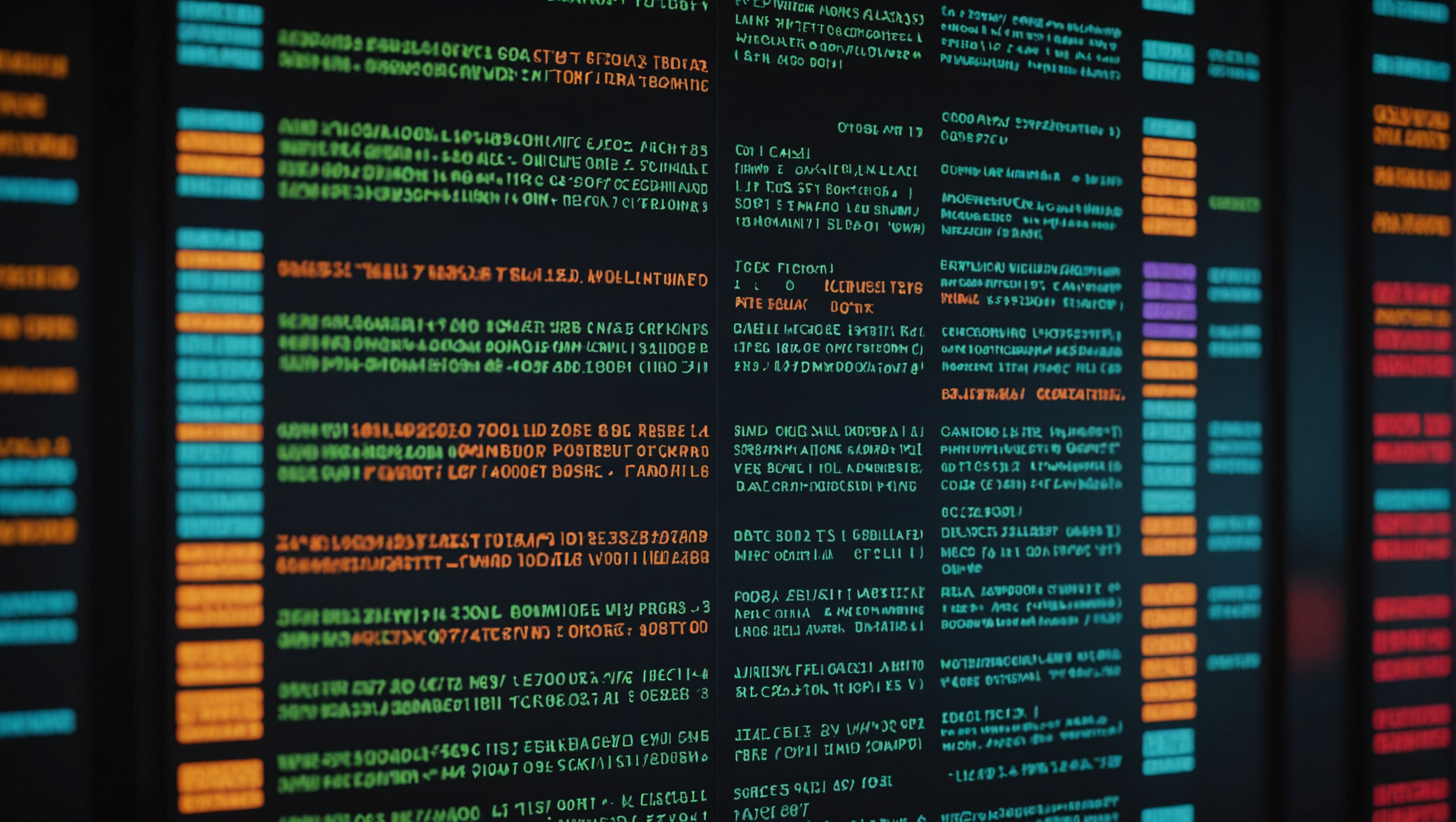
Advanced File Operations in Bash
Mastering file manipulation commands in Bash is akin to wielding a powerful toolset that can streamline your workflow and enhance productivity. The core commands you’ll employ are cp
, mv
, rm
, and touch
, each serving specific purposes that can be combined for greater effect.
The cp command is your go-to for copying files and directories. To copy a file, the syntax is straightforward:
cp source_file destination_file
For example, if you want to duplicate file.txt
to copy_file.txt
, you would use:
cp file.txt copy_file.txt
To copy an entire directory, you must use the -r (recursive) option:
cp -r source_directory/ destination_directory/
Next, the mv command, as the name implies, is used for moving files and directories. It can also rename files. The basic syntax for moving or renaming is:
mv old_name new_name
For instance, to rename old_file.txt
to new_file.txt
:
mv old_file.txt new_file.txt
Moving a file to a different directory is just as simple:
mv file.txt /path/to/destination/
The rm command is potent and should be used with caution, as it permanently deletes files without confirmation. The basic usage is:
rm file.txt
To remove a directory and its contents recursively, the -r option is again necessary:
rm -r directory_name/
Adding the -i option prompts for confirmation before each deletion, adding a safety net:
rm -i file.txt
Lastly, the touch command serves primarily to create empty files or to update the timestamps of existing ones. The syntax is straightforward:
touch new_file.txt
By mastering these commands, you can efficiently navigate and manipulate your file system, laying the groundwork for more complex operations and scripting endeavors.
Using Wildcards and Regular Expressions
In the realm of Bash scripting, wildcards and regular expressions are not just useful—they’re essential for advanced file operations. Understanding how to utilize these powerful features can significantly amplify your ability to work with files in a flexible and efficient manner.
Wildcards are special characters that allow you to match multiple files based on specific patterns. The most commonly used wildcards are:
- Matches any number of characters (including none). For example,
*.txt
matches all text files in the current directory. - Matches a single character. For instance,
file?.txt
would matchfile1.txt
but notfile10.txt
. - Matches any one of the enclosed characters. For example,
file[1-3].txt
matchesfile1.txt
,file2.txt
, andfile3.txt
.
These wildcards can be applied directly in commands. For instance, if you want to delete all log files in a directory, you can execute:
rm *.log
Regular expressions, on the other hand, provide a more robust way to define search patterns. They enable complex matching scenarios that wildcards alone cannot handle. In Bash, regular expressions can be utilized with commands like grep
, sed
, and awk
.
To illustrate, think using grep
to search for files containing a specific pattern. If you want to find all lines in a text file that start with the letter ‘A’, you could use:
grep '^A' file.txt
Additionally, regular expressions allow for grouping and repetition. The character . matches any single character, while * matches zero or more occurrences. For example, file.*
matches any file name that starts with ‘file’ followed by any characters.
When dealing with filenames, you may want to rename multiple files based on a pattern. This can be achieved using a combination of wildcards and a loop. Ponder renaming all text files to have a prefix:
for file in *.txt; do mv "$file" "prefix_$file" done
This snippet will prepend “prefix_” to all text files in the current directory.
Combining wildcards and regular expressions with other Bash commands can lead to incredibly powerful scripts. For instance, suppose you want to find and delete files that match a certain pattern. You can first list those files using ls
combined with grep
, then pipe the output to xargs
to delete them:
ls | grep '.bak$' | xargs rm
The above command will delete all files with a .bak extension, demonstrating the synergy between these tools. Mastering wildcards and regular expressions allows you to handle files with an elegant precision that’s fundamental to advanced file operations in Bash.
Efficiently Handling File Permissions
Handling file permissions in Bash is essential for maintaining security and ensuring that your scripts and applications run smoothly. The Linux file permission system is built around three key types of users: the owner of the file, the group associated with the file, and all other users. Each of these user types can have three types of permissions: read (r), write (w), and execute (x). Understanding how to view and modify these permissions effectively will empower you to control access to your files and directories.
To view the current permissions of a file or directory, you can use the ls -l
command, which lists files in long format. The first column in the output indicates the permissions:
ls -l myfile.txt
The output will look something like this:
-rw-r--r-- 1 user group 0 Oct 1 12:00 myfile.txt
In this output, the first part -rw-r--r--
describes the permissions. The first character indicates the type (a dash for a regular file), followed by three sets of characters indicating permissions for the owner, group, and others, respectively. In this example, the owner can read and write the file, while the group and others can only read it.
Modifying file permissions can be achieved with the chmod
command, which stands for “change mode.” There are two primary ways to specify permissions: symbolic and numeric.
Using symbolic notation, you can add or remove permissions using the following syntax:
chmod u+x myfile.txt
This command adds execute permission for the file’s owner (user). Conversely, to remove write permission for the group:
chmod g-w myfile.txt
Numeric notation, on the other hand, uses three digits, with each digit representing the permissions for the owner, group, and others, respectively. Each permission type has a numeric value: read is 4, write is 2, and execute is 1. To set permissions, you sum these values. For instance, to set read and write permissions for the owner, and read permissions for the group and others, you would use:
chmod 644 myfile.txt
An alternative way to manage permissions is by using the chown
command, which changes the ownership of files and directories. Changing both the owner and group can be done with:
chown user:group myfile.txt
When you need to modify permissions recursively for a directory and all its contents, the -R
option becomes invaluable:
chmod -R 755 my_directory/
Here, all files and directories within my_directory
will receive read, write, and execute permissions for the owner, and read and execute permissions for the group and others.
Lastly, understanding the umask
command very important for setting default permissions for newly created files and directories. This command defines the permissions that will not be set. For instance, if your current umask is 0022
, files will generally be created with permissions of 644
and directories with 755
. You can view your current umask value by simply typing:
umask
Adjusting the umask can be done with:
umask 0077
This setting means that new files will only be accessible by the owner, which is often a desirable level of security for sensitive files.
Through careful management of file permissions, you can create a robust security model that protects your data while still allowing necessary access. Mastering these concepts will greatly enhance your Bash scripting capabilities and ensure the safety of your file operations.
Advanced Techniques for File Backup and Recovery
In the realm of file management, the importance of implementing effective backup and recovery techniques cannot be overstated. Whether you’re safeguarding critical data or merely ensuring peace of mind, a structured approach to backups can save you from potential disasters. In Bash, there are numerous strategies and tools at your disposal to facilitate efficient file backup and recovery, each tailored to different scenarios and requirements.
One fundamental method for backing up files is simply using the cp
command to create copies in a designated backup directory. For a simpler backup of an entire directory, you would employ the recursive option -r
, like so:
cp -r /path/to/source_directory /path/to/backup_directory
This method is effective for one-off backups but may not suffice for ongoing data safety. For a more automated approach, think using the tar
command, which bundles files into a single archive, making it easier to manage and transfer. The basic command to create a compressed archive is:
tar -czf backup.tar.gz /path/to/directory
This command compresses the specified directory into a .tar.gz
file, preserving the structure and contents. To restore from this archive, you would use:
tar -xzf backup.tar.gz
Another powerful tool for backups is rsync
, which not only copies files but also synchronizes them, only transferring changes since the last backup. This utility is especially useful for large datasets or when performing incremental backups. A typical rsync
command might look like this:
rsync -av --delete /path/to/source/ /path/to/backup/
Here, the -a
option enables archive mode to preserve permissions and timestamps, while -v
provides verbose output. The --delete
option ensures that files deleted in the source are also removed from the backup, keeping both directories in sync.
When considering recovery, the approach depends heavily on how you manage your backups. If you’ve implemented regular backups using the aforementioned tools, the recovery process can be relatively simpler. However, in situations where a file might be inadvertently deleted, you can utilize the find
command to locate and restore files if you have a systematic way of tracking changes. For example, to search for recently modified files, you could use:
find /path/to/backup/ -type f -mtime -7
This command lists files in the backup directory that have been modified in the last seven days. You can then restore these files as needed.
For more robust recovery systems, think implementing version control using tools like git
. By initializing a git repository in your working directory, you gain the ability to track changes, revert to previous states, and manage multiple versions of your files. Initializing a repository can be as simple as:
git init
After making changes to your files, you can commit those changes with:
git add .
git commit -m "Backup before major changes"
This setup allows you to recover previous versions of files with ease, giving you a safety net that extends beyond traditional backup strategies.
To summarize, employing a combination of cp
, tar
, rsync
, and version control systems like git
can significantly enhance your file backup and recovery capabilities in Bash. These methods not only protect your data but also empower you to manage it effectively, ensuring that you remain resilient against data loss.