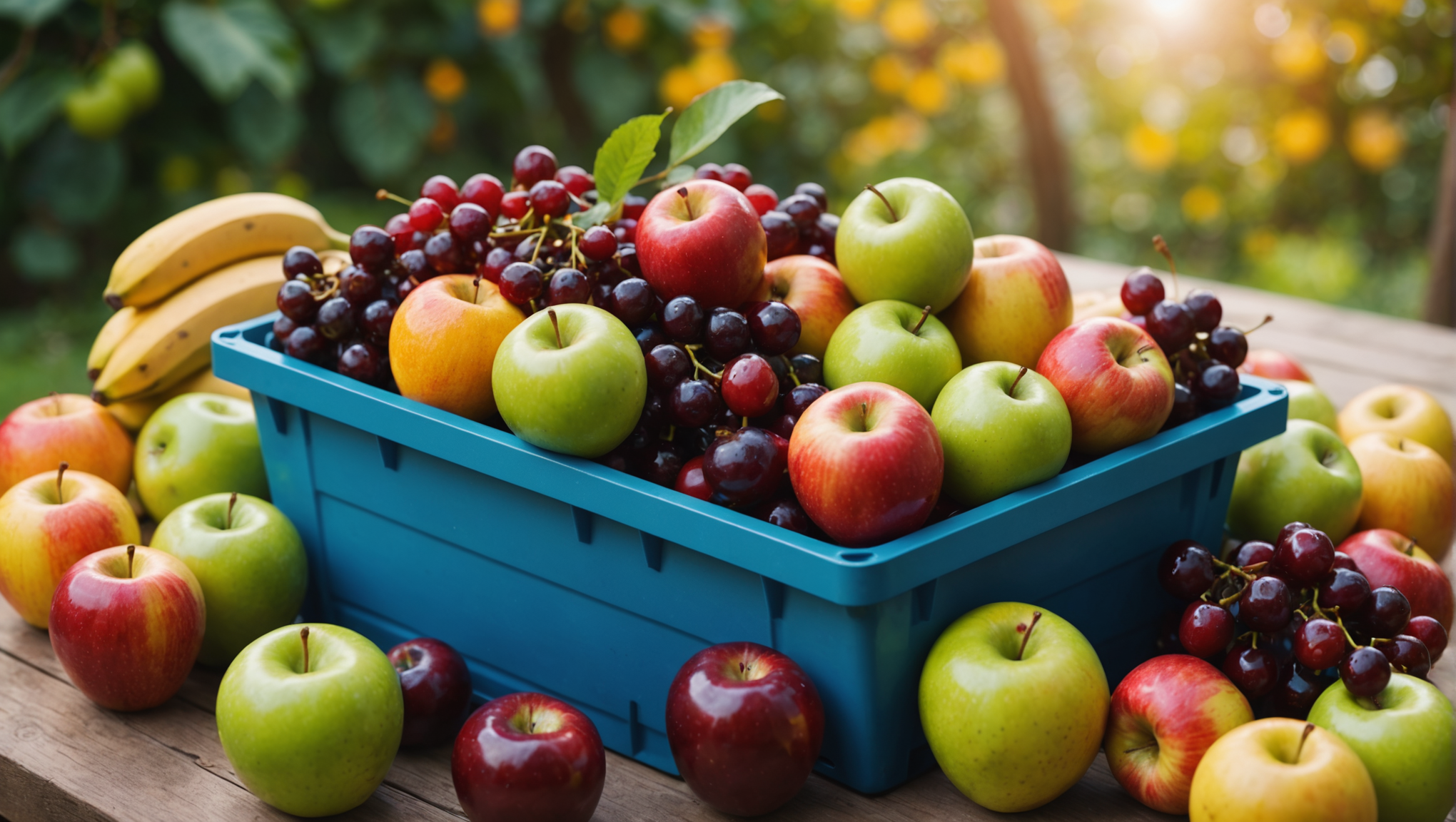
Swift Collections: Arrays
Arrays in Swift are fundamental data structures that allow you to store ordered collections of values. They can hold elements of any type, and you can also create arrays of various data types using generics. The power of arrays lies not only in their ability to store multiple values but also in the flexibility and efficiency with which you can manipulate those values.
Swift arrays are implemented as structs, which means they’re value types. This choice has significant implications for how arrays behave in your code. When you assign an array to a new variable or pass it to a function, Swift creates a copy of that array. This contrasts with reference types, where assignments and function calls share the same instance. The result is that Swift arrays are more predictable in terms of memory management and side effects, allowing developers to reason about their code more effectively.
Arrays can grow and shrink dynamically, which means you don’t need to specify the size when creating them. Under the hood, Swift manages the memory allocations, which contributes to the language’s performance characteristics. However, like all dynamic structures, operations that modify the size of an array can be costly, particularly if they require reallocating memory.
To declare an array, you can use the following syntax:
var numbers: [Int] = []
This initializes an empty array of integers. You can also create and initialize an array with default values:
var repeatedValues = Array(repeating: 0, count: 5) // [0, 0, 0, 0, 0]
Alternatively, you can create arrays using array literals, which is often more succinct:
var fruits = ["Apple", "Banana", "Cherry"]
Arrays in Swift not only maintain the order of elements but also allow for duplicate values. Thus, you can have multiple instances of the same item in an array. This can be advantageous when the frequency of certain elements is essential to your application’s logic.
To summarize, Swift arrays are versatile, efficient, and effortless to handle. Their behavior as value types provides a robust foundation for developing applications while minimizing the complexity of memory management. Understanding the nuances of arrays is critical for all Swift developers, as they are a core aspect of data handling in the language.
Creating and Initializing Arrays
Creating and initializing arrays in Swift is simpler, and the language provides multiple ways to do this, catering to different needs and scenarios. This flexibility allows you to leverage arrays effectively right from the start of your development process.
To create an empty array, you can simply declare it with the specified type. For example, if you need an empty array of `String`, you can do the following:
var names: [String] = []
This initializes an empty array ready to hold `String` values. Similarly, if you want to create an empty array of a custom type, you can do so in the same way:
struct Person { let name: String let age: Int } var people: [Person] = []
Another common use case is to initialize an array with a specific number of repeated values. Swift provides a convenient initializer for this, so that you can create an array filled with a default value. For example, if you want an array that contains five zeros, you can utilize the following syntax:
var zeros = Array(repeating: 0, count: 5) // [0, 0, 0, 0, 0]
Additionally, creating an array using array literals is a clean and concise approach. When you already know the values that should be in the array, this method is both readable and efficient. For instance, to create an array of fruits, you can simply write:
var fruits = ["Apple", "Banana", "Cherry"]
In cases where you need to create an array from another collection, Swift makes this easy. You can initialize an array with an existing collection, such as a set or a sequence. For example:
let set: Set = ["Apple", "Banana", "Cherry"] var fruitArray = Array(set)
When creating arrays containing optional values, you can directly include optional types in the array definition. Here’s how you could create an array that may hold `Int?` values:
var optionalNumbers: [Int?] = [1, nil, 3, nil, 5]
This array can effectively represent the presence or absence of values, allowing for more sophisticated data modeling when necessary.
Swift also aids in creating multidimensional arrays, commonly known as arrays of arrays. A two-dimensional array can be initialized as follows:
var matrix: [[Int]] = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
This structure enables handling complex data representations, such as grids or tables, making arrays highly versatile for various applications.
Combining these methods for creating and initializing arrays not only simplifies the process of data handling but also enhances code readability and maintainability. Understanding how to effectively create arrays is a vital skill for any Swift developer, as it lays the groundwork for more advanced data manipulation techniques.
Accessing and Modifying Array Elements
Accessing and modifying elements within an array in Swift is both intuitive and powerful, thanks to the array’s zero-based indexing system. This means that the first element of an array is accessed using an index of `0`, the second with an index of `1`, and so on. Swift provides a variety of methods for accessing, reading, and updating array elements, allowing developers to manipulate data collections efficiently.
To access elements in an array, you can use subscripting. For instance, if you have an array of integers, you can retrieve a specific value like this:
let numbers = [10, 20, 30, 40, 50] let firstNumber = numbers[0] // Accesses the first element: 10
Additionally, you can use the `count` property to determine the number of elements in an array, which helps prevent out-of-bounds errors when accessing elements:
if numbers.count > 3 { let fourthNumber = numbers[3] // Safely accesses the fourth element: 40 }
When you want to modify an element in an array, you can also use subscripting. Simply assign a new value to the desired index:
var mutableNumbers = [1, 2, 3, 4, 5] mutableNumbers[2] = 99 // Changes the third element to 99 // mutableNumbers is now [1, 2, 99, 4, 5]
If you attempt to access an index this is out of bounds, Swift will throw a runtime error, which is a useful safeguard against programming mistakes. For example:
let outOfBoundsNumber = numbers[10] // This will cause a runtime error
Another aspect of accessing arrays is that you can use the `first` and `last` properties to obtain the first and last elements, respectively. These properties return optional values, as the array could be empty:
if let firstValue = numbers.first { print("First value is: (firstValue)") // Prints the first element } if let lastValue = numbers.last { print("Last value is: (lastValue)") // Prints the last element }
In addition to basic access and modification, Swift arrays also support a variety of methods for inserting or removing elements. For example, you can append new values to the end of an array using the `append` method:
var fruits = ["Apple", "Banana"] fruits.append("Cherry") // fruits is now ["Apple", "Banana", "Cherry"]
To insert an element at a specific position, use the `insert(_:at:)` method:
fruits.insert("Mango", at: 1) // fruits is now ["Apple", "Mango", "Banana", "Cherry"]
Removing elements can be done with the `remove(at:)` method, which deletes an element at the specified index, shifting subsequent elements down to fill the gap:
fruits.remove(at: 2) // Removes "Banana"; fruits is now ["Apple", "Mango", "Cherry"]
There’s also the `removeLast()` method, which will remove the last element of the array:
fruits.removeLast() // fruits is now ["Apple", "Mango"]
Swift arrays also provide functionalities for slicing. You can create a new array with a subset of the elements using the `Array` slice syntax. For example:
let slicedFruits = fruits[0...1] // Slices the array; results in ["Apple", "Mango"]
It is important to note that slices share storage with the original array. Hence, changes to the original array can affect the slice and vice versa, which can lead to unintended side effects if not handled carefully.
With these tools at your disposal, accessing and modifying array elements in Swift becomes a simpler task, so that you can focus on building robust and efficient applications. By understanding how to effectively manipulate arrays, you can manage collections of data efficiently, which is essential for any Swift developer aiming to build performant applications.
Common Array Operations and Methods
When it comes to performing operations on arrays in Swift, the language offers a rich set of methods that enhances its usability and efficiency. These operations can be broadly categorized into functions that manipulate the array’s content, as well as methods that allow you to query its state. Understanding these operations is key to using the full power of arrays in your applications.
One of the most commonly used methods is append(_:)
, which allows you to add a new element to the end of an array. This operation is simple yet powerful, allowing for dynamic data handling. For example:
var numbers = [1, 2, 3] numbers.append(4) // numbers is now [1, 2, 3, 4]
You can also add multiple elements at the same time using append(contentsOf:)
. This method takes another collection and appends its elements in order:
var moreNumbers = [5, 6, 7] numbers.append(contentsOf: moreNumbers) // numbers is now [1, 2, 3, 4, 5, 6, 7]
In addition to appending, Swift provides the insert(_:at:)
method for adding an element at a specific index. This operation can be useful when you need to maintain a particular order:
numbers.insert(0, at: 0) // Inserts 0 at the beginning; numbers is now [0, 1, 2, 3, 4, 5, 6, 7]
Removing elements is just as simpler. The remove(at:)
method allows you to remove an element at a specified index:
numbers.remove(at: 2) // Removes the element at index 2; numbers is now [0, 1, 3, 4, 5, 6, 7]
For convenience, Swift also provides removeLast()
and removeAll()
, which can be used to remove the last element or clear the entire array, respectively:
numbers.removeLast() // Removes the last element; numbers is now [0, 1, 3, 4, 5, 6] numbers.removeAll() // Clears the array; numbers is now []
Furthermore, the first
and last
properties allow you to easily access the first and last elements of the array without needing to specify an index. Both properties return optional values, safeguarding against accessing elements in an empty array:
if let firstNumber = numbers.first { print("First number is (firstNumber)") } if let lastNumber = numbers.last { print("Last number is (lastNumber)") }
For more complex manipulations, Swift offers methods like map(_:)
, filter(_:)
, and reduce(_:)
. These functional programming techniques allow for elegant transformations and reductions of array data:
let doubled = numbers.map { $0 * 2 } // Doubles each number let evens = numbers.filter { $0 % 2 == 0 } // Filters even numbers let sum = numbers.reduce(0, +) // Sums all numbers
Moreover, Swift arrays provide capabilities to sort and reverse their elements with sort()
and reverse()
methods. The sort()
method sorts the array in place, while sorted()
returns a new sorted array:
var unsortedNumbers = [3, 1, 4, 1, 5, 9] unsortedNumbers.sort() // Now unsortedNumbers is [1, 1, 3, 4, 5, 9] let reversedNumbers = unsortedNumbers.reversed() // Returns a reversed view of the array
For context-aware operations, Swift allows for indexed access and slicing. The syntax for slicing arrays is both intuitive and flexible. For example, you can create a subarray using a range:
let slicedArray = unsortedNumbers[1...3] // Creates a slice containing [1, 3, 4]
These methods and operations demonstrate how Swift empowers developers to handle arrays with a high degree of efficiency and clarity. By mastering these common array operations, you can enhance your data manipulation skills, paving the way for more maintainable and efficient applications.
Performance Considerations for Arrays in Swift
When considering the performance characteristics of arrays in Swift, it’s crucial to recognize that arrays are implemented as a contiguous block of memory, which allows for efficient access to elements via their indices. However, this design also introduces trade-offs, particularly in scenarios involving dynamic resizing or modifications that change the array’s structure.
One of the primary performance considerations is the cost associated with resizing an array. When an array grows beyond its current capacity, Swift allocates a new block of memory that’s larger than the original. It then copies the existing elements to this new location, which can be an expensive operation, particularly if the array contains a large number of elements. Swift uses a growth strategy that typically doubles the capacity of the array whenever it needs to resize, minimizing the frequency of these costly operations.
var largeArray = [Int](repeating: 0, count: 10) for i in 0..<1000 { largeArray.append(i) // May cause multiple reallocations }
This illustrates a common scenario where repeated appends can lead to performance degradation if the array frequently exceeds its capacity. For performance-critical applications, preallocating the array with a known size or using `reserveCapacity(_:)` can mitigate this issue:
var preallocatedArray = [Int]() preallocatedArray.reserveCapacity(1000) // Reserves space for 1000 elements for i in 0..<1000 { preallocatedArray.append(i) // No reallocations needed }
Another important aspect of array performance is related to element access and iteration. Accessing elements by their index is an O(1) operation, allowing for fast lookups. However, iterating over an array is O(n), where n is the number of elements. This means that as the size of the array increases, the time taken to process all elements in a loop will grow linearly:
for number in largeArray { print(number) // O(n) operation }
As for memory usage, Swift arrays are generally efficient, but it is important to note that they can consume more memory than strictly necessary due to their capacity management. When you remove elements from an array, the capacity does not shrink automatically, leading to potential memory overhead. To reclaim this unused memory, you can use the `shrinkToFit()` method, which resizes the array to fit its current number of elements:
largeArray.removeLast(500) // Removes elements but doesn't shrink capacity largeArray.shrinkToFit() // Now the capacity matches the current size
In terms of concurrency, the value type nature of arrays means that they can be safely used across multiple threads without the risk of race conditions, as each thread will operate on its own copy of the array. However, when passing large arrays between threads, it’s still important to think the potential performance impact of copying large amounts of data.
Overall, the performance of arrays in Swift is optimized for typical usage patterns, but understanding the underlying behavior—particularly with respect to memory allocation, resizing, and iteration—is vital for writing efficient and effective Swift code. By considering these performance considerations, developers can make more informed decisions that lead to better application performance.