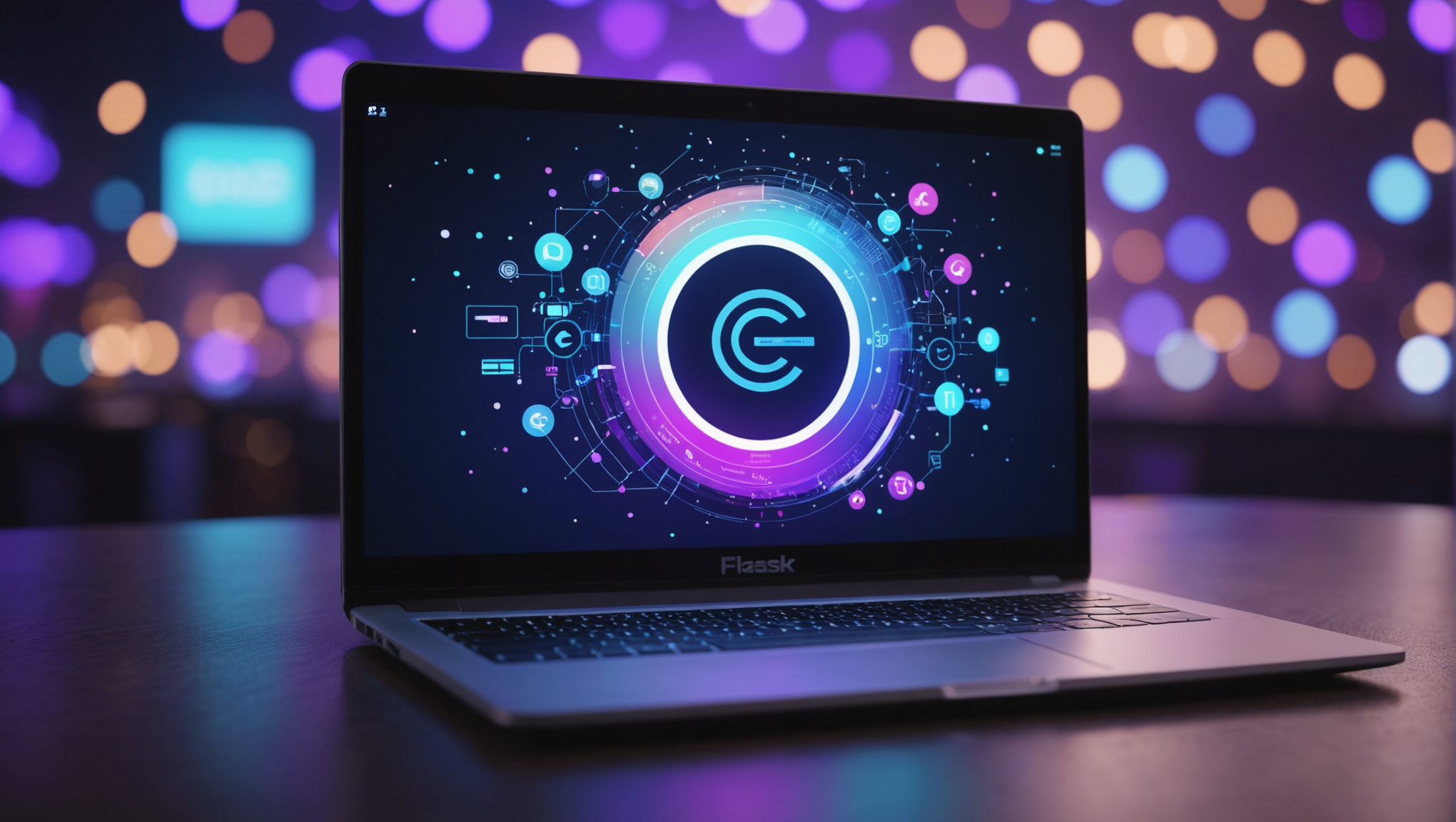
Python and Flask: Building a Web Application
Flask is a microframework for Python that simplifies web application development. Unlike full-fledged frameworks, Flask offers the essentials needed to get a web app off the ground without imposing a rigid structure. It embraces simplicity and flexibility, enabling developers to choose the tools and libraries they prefer.
At its core, Flask is built on the WSGI toolkit and Jinja2 template engine. This allows for clean and manageable code while still providing a powerful set of features. Flask does not come bundled with built-in database layers or form validation tools; instead, it encourages developers to integrate third-party libraries, which can be tailored to specific project needs.
The beauty of Flask lies in its minimalism. You start with a basic app and grow it as necessary. Creating a simple web server with Flask is as easy as:
from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run(debug=True)
In the example above, we import the Flask class and create an instance of it. The @app.route decorator is used to tell Flask what URL should call the associated function. That’s the essence of routing in Flask.
Flask’s modular design means you can easily scale your application. You can build RESTful APIs, integrate authentication mechanisms, or create data-driven applications. The separation of concerns is clean, allowing for easy maintenance and updates.
Furthermore, Flask supports extensions that add functionality as needed, such as Flask-SQLAlchemy for database integration or Flask-WTF for handling forms. This extensibility is one of the reasons why developers are drawn to Flask for both small projects and large applications.
Debugging is simpler thanks to Flask’s built-in debugger, which provides detailed error messages and interactive console access when an error occurs during development. This feature makes it easier to understand and rectify issues quickly.
Flask is not just a tool; it’s a framework that respects your choices as a developer. It allows you to build applications your way, using the strengths of Python while providing a simple yet powerful foundation for web development.
Setting Up Your Development Environment
To begin working with Flask, the first step is to establish a suitable development environment. This ensures that you have all the necessary tools and libraries to write and run Flask applications effectively. Let’s walk through the setup process step-by-step.
First, ensure you have Python installed on your machine. Flask is compatible with Python 3.5 and later, so it’s essential to have an appropriate version. You can download Python from the official Python website. Once installed, verify the installation by running the following command in your terminal:
python --version
This should return the version of Python that is installed. If you see an error, revisit the installation process.
Once Python is installed, the next step is to create a virtual environment. Virtual environments are crucial as they allow you to manage dependencies for different projects separately, preventing conflicts between packages.
You can create a virtual environment using the following command:
python -m venv myflaskenv
Here, “myflaskenv” is the name of the virtual environment, which you can customize. To activate the virtual environment, use the following command depending on your operating system:
For Windows:
myflaskenvScriptsactivate
For macOS and Linux:
source myflaskenv/bin/activate
After activation, your terminal prompt should change, indicating that you are now working within the virtual environment. That is where you’ll install Flask and any other dependencies without affecting your global Python installation.
Now, install Flask using pip:
pip install Flask
To ensure that Flask is installed correctly, you can check the installed packages with the following command:
pip list
This should display Flask in the list of installed packages. If you see it, congratulations! Your environment is now set up for Flask development.
As you dive deeper into Flask, you might find additional libraries useful, such as Flask-SQLAlchemy for database management or Flask-Migrate for handling database migrations. To install these, you can use pip in the same manner:
pip install Flask-SQLAlchemy Flask-Migrate
Remember, whenever you want to work on your Flask application, activate your virtual environment first to ensure you are using the correct dependencies.
With your development environment set up, you’re now ready to start building your first Flask application. The next step will guide you through creating a simple web application to familiarize yourself with Flask’s capabilities.
Creating Your First Flask Application
Now that your development environment is set up, it is time to create your first Flask application. This initial foray will help solidify your understanding of Flask’s routing, request handling, and response generation. Let’s dive right in.
Begin by creating a new Python file for your application. You might name it app.py. Open this file in your preferred code editor and start with the basic structure we discussed earlier:
from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run(debug=True)
This code forms the backbone of your Flask application. Here’s a breakdown of what’s happening:
- The first line imports the Flask class, which is essential for creating a Flask web application.
- The app variable is an instance of the Flask class. This instance is your WSGI application.
- The
@app.route('/')
decorator tells Flask to execute thehello_world()
function whenever a user accesses the root URL of your application. - The
app.run(debug=True)
method starts the Flask application. The debug mode is enabled to help you catch errors during development.
To run your application, navigate to the directory of app.py in your terminal and execute:
python app.py
You should see output indicating that the Flask server is running. By default, it will be accessible at http://127.0.0.1:5000/. Open this URL in your web browser, and you should see the message “Hello, World!” displayed.
Next, let’s make your application a little more interesting by adding additional routes. Modify your app.py file as follows:
@app.route('/about') def about(): return 'This is the About Page' @app.route('/contact') def contact(): return 'This is the Contact Page'
Now you have two new routes: /about and /contact. When you access these URLs, you will see their respective messages. This demonstrates how you can expand your application by simply defining more routes and their corresponding view functions.
Flask also allows you to pass variables through routes. For example, let’s create a dynamic route that greets a user by name:
@app.route('/greet/<name>') def greet(name): return f'Hello, {name}!
In this case, when you navigate to http://127.0.0.1:5000/greet/Alice, it will respond with “Hello, Alice!” This feature showcases Flask’s capability to handle dynamic content and user input seamlessly.
To enhance the application’s structure, ponder organizing your code into blueprints or separate modules as your project grows. Flask’s modular architecture supports this, enabling you to keep your code organized and maintainable.
As you develop your application, remember to save your changes and refresh the browser to see updates. The debug mode automatically reloads the server when it detects changes, which can significantly enhance your development workflow.
With these foundational elements in place, you’re now well on your way to creating more complex and feature-rich applications using Flask. Experiment with routes, respond to different HTTP methods like POST, and integrate templates to create a more interactive user experience. Each addition will deepen your understanding of how Flask operates and prepare you for building robust web applications.
Deploying Your Flask Web Application
Once your Flask application is running smoothly in your development environment, the next logical step is to deploy it so that others can access it over the internet. Deploying a Flask application involves moving it from your local machine to a server and ensuring that it remains accessible and performs optimally. Let’s explore the deployment process in detail.
There are several options available for deploying Flask applications. You can use cloud platforms such as Heroku, AWS, or DigitalOcean. For the sake of simplicity, we’ll focus on deploying to Heroku, which is a popular choice due to its usability and free tier for small applications.
Before deploying, you need to prepare your application. Start by ensuring your application can run in a production environment. This typically involves creating a requirements.txt file and a Procfile.
The requirements.txt file lists all the dependencies your application needs. You can generate this file automatically by running the following command in your terminal:
pip freeze > requirements.txt
This command captures all installed packages in your virtual environment and stores them in requirements.txt. Make sure to include Flask and any other packages you’ve added, such as Flask-SQLAlchemy.
The Procfile tells Heroku how to run your application. Create a new text file named Procfile (without any file extension) in the root directory of your project and add the following line:
web: python app.py
This command specifies that Heroku should run your application using the app.py file.
Now that your application is ready for deployment, you’ll need to install the Heroku Command Line Interface (CLI). This tool allows you to interact with Heroku directly from your terminal. You can download it from the Heroku website.
Once the Heroku CLI is installed, you can log in to your Heroku account by running:
heroku login
This will prompt you to open a web page to authenticate your Heroku account. After logging in, navigate to your project directory and create a new Heroku app using:
heroku create your-app-name
Replace your-app-name with a unique name for your application. This command sets up a new application on Heroku and prepares a Git repository for deployment.
Next, you need to push your application to Heroku. If you haven’t already initialized a Git repository in your project, do so with:
git init
Then, add your files and commit them:
git add . git commit -m "Initial commit"
Now, you can deploy your application to Heroku with the following command:
git push heroku master
This command pushes your committed code to Heroku’s Git repository. The Heroku platform will automatically detect your requirements.txt and install the dependencies listed there.
After the deployment is complete, you can open your application by running:
heroku open
This command launches your default web browser to the URL of your newly deployed application. If everything has been done correctly, you should see your Flask application running live!
For persistent data storage, consider integrating a database like PostgreSQL, which is readily supported by Heroku. You can add it to your application with:
heroku addons:create heroku-postgresql:hobby-dev
After this, you’ll need to configure your application to connect to the PostgreSQL database by retrieving the database URL from Heroku’s configuration variables. You can access these variables using:
heroku config
The deployment process may seem daunting at first, but following these steps will help you get your Flask application up and running in a production environment. As you gain experience, you can explore more advanced deployment practices, such as using Docker containers or setting up continuous integration pipelines. Every deployment experience strengthens your skills and prepares you for more complex projects in the future.
The article provides a solid foundation for getting started with Flask, but it could benefit from discussing best practices for structuring larger applications. As projects grow, organizing code into blueprints and maintaining clear separation of concerns becomes crucial for maintainability and collaboration. Additionally, mentioning the importance of using environment variables for configuration, particularly for sensitive information like API keys and database credentials, would enhance security practices for new developers. Exploring these topics could provide a more comprehensive guide for building robust Flask applications.