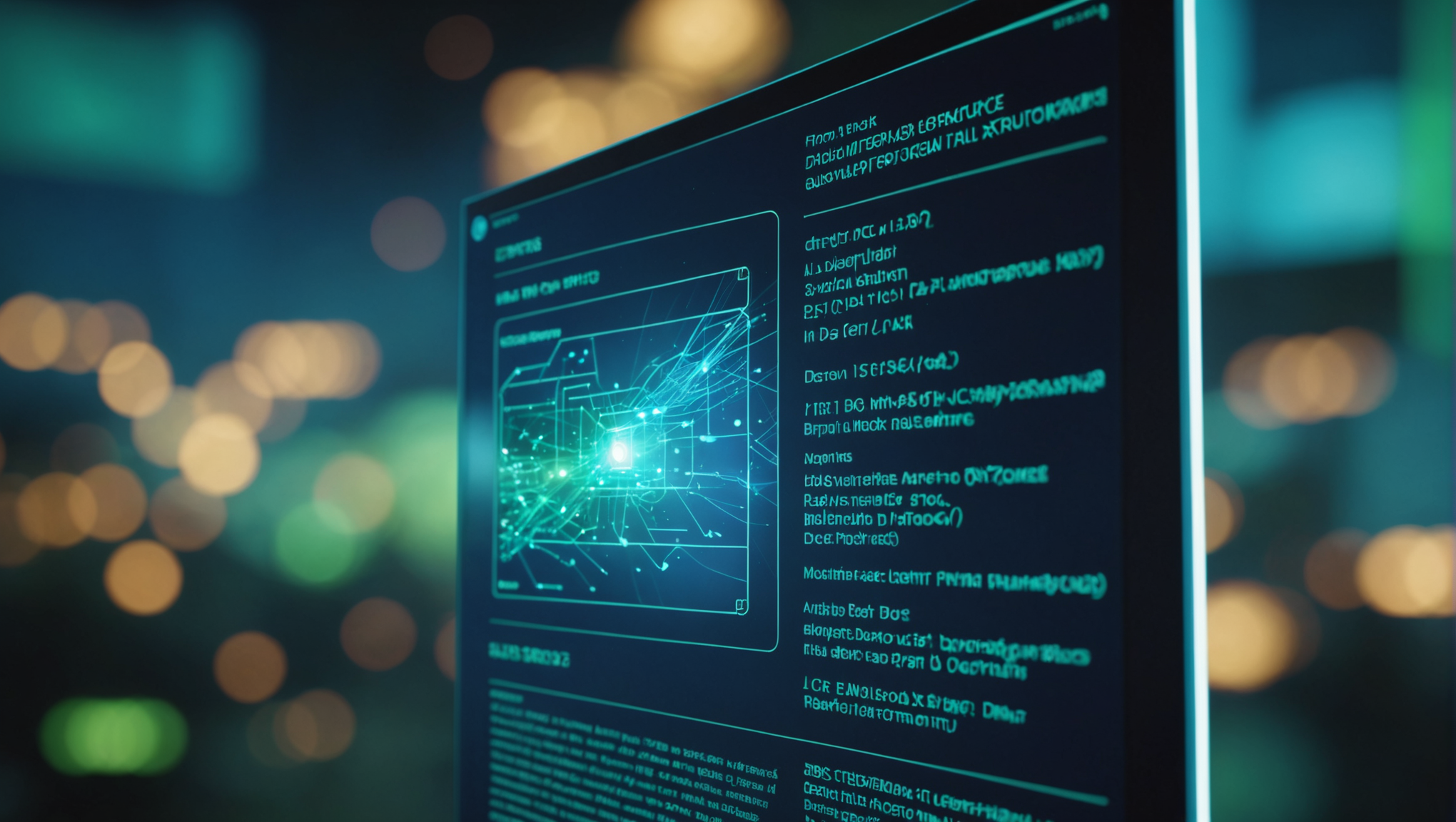
Java and XML: Parsing and Processing
XML, which stands for Extensible Markup Language, serves as a flexible way to create information formats and share structured data across different systems. At its core, XML is designed to be both human-readable and machine-readable, allowing for easy data interchange between disparate systems.
The structure of XML is based on a hierarchy of elements that are defined by tags. Each XML document starts with a declaration that specifies the XML version and, optionally, the encoding. For example:
<?xml version="1.0" encoding="UTF-8"?>
Following the declaration, the document consists of a single root element that contains all other elements. Elements are defined using opening and closing tags, which can contain attributes that provide additional information. Here’s a simple example of an XML document:
<note> <to>Tove</to> <from>Jani</from> <heading>Reminder</heading> <body>Don't forget me this weekend!</body> </note>
In this example, is the root element, while , , , and are child elements. Each element can contain text, attributes, and even nested elements, providing a robust way to represent complex data structures.
Attributes are defined within the opening tag of an element and provide additional details. For instance:
<note date="2023-10-01"> <to>Tove</to> <from>Jani</from> <heading>Reminder</heading> <body>Don't forget me this weekend!</body> </note>
In this modified example, the element has an attribute called date that provides contextual information about when the note was created.
It’s essential to adhere to a strict syntax when writing XML. For instance, all elements must be properly nested, and tags must be closed correctly. Characters like cannot appear in text unless they are escaped as < and >, respectively. Ensuring well-formed XML is important for successful parsing and processing.
XML also supports comments, which can be added for documentation purposes. Comments are not processed by XML parsers and are written as follows:
<!-- That is a comment -->
This structure and syntax make XML a powerful tool for data representation. Understanding these fundamentals is critical for effectively using Java to parse and manipulate XML data in various applications.
Java XML Parsing Techniques
When it comes to parsing XML in Java, there are several techniques available that cater to different needs and use cases. Each parsing method has its unique advantages and trade-offs, and understanding these can significantly affect the performance and maintainability of your applications.
Java provides several powerful libraries for XML parsing, with the most prominent being the Document Object Model (DOM) and Simple API for XML (SAX). Additionally, Java Architecture for XML Binding (JAXB) offers a way to bind XML to Java objects. In this section, we will delve into the DOM and SAX parsing techniques, comparing their functionality and performance.
The DOM parser reads the entire XML document and constructs a complete in-memory tree representation of the document. This allows for easy navigation and manipulation of XML data, but it comes at the cost of higher memory consumption. A DOM parser is ideal when you need random access to elements or need to modify the XML document. Here is a simple example of using a DOM parser:
import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.NodeList; public class DOMParserExample { public static void main(String[] args) { try { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("note.xml"); // Normalize the document document.getDocumentElement().normalize(); // Get the root element Element root = document.getDocumentElement(); System.out.println("Root element: " + root.getNodeName()); // Get child elements NodeList nodeList = document.getElementsByTagName("to"); for (int i = 0; i < nodeList.getLength(); i++) { Element element = (Element) nodeList.item(i); System.out.println("To: " + element.getTextContent()); } } catch (Exception e) { e.printStackTrace(); } } }
On the other hand, the SAX parser operates in a fundamentally different manner. It reads the XML document sequentially and triggers events as it encounters elements. This event-driven model allows for lower memory usage, making SAX a preferred choice for large XML files where you only need to read data without modifying the content. However, SAX does not allow for random access to the XML structure, which can be a limitation in certain scenarios. Below is a simple example demonstrating SAX parsing:
import org.xml.sax.Attributes; import org.xml.sax.SAXException; import org.xml.sax.helpers.DefaultHandler; import javax.xml.parsers.SAXParser; import javax.xml.parsers.SAXParserFactory; public class SAXParserExample { public static void main(String[] args) { try { SAXParserFactory factory = SAXParserFactory.newInstance(); SAXParser saxParser = factory.newSAXParser(); DefaultHandler handler = new DefaultHandler() { boolean to = false; public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException { if (qName.equalsIgnoreCase("to")) { to = true; } } public void characters(char ch[], int start, int length) throws SAXException { if (to) { System.out.println("To: " + new String(ch, start, length)); to = false; } } }; saxParser.parse("note.xml", handler); } catch (Exception e) { e.printStackTrace(); } } }
The choice between DOM and SAX depends largely on your specific needs. If your application requires accessing and manipulating XML data extensively, DOM is advantageous despite its higher memory usage. Conversely, if you are dealing with large datasets and simply need to read information, SAX is the clear winner due to its lower resource footprint. Understanding these parsing techniques will equip you with the tools to efficiently handle XML data in your Java applications.
DOM vs. SAX: Choosing the Right Parser
When choosing between DOM and SAX for XML parsing in Java, it is crucial to evaluate the specific requirements of your application. Each parser has unique strengths and weaknesses that can significantly influence performance, memory usage, and ease of use.
The Document Object Model (DOM) parser is particularly beneficial when you need to work with the entire XML document structure. Once parsed, DOM creates an in-memory representation of the XML tree, so that you can easily navigate, edit, and manipulate elements. For example, you can add or remove nodes, change attributes, or retrieve information with simpler method calls. However, this flexibility comes with a trade-off: DOM consumes more memory and can be slower for large XML files since it loads the entire document into memory.
Think a scenario where you might want to modify an XML document. Here’s how you can utilize the DOM parser to change a value:
import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import javax.xml.transform.Transformer; import javax.xml.transform.TransformerFactory; import javax.xml.transform.dom.DOMSource; import javax.xml.transform.stream.StreamResult; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.NodeList; public class ModifyDOMExample { public static void main(String[] args) { try { DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse("note.xml"); // Modify the XML content NodeList nodeList = document.getElementsByTagName("body"); if (nodeList.getLength() > 0) { Element bodyElement = (Element) nodeList.item(0); bodyElement.setTextContent("Don't forget me this weekend! (Updated)"); } // Save the changes to the XML file TransformerFactory transformerFactory = TransformerFactory.newInstance(); Transformer transformer = transformerFactory.newTransformer(); DOMSource source = new DOMSource(document); StreamResult result = new StreamResult("note.xml"); transformer.transform(source, result); System.out.println("XML file updated successfully."); } catch (Exception e) { e.printStackTrace(); } } }
On the other hand, SAX (Simple API for XML) employs an event-driven approach, reading the document sequentially. This means SAX doesn’t store the entire XML structure in memory; instead, it generates events for start and end tags, as well as for character data. This makes SAX highly suitable for parsing large XML files where memory consumption is a concern. However, the downside is that you cannot traverse back to elements once they have been processed, limiting the flexibility of data access.
For instance, think a situation where you only need to extract specific pieces of information from a large XML document without modifying it. You would use SAX like this:
import org.xml.sax.Attributes; import org.xml.sax.SAXException; import org.xml.sax.helpers.DefaultHandler; import javax.xml.parsers.SAXParser; import javax.xml.parsers.SAXParserFactory; public class FilterSAXExample { public static void main(String[] args) { try { SAXParserFactory factory = SAXParserFactory.newInstance(); SAXParser saxParser = factory.newSAXParser(); DefaultHandler handler = new DefaultHandler() { boolean from = false; public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException { if (qName.equalsIgnoreCase("from")) { from = true; } } public void characters(char ch[], int start, int length) throws SAXException { if (from) { System.out.println("From: " + new String(ch, start, length)); from = false; } } }; saxParser.parse("note.xml", handler); } catch (Exception e) { e.printStackTrace(); } } }
The choice between DOM and SAX parsing in Java hinges on the specific use case at hand. If your application demands extensive interaction with the XML data, DOM is the way to go. However, for performance-sensitive applications that handle large XML documents without requiring modifications, SAX is the more efficient option. By understanding these differences, you can select the right parser that aligns with your application’s needs, ensuring optimal performance and resource management.
Processing XML with JAXB
JAXB, or Java Architecture for XML Binding, is a powerful framework that allows developers to convert Java objects to XML and vice versa seamlessly. It simplifies the process of working with XML data in Java applications by providing an easy way to map XML elements to Java classes, allowing developers to focus more on the business logic than on the intricacies of XML parsing. That is particularly beneficial in enterprise applications where XML data interchange is prevalent.
To use JAXB, the first step is to define your Java classes that will represent the XML structure. Each class will typically correspond to an XML element, and you can use JAXB annotations to specify how each field should be mapped to XML. For instance, consider the following Java class that represents a note:
import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Note { private String to; private String from; private String heading; private String body; // Getters and setters @XmlElement public String getTo() { return to; } public void setTo(String to) { this.to = to; } @XmlElement public String getFrom() { return from; } public void setFrom(String from) { this.from = from; } @XmlElement public String getHeading() { return heading; } public void setHeading(String heading) { this.heading = heading; } @XmlElement public String getBody() { return body; } public void setBody(String body) { this.body = body; } }
In this example, the Note
class is annotated with @XmlRootElement
, indicating that it represents the root element of the XML. Each field in the class is annotated with @XmlElement
, which tells JAXB to map these fields to corresponding XML elements.
Once you have defined your Java classes, you can marshal (write) Java objects to XML and unmarshal (read) XML back into Java objects. Marshalling is simpler with JAXB. Here’s how you can convert a Note
object to XML:
import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBException; import javax.xml.bind.Marshaller; public class MarshalExample { public static void main(String[] args) { try { Note note = new Note(); note.setTo("Tove"); note.setFrom("Jani"); note.setHeading("Reminder"); note.setBody("Don't forget me this weekend!"); JAXBContext context = JAXBContext.newInstance(Note.class); Marshaller marshaller = context.createMarshaller(); marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE); // Marshal the Note object to XML marshaller.marshal(note, System.out); } catch (JAXBException e) { e.printStackTrace(); } } }
In this example, we create a Note
object, set its properties, and then marshal it to XML using Marshaller
. The output will be a well-formatted XML representation of the note.
Unmarshalling is just as simple. Here’s how you can read an XML file and convert it back into a Note
object:
import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBException; import javax.xml.bind.Unmarshaller; import java.io.File; public class UnmarshalExample { public static void main(String[] args) { try { File file = new File("note.xml"); JAXBContext context = JAXBContext.newInstance(Note.class); Unmarshaller unmarshaller = context.createUnmarshaller(); // Unmarshal the XML file to a Note object Note note = (Note) unmarshaller.unmarshal(file); System.out.println("To: " + note.getTo()); System.out.println("From: " + note.getFrom()); System.out.println("Heading: " + note.getHeading()); System.out.println("Body: " + note.getBody()); } catch (JAXBException e) { e.printStackTrace(); } } }
In this unmarshal example, we read an XML file named note.xml
and convert it back into a Note
object. The process is efficient and simpler, demonstrating the elegance of JAXB in handling XML data.
JAXB also supports complex XML structures, including nested elements and collections. By using JAXB, developers can manage XML data more intuitively and reduce the boilerplate code typically associated with XML parsing in Java. This makes JAXB an invaluable tool in any Java developer’s toolkit, particularly in applications that heavily interact with XML data.
Handling Errors and Exceptions in XML Parsing
When working with XML in Java, handling errors and exceptions effectively very important for ensuring that your application can gracefully manage unexpected issues. XML parsing can encounter various problems such as malformed XML, unexpected element types, or compatibility issues between the XML data and the expected Java data structures. Each of these scenarios can throw exceptions that must be handled appropriately to maintain application stability and provide meaningful feedback to the user.
Java XML parsing libraries, whether using DOM, SAX, or JAXB, provide a range of exceptions that can occur during the parsing process. The most common exceptions include:
- Thrown when a DocumentBuilder cannot be created due to configuration issues.
- A general exception that can occur in SAX parsing, indicating that the parser has encountered an error.
- Thrown when there are problems reading the XML data, such as file not found or access issues.
- Occurs during JAXB operations, typically when the XML does not conform to the expected structure of the Java class.
To show how to handle these exceptions, let’s look at a simple example of using a DOM parser to read an XML file. This example demonstrates how to catch and process potential exceptions effectively:
import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import javax.xml.parsers.ParserConfigurationException; import org.w3c.dom.Document; import org.xml.sax.SAXException; import java.io.File; import java.io.IOException; public class ErrorHandlingExample { public static void main(String[] args) { try { File file = new File("note.xml"); DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse(file); // Process the document as needed System.out.println("XML parsed successfully."); } catch (ParserConfigurationException e) { System.err.println("Error configuring the parser: " + e.getMessage()); } catch (SAXException e) { System.err.println("Error parsing the XML: " + e.getMessage()); } catch (IOException e) { System.err.println("Input/output error: " + e.getMessage()); } catch (Exception e) { System.err.println("An unexpected error occurred: " + e.getMessage()); } } }
In this example, we have wrapped the parsing logic within a try-catch block to capture specific exceptions. Each catch clause provides a descriptive message, allowing developers to understand the nature of the error more clearly. This approach can greatly improve debugging and user experience, as it prevents the application from crashing and provides actionable feedback.
For SAX parsing, error handling is similarly crucial. The SAX parser can throw SAXExceptions during the parsing process, particularly if the XML structure is not as expected. Here’s how you could implement error handling in a SAX parser:
import org.xml.sax.Attributes; import org.xml.sax.SAXException; import org.xml.sax.helpers.DefaultHandler; import javax.xml.parsers.SAXParser; import javax.xml.parsers.SAXParserFactory; public class SAXErrorHandlingExample { public static void main(String[] args) { try { SAXParserFactory factory = SAXParserFactory.newInstance(); SAXParser saxParser = factory.newSAXParser(); DefaultHandler handler = new DefaultHandler() { public void startElement(String uri, String localName, String qName, Attributes attributes) throws SAXException { // Logic for handling start of an element } public void endElement(String uri, String localName, String qName) throws SAXException { // Logic for handling end of an element } public void characters(char ch[], int start, int length) throws SAXException { // Logic for handling character data } public void error(SAXParseException e) throws SAXException { System.err.println("Parsing error: " + e.getMessage()); } }; saxParser.parse("note.xml", handler); System.out.println("SAX parsing completed successfully."); } catch (Exception e) { System.err.println("Error during SAX parsing: " + e.getMessage()); } } }
In this SAX example, we override the error method in the DefaultHandler to catch parsing errors specifically. This allows us to handle and report errors directly related to XML parsing during the event-driven process, enhancing our application’s robustness.
When working with JAXB, proper exception handling is also vital. JAXB can throw JAXBException for a variety of reasons, such as incorrect XML structure or mismatched types. Here’s how to manage exceptions during JAXB unmarshalling:
import javax.xml.bind.JAXBContext; import javax.xml.bind.JAXBException; import javax.xml.bind.Unmarshaller; import java.io.File; public class JAXBErrorHandlingExample { public static void main(String[] args) { try { File file = new File("note.xml"); JAXBContext context = JAXBContext.newInstance(Note.class); Unmarshaller unmarshaller = context.createUnmarshaller(); Note note = (Note) unmarshaller.unmarshal(file); System.out.println("To: " + note.getTo()); System.out.println("From: " + note.getFrom()); System.out.println("Heading: " + note.getHeading()); System.out.println("Body: " + note.getBody()); } catch (JAXBException e) { System.err.println("Error during JAXB unmarshalling: " + e.getMessage()); } catch (Exception e) { System.err.println("An unexpected error occurred: " + e.getMessage()); } } }
In this JAXB example, we catch JAXBException specifically, allowing us to provide feedback if the XML structure doesn’t match the expected Java object. General exceptions are also caught to prevent unexpected application crashes.
Handling errors and exceptions in XML parsing is not only a best practice but a necessity for building resilient Java applications. By implementing robust error handling strategies, you can enhance user experience, simplify debugging, and maintain the integrity of your application’s operations.
It might be helpful to mention the growing trend towards using alternatives to XML, such as JSON, especially in web applications where lighter payloads and simpler data structures are often preferred. While XML has its advantages in certain contexts, highlighting the trade-offs and scenarios where JSON can be more effective would provide a more balanced perspective on data interchange formats. This comparison could also extend to how Java handles both formats and the libraries available for each.