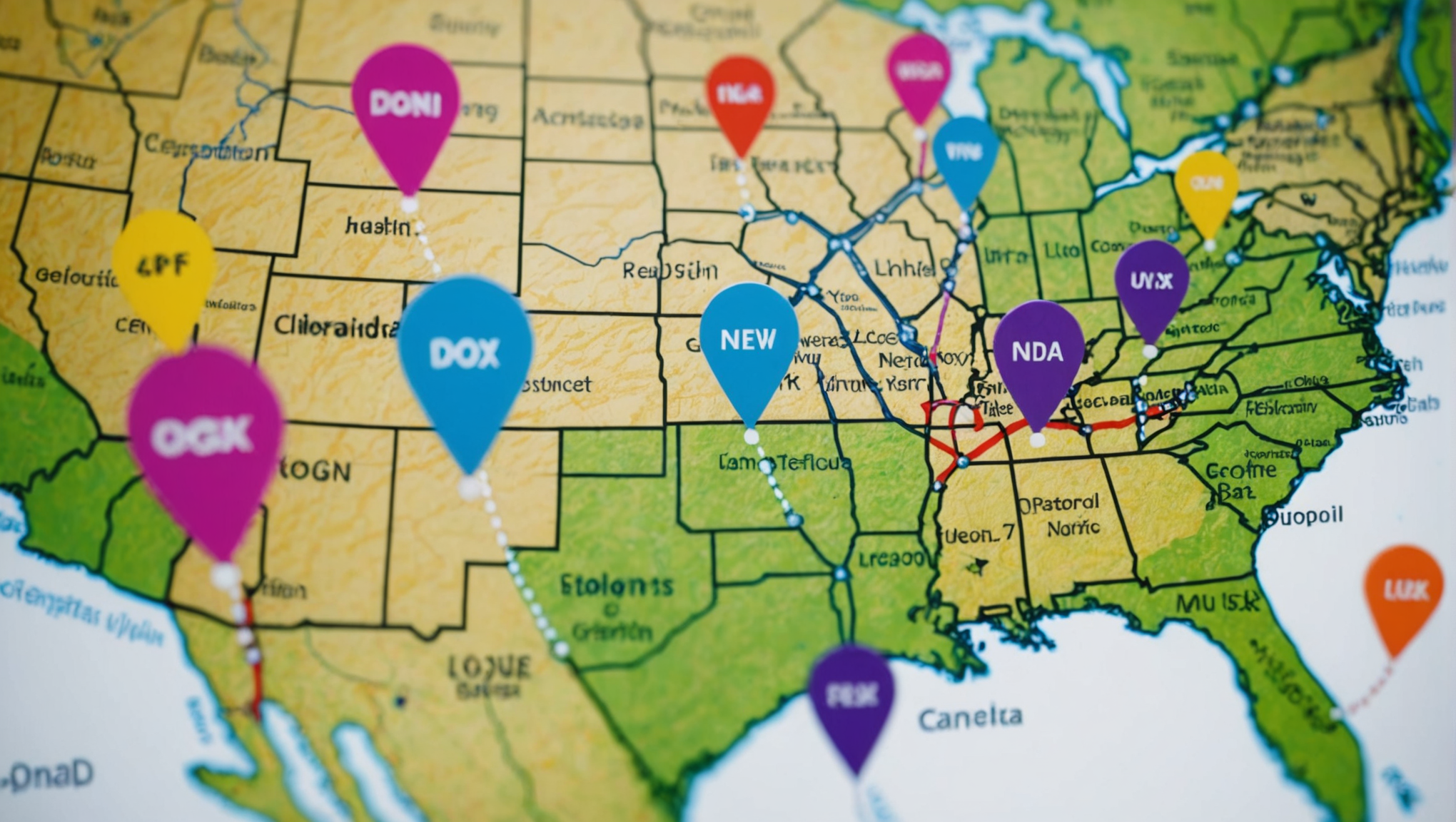
Java and Geographic Information Systems (GIS)
Java has emerged as an important player in the field of Geographic Information Systems (GIS). Its platform-independent nature, robust libraries, and extensive community support make it an attractive choice for developers working on spatial data applications. Unlike some other programming languages, Java’s architecture allows it to run seamlessly across different operating systems, which is vital when dealing with diverse GIS data sources and deployment environments.
At the core of GIS technology lies the need to process and visualize geographical data efficiently. Java excels in this domain thanks to its object-oriented design, which promotes code reusability and organization. GIS applications often require handling complex data structures like maps, layers, and geographical features, all of which can be modeled effectively using Java’s class system.
Moreover, Java’s strong integration capabilities allow it to work with a wide array of data formats and external libraries, making it possible to interface with various GIS databases such as PostGIS and spatial data formats like GeoJSON and Shapefiles. This makes Java not only a powerful language for developing GIS applications but also a versatile tool for data manipulation and analysis.
Here’s an example of how Java can be used to create a simple representation of geographical points:
import java.util.ArrayList; class GeoPoint { private double latitude; private double longitude; public GeoPoint(double latitude, double longitude) { this.latitude = latitude; this.longitude = longitude; } public double getLatitude() { return latitude; } public double getLongitude() { return longitude; } @Override public String toString() { return "GeoPoint{" + "latitude=" + latitude + ", longitude=" + longitude + '}'; } } public class GISExample { public static void main(String[] args) { ArrayList points = new ArrayList(); points.add(new GeoPoint(34.0522, -118.2437)); // Los Angeles points.add(new GeoPoint(40.7128, -74.0060)); // New York for (GeoPoint point : points) { System.out.println(point); } } }
This snippet demonstrates the creation of a simple GeoPoint
class that encapsulates the geographical coordinates of a location. The GISExample
class shows how to instantiate GeoPoint
objects and print their values, which illustrates the foundational aspects of modeling geographical data in Java.
Java’s scalability is another pivotal factor; GIS applications can start small and grow significantly, accommodating larger datasets and more complex analyses without a complete overhaul of the underlying codebase. This flexibility is essential for applications that evolve with user needs and technological advancements.
Java’s role in GIS is not just about providing a means to manipulate geographical data; it transcends that to encompass a fully-fledged ecosystem where developers can build, integrate, and expand GIS applications with relative ease, using the language’s strengths to address real-world geographic challenges.
Key Java Libraries for GIS Development
When diving into the landscape of GIS development in Java, several key libraries stand out, each offering unique functionalities that cater to the diverse needs of GIS applications. These libraries not only simplify the development process but also provide robust tools for spatial data manipulation, rendering, and analysis.
GeoTools is one of the most prominent libraries in the Java GIS ecosystem. It’s an open-source library designed for geospatial data. GeoTools provides a rich set of features, including support for various data formats, rendering, and spatial analysis capabilities. Its modular architecture allows developers to include only the components they need, keeping applications lightweight while offering comprehensive GIS functionality.
For instance, to read a Shapefile using GeoTools, you might employ a snippet like the following:
import org.geotools.data.DataStore; import org.geotools.data.FileDataStoreFactory; import org.geotools.data.shapefile.ShapefileDataStoreFactory; import java.io.File; public class ShapefileReader { public static void main(String[] args) throws Exception { File file = new File("path/to/your/shapefile.shp"); FileDataStoreFactory factory = new ShapefileDataStoreFactory(); DataStore dataStore = factory.createDataStore(file.toURI().toURL()); // Process features from the Shapefile String typeName = dataStore.getTypeNames()[0]; FeatureCollection features = dataStore.getFeatureSource(typeName).getFeatures(); features.forEach(feature -> { System.out.println(feature); }); } }
Another notable library is JTS (Java Topology Suite), which provides a set of classes for geometry and spatial operations. JTS is particularly useful for performing geometric operations such as intersection, union, and buffer calculations. Its powerful capabilities enable developers to handle complex geometries efficiently. Here’s an example of creating geometries and performing a union operation:
import org.locationtech.jts.geom.GeometryFactory; import org.locationtech.jts.geom.Point; import org.locationtech.jts.geom.Polygon; import org.locationtech.jts.geom.Geometry; public class GeometryOperations { public static void main(String[] args) { GeometryFactory geometryFactory = new GeometryFactory(); // Create a Point Point point = geometryFactory.createPoint(new Coordinate(1.0, 1.0)); // Create a Polygon Polygon polygon = geometryFactory.createPolygon(new Coordinate[] { new Coordinate(0.0, 0.0), new Coordinate(2.0, 0.0), new Coordinate(2.0, 2.0), new Coordinate(0.0, 2.0), new Coordinate(0.0, 0.0) // Closing the polygon }); // Perform union operation Geometry result = point.union(polygon); System.out.println(result); } }
Additionally, OpenJUMP is a Java-based GIS application that serves as both a desktop GIS and a plugin architecture for developers. OpenJUMP is built on top of JTS and provides many tools for spatial analysis and data visualization. It is highly extensible, allowing developers to create plugins that can enhance its functionality further.
Last but not least, integration with web mapping frameworks such as GeoServer and Leaflet can be achieved using Java. These tools enable developers to serve spatial data over the web, making it accessible for various client applications. For instance, integrating GeoServer can facilitate the creation of web services that deliver maps and spatial data to clients, using Java’s capabilities for backend processing.
The array of libraries available for Java GIS development equips developers with the necessary tools to handle an extensive range of geospatial tasks. From data storage and manipulation to advanced spatial analysis, these libraries not only enhance productivity but also allow for the creation of sophisticated GIS applications that can meet the evolving demands of users and stakeholders alike.
Building GIS Applications with Java
Building GIS applications with Java is a multifaceted endeavor that requires a thorough understanding of both the language and the spatial concepts that underpin the domain. At its core, a GIS application is a complex system that integrates various components, such as data input, storage, manipulation, analysis, and visualization. Each of these components can be addressed effectively using Java’s robust features and libraries.
One of the first steps in building a GIS application is to define the data model. This involves selecting the appropriate data structures that will represent geographical entities. In Java, this can be achieved using classes to encapsulate various geographical features, such as points, lines, and polygons. Here’s an example of a more extensive model that includes different geometric shapes:
import java.util.ArrayList; abstract class GeoShape { abstract double area(); } class GeoPoint extends GeoShape { private double latitude; private double longitude; public GeoPoint(double latitude, double longitude) { this.latitude = latitude; this.longitude = longitude; } @Override double area() { return 0; // A point has no area } @Override public String toString() { return "GeoPoint{" + "latitude=" + latitude + ", longitude=" + longitude + '}'; } } class GeoPolygon extends GeoShape { private ArrayList vertices; public GeoPolygon(ArrayList vertices) { this.vertices = vertices; } @Override double area() { // Implementation for calculating the area of a polygon // This would involve advanced geometric calculations return 0; // Placeholder } @Override public String toString() { return "GeoPolygon{" + "vertices=" + vertices + '}'; } }
Once the data model is established, the next step is to implement data storage and management. That is where Java’s integration capabilities shine, particularly with relational databases that support spatial data, such as PostGIS. Using JDBC (Java Database Connectivity), developers can interact with databases to store and retrieve geographic data. Here’s an illustrative example of how to connect to a PostGIS database:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; public class DatabaseConnection { public static void main(String[] args) { String url = "jdbc:postgresql://localhost:5432/your_database"; String user = "your_username"; String password = "your_password"; try (Connection connection = DriverManager.getConnection(url, user, password); Statement stmt = connection.createStatement()) { String query = "SELECT * FROM spatial_table"; ResultSet rs = stmt.executeQuery(query); while (rs.next()) { System.out.println("Found spatial data: " + rs.getString("geom")); } } catch (Exception e) { e.printStackTrace(); } } }
As the application grows, developers often need to implement spatial analysis capabilities. Using libraries like JTS (Java Topology Suite) can significantly enhance these functionalities. JTS can be used to perform various geometric operations such as intersections, unions, and buffer zones. Here’s a concise example demonstrating how to calculate a buffer around a point:
import org.locationtech.jts.geom.GeometryFactory; import org.locationtech.jts.geom.Point; import org.locationtech.jts.geom.Geometry; public class BufferExample { public static void main(String[] args) { GeometryFactory geometryFactory = new GeometryFactory(); Point point = geometryFactory.createPoint(new Coordinate(1.0, 1.0)); // Create a buffer of 1.0 around the point Geometry buffered = point.buffer(1.0); System.out.println("Buffered area: " + buffered); } }
To visualize the geographical data, developers can integrate Java with various mapping libraries or frameworks. Libraries like JavaFX or Swing can be used to create graphical user interfaces, while frameworks like GeoServer help serve maps and spatial data to web clients effectively. This combination allows for the development of rich desktop and web-based GIS applications.
Furthermore, as GIS applications often involve a significant amount of data, efficiency is paramount. Java’s concurrency features enable developers to perform data processing and rendering tasks in parallel, thus enhancing the responsiveness of applications. For example, implementing a multi-threaded approach for loading large datasets can significantly reduce wait times for users:
import java.util.concurrent.Executors; import java.util.concurrent.ExecutorService; public class DataLoader { public static void main(String[] args) { ExecutorService executor = Executors.newFixedThreadPool(4); for (int i = 0; i { // Simulate data loading System.out.println("Loading dataset " + index); }); } executor.shutdown(); } }
By combining these various aspects—data modeling, storage, analysis, and visualization—Java developers can create powerful GIS applications that not only meet current user needs but also adapt to future challenges. The flexibility and scalability of Java, coupled with its abundance of libraries, make it a formidable choice for anyone looking to harness the power of geographic information systems.
Data Formats and Standards in GIS
Within the scope of Geographic Information Systems (GIS), the proper handling of data formats and adherence to standards is paramount. GIS data can come in various formats, each with its own specifications for structure and storage, making it essential for developers to understand how to manage these formats effectively within their Java applications. Java excels in this area due to its capability to interface with numerous libraries that facilitate the parsing, reading, and writing of different GIS data formats.
Common data formats in GIS include Shapefiles, GeoJSON, KML (Keyhole Markup Language), and GML (Geography Markup Language). Each of these formats has unique characteristics and serves different use cases, from simple data exchange to complex geographical modeling. For instance, Shapefiles are widely used for representing vector data and can store points, lines, and polygons, while GeoJSON leverages a more modern, JSON-based structure that is easily readable and interoperable with web applications.
Let’s delve into how Java handles these formats, starting with Shapefiles. Using the GeoTools library, developers can easily read and manipulate Shapefiles, as illustrated in the following example:
import org.geotools.data.DataStore; import org.geotools.data.FileDataStoreFactory; import org.geotools.data.shapefile.ShapefileDataStoreFactory; import java.io.File; public class ShapefileExample { public static void main(String[] args) throws Exception { File shapefile = new File("path/to/your/shapefile.shp"); FileDataStoreFactory factory = new ShapefileDataStoreFactory(); DataStore dataStore = factory.createDataStore(shapefile.toURI().toURL()); // Access features in the Shapefile String typeName = dataStore.getTypeNames()[0]; FeatureCollection features = dataStore.getFeatureSource(typeName).getFeatures(); features.forEach(feature -> { System.out.println("Feature: " + feature.getProperty("name").getValue()); }); } }
GeoJSON, on the other hand, is a popular format for web applications due to its lightweight and text-based nature. Fortunately, Java provides libraries such as Jackson for parsing JSON, allowing developers to easily convert GeoJSON to Java objects. Below is a brief example of how to read a GeoJSON file:
import com.fasterxml.jackson.databind.ObjectMapper; import java.io.File; import java.util.Map; public class GeoJSONReader { public static void main(String[] args) throws Exception { ObjectMapper mapper = new ObjectMapper(); Map geoJsonMap = mapper.readValue(new File("path/to/your/file.geojson"), Map.class); System.out.println("GeoJSON Data: " + geoJsonMap); } }
Another format worth mentioning is KML, which is often used for representing geographic data in applications like Google Earth. The Java library for KML supports reading and writing KML files, making it a seamless choice for developers working with this format. Below is an example of how to parse a KML file:
import org.kxml2.kdom.Element; import org.kxml2.kdom.Document; import org.kxml2.kdom.Node; import org.kxml2.kparser.KXmlParser; import java.io.FileInputStream; public class KMLReader { public static void main(String[] args) throws Exception { KXmlParser parser = new KXmlParser(); parser.setInput(new FileInputStream("path/to/your/file.kml"), null); Element documentElement = (Element) parser.read(); for (Node node : documentElement.getChildren()) { if (node instanceof Element) { System.out.println("KML Element: " + ((Element) node).getName()); } } } }
Standards such as OGC (Open Geospatial Consortium) specifications further guide the interoperability of these formats, ensuring that GIS systems can work together seamlessly. Java developers can implement OGC standards through libraries like GeoTools, which includes support for the Web Feature Service (WFS) and Web Map Service (WMS), allowing for the retrieval and rendering of spatial data over the web.
Handling these various data formats requires not only understanding the syntax and structure of each format but also efficiently managing the data within Java applications. The ability to serialize and deserialize these formats effectively can significantly enhance the performance and usability of GIS applications. Therefore, the choice of data format and adherence to established standards is not merely a technical concern but a foundational aspect of developing robust and interoperable GIS solutions in Java.
Integrating Java with Mapping Services
Integrating Java with mapping services elevates the capabilities of GIS applications, allowing them to leverage real-time data and advanced visualization techniques. Mapping services facilitate the display of geographical information over web-based platforms, enabling users to interact with spatial data dynamically. This integration is typically achieved through RESTful APIs provided by various mapping services such as Google Maps, OpenStreetMap, or Mapbox. Java’s robust networking libraries and JSON parsing capabilities make it a strong candidate for these integrations.
To begin with, you can use Java’s built-in libraries like HttpURLConnection
or third-party libraries such as Apache HttpClient
to communicate with these mapping services. The following example illustrates how to make a GET request to the Google Maps API to retrieve geographical data:
import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class GoogleMapsAPIExample { public static void main(String[] args) { String apiKey = "YOUR_API_KEY"; String location = "34.0522,-118.2437"; // Los Angeles coordinates String urlString = "https://maps.googleapis.com/maps/api/geocode/json?latlng=" + location + "&key=" + apiKey; try { URL url = new URL(urlString); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setRequestMethod("GET"); BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream())); String inputLine; StringBuilder response = new StringBuilder(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); System.out.println("Response: " + response.toString()); } catch (Exception e) { e.printStackTrace(); } } }
This code snippet constructs a URL with the required parameters for the Google Maps Geocoding API, makes the GET request, and reads the response. The JSON response can then be parsed to extract relevant information, such as addresses or geographic features.
For parsing JSON in Java, libraries like Jackson
or Gson
are beneficial. The following example demonstrates how to parse a response from the Google Maps API using Jackson
:
import com.fasterxml.jackson.databind.JsonNode; import com.fasterxml.jackson.databind.ObjectMapper; public class ParseGoogleMapsResponse { public static void main(String[] args) { String jsonResponse = "{"results": [{"formatted_address": "Los Angeles, CA, USA"}], "status": "OK"}"; // Mock response try { ObjectMapper mapper = new ObjectMapper(); JsonNode rootNode = mapper.readTree(jsonResponse); String address = rootNode.path("results").get(0).path("formatted_address").asText(); System.out.println("Address: " + address); } catch (Exception e) { e.printStackTrace(); } } }
After obtaining and parsing data from mapping services, the next step involves visualizing this data. JavaFX or Swing can be employed to create user interfaces that render maps and overlay the retrieved data points. For instance, JavaFX can utilize WebView to embed web-based maps directly into a Java application, allowing for a seamless user experience. The following code illustrates how to use WebView to display a simple map:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.web.WebEngine; import javafx.scene.web.WebView; import javafx.stage.Stage; public class MapViewer extends Application { @Override public void start(Stage primaryStage) { WebView webView = new WebView(); WebEngine webEngine = webView.getEngine(); String mapUrl = "https://www.google.com/maps/@34.0522,-118.2437,12z"; // Los Angeles map webEngine.load(mapUrl); primaryStage.setScene(new Scene(webView, 800, 600)); primaryStage.setTitle("Map Viewer"); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
This simple JavaFX application creates a window that loads a Google Maps view centered on Los Angeles. By integrating real-time data with visual mapping tools, developers can create comprehensive GIS applications that provide users with invaluable geographic insights.
Moreover, handling user interactions on the map, such as clicks or zoom events, can be achieved by adding event listeners within the JavaFX application. This interaction can further extend the capabilities of GIS applications, allowing users to explore data in a more engaging manner.
Integrating Java with mapping services not only enhances the functionality of GIS applications but also transforms them into powerful tools for data visualization and user interaction. The flexibility of Java, coupled with its ability to seamlessly interface with web-based APIs, creates endless possibilities for developers in the GIS domain.
Future Trends in Java and GIS Technology
As we gaze into the future of Java and Geographic Information Systems (GIS), several trends are poised to reshape the landscape of geospatial technology. The evolution of GIS is increasingly intertwined with advancements in Java development, offering innovative functionalities that cater to growing demands in data processing, analysis, and visualization.
One pivotal trend is the rise of cloud-based GIS solutions. As organizations continue to transition to cloud infrastructures, Java developers are finding opportunities to leverage platforms such as AWS, Google Cloud, and Azure. These platforms not only provide scalable storage but also powerful APIs and services for big data processing and machine learning. By integrating Java applications with cloud capabilities, developers can create GIS systems that analyze vast datasets more efficiently, leading to real-time insights and more responsive applications. For instance, using services like AWS Lambda with Java can facilitate serverless GIS applications that scale according to demand.
import com.amazonaws.services.lambda.runtime.Context; import com.amazonaws.services.lambda.runtime.RequestHandler; public class GeoAnalysisFunction implements RequestHandler<Map, String> { public String handleRequest(Map input, Context context) { // Your GIS analysis code here return "Analysis Complete"; } }
Furthermore, the incorporation of artificial intelligence (AI) and machine learning into GIS systems is gaining traction. Java’s compatibility with popular machine learning libraries, such as DeepLearning4j and Weka, enables developers to implement predictive analytics directly within GIS applications. This integration allows for smarter spatial analysis, such as predicting urban growth patterns or optimizing resource allocation based on historical geospatial data. The ability to process and analyze data through AI will significantly enhance decision-making processes in various sectors, from urban planning to environmental monitoring.
import org.deeplearning4j.nn.multilayer.MultiLayerNetwork; import org.deeplearning4j.datasets.iterator.Iterator; import org.nd4j.linalg.dataset.api.iterator.DataSetIterator; public class GISMachineLearning { public static void main(String[] args) { MultiLayerNetwork model = new MultiLayerNetwork(...); // Model definition here model.init(); DataSetIterator iterator = ...; // Load your GIS data model.fit(iterator); // Train model on GIS data } }
Another trend signaling the future of GIS in Java is the emphasis on open-source technologies. The GIS community has long benefited from open-source projects, and this trend is growing with the rise of initiatives like the Open Geospatial Consortium (OGC). Java developers can contribute to and benefit from a rich ecosystem of open-source libraries and tools that promote collaboration and innovation. This collaborative environment fosters the development of new standards, improving interoperability among GIS applications and facilitating the sharing of geographical data across platforms.
Moreover, the demand for mobile GIS applications is on the rise. With the increasing ubiquity of mobile devices, the need for GIS solutions that operate effectively on smartphones and tablets is paramount. Java, particularly through its Android SDK, provides a robust framework for developing mobile applications that can leverage GIS capabilities. These applications can allow users to interact with geospatial data while on the go, enabling functionalities like route optimization, real-time navigation, and field data collection.
import android.location.Location; public class MobileGISActivity { public void getUserLocation() { Location location = ...; // Obtain user's current location System.out.println("Latitude: " + location.getLatitude() + ", Longitude: " + location.getLongitude()); } }
Lastly, user experience (UX) design will play an increasingly crucial role in GIS application development. As more stakeholders engage with GIS technology, creating user-centric applications that are intuitive and accessible becomes vital. Java developers will need to focus on employing state-of-the-art UI frameworks like JavaFX for desktop applications or responsive web designs for web-based GIS platforms. Enhancing the user interface and ensuring that it effectively communicates complex geographic information will help democratize GIS technology and make it more approachable for non-technical users.
The future of Java and GIS technology is bright, filled with opportunities for innovation and advancement. By embracing cloud computing, artificial intelligence, open-source collaboration, mobile accessibility, and simple to operate design, Java developers can pave the way for highly functional, scalable, and intelligent GIS applications. This evolution not only caters to current industry needs but anticipates future challenges, ensuring that geospatial technology continues to thrive in an ever-changing landscape.