing techniques, you can significantly enhance the quality of your text data, paving the way for more accurate and meaningful insights during analysis and modeling. Preprocessing sets the stage for building effective NLP models, enabling machines to better understand and process human language.
Building NLP Models with Python
import numpy as np from sklearn.model_selection import train_test_split from sklearn.metrics import classification_report from sklearn.naive_bayes import MultinomialNB from sklearn.feature_extraction.text import TfidfVectorizer # Sample dataset documents = [ "I love programming in Python.", "Python is great for data science!", "I dislike debugging code.", "Natural language processing with Python is fascinating.", "I enjoy solving algorithms." ] labels = [1, 1, 0, 1, 1] # 1: positive, 0: negative # Split the dataset into training and testing sets X_train, X_test, y_train, y_test = train_test_split(documents, labels, test_size=0.2, random_state=42) # Vectorization using TF-IDF vectorizer = TfidfVectorizer() X_train_tfidf = vectorizer.fit_transform(X_train) X_test_tfidf = vectorizer.transform(X_test) # Building a model using Naive Bayes model = MultinomialNB() model.fit(X_train_tfidf, y_train) # Making predictions y_pred = model.predict(X_test_tfidf) # Evaluating the model print(classification_report(y_test, y_pred))
Building NLP models with Python is not just about implementing algorithms; it involves understanding the data, choosing the right model, and fine-tuning it to achieve the best results. Once the preprocessing of text data is complete, you can then proceed to build various types of models depending on the NLP task at hand, including classification, regression, and even generative models.
For instance, when tackling a sentiment analysis problem, a simple yet effective approach is to use a Naive Bayes classifier, which works well for text classification tasks. The code snippet above demonstrates how to train a Naive Bayes model on a small dataset after converting the text to a TF-IDF representation.
In the code, we first create a sample dataset consisting of a few sentences with corresponding labels indicating whether the sentiment is positive or negative. We then split this dataset into training and testing sets, which is vital for evaluating the performance of our model.
After splitting the data, we apply the TF-IDF vectorization technique to transform our text data into a format suitable for the model. This approach captures the importance of each word in the context of the documents, allowing the model to learn more effectively.
Once the model is trained, we can make predictions on the test set and evaluate the model’s performance using metrics like precision, recall, and F1-score. Such evaluations are crucial for understanding how well your model performs and where it might need improvement.
Other models can also be employed depending on the complexity of the task, such as Support Vector Machines (SVM), Random Forests, or even deep learning models like Recurrent Neural Networks (RNNs) and Transformers. Libraries like scikit-learn, TensorFlow, and PyTorch offer robust support for building and training these models.
Moreover, tracking and tuning hyperparameters becomes essential as you progress in model building. Techniques such as Grid Search or Random Search can be employed to find the optimal settings for your models, significantly enhancing their performance.
As you delve deeper into building NLP models, you’ll find that the combination of an effective preprocessing pipeline, the right choice of model, and diligent tuning can result in powerful applications capable of understanding and generating human language with impressive accuracy.
Applications of NLP in Real-World Scenarios
The applications of Natural Language Processing (NLP) in the real world are vast and varied, reflecting the growing importance of language understanding in technology. NLP has revolutionized sectors like customer service, healthcare, marketing, and education by providing tools that automate and enhance human communication. Let’s dive into some concrete applications that illustrate the transformative power of NLP.
One of the most prevalent applications of NLP is in chatbots and virtual assistants. Companies leverage NLP to create sophisticated customer service solutions that can understand and respond to user inquiries in real-time. For instance, a simple chatbot can be built using the Python library NLTK to handle customer queries about product details:
from nltk.chat.util import Chat, reflections pairs = [ (r'hi|hello|hey', ['Hello!', 'Hi there!']), (r'what is your name?', ['I am a chatbot created to assist you.']), (r'how can I help you?', ['You can ask me questions about our products.']), (r'quit', ['Bye! Take care!']) ] chatbot = Chat(pairs, reflections) chatbot.converse()
This chatbot can engage users and provide answers, significantly reducing the workload for human customer service representatives.
Another critical application lies in sentiment analysis, which allows businesses to gauge public opinion about their brand, products, or services. By analyzing customer reviews or social media posts, companies can understand how their audience feels. Here’s how you can perform sentiment analysis using the Transformers library:
from transformers import pipeline sentiment_analyzer = pipeline("sentiment-analysis") reviews = [ "I absolutely love the product!", "This is the worst experience I've ever had." ] results = sentiment_analyzer(reviews) for review, result in zip(reviews, results): print(f"Review: {review}nSentiment: {result['label']} (Score: {result['score']})n")
This code snippet processes a list of customer reviews and identifies the sentiment associated with each, enabling companies to take necessary actions based on customer feedback.
NLP is also pivotal in information extraction, where it helps in automatically retrieving structured information from unstructured text. For example, extracting company names, dates, and monetary values from news articles can be done using named entity recognition (NER). The spaCy library excels in this area:
import spacy nlp = spacy.load("en_core_web_sm") text = "Apple is acquiring a startup in the UK for $1 billion." doc = nlp(text) for entity in doc.ents: print(entity.text, entity.label_)
This capability allows organizations to keep track of relevant business activities efficiently, providing insights that can inform strategic decisions.
In the realm of content generation, NLP can be leveraged to create content for websites, articles, or even social media posts. Tools like OpenAI’s GPT-3 can generate human-like text based on a prompt. Below is a simple example of generating text using the OpenAI API:
import openai openai.api_key = "your-api-key" response = openai.Completion.create( engine="text-davinci-003", prompt="Write a short introduction about the importance of AI in modern technology.", max_tokens=100 ) print(response.choices[0].text.strip())
This capability can save content creators significant time and enhance productivity by providing a starting point for various types of written content.
Another fascinating application of NLP is in language translation, which allows instant communication across different languages. Services like Google Translate utilize advanced NLP strategies to translate text from one language to another accurately. You can mimic basic translation using the `translate` module in Python:
from translate import Translator translator = Translator(to_lang="es") translation = translator.translate("Hello, how are you?") print(translation) # Output: "Hola, ¿cómo estás?"
As you can see, the applications of NLP are not just theoretical; they manifest in practical tools and systems that enhance our daily lives and business operations. Whether it’s through improving user interactions, automating content creation, or facilitating cross-linguistic communication, NLP’s impact is profound and growing in every sector.
The article provides a comprehensive view of NLP and its applications, but it could benefit from highlighting the ethical considerations surrounding NLP technologies. Issues like bias in language models, privacy concerns with user data, and the impact of automated content generation on misinformation are critical discussions that have gained traction. For a holistic understanding of NLP’s role in society, mentioning frameworks for responsible AI use and promoting transparency in model training would enhance the dialogue and awareness around these important topics.