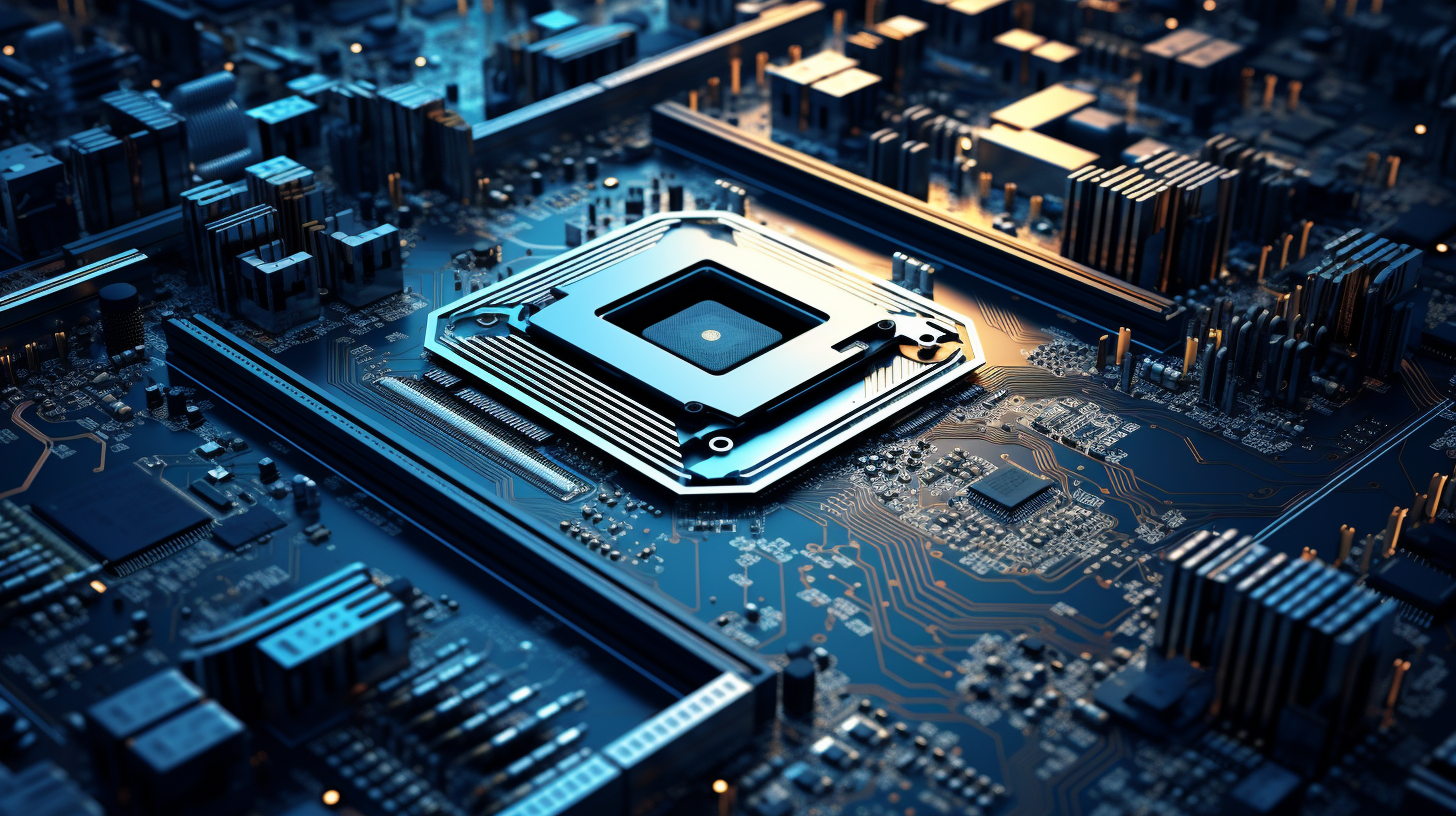
Python in Retail: Sales Analysis and Prediction
In the dynamic landscape of retail, using data analytics is not just an option; it has become a necessity for businesses aiming to thrive. Retailers are inundated with vast amounts of data encompassing everything from sales transactions to customer behavior. This treasure trove of information can be transformed into actionable insights, leading to enhanced decision-making and increased profitability.
Data analytics enables retailers to uncover patterns, identify trends, and make informed predictions. By analyzing historical sales data, businesses can discern which products are performing well and which are not, thus optimizing inventory management. Furthermore, understanding customer preferences through data can lead to tailored marketing strategies, ensuring that promotions resonate with the target audience.
To effectively harness the power of data analytics, retailers often rely on Python due to its simplicity and the robust ecosystem of libraries available. Libraries such as Pandas for data manipulation, NumPy for numerical operations, and Matplotlib for visualization play an important role in this process.
For example, think a retail dataset containing sales records. We can use Pandas to load and manipulate this data effortlessly. Below is a sample code snippet demonstrating how to read a CSV file containing sales data and perform basic analytics:
import pandas as pd # Load the sales data sales_data = pd.read_csv('sales_data.csv') # Display the first few rows of the dataset print(sales_data.head()) # Calculate total sales for each product total_sales = sales_data.groupby('product_id')['sales_amount'].sum().reset_index() print(total_sales)
This simple yet powerful example illustrates how we can start uncovering insights from our data. By grouping the sales data by product ID, we can quickly ascertain which products contribute most significantly to our revenue.
Moreover, the application of data analytics extends beyond mere sales figures. Retailers can analyze customer demographics, purchase frequency, and even seasonal trends. For instance, using Python, retailers can segment customers based on their purchasing behavior, allowing for more personalized marketing efforts.
Here’s a brief example showcasing how to segment customers based on their purchase frequency:
# Calculate purchase frequency purchase_frequency = sales_data.groupby('customer_id')['transaction_id'].count().reset_index() purchase_frequency.columns = ['customer_id', 'purchase_count'] # Segment customers purchase_frequency['segment'] = pd.cut(purchase_frequency['purchase_count'], bins=[0, 1, 3, 5, 10, float('inf')], labels=['New', 'Occasional', 'Frequent', 'Very Frequent', 'Loyal']) print(purchase_frequency)
By categorizing customers, retailers can develop targeted marketing campaigns. For example, “Loyal” customers could receive special discounts, while “New” customers might be enticed with introductory offers. The insights garnered from data analytics enable retailers to tailor their strategies effectively, fostering customer loyalty and driving sales growth.
The power of data analytics in retail cannot be overstated. By using Python and its powerful libraries, retailers can unlock valuable insights from their data, leading to enhanced operational efficiency and improved customer satisfaction. As the retail environment continues to evolve, those who master data analytics will undoubtedly stay ahead of the curve.
Building Predictive Models with Python
Building predictive models is a pivotal step in transforming insights derived from data analytics into actionable strategies in retail. Predictive modeling involves using historical data to forecast future outcomes, and Python provides an array of powerful libraries to facilitate this process. Among the most popular libraries for building predictive models are Scikit-learn, Statsmodels, and TensorFlow. Scikit-learn, in particular, is well-suited for standard machine learning tasks such as regression, classification, and clustering.
To illustrate the process of building a predictive model, let’s think a scenario where a retailer aims to predict future sales based on historical data. The first step is to prepare the data, which involves cleaning and transforming it into a suitable format for modeling. This may include handling missing values, encoding categorical variables, and scaling numerical features. Below is a code snippet demonstrating how to preprocess the data using Pandas:
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler # Load the sales data sales_data = pd.read_csv('sales_data.csv') # Handle missing values sales_data.fillna(method='ffill', inplace=True) # Convert categorical variables into dummy/indicator variables sales_data = pd.get_dummies(sales_data, columns=['product_category'], drop_first=True) # Scale numeric features scaler = StandardScaler() sales_data[['sales_amount', 'quantity_sold']] = scaler.fit_transform(sales_data[['sales_amount', 'quantity_sold']]) # Split the dataset into features and target variable X = sales_data.drop('future_sales', axis=1) # Feature set y = sales_data['future_sales'] # Target variable # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
With the data preprocessed and split into training and testing sets, we can now select a predictive model. For our sales forecasting task, a linear regression model is a good starting point. Below is an example of how to implement linear regression using Scikit-learn:
from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error, r2_score # Initialize the model model = LinearRegression() # Fit the model to the training data model.fit(X_train, y_train) # Make predictions on the testing set predictions = model.predict(X_test) # Evaluate the model's performance mse = mean_squared_error(y_test, predictions) r2 = r2_score(y_test, predictions) print(f'Mean Squared Error: {mse}') print(f'R^2 Score: {r2}') # R^2 score indicates how well the model explains the variance in the data
The performance metrics, such as Mean Squared Error (MSE) and R² score, provide insight into the model’s effectiveness. A lower MSE indicates better predictive accuracy, while an R² score closer to 1 suggests that the model captures a significant portion of the variability in the sales data.
Beyond linear regression, retailers can explore more sophisticated modeling techniques such as decision trees, random forests, or even neural networks for complex datasets. These models can capture non-linear relationships in the data, potentially leading to more accurate predictions. The flexibility of Python allows retailers to experiment with various algorithms and fine-tune their models through hyperparameter optimization.
Ultimately, the goal of building predictive models in retail is to enable data-driven decision-making. By accurately forecasting future sales, retailers can optimize inventory levels, tailor marketing strategies, and enhance customer experiences. As retail continues to evolve, using predictive analytics will be crucial for maintaining a competitive edge in the market.
Visualizing Sales Trends and Patterns
Visualizing sales trends and patterns is an essential aspect of data analytics in retail. Effective visualization aids retailers in comprehending complex datasets, identifying anomalies, and discerning trends that might otherwise be obscured in the raw data. Python, with its powerful visualization libraries such as Matplotlib and Seaborn, provides a robust toolkit for creating insightful visual representations of sales data.
To start visualizing sales trends, we can plot time series data that reflects sales over a specified period. This allows retailers to observe patterns such as seasonality and growth trends. Below is an example code snippet demonstrating how to visualize monthly sales data using Matplotlib:
import pandas as pd import matplotlib.pyplot as plt # Load the sales data sales_data = pd.read_csv('sales_data.csv') # Convert the date column to datetime format sales_data['date'] = pd.to_datetime(sales_data['date']) # Group by month and calculate total sales monthly_sales = sales_data.resample('M', on='date')['sales_amount'].sum() # Plotting the monthly sales data plt.figure(figsize=(12, 6)) plt.plot(monthly_sales.index, monthly_sales.values, marker='o', linestyle='-') plt.title('Monthly Sales Trend') plt.xlabel('Month') plt.ylabel('Total Sales Amount') plt.grid() plt.xticks(rotation=45) plt.tight_layout() plt.show()
This code creates a line chart that visualizes the total sales amount for each month. The use of markers and gridlines enhances the readability of the chart, allowing retailers to quickly identify periods of growth or decline.
In addition to time series analysis, retailers often seek to understand the relationships between different variables. Scatter plots can be utilized to explore the correlation between sales amount and various factors such as advertising spend or store location. Here’s an example of how to create a scatter plot using Seaborn:
import seaborn as sns # Load the data sales_data = pd.read_csv('sales_data.csv') # Create a scatter plot to examine the relationship between sales amount and advertising spend plt.figure(figsize=(10, 6)) sns.scatterplot(data=sales_data, x='advertising_spend', y='sales_amount', hue='product_category') plt.title('Sales Amount vs Advertising Spend') plt.xlabel('Advertising Spend') plt.ylabel('Sales Amount') plt.grid() plt.tight_layout() plt.show()
In this scatter plot, the color coding by product category helps to differentiate the sales performance across various products, offering insights into how advertising spend influences sales outcomes. Such visualizations enable retailers to make informed decisions regarding budget allocation on marketing and advertising.
Another powerful visualization technique is the heatmap, which can be used to identify patterns in sales data across different dimensions, such as time and product category. For example, a heatmap can effectively illustrate sales performance by day of the week and product category:
# Create a pivot table for heatmap pivot_table = sales_data.pivot_table(values='sales_amount', index='day_of_week', columns='product_category', aggfunc='sum') # Plotting the heatmap plt.figure(figsize=(12, 8)) sns.heatmap(pivot_table, annot=True, fmt='.0f', cmap='YlGnBu') plt.title('Sales Amount Heatmap by Day of Week and Product Category') plt.xlabel('Product Category') plt.ylabel('Day of Week') plt.tight_layout() plt.show()
This heatmap provides a visual representation of how different product categories perform on various days of the week, helping retailers identify peak sales days for specific products. Such insights can guide inventory decisions and promotional strategies, ensuring that stock levels align with anticipated demand.
Visualizing sales trends and patterns using Python’s visualization libraries not only aids in communicating insights derived from data analytics but also empowers retailers to make more informed, data-driven decisions. Whether through time series plots, scatter plots, or heatmaps, the ability to visualize complex data effectively is indispensable in the ever-evolving retail landscape.
Case Studies: Successful Implementations in Retail
In the realm of retail, the application of data analytics is not merely theoretical; it finds practical expression through a high number of case studies where businesses have effectively implemented these strategies to drive success and innovation. One such notable example is Walmart, the retail giant that has harnessed the power of predictive analytics and data-driven decision-making to streamline its operations and enhance customer satisfaction.
Walmart leverages its vast amount of transactional data, collected from millions of customers across its global stores, to gain insights into sales patterns and inventory management. For instance, by analyzing historical sales data, Walmart can forecast demand for products during key shopping periods, such as holidays or back-to-school seasons. This predictive capability allows the company to stock the right amount of inventory at the right time, minimizing excess stock and reducing out-of-stock occurrences.
An illustrative case involves the analysis of supermarket sales during the hurricane season. Walmart discovered that sales of certain products, like flashlights and batteries, surged before hurricanes struck. Armed with this insight, Walmart’s analytics team developed a predictive model that anticipated consumer demand for these products when storms approached. This proactive approach not only ensured product availability but also enhanced customer trust, as shoppers found essential items ready for purchase when they needed them the most.
Another noteworthy example comes from Target, which has famously employed predictive analytics to tailor marketing strategies and enhance customer engagement. Through analyzing purchasing data, Target identified patterns that allowed them to segment their customers based on behavior and preferences. One of the key breakthroughs was the ability to predict life events, such as pregnancy, based on customers’ buying habits.
By monitoring changes in product purchasing patterns, Target could send personalized coupons and advertisements to expecting parents, significantly increasing their marketing effectiveness. The success of this strategy dramatically improved customer loyalty and sales for the relevant product categories. In one famous incident, a father received pregnancy-related promotional materials intended for his daughter who was still in high school, leading to an unexpected revelation about her condition. This anecdote underscores the power of data analytics to uncover insights that can drive tailored marketing campaigns.
Retailers are also using advanced data analytics in e-commerce platforms. For example, Amazon employs sophisticated recommendation algorithms that analyze user behavior to suggest products tailored to individual preferences. This not only enhances the customer experience but also increases sales through upselling and cross-selling strategies. These algorithms continually learn from customer interactions, refining their recommendations over time and ensuring that the suggestions remain relevant.
Furthermore, smaller retailers are also tapping into the power of data analytics. A boutique clothing store, for instance, might analyze its sales data to identify which items are most popular among different customer demographics. Armed with this information, the store can adjust its inventory and marketing strategies to better cater to its clientele, thereby maximizing sales and minimizing unsold stock.
Ultimately, these case studies illustrate that the successful implementation of data analytics in retail is not exclusive to industry giants. Businesses of all sizes can harness these techniques to gain a competitive edge, improve operational efficiencies, and cultivate lasting relationships with their customers. As the retail landscape continues to evolve, those who adeptly integrate data analytics into their strategies will be well-positioned to navigate the complexities of the market and thrive.