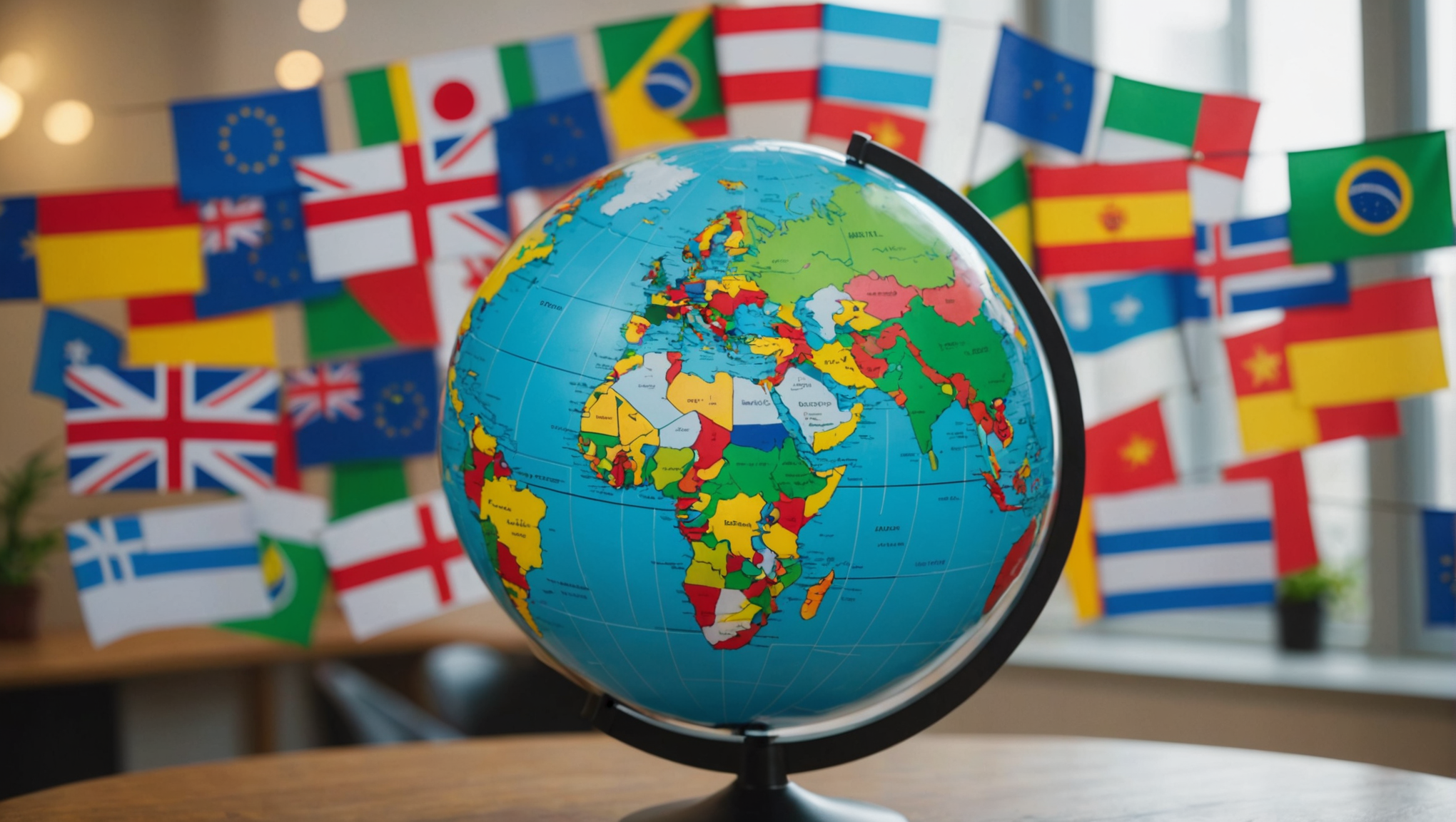
Java and Internationalization: Creating Multilingual Applications
Internationalization, often abbreviated as i18n, is the process of designing a software application so that it can be adapted to various languages and regions without engineering changes. In the realm of Java, that is a critical consideration, as the language is widely used in applications that cater to a global audience.
Java provides a robust framework for internationalization, encapsulating the complexities of different languages, cultures, and formats. At its core, internationalization is about separating the application’s core functionality from its locale-specific aspects. This enhances maintainability and scalability, allowing developers to support multiple languages and cultures with minimal effort.
One of the primary building blocks of internationalization in Java is the Locale class. A Locale
object represents a specific geographical, political, or cultural region. It is used throughout the Java API to tailor the behavior of certain classes to specific locales. Here’s a quick example:
import java.util.Locale; public class LocaleExample { public static void main(String[] args) { Locale localeUS = new Locale("en", "US"); Locale localeFR = new Locale("fr", "FR"); System.out.println("Locale: " + localeUS); System.out.println("Locale: " + localeFR); } }
In the example above, we create two Locale instances: one for the United States and another for France. This distinction is essential since the same language can have variations based on the country, affecting everything from spelling to date formats.
Java’s internationalization capabilities extend to handling text, messages, and even graphical user interfaces. Resource bundles are an important aspect of this, enabling developers to store locale-specific objects in a centralized location. By doing so, they can easily retrieve the appropriate strings and other resources based on the user’s locale.
For instance, if you have a greeting message that varies by language, you can define it in a resource bundle like so:
import java.util.ResourceBundle; public class Greeting { public static void main(String[] args) { Locale currentLocale = Locale.getDefault(); ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle", currentLocale); System.out.println(messages.getString("greeting")); } }
In this example, the ResourceBundle
retrieves the appropriate greeting based on the user’s default locale. This demonstrates how you can easily switch languages without altering the underlying code, simply by providing different resource bundles.
Moreover, the support for internationalization in Java covers more than just text. It also includes formatting for numbers, dates, and currencies, ensuring that your application behaves as expected in different regions. For example, the NumberFormat and DateFormat classes allow you to format numbers and dates according to the conventions of the user’s locale.
import java.text.NumberFormat; import java.text.DateFormat; import java.util.Date; public class FormatExample { public static void main(String[] args) { double number = 1234567.89; NumberFormat numberFormat = NumberFormat.getInstance(Locale.GERMANY); System.out.println("Formatted Number: " + numberFormat.format(number)); Date date = new Date(); DateFormat dateFormat = DateFormat.getDateInstance(DateFormat.LONG, Locale.JAPAN); System.out.println("Formatted Date: " + dateFormat.format(date)); } }
This code demonstrates how numbers and dates are formatted according to the conventions of Germany and Japan, respectively. Such built-in capabilities make Java a powerful ally for developers aiming to deliver truly global applications.
Key Java Libraries for Localization
When it comes to localization in Java, several key libraries and frameworks assist developers in streamlining the process. The most notable among these is the java.util.ResourceBundle class, which allows for the easy management of locale-specific resources. It can load resource bundles from different file types such as property files, enabling developers to create applications that can dynamically adapt to the user’s language and region.
A typical resource bundle is structured as a set of property files, each corresponding to a different locale. For instance, you may have MessagesBundle_en.properties
for English and MessagesBundle_fr.properties
for French. Each file contains key-value pairs representing the strings used throughout your application:
# MessagesBundle_en.properties greeting=Hello farewell=Goodbye # MessagesBundle_fr.properties greeting=Bonjour farewell=Au revoir
To access these localized strings in your Java application, utilize the resource bundle as shown in the previous example. The ResourceBundle.getBundle() method fetches the appropriate bundle based on the current locale. This mechanism ensures that your application can easily switch languages based on user preferences.
Another essential library for localization is the java.text package, which contains classes for formatting numbers, currencies, and dates. The flexibility of the NumberFormat and DateFormat classes allows developers to provide easy to use representations of these data types that conform to local customs.
For instance, if you’re developing a financial application that needs to display monetary values in different currency formats, you might use the NumberFormat class like this:
import java.text.NumberFormat; import java.util.Locale; public class CurrencyFormatExample { public static void main(String[] args) { double amount = 123456.78; NumberFormat currencyFormatUS = NumberFormat.getCurrencyInstance(Locale.US); System.out.println("US Currency: " + currencyFormatUS.format(amount)); NumberFormat currencyFormatFR = NumberFormat.getCurrencyInstance(Locale.FRANCE); System.out.println("French Currency: " + currencyFormatFR.format(amount)); } }
In this example, the output will display the currency formatted appropriately for both the US and France, allowing users to easily understand the monetary value in their local context. This highlights how crucial it is to utilize the built-in Java libraries to imropve the user experience when dealing with internationalization.
Further extending this functionality, the java.time package, introduced in Java 8, provides comprehensive support for date and time handling. The DateTimeFormatter class allows formatting of date-time objects according to the locale, making it easier to present temporal data that adheres to regional standards.
import java.time.LocalDate; import java.time.format.DateTimeFormatter; import java.util.Locale; public class DateFormatExample { public static void main(String[] args) { LocalDate today = LocalDate.now(); DateTimeFormatter formatterUS = DateTimeFormatter.ofPattern("MMMM d, yyyy", Locale.US); System.out.println("US Date Format: " + today.format(formatterUS)); DateTimeFormatter formatterFR = DateTimeFormatter.ofPattern("d MMMM yyyy", Locale.FRANCE); System.out.println("French Date Format: " + today.format(formatterFR)); } }
In the above code, the date is formatted differently for the US and France. Using the DateTimeFormatter ensures that the presentation of date and time is not only accurate but also culturally relevant, significantly enhancing the user experience for international users.
In conclusion, using Java’s powerful localization libraries allows developers to build applications that resonate with a global audience. By using ResourceBundle, NumberFormat, and DateTimeFormatter, you can ensure that your applications support a diverse range of languages, cultures, and formatting preferences, ultimately leading to a better, more accessible product for users worldwide.
Best Practices for Resource Bundles
Resource bundles play a fundamental role in creating multilingual applications with Java. They allow developers to encapsulate locale-specific data, such as strings and other resources, in a systematic manner. This modular approach facilitates easy retrieval and management of localized content, enabling a smoother development process and a more cohesive user experience.
When designing resource bundles, it’s vital to follow best practices that enhance maintainability and scalability. Here are some key considerations:
1. Consistent Naming Conventions: Use clear and consistent naming conventions for your resource files. This typically includes the base name followed by the locale, for example, MessagesBundle_en.properties
for English and MessagesBundle_fr.properties
for French. This naming structure not only keeps your project organized but also makes it easier to identify and manage different language packs.
# MessagesBundle_en.properties greeting=Hello farewell=Goodbye # MessagesBundle_fr.properties greeting=Bonjour farewell=Au revoir
2. Use Key Values Judiciously: When defining keys in your resource bundles, choose meaningful and descriptive names. Avoid embedding any language-specific information in the keys themselves, as this can lead to confusion and errors during localization. For instance, instead of using keys like greeting_en
, simply use greeting
.
3. Group Related Messages: Organize your keys logically. Group related messages together to enhance readability and maintainability. For example, if you have messages related to user actions, such as login and registration, you could create a separate section for those in your properties file. This approach streamlines the localization process and makes it easier for translators to work with your resource bundles.
# MessagesBundle_en.properties user.login=Login user.register=Register # MessagesBundle_fr.properties user.login=Connexion user.register=Inscription
4. Avoid Hardcoding Strings: Always utilize resource bundles for strings instead of hardcoding them directly into your Java classes. This practice not only decouples your application logic from the user interface but also simplifies the process of adding new languages in the future. For instance:
import java.util.ResourceBundle; public class UserActions { public static void main(String[] args) { Locale currentLocale = Locale.getDefault(); ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle", currentLocale); System.out.println(messages.getString("user.login")); System.out.println(messages.getString("user.register")); } }
5. Externalize All User-Facing Strings: Ensure that all user-facing strings are externalized in resource bundles. This includes not just static text, but also dynamic content that can change based on user interaction, such as error messages and notifications. By externalizing all such strings, you prepare your application better for future localization efforts.
6. Testing Resource Bundles: Regularly test your resource bundles to ensure that all keys are correctly mapped and that translations are accurate. Utilize automated testing frameworks to validate that your application behaves as expected under various locales. This step is important in catching issues early in the development cycle.
import org.junit.Test; import java.util.Locale; import java.util.ResourceBundle; import static org.junit.Assert.assertEquals; public class ResourceBundleTest { @Test public void testFrenchMessages() { ResourceBundle messages = ResourceBundle.getBundle("MessagesBundle", Locale.FRENCH); assertEquals("Bonjour", messages.getString("greeting")); } }
By adhering to these best practices, developers can ensure that their applications not only support multiple languages but also maintain a high level of quality and user experience. Properly implemented resource bundles are the cornerstone of effective internationalization, allowing your applications to resonate across diverse cultural landscapes.
Handling Date, Time, and Number Formats
When developing multilingual applications in Java, handling date, time, and number formats is essential for creating a seamless user experience across different locales. Different regions have distinct conventions for presenting these data types, and Java offers an extensive framework to manage these variations effortlessly.
The core classes that facilitate this functionality are java.text.NumberFormat
and java.text.DateFormat
, which allow developers to format numbers and dates in a way that is appropriate for the user’s locale. These classes utilize the Locale
object to determine the correct formatting rules for the target region.
To illustrate, let’s think how to format numbers and dates according to the conventions of various locales. The NumberFormat
class can be used to format currency, percentages, and general numbers. Here’s a practical example:
import java.text.NumberFormat; import java.util.Locale; public class NumberFormatExample { public static void main(String[] args) { double number = 1234567.89; NumberFormat numberFormatUS = NumberFormat.getInstance(Locale.US); System.out.println("US Number: " + numberFormatUS.format(number)); NumberFormat numberFormatFR = NumberFormat.getInstance(Locale.FRANCE); System.out.println("French Number: " + numberFormatFR.format(number)); } }
In this example, the output will show the formatted number according to the conventions of the United States and France, allowing users to easily interpret the numerical values based on their familiarity with local formatting.
For handling dates, Java provides the DateFormat
class, which can format date objects into locale-specific strings. For instance, you may want to display the current date in different formats depending on the user’s locale:
import java.text.DateFormat; import java.util.Date; import java.util.Locale; public class DateFormatExample { public static void main(String[] args) { Date today = new Date(); DateFormat dateFormatUS = DateFormat.getDateInstance(DateFormat.LONG, Locale.US); System.out.println("US Date Format: " + dateFormatUS.format(today)); DateFormat dateFormatFR = DateFormat.getDateInstance(DateFormat.LONG, Locale.FRANCE); System.out.println("French Date Format: " + dateFormatFR.format(today)); } }
This code snippet demonstrates how the date is formatted according to the standards used in the United States and France. The use of DateFormat
ensures that users receive date information in a culturally relevant manner, thus enhancing the overall usability of the application.
With the introduction of the java.time
package in Java 8, developers have even more powerful options for managing date and time. The DateTimeFormatter
class allows for comprehensive date formatting that adheres to locale-specific conventions. Here’s how you can use it to format dates:
import java.time.LocalDate; import java.time.format.DateTimeFormatter; import java.util.Locale; public class DateTimeFormatterExample { public static void main(String[] args) { LocalDate today = LocalDate.now(); DateTimeFormatter formatterUS = DateTimeFormatter.ofPattern("MMMM d, yyyy", Locale.US); System.out.println("US Date Format: " + today.format(formatterUS)); DateTimeFormatter formatterFR = DateTimeFormatter.ofPattern("d MMMM yyyy", Locale.FRANCE); System.out.println("French Date Format: " + today.format(formatterFR)); } }
In this example, the date is formatted differently for the US and France, demonstrating how the DateTimeFormatter
can produce date strings that are intuitive for users across different locales.
Additionally, when it comes to number formats, handling currencies is a common requirement in many applications. The NumberFormat.getCurrencyInstance(Locale)
method allows you to format currency values according to the user’s locale, ensuring that monetary values are presented in an understandable way:
import java.text.NumberFormat; import java.util.Locale; public class CurrencyFormatExample { public static void main(String[] args) { double amount = 123456.78; NumberFormat currencyFormatUS = NumberFormat.getCurrencyInstance(Locale.US); System.out.println("US Currency: " + currencyFormatUS.format(amount)); NumberFormat currencyFormatFR = NumberFormat.getCurrencyInstance(Locale.FRANCE); System.out.println("French Currency: " + currencyFormatFR.format(amount)); } }
By employing these formatting classes, developers can ensure that their applications not only handle data accurately but also respect the cultural nuances of their users. The ability to format dates, numbers, and currencies appropriately makes Java a robust choice for building applications that serve a global audience, ensuring that users feel at home, no matter where they’re located.