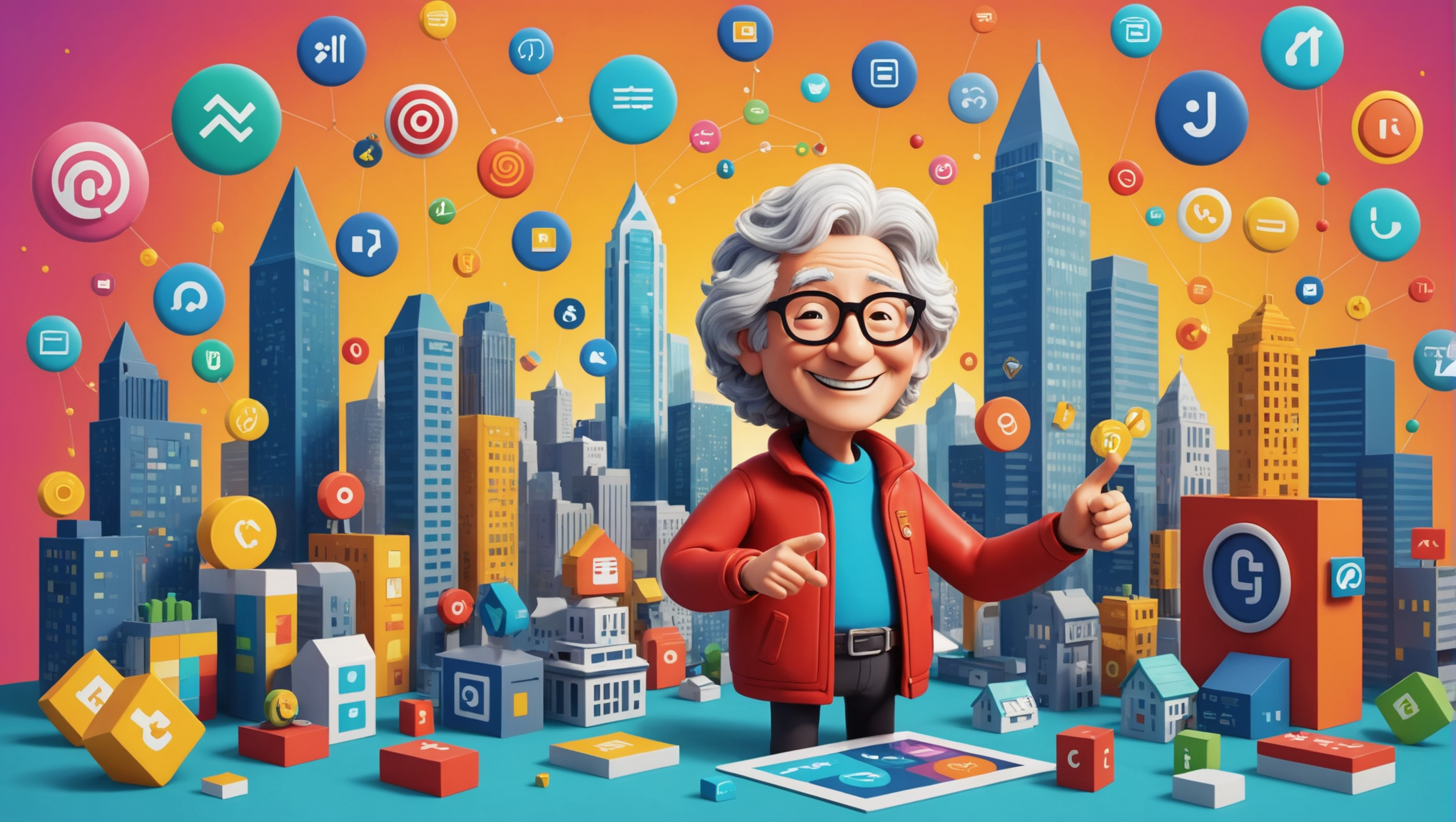
Mastering JavaScript Objects and Properties
JavaScript objects serve as the fundamental building blocks for data manipulation and organization, mirroring real-world entities through key-value pairs. At the core, an object is a collection of properties, where each property consists of a key (or name) and a corresponding value. These values can be of any data type, including other objects, functions, or even primitive types like numbers and strings.
To create a basic object, you can use either the object literal syntax or the Object
constructor. The object literal syntax is more concise and widely adopted due to its readability:
const person = { name: "Albert Lee", age: 30, profession: "Developer" };
In this example, person
is an object with three properties: name
, age
, and profession
. You can access these properties using dot notation:
console.log(person.name); // "Mitch Carter" console.log(person.age); // 30
Alternatively, you can also access properties using bracket notation, which is particularly useful when dealing with dynamic keys or keys that contain spaces or special characters:
console.log(person["profession"]); // "Developer"
Creating objects using the Object
constructor looks like this:
const car = new Object(); car.make = "Toyota"; car.model = "Corolla"; car.year = 2021;
While both methods achieve the same goal, the object literal syntax is generally preferred for its brevity and clarity.
Understanding how to add, modify, or delete properties from an object is important. You can add or change properties simply by assigning a value to a key:
person.age = 31; // Modify existing property person.city = "New York"; // Add new property
To remove a property, the delete
operator comes into play:
delete person.profession;
After executing the above line, person
will no longer have the profession
property.
It is also essential to note that objects in JavaScript are reference types. When you assign an object to a variable, you’re assigning a reference to that object, not the object itself. This characteristic can lead to some unintuitive behavior:
const anotherPerson = person; anotherPerson.name = "Jane Smith"; console.log(person.name); // "Jane Smith" - both variables reference the same object
To create a true copy of an object, you can use methods like Object.assign()
or the spread operator:
const copyOfPerson = Object.assign({}, person); // or using the spread operator const copyOfPerson = {...person};
These methods allow for shallow copies, meaning nested objects will still reference the original objects. For deep cloning, third-party libraries like Lodash or implementing custom recursive functions may be necessary.
Mastering the basics of JavaScript objects lays the foundation for advanced features and techniques, enabling developers to model complex systems efficiently.
Creating and Modifying Objects
JavaScript provides a robust and flexible means of creating and modifying objects, which can significantly enhance your development workflow. Once you’ve grasped the fundamentals of creating objects, the next logical step is to delve into how you can manipulate these objects to suit your needs.
To modify an existing object, you can directly set new values or update existing properties. That is a simpler process that allows you to adapt your objects at runtime. For instance, if you want to change the age of the person object we created earlier, you simply assign a new value as follows:
person.age = 31;
This line updates the age property of the person object. Similarly, you can add new properties in the same manner:
person.city = "New York";
With this addition, the person object now includes a city property along with the existing ones. The ability to dynamically modify objects is one of the powerful features of JavaScript, allowing for the creation of highly interactive applications.
Removing properties is just as intuitive. The delete operator is your tool of choice for this task. By invoking it on a property, you can effectively erase that property from the object:
delete person.profession;
After this operation, the profession property will no longer exist on the person object, reflecting the dynamic nature of JavaScript objects.
Another crucial aspect of object manipulation is understanding how JavaScript handles object references. When you assign one object to another variable, you’re not creating a new object; instead, you are creating a reference to the original object. Ponder the following code:
const anotherPerson = person; anotherPerson.name = "Jane Smith"; console.log(person.name); // "Jane Smith"
As illustrated, changing the name property via anotherPerson also changes it for person since both variables reference the same object in memory. This behavior can lead to unintended consequences if not carefully managed.
To create a true copy of an object—one that does not share references—you can utilize methods like Object.assign() or the spread operator. These methods are useful when you need to duplicate an object while preserving the original:
const copyOfPerson = Object.assign({}, person); // or using the spread operator const copyOfPerson = {...person};
Keep in mind that these methods create a shallow copy, which means that if your object contains other nested objects, those nested objects will still reference the original objects. For instance, if person included a nested object representing an address, changes to that nested object in the copy would also affect the original. To achieve deep cloning, you might need to rely on third-party libraries like Lodash or implement your own recursive cloning functions.
The ability to create and modify objects with ease is a core competency for any JavaScript developer. Mastering these skills not only enhances your ability to manage data but also opens up further avenues for advanced techniques that leverage the full power of JavaScript objects.
Advanced Object Properties and Descriptors
To fully harness the capabilities of JavaScript objects, it is crucial to understand advanced object properties and descriptors. JavaScript allows for fine-grained control over properties through the use of property descriptors, which are objects that describe the configuration of a property on an object. Each property descriptor can contain several attributes: value, writable, enumerable, and configurable.
When defining a property, you can choose to control its behavior using these descriptors. For example, if you want to create a property that cannot be altered (non-writable), you can define it using the Object.defineProperty()
method:
const car = {}; Object.defineProperty(car, 'make', { value: 'Toyota', writable: false, enumerable: true, configurable: true }); car.make = 'Honda'; // This will not change the value console.log(car.make); // "Toyota"
In this example, the make
property is created with a writable attribute set to false, meaning it cannot be changed after its initial assignment. If you attempt to modify it, the value remains unchanged.
The enumerable attribute determines whether the property will show up in loops like for...in
or when using Object.keys()
. In the example above, since enumerable
is set to true, the make
property will be included in these iterations.
Another important attribute is configurable, which indicates whether the property descriptor can be changed and whether the property can be deleted from the object. If configurable
is set to false, you cannot change any of the other attributes or delete the property:
Object.defineProperty(car, 'model', { value: 'Corolla', writable: true, enumerable: true, configurable: false }); delete car.model; // This will fail silently in non-strict mode console.log(car.model); // "Corolla"
Here, the model
property is created but marked as non-configurable. Attempts to delete it will fail, and any changes to its descriptor will not be allowed.
To read a property’s descriptor, you can use the Object.getOwnPropertyDescriptor()
method, which returns the descriptor for a specific property:
const descriptor = Object.getOwnPropertyDescriptor(car, 'make'); console.log(descriptor); // Outputs: { value: 'Toyota', writable: false, enumerable: true, configurable: true }
Understanding and using these property descriptors effectively allows you to create robust and predictable behaviors in your JavaScript objects. This control allows you to encapsulate object properties, making your code more maintainable and easier to understand. Furthermore, using property descriptors can enhance performance in complex applications by reducing unintended side effects and ensuring that your objects behave as expected.
In addition to basic properties, you can also leverage getters and setters to define properties that execute custom code when accessed or updated. This approach not only encapsulates the internal representation of the data but can also provide validation or transformation logic:
const person = { firstName: 'John', lastName: 'Doe', get fullName() { return `${this.firstName} ${this.lastName}`; }, set fullName(name) { [this.firstName, this.lastName] = name.split(' '); } }; console.log(person.fullName); // "Neil Hamilton" person.fullName = 'Jane Smith'; console.log(person.firstName); // "Jane" console.log(person.lastName); // "Smith"
In this case, the fullName
property is defined with a getter and a setter, allowing you to read and write the full name in a more intuitive way. This encapsulation of logic ensures that any changes are handled properly, showcasing the power of property descriptors in JavaScript.
By mastering advanced object properties and descriptors, you can significantly increase the robustness and flexibility of your JavaScript applications, allowing for cleaner and more maintainable code structures.
Prototypes and Inheritance in JavaScript
// Continuing with prototypes and inheritance in JavaScript // JavaScript utilizes a prototype-based inheritance model, which is fundamentally different from classical inheritance seen in other programming languages. // Every JavaScript object has a prototype, and objects can inherit properties and methods from their prototypes. // Let's take a look at how this works in practice. function Animal(name) { this.name = name; } // Adding a method to the Animal prototype Animal.prototype.speak = function() { console.log(this.name + ' makes a noise.'); }; // Creating an instance of Animal const dog = new Animal('Dog'); dog.speak(); // Outputs: Dog makes a noise. // Now, if we want to create a more specific type of animal, we can create a Dog constructor function that inherits from Animal. function Dog(name) { Animal.call(this, name); // Call the parent constructor with the current context } // Setting Dog's prototype to an instance of Animal Dog.prototype = Object.create(Animal.prototype); Dog.prototype.constructor = Dog; // Setting the constructor property back to Dog // Adding a method specific to Dog Dog.prototype.speak = function() { console.log(this.name + ' barks.'); }; // Creating an instance of Dog const myDog = new Dog('Rex'); myDog.speak(); // Outputs: Rex barks. // This pattern of using constructors and prototypes allows for a clear inheritance structure. // The Dog instance inherits the speak method from Animal but can also have its own implementation of speak. // Prototypes also allow you to extend built-in objects. Array.prototype.first = function() { return this[0]; }; // Now we can use our new method on any array const arr = [1, 2, 3, 4]; console.log(arr.first()); // Outputs: 1 // However, modifying built-in prototypes is generally discouraged as it can lead to unexpected behavior in your code and in third-party libraries. // To check if an object inherits from a certain prototype, you can use the isPrototypeOf method: console.log(Dog.prototype.isPrototypeOf(myDog)); // true console.log(Animal.prototype.isPrototypeOf(myDog)); // true // You can also use the instanceof operator to check inheritance: console.log(myDog instanceof Dog); // true console.log(myDog instanceof Animal); // true // Understanding prototypes and inheritance in JavaScript allows developers to create more organized and maintainable code. // By using prototypes, you can share methods and properties across instances, reducing memory usage and improving performance.
Object Methods and Best Practices
In the realm of JavaScript, object methods are not merely functions associated with objects; they’re the very essence of how we can manipulate and interact with those objects effectively. When we encapsulate functions within an object, we create methods that can operate on the data contained within that object. This encapsulation serves to imropve modularity and readability in our code. Let’s explore how to define and utilize methods within objects, as well as best practices to follow.
To define a method within an object, you can simply include a function as a property. For example, think the following code that defines a simple object representing a counter:
const counter = { count: 0, increment: function() { this.count++; }, getCount: function() { return this.count; } };
In this example, the counter
object has two methods: increment
, which increases the value of count
, and getCount
, which retrieves the current count. By using the this
keyword, these methods can access properties of their own object.
To use these methods, you simply call them on the object:
counter.increment(); console.log(counter.getCount()); // Outputs: 1
It’s important to adhere to best practices when defining methods within your objects. One common practice is to use concise method definitions, especially when targeting contemporary JavaScript environments. This can enhance readability. For instance:
const counter = { count: 0, increment() { this.count++; }, getCount() { return this.count; } };
In addition to defining methods directly on objects, you can also create methods that interact with other objects. For example, you might develop a simple banking system:
const bankAccount = { balance: 0, deposit(amount) { this.balance += amount; }, withdraw(amount) { if (this.balance >= amount) { this.balance -= amount; } else { console.log('Insufficient funds'); } } };
With this setup, you can easily manage the account:
bankAccount.deposit(100); console.log(bankAccount.balance); // Outputs: 100 bankAccount.withdraw(30); console.log(bankAccount.balance); // Outputs: 70 bankAccount.withdraw(100); // Outputs: Insufficient funds
When implementing methods, think the implications of this
. The context in which a method is invoked can significantly affect its behavior. For instance, if we were to pass a method as a callback, this
may not refer to the original object:
const logBalance = bankAccount.getBalance; logBalance(); // Undefined or error
To overcome this issue, you can use arrow functions or the bind
method to preserve the context:
const logBalance = bankAccount.getBalance.bind(bankAccount); logBalance(); // Now retrieves the correct balance
Another best practice is to avoid modifying built-in objects’ prototypes. While JavaScript allows for extending built-in objects, doing so can lead to conflicts and unexpected behaviors, especially when using third-party libraries. It’s generally safer to extend your own custom objects rather than altering native prototypes.
Implementing methods within JavaScript objects provides a structured approach to managing functionality and data. By adhering to best practices and being mindful of context, you can create robust, maintainable code that leverages the full power of object-oriented programming in JavaScript.