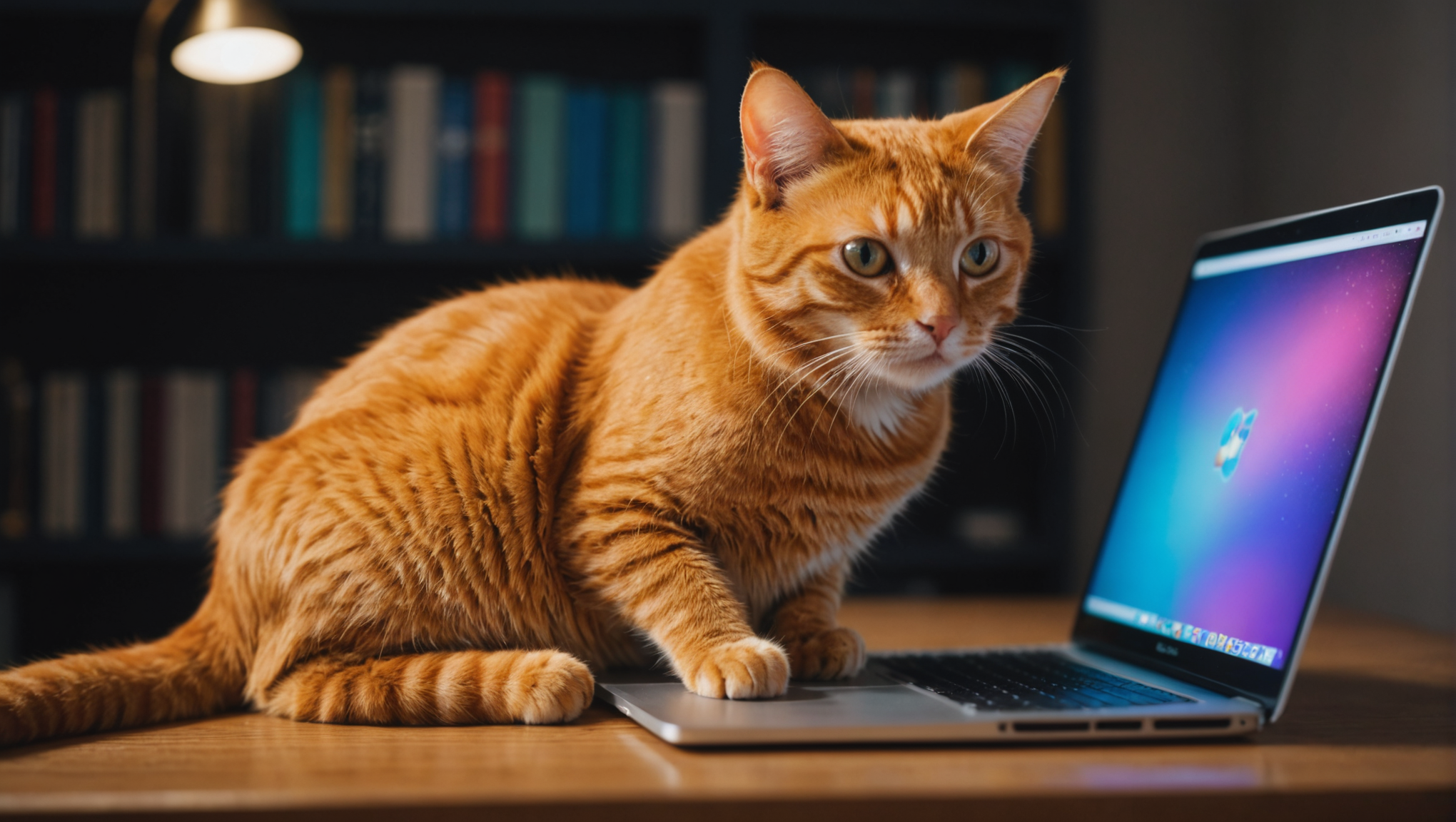
JavaScript Regular Expressions for Pattern Matching
Regular expressions (often abbreviated as regex or regexp) are powerful tools for matching patterns within strings. In JavaScript, they provide a means to search for specific sequences of characters or to perform complex transformations on text using a concise syntax. Understanding how regex works in JavaScript is essential for developers who wish to manipulate strings efficiently and effectively.
At the core of regex functionality is the ability to define a search pattern using a combination of characters and special symbols. For example, if you are looking to find occurrences of the word “cat” in a sentence, you could use the simple pattern cat
. However, the real strength of regular expressions lies in their capacity to work with more complex patterns that can match a wide variety of strings.
In JavaScript, regular expressions can be created using either the RegExp
constructor or by using regex literals. The latter is often favored for its readability and ease of use. Here’s an example of each method:
// Using a regex literal const regexLiteral = /cat/; // Using the RegExp constructor const regexConstructor = new RegExp('cat');
Once created, regex patterns can be employed in various ways, such as searching for matches, replacing text, or validating input formats. The test()
method checks if a pattern exists within a given string, returning true or false. For instance:
const str = 'The cat sat on the mat.'; const hasCat = regexLiteral.test(str); // true
Additionally, the exec()
method retrieves the matches when matching a string against a regex pattern. This method returns an array containing the matched text and its position in the original string:
const match = regexLiteral.exec(str); console.log(match); // ['cat', index: 4, input: 'The cat sat on the mat.', groups: undefined]
Understanding the nuances of regular expressions in JavaScript opens a door to a wide array of string manipulation capabilities. With practice and familiarity, developers can harness regex for tasks ranging from simple searches to complex validation and parsing tasks, making it an invaluable skill in a JavaScript developer’s toolkit.
Common Syntax and Special Characters
Regular expressions in JavaScript are built upon a syntax that includes both common characters and a set of special characters, each serving unique purposes in pattern matching. Mastery of these components especially important for crafting effective regex patterns that meet specific string manipulation needs.
At its simplest, a regular expression can include literal characters that represent themselves. For example, the regex /dog/
will match any occurrence of the string “dog” in a larger text. However, when you explore the world of special characters, the complexity and power of regex begin to unfold.
One of the most foundational elements of regex is the use of anchors. The caret (^
) symbol asserts the position at the start of a string, while the dollar sign ($
) asserts the position at the end. For instance:
const startWithHello = /^Hello/; // matches 'Hello' at the start of a string const endWithWorld = /world$/; // matches 'world' at the end of a string
Another important aspect is the use of quantifiers, which specify how many instances of a character or group should be present. The asterisk (*
) matches zero or more occurrences, the plus sign (+
) matches one or more, and the question mark (?
) matches zero or one. For example:
const zeroOrMore = /ba*/; // matches 'b', 'ba', 'baa', etc. const oneOrMore = /ba+/; // matches 'ba', 'baa', but not 'b' const zeroOrOne = /ba?/; // matches 'b' or 'ba'
Character classes offer yet another layer of flexibility, so that you can define a set of characters for matching. For example, [abc]
matches any single occurrence of ‘a’, ‘b’, or ‘c’. You can also specify ranges, such as [a-z]
for any lowercase letter. Here’s how you can use character classes:
const vowels = /[aeiou]/; // matches any vowel const digits = /[0-9]/; // matches any digit const alphabets = /[A-Za-z]/; // matches any alphabet
To match a specific number of occurrences, you can use curly braces ({n}
), where n
is the exact number of times the preceding character or group should appear. For example:
const exactlyThree = /[abc]{3}/; // matches 'aaa', 'abc', 'bca', etc., but not 'ab' const atLeastTwo = /[abc]{2,}/; // matches 'aa', 'b', 'abc', etc.
Special characters further enhance regex functionality. The dot (.
) matches any single character except for newline characters, while the backslash () serves as an escape character to give special meaning to the character that follows it or to escape special characters.
const anyCharacter = /.at/; // matches 'cat', 'hat', 'bat', etc. const escapeExample = /d/; // matches any digit, equivalent to [0-9]
Finally, regex can be combined with logical operations using the pipe (|
) to represent “or” conditions. For example, <code/dog|cat/ matches either “dog” or “cat”. This can be useful for constructing patterns that need to match multiple possibilities within a single expression.
const petChoice = /dog|cat/; // matches either 'dog' or 'cat'
With the understanding of these common syntax elements and special characters, you can start building more sophisticated patterns that fit your specific string matching needs, making JavaScript regular expressions an essential tool in your programming arsenal.
Using the RegExp Object and Literal Notation
the regex /cat|dog/ will match either “cat” or “dog” in a string. This allows for more flexible pattern matching when dealing with multiple possibilities.
const pet = /cat|dog/; const str1 = 'I have a cat.'; const str2 = 'I have a dog.'; console.log(pet.test(str1)); // true console.log(pet.test(str2)); // true
Using regular expressions effectively involves a deep understanding of both the syntax and the unique characters available. Once you have grasped these principles, you can create highly efficient patterns for matching text, validating user input, or parsing data strings. The next step is to dive deeper into the practical applications of regular expressions, where their real power and utility come to light. This knowledge will empower you to solve complex problems with simple, elegant regex solutions.
Pattern Matching Techniques and Examples
the regex /cat|dog/ would match either “cat” or “dog” in a string. This capability allows you to create versatile patterns that can match multiple possibilities, which is particularly useful when dealing with varied input formats or searching through large text bodies.
With a solid understanding of the syntax and special characters, we can delve into the practical application of regular expressions through a variety of matching techniques. These techniques illustrate how regex can be effectively employed to handle different string manipulation tasks.
One common technique is validating patterns against specific formats, such as email addresses or phone numbers. For instance, to match a simple email structure, you might use the following regex pattern:
const emailPattern = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}$/;
This pattern ensures that the string starts with alphanumeric characters, followed by an ‘@’ symbol, a domain name, and a top-level domain, effectively capturing a broad range of valid email formats.
Another practical example is extracting specific information from a string using capturing groups, which are defined by parentheses. For instance, if you want to extract the area code and the phone number from a format like “(123) 456-7890”, you can use:
const phonePattern = /^((d{3})) (d{3})-(d{4})$/;
Using this pattern, the exec() method will return an array containing each captured group:
const phoneStr = '(123) 456-7890'; const phoneMatch = phonePattern.exec(phoneStr); console.log(phoneMatch); // ['(123) 456-7890', '123', '456', '7890']
Moreover, regex can be pivotal in text replacement tasks. The String.prototype.replace() method can leverage regex to replace substrings that match a certain pattern. For example, to replace all instances of “cat” with “dog” in a string:
const text = 'The cat sat on the mat. The cat is happy.'; const newText = text.replace(/cat/g, 'dog'); console.log(newText); // 'The dog sat on the mat. The dog is happy.'
In this case, the ‘g’ flag is used to indicate a global search, ensuring that all occurrences of “cat” are replaced, not just the first one.
Another challenge regex can tackle is the removal of unwanted characters or formatting inconsistencies. For instance, if you have a string with extra spaces or punctuation that need to be cleaned up, you can use a regex pattern to target these undesired elements:
const messyString = 'Hello!!! That's a test... '; const cleanedString = messyString.replace(/[!.s]+/g, ' ').trim(); console.log(cleanedString); // 'Hello That's a test'
This example demonstrates how regex can simplify the process of sanitizing input strings, which can be especially helpful when preparing data for further processing or display.
By employing these techniques and understanding how to effectively use regex patterns, JavaScript developers can streamline their string manipulation tasks and enhance the robustness of their applications. Whether it is for validation, extraction, replacement, or cleanup, mastering regex opens up a realm of possibilities for efficient coding practices.
Practical Applications of Regular Expressions in JavaScript
the regex /cat|dog/ will match either “cat” or “dog”. This flexibility allows you to create complex search patterns that adapt to various scenarios.
As you delve deeper into the practical applications of regular expressions in JavaScript, their versatility becomes evident. Regular expressions are not merely a tool for pattern matching; they facilitate data validation, text parsing, and manipulation, making them indispensable in web development and data processing.
One of the most common applications of regex is in validating user input. For instance, if you’re creating a form that requires users to enter a valid email address, a regex can help ensure that the input matches the expected format. Here’s how you can implement such validation:
const emailPattern = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]{2,}$/; const isValidEmail = emailPattern.test('[email protected]'); // true
This regex checks for a standard email structure, ensuring that it contains characters before the ‘@’ symbol, a domain name, and a proper top-level domain.
Another practical use of regular expressions lies in string manipulation. The replace() method in JavaScript can leverage regex to conduct bulk text replacements. For instance, if you want to sanitize user input by removing all non-alphanumeric characters, you can employ a regex pattern in conjunction with replace:
const userInput = 'Hello @World! 123'; const sanitizedInput = userInput.replace(/[^a-zA-Z0-9 ]/g, ''); // 'Hello World 123'
In this example, the regex /[^a-zA-Z0-9 ]/ matches any character that’s not a letter, digit, or space, effectively filtering out unwanted symbols.
Parsing complex data formats, such as logs or CSV files, also benefits greatly from regular expressions. By using regex, developers can extract meaningful information from strings with precision. For example, if you have a log entry and need to extract the timestamp, log level, and message, you can use a regex pattern as follows:
const logEntry = '[2023-10-12 10:00:00] INFO: User logged in'; const logPattern = /^[(.*?)] (.*?): (.*)$/; const match = logEntry.match(logPattern); console.log(match[1]); // '2023-10-12 10:00:00' console.log(match[2]); // 'INFO' console.log(match[3]); // 'User logged in'
Here, the regex captures the timestamp, log level, and message by using capturing groups, making it simple to process the log entries systematically.
Moreover, regular expressions serve an important role in search-and-replace functionalities, commonly found in text editors or code IDEs. Developers can define patterns to find specific code snippets or text and replace them with new content. This capability not only accelerates the development process but also helps maintain consistency across codebases.
const codeSnippet = 'function oldFunction() {}'; const updatedSnippet = codeSnippet.replace(/oldFunction/g, 'newFunction'); console.log(updatedSnippet); // 'function newFunction() {}'
In this code, the regex pattern matches all instances of “oldFunction” in the string, allowing for efficient code refactoring.
The practical applications of regular expressions in JavaScript extend far beyond basic pattern matching. They empower developers to implement robust validation, enhance data processing, facilitate string manipulation, and streamline search-and-replace tasks. By mastering regex, developers can unlock a new level of efficiency and precision in their JavaScript programming endeavors.