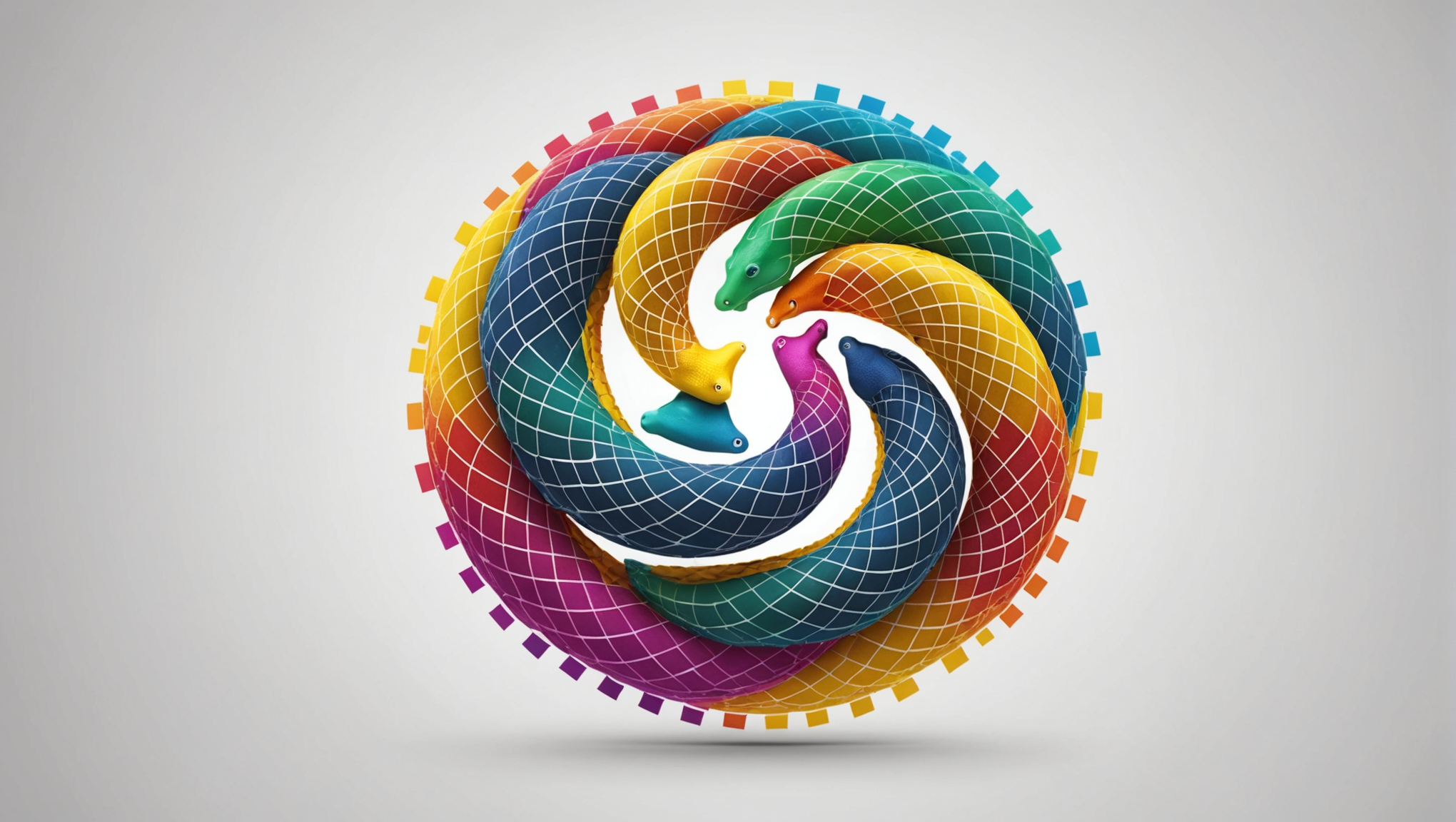
Python for Scientific Computing: An Introduction
Scientific computing encompasses a broad range of computational techniques and methodologies employed to solve complex scientific problems. In the context of Python, this discipline has flourished due to the language’s simplicity, versatility, and an extensive ecosystem of libraries designed specifically for scientific applications. Python’s syntax is clean and intuitive, enabling scientists and engineers to implement algorithms and models efficiently without the overhead often associated with other programming languages.
The core philosophy of Python makes it particularly appealing for scientific computing: it emphasizes readability and simplicity, allowing researchers to focus on their work rather than the intricacies of the programming language itself. Over the years, Python has established itself as a solid choice for both academic research and industry applications, supported by its active community and a wealth of resources.
At the heart of scientific computing in Python lies the ability to perform numerical calculations and manage data effectively. The language can handle various data types and structures, making it suitable for diverse tasks, from simple data analysis to complex simulations. Python’s dynamic typing and interpreted nature facilitate rapid prototyping, which is essential when scientists need to explore hypotheses or analyze experimental results quickly.
One of the defining aspects of Python’s role in scientific computing is its integration with other languages and tools. For instance, Python can seamlessly interface with C and Fortran code, allowing for high-performance computing when necessary. This characteristic is critical in scenarios where execution speed is paramount, such as in large-scale numerical simulations.
The collaborative nature of Python has also led to the development of a high number of libraries tailored for scientific purposes. These libraries, such as NumPy, SciPy, and Matplotlib, provide a robust framework for performing mathematical operations, statistical analysis, and data visualization. The synergy between these tools empowers users to tackle a wide range of scientific problems efficiently.
Consider a simple example where we perform a numerical integration using the trapezoidal rule. This rule approximates the area under a curve by breaking it down into trapezoids. Here’s how we might implement this in Python:
def trapezoidal_rule(f, a, b, n): h = (b - a) / n integral = 0.5 * (f(a) + f(b)) for i in range(1, n): integral += f(a + i * h) integral *= h return integral # Example usage import math result = trapezoidal_rule(math.sin, 0, math.pi, 1000) print("Approximate integral of sin(x) from 0 to π:", result)
This code snippet illustrates how Python can be used to implement a numerical method with minimal effort, emphasizing its suitability for scientific computing. The clear structure of the code not only enhances readability but also facilitates debugging and collaboration among researchers.
As the landscape of scientific computing continues to evolve, Python stands out as a leading choice due to its strong community support, extensive libraries, and inherent flexibility. This makes it a powerful tool for effectively addressing the multifaceted challenges faced in scientific research today.
Key Libraries for Scientific Computing
In the realm of scientific computing with Python, several key libraries have emerged as indispensable tools. Each library addresses specific computational needs, thereby enhancing the capabilities of Python in handling scientific tasks. Understanding these libraries very important for any researcher or developer aiming to leverage Python for scientific applications.
NumPy is one of the fundamental packages for numerical computing in Python. It provides support for arrays and matrices, along with a collection of mathematical functions to operate on these data structures. NumPy’s array processing capabilities enable efficient storage and manipulation of large datasets, which is essential in scientific computing. By using NumPy, one can perform operations such as element-wise addition, multiplication, and other linear algebra operations with remarkable ease.
import numpy as np # Creating a NumPy array data = np.array([1, 2, 3, 4, 5]) # Performing element-wise operations squared = data ** 2 mean_value = np.mean(data) print("Data:", data) print("Squared:", squared) print("Mean:", mean_value)
Next, we have SciPy, which builds on NumPy and provides additional functionality for scientific and technical computing. It offers modules for optimization, integration, interpolation, eigenvalue problems, and more. SciPy is particularly beneficial for performing complex mathematical operations and for solving differential equations, making it a cornerstone library for many scientific applications.
from scipy.integrate import quad # Define the function to integrate def integrand(x): return x**2 # Perform the integration of x^2 from 0 to 1 result, error = quad(integrand, 0, 1) print("Integral of x^2 from 0 to 1:", result) print("Estimated error:", error)
Pandas is another critical library, particularly for data manipulation and analysis. It provides data structures like DataFrames, which are analogous to tables in relational databases. This makes it particularly suitable for handling structured data, allowing users to easily filter, aggregate, and transform datasets. Its integration with NumPy makes operations seamless, enabling quick analyses and manipulations of data.
import pandas as pd # Creating a DataFrame data = {'A': [1, 2, 3], 'B': [4, 5, 6]} df = pd.DataFrame(data) # Calculating the mean of each column mean_values = df.mean() print("DataFrame:n", df) print("Mean values:n", mean_values)
For visualizing scientific data, Matplotlib serves as the go-to library. It allows for the creation of static, animated, and interactive visualizations in Python. The ability to generate plots, histograms, and other graphical representations of data especially important for interpreting results and communicating findings in a visually compelling way.
import matplotlib.pyplot as plt # Sample data for plotting x = np.linspace(0, 10, 100) y = np.sin(x) # Creating a plot plt.plot(x, y) plt.title("Sine Wave") plt.xlabel("x") plt.ylabel("sin(x)") plt.grid(True) plt.show()
Finally, SymPy is a powerful library for symbolic mathematics. It allows for algebraic computations, calculus, and even equation solving. This capability can be particularly beneficial for researchers needing to derive analytical solutions to mathematical problems.
from sympy import symbols, integrate # Define symbols x = symbols('x') # Define the function to integrate function = x**2 # Perform symbolic integration integral = integrate(function, x) print("Symbolic integral of x^2:", integral)
Each of these libraries contributes uniquely to the scientific computing landscape in Python, providing researchers and engineers with the tools necessary to conduct complex analyses and simulations. Their combined power makes Python a robust choice for tackling a wide array of scientific challenges, from data analysis to algorithm development and visualization.
Data Manipulation and Analysis with NumPy
Data manipulation and analysis are fundamental aspects of scientific computing, and NumPy plays a pivotal role in these processes. Designed specifically for numerical computing, NumPy introduces a powerful data structure called the ndarray (N-dimensional array), which very important for handling large datasets efficiently. This array object supports a wide range of operations that can be applied element-wise, enabling high-performance computations with minimal code.
One of the key advantages of using NumPy is its ability to perform vectorized operations. Instead of using loops—which can be slow and cumbersome—NumPy allows for operations to be applied directly to entire arrays. This not only results in cleaner code but also significantly improves performance. For instance, think the following example where we generate a sequence of numbers and perform some mathematical operations:
import numpy as np # Create an array of numbers from 0 to 9 data = np.arange(10) # Perform mathematical operations squared = data ** 2 sin_values = np.sin(data) print("Original data:", data) print("Squared values:", squared) print("Sine values:", sin_values)
In this snippet, we use NumPy to create an array of integers from 0 to 9. We then calculate the square of each element and the sine of each integer in the array. The clarity of the operations reflects Python’s readability and NumPy’s efficiency.
Another powerful feature of NumPy is its broadcasting capability, which allows for operations between arrays of different shapes. That’s particularly useful in scientific computations where datasets may not always match in size but need to be combined mathematically. Here’s an illustration of how broadcasting works:
# Create a 2D array (matrix) matrix = np.array([[1, 2, 3], [4, 5, 6]]) # Create a 1D array vector = np.array([1, 0, 1]) # Add vector to each row of the matrix result = matrix + vector print("Matrix:n", matrix) print("Vector:", vector) print("Result after broadcasting:n", result)
In this example, we define a 2D array and a 1D array. By adding the vector to the matrix, NumPy automatically broadcasts the addition across the rows, showcasing its ability to handle operations between differently shaped arrays seamlessly.
NumPy also provides a host of linear algebra functions, which are essential in many scientific applications. These include operations such as matrix multiplication, determinant calculation, and eigenvalue decomposition. Below is a demonstration of how to perform matrix multiplication:
# Define two matrices A = np.array([[1, 2], [3, 4]]) B = np.array([[5, 6], [7, 8]]) # Multiply matrices product = np.matmul(A, B) print("Matrix A:n", A) print("Matrix B:n", B) print("Product of A and B:n", product)
In this snippet, we define two matrices and calculate their product using NumPy’s matmul function. This operation demonstrates how NumPy can handle complex linear algebra tasks efficiently, allowing scientists to focus on the results rather than the underlying implementation details.
Furthermore, NumPy’s integration with other libraries, such as SciPy and Pandas, enhances its capabilities for data manipulation and analysis. For instance, when handling structured data or performing statistical analysis, Pandas utilizes NumPy arrays under the hood, ensuring high performance combined with uncomplicated to manage interfaces.
NumPy is an indispensable library for data manipulation and analysis in Python’s scientific computing ecosystem. Its support for N-dimensional arrays, vectorized operations, broadcasting, and linear algebra makes it a powerful tool for researchers and engineers alike. By using NumPy, users can manipulate and analyze data efficiently, paving the way for deeper insights and advancements in scientific research.
Visualization Tools for Scientific Data
When it comes to scientific computing, visualizing data effectively is as crucial as performing the computations that generate it. Python provides a rich variety of libraries that facilitate data visualization, making it easier for researchers and scientists to interpret and communicate their findings. Among these libraries, Matplotlib stands as the most widely used tool for creating static, animated, and interactive visualizations in Python.
Matplotlib’s versatility allows users to create various types of plots and charts, including line plots, scatter plots, histograms, and bar charts. It operates on a MATLAB-like interface, which makes it accessible to those familiar with that environment, while also providing extensive customization options for advanced users. Let’s delve into how we can use Matplotlib for basic data visualization.
import matplotlib.pyplot as plt import numpy as np # Sample data for plotting x = np.linspace(0, 10, 100) y = np.sin(x) # Creating a line plot plt.plot(x, y, label='sin(x)', color='blue') plt.title("Sine Wave") plt.xlabel("x") plt.ylabel("sin(x)") plt.axhline(0, color='black',linewidth=0.5, ls='--') plt.axvline(0, color='black',linewidth=0.5, ls='--') plt.grid(True) plt.legend() plt.show()
This snippet generates a simple sine wave plot. The use of NumPy’s linspace
function creates an array of 100 points between 0 and 10, which we then feed into the sine function. The resulting plot visually represents the mathematical function, allowing for easy interpretation of its oscillatory nature.
To imropve the visualization, Matplotlib allows for the customization of almost every aspect of a plot. You can change colors, add titles, labels, legends, and even grid lines. This flexibility is essential for creating informative and visually appealing graphics that convey the intended message effectively.
For more complex datasets, 3D visualizations can be highly beneficial. Matplotlib supports 3D plotting through its mpl_toolkits.mplot3d
module. Here’s an example of how to create a 3D surface plot:
from mpl_toolkits.mplot3d import Axes3D # Create a grid of points X = np.linspace(-5, 5, 100) Y = np.linspace(-5, 5, 100) X, Y = np.meshgrid(X, Y) Z = np.sin(np.sqrt(X**2 + Y**2)) # Creating a 3D surface plot fig = plt.figure() ax = fig.add_subplot(111, projection='3d') ax.plot_surface(X, Y, Z, cmap='viridis') ax.set_title("3D Surface Plot of sin(sqrt(x^2 + y^2))") ax.set_xlabel("X axis") ax.set_ylabel("Y axis") ax.set_zlabel("Z axis") plt.show()
This 3D plot represents the sine of the distance from the origin in a three-dimensional space. Such visualizations can help in understanding more complex relationships between multiple variables, which is often a requirement in scientific research.
Another noteworthy library for visualization is Seaborn, which is built on top of Matplotlib and provides a high-level interface for drawing attractive statistical graphics. Seaborn improves upon Matplotlib by simplifying the syntax and enhancing the aesthetics of the plots. It’s particularly beneficial when dealing with statistical data and allows for easy visualization of complex datasets.
import seaborn as sns # Sample data for Seaborn tips = sns.load_dataset("tips") # Creating a scatter plot with a regression line sns.regplot(x='total_bill', y='tip', data=tips) plt.title("Tip Amount vs Total Bill") plt.xlabel("Total Bill") plt.ylabel("Tip") plt.show()
In this example, we leverage Seaborn to create a scatter plot with a regression line, demonstrating the relationship between the total bill and the tip amount in a restaurant setting. The simplicity of the syntax coupled with the aesthetically pleasing output makes Seaborn a preferred choice for many data scientists.
Ultimately, the ability to visualize scientific data is an indispensable skill in research. Whether using Matplotlib for detailed custom plots or Seaborn for rapid statistical visualizations, Python provides robust tools that empower scientists to uncover insights and communicate results effectively. These visualization techniques not only enhance data analysis but also help convey complex findings to both technical and non-technical audiences alike.
Case Studies: Applications of Python in Science
Python has garnered significant traction in various scientific domains, facilitating groundbreaking research and innovation. The language’s versatility is evident in its application across diverse fields, each using Python’s strengths to tackle unique challenges. Here are a few notable case studies that illustrate Python’s impact on scientific computing.
One classic example comes from the field of astronomy, where researchers use Python to analyze vast amounts of data collected from telescopes. The European Southern Observatory has developed a suite of tools based on Python that enables astronomers to manage and analyze data from their facilities effectively. With libraries such as AstroPy, scientists can easily manipulate astronomical data, perform celestial coordinate transformations, and even simulate the dynamics of celestial bodies. The simplicity of these operations allows astronomers to focus on their analyses rather than getting bogged down by complex programming syntax.
Consider the following example, where we utilize NumPy for basic astronomical calculations. Let’s say we want to compute the distance to a star given its parallax:
import numpy as np # Function to calculate distance based on parallax def calculate_distance(parallax_arcsec): if parallax_arcsec == 0: raise ValueError("Parallax cannot be zero.") distance_parsecs = 1 / parallax_arcsec return distance_parsecs # Example usage parallax = 0.1 # Parallax in arcseconds distance = calculate_distance(parallax) print(f"Distance to star: {distance} parsecs")
This snippet demonstrates a simple yet critical calculation in astronomy—determining the distance to a star. By using Python’s capabilities, astronomers can perform such calculations quickly and efficiently.
In the realm of climate science, Python has become a linchpin for modeling and analyzing climate data. The National Oceanic and Atmospheric Administration (NOAA) employs Python in various capacities, from data wrangling to building complex climate models. The use of libraries like Pandas and Matplotlib enables researchers to analyze historical climate data and visualize trends over time effectively.
For instance, by using a combination of Pandas for data manipulation and Matplotlib for visualization, researchers can create informative graphs depicting changes in global temperatures:
import pandas as pd import matplotlib.pyplot as plt # Load climate data data = pd.read_csv('global_temperature.csv') # Assume this file contains annual temperature data # Plotting global temperature trends plt.plot(data['Year'], data['Temperature']) plt.title("Global Temperature Change Over Time") plt.xlabel("Year") plt.ylabel("Temperature (°C)") plt.grid(True) plt.show()
This example highlights how Python streamlines the process of analyzing and visualizing climate data, allowing scientists to derive insights more efficiently.
Another compelling case is in the field of bioinformatics, where Python plays a critical role in genomic data analysis. The Biopython library provides tools for biological computation, enabling researchers to manipulate DNA sequences and perform complex biological analyses. For instance, genome assembly and variant calling are increasingly performed using Python, significantly speeding up the analysis pipeline.
Here’s a simplified example of how one might use Biopython to read a DNA sequence from a file and calculate its GC content:
from Bio import SeqIO # Function to calculate GC content def calculate_gc_content(seq): g = seq.count('G') c = seq.count('C') total = len(seq) gc_content = (g + c) / total * 100 return gc_content # Read a DNA sequence from a FASTA file for record in SeqIO.parse('example.fasta', 'fasta'): gc_content = calculate_gc_content(str(record.seq)) print(f"GC Content of {record.id}: {gc_content:.2f}%")
This code snippet illustrates how Python can facilitate biological research, allowing researchers to perform sophisticated analyses with ease.
These case studies exemplify Python’s ability to meet the needs of various scientific fields. By providing a robust framework for data analysis, visualization, and numerical computation, Python serves as an invaluable tool for researchers, helping them push the boundaries of knowledge in their respective disciplines. As Python continues to evolve and grow, its applications in scientific computing are destined to expand even further, fostering a new generation of scientific breakthroughs.