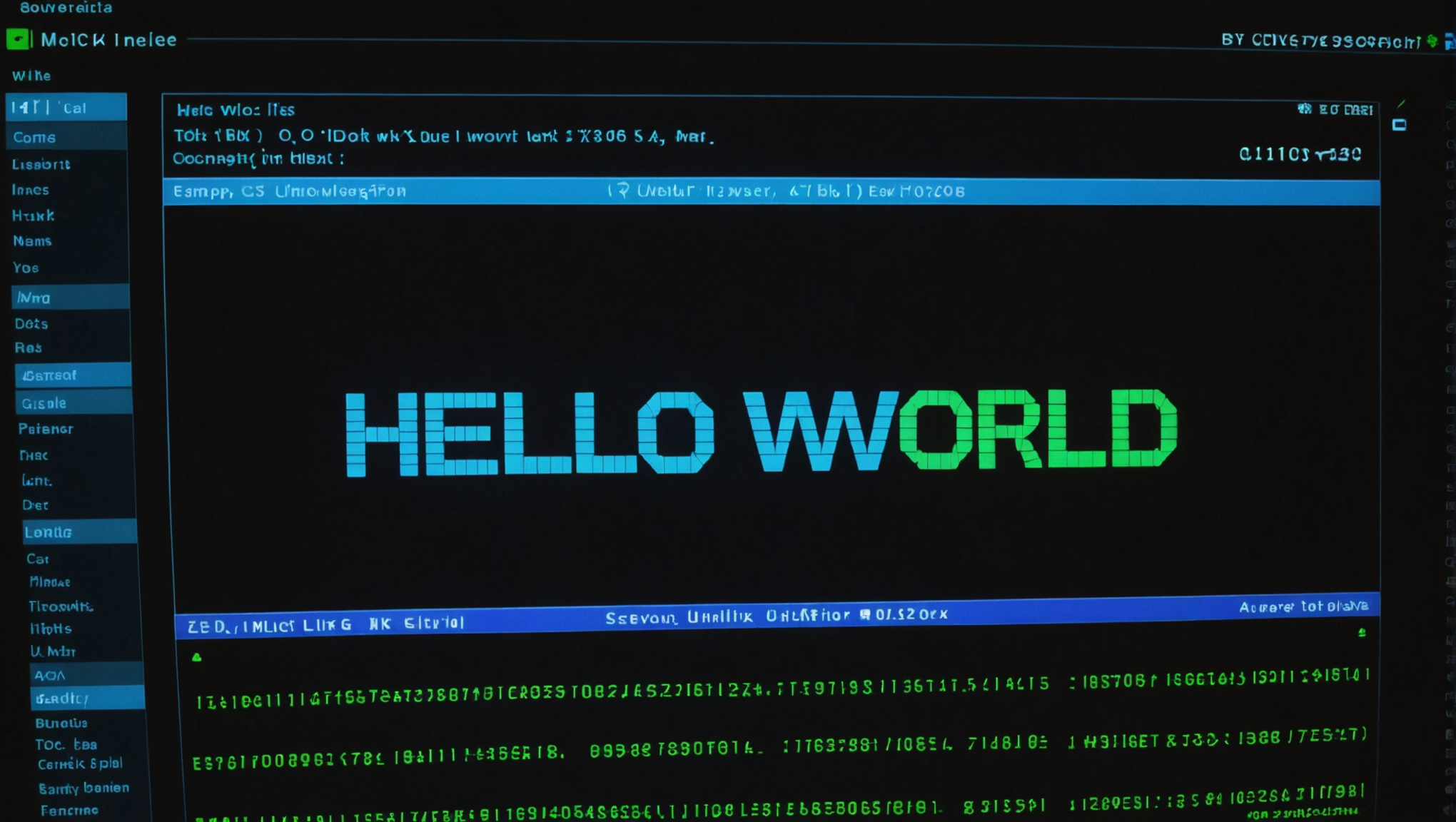
Stream Editing with Sed in Bash
When working with text data in Bash, sed, short for stream editor, becomes an indispensable tool. It excels in parsing and transforming text in a pipeline, allowing you to manipulate data efficiently without the need for complex programming. At its core, sed operates in a non-interactive manner; it reads input, carries out specified edits, and outputs the result, all while maintaining performance and simplicity.
sed is particularly powerful for several reasons:
- It processes streams of data quickly, making it ideal for large files or data streams.
- Beyond simple substitutions, sed can perform complex text transformations, including deletions, insertions, and even conditional processing.
- Since it works seamlessly with standard input and output, it can be easily combined with other Unix commands in a pipeline.
At its foundation, sed operates using a set of commands that specify how to manipulate the input text. The general syntax of a basic sed command looks like this:
sed 'command' file
Here, command represents the editing operation you wish to perform, and file is the target text file. If no file is specified, sed reads from standard input, which opens up possibilities for dynamic processing where data is piped in from other commands.
One of the fundamental commands used in sed is s///
, which stands for substitute. This command allows you to replace occurrences of a specified string with another string throughout the input:
echo "Hello World" | sed 's/World/Unix/'
In this example, the output will be Hello Unix
, demonstrating how sed can alter text in-stream without saving to an intermediate file.
Understanding sed requires familiarity with its addressing system, which allows you to target specific lines or patterns. The addressing can be as simple as line numbers or as complex as regular expressions, giving you precise control over the text you want to manipulate.
Another vital point is the ability to chain commands, enabling a series of transformations to occur sequentially. This capability allows for powerful scripting and automation of text processing tasks, solidifying sed as a cornerstone of text manipulation in the Unix/Linux toolkit.
As you delve deeper into sed, you’ll discover its rich functionality, including the use of flags and options that enhance its capabilities, accommodating a wide range of text processing needs. The journey into the world of stream editing with sed is just beginning, and as you explore its depths, you’ll find it to be a remarkably potent ally in your Bash toolkit.
Basic Sed Commands and Syntax
The basic syntax of sed commands extends beyond the simple substitution provided by the s/// command. Sed supports a variety of commands, each serving different purposes, and understanding these commands very important for effective stream editing.
Another common sed command is d, which is used for deleting specific lines from the input. For example, if you want to delete the second line of a text file, you would use:
sed '2d' filename.txt
This command will read filename.txt
, remove the second line, and output the remaining lines to standard output.
You can also use the p command to print specific lines. By combining addressing with the print command, you can selectively display lines. Here’s an example that prints only the first line of the input:
sed -n '1p' filename.txt
The -n
option tells sed not to print anything by default, so that you can control output more precisely with the p
command.
In addition to substitutions and deletions, sed can also perform insertions using the a (append) and i (insert) commands. To append a line after the third line of a file, use:
sed '3a New line of text' filename.txt
Conversely, to insert a line before the second line, you would do:
sed '2i Inserted line of text' filename.txt
The escape character at the end of the line ensures that the text being inserted goes onto the next line in the command.
Another useful command is y, which performs character translation. This can be employed to change specific characters in the text. For instance, the command below translates all lowercase vowels to uppercase:
echo "hello world" | sed 'y/aeiou/AEIOU/'
This will yield the output hEllO wOrld
, showcasing how sed can manipulate individual characters efficiently.
Mastering these basic commands and understanding their syntax allows for more complex text-processing tasks. By using sed’s powerful features, you can tackle a wide array of text manipulation challenges, setting the stage for more advanced techniques in stream editing.
Common Use Cases for Sed
When it comes to practical applications of sed, it shines in various scenarios, making it an essential skill for anyone working with text data in Bash. Here are some common use cases where sed proves its mettle.
One common use case is text substitution in configuration files. When you need to change a specific value in a settings file, sed can do this in a single command. For example, if you want to update a database connection string in a config file, you can use:
sed -i 's/old_connection_string/new_connection_string/' config.txt
The -i option allows you to edit the file in place, replacing the old connection string with the new one directly in the file.
Another frequent scenario is the cleaning of log files. Often, logs can contain sensitive information that needs redaction. For instance, if you need to remove all occurrences of an email address pattern from a log file, sed can help you achieve this efficiently:
sed -i 's/[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+.[a-zA-Z]+//g' logfile.txt
This command uses a regular expression to match email addresses and replace them with nothing, thereby removing them from the log.
Text formatting is another area where sed excels. Suppose you have a CSV file and you want to change all commas to tabs for better readability or further processing. You can do this easily with:
sed 's/,/\t/g' data.csv
By replacing commas with tab characters, you can transform the data for use in applications that accept tab-delimited input.
Sed is also invaluable for batch processing multiple files. If you want to replace a string across all text files in a directory, a simple combination with a wildcard can do the trick:
sed -i 's/old_string/new_string/g' *.txt
This command will go through every .txt file in the current directory, replacing all instances of old_string with new_string, streamlining your workflow significantly.
Moreover, when working with structured files like XML or JSON, sed can help in limited scenarios where complex parsing isn’t required. For instance, to remove all comments from an XML file, you could use:
sed '//d' file.xml
This command deletes all lines between the XML comment tags, effectively cleaning up the file for further processing.
In addition to these examples, another particularly effective use case for sed is to extract specific data from a file. For example, if you want to pull out all lines containing a certain keyword from a document, you can employ:
sed -n '/keyword/p' document.txt
The -n option suppresses automatic printing, and the /keyword/p command tells sed to print only the lines that match the specified pattern.
These common use cases illustrate how sed can solve a range of text processing challenges with elegance and efficiency. By mastering these applications, you can leverage sed to enhance your Bash scripting capabilities significantly.
Advanced Sed Techniques and Options
As you delve deeper into the capabilities of sed, you’ll encounter more advanced techniques that extend its utility beyond basic text processing. These techniques allow for intricate manipulations, making sed a versatile tool for diverse scripting needs. One particularly powerful feature of sed is its ability to work with regular expressions, enabling complex pattern matching and text transformations.
One of the key aspects of sed’s functionality is its support for extended regular expressions (ERE). By using the -E flag, you can unlock additional regex features, such as the use of alternation (|) and grouping. For example, if you want to replace either “cat” or “dog” with “pet” in a text file, you can execute:
sed -E 's/(cat|dog)/pet/g' animals.txt
This command showcases how sed can match multiple patterns in a single substitution, demonstrating its prowess in handling complex text scenarios.
Another advanced technique involves the use of hold space—an additional buffer that sed maintains alongside the pattern space. This feature allows for more sophisticated editing operations, such as rearranging text. Consider a scenario where you want to swap the first and second lines of a file:
sed '1h; 2H; 2d; x; 1d; x' file.txt
Here’s how this command works:
- The first part (1h) copies the first line into the hold space.
- The second part (2H) appends the second line to the hold space.
- The 2d command deletes the second line from the pattern space.
- The x command exchanges the contents of the pattern space and hold space.
- Finally, the 1d command deletes the first line from the pattern space, effectively leaving the desired lines swapped.
This technique highlights the power of sed’s hold space, enabling operations that would be difficult to perform with simple commands alone.
Another advanced option is the use of the -n flag combined with the p command for selective output. This approach allows for conditional printing based on certain criteria, which can be extraordinarily helpful in complex scripts. For example, suppose you want to print only the lines that contain a specific pattern while ensuring that the output does not include any other lines:
sed -n '/pattern/p' file.txt
This command is particularly useful for filtering large data sets, offering a streamlined approach to extracting relevant information while minimizing clutter in your output.
Moreover, the ability to execute multiple sed commands in a single invocation is another strength. You can group commands using the -e option, which allows you to apply several transformations concurrently. For instance, if you want to replace “foo” with “bar” and delete all empty lines, you could use:
sed -e 's/foo/bar/g' -e '/^$/d' file.txt
In this command, the first expression substitutes “foo” with “bar,” while the second expression deletes any lines that are empty. This streamlined approach not only saves time but also simplifies your Bash scripts.
As you advance in your sed mastery, understanding the nuances of command flags, regular expressions, and hold space manipulation will tremendously enhance your text processing capabilities. The more you experiment with these advanced techniques, the more you’ll appreciate sed as an ally in the realm of stream editing, capable of handling even the most intricate scenarios with finesse.
Best Practices and Tips for Using Sed
To maximize your efficiency and effectiveness when using sed, adhering to best practices can make a significant difference in both performance and maintainability of your scripts. Here are some key tips to keep in mind while wielding the power of sed.
1. Backup Your Files
When editing files in place with the -i
option, it’s prudent to create backups. You can specify a backup extension to ensure you have the original file preserved, so that you can easily revert if necessary:
sed -i.bak 's/original/replacement/g' filename.txt
In this example, a backup of filename.txt
is created as filename.txt.bak
before applying the substitution.
2. Test Your Commands
Before running sed commands on critical data, especially when using the -i
option, it is wise to test your commands on sample data. You can redirect the output to the console or to a temporary file to verify the results:
sed 's/old/new/g' filename.txt > temp.txt
This method allows you to ensure that the command behaves as expected without risking the integrity of the original file.
3. Use Meaningful Regular Expressions
When crafting your regular expressions, clarity is key. Avoid overly complex patterns that can be difficult to read and maintain. Commenting on your regex can enhance readability and help others (or your future self) understand your intentions:
sed -E 's/(foo|bar)/replacement # replacing either foo or bar/g' filename.txt
Using the -E
option allows for extended regex features, which can simplify your expressions while maintaining comprehensibility.
4. Chain Commands Wisely
When performing multiple transformations, ponder chaining your commands using the -e
option. This keeps your sed script organized and enables batch processing in a single pass:
sed -e 's/foo/bar/g' -e '/^$/d' filename.txt
This example replaces all instances of “foo” with “bar” and removes empty lines, showcasing a clean and efficient structure.
5. Utilize Hold Space for Complex Operations
When faced with intricate editing tasks, taking advantage of sed’s hold space can provide a profound increase in capability. It allows you to save and manipulate text between commands effectively, enabling more sophisticated workflows:
sed '1h; 2H; 2d; x; 1d; x' filename.txt
This command demonstrates the process of swapping the first two lines, highlighting the power of hold space manipulation.
6. Keep Performance in Mind
When working with large files, performance can become an issue. Utilize sed’s efficiency by minimizing unnecessary substitutions or deletions. For example, if you only need to replace a specific string in a subset of lines, use addressing to target only those lines:
sed '10,20s/foo/bar/g' filename.txt
This command ensures that only lines 10 through 20 are processed, conserving resources and time.
7. Document Your Scripts
As with any coding endeavor, documentation is important. Comment your sed scripts to explain complex expressions, command sequences, or the overall purpose. This practice not only aids your understanding but also assists collaborators in grasping your workflows:
# Replace foo with bar and remove empty lines sed -e 's/foo/bar/g' -e '/^$/d' filename.txt
By following these best practices, you can harness the true power of sed, ensuring your text processing tasks are executed efficiently and effectively. The combination of careful planning, robust testing, and clear documentation will transform your sed usage into an art form, so that you can manage and manipulate text with confidence.