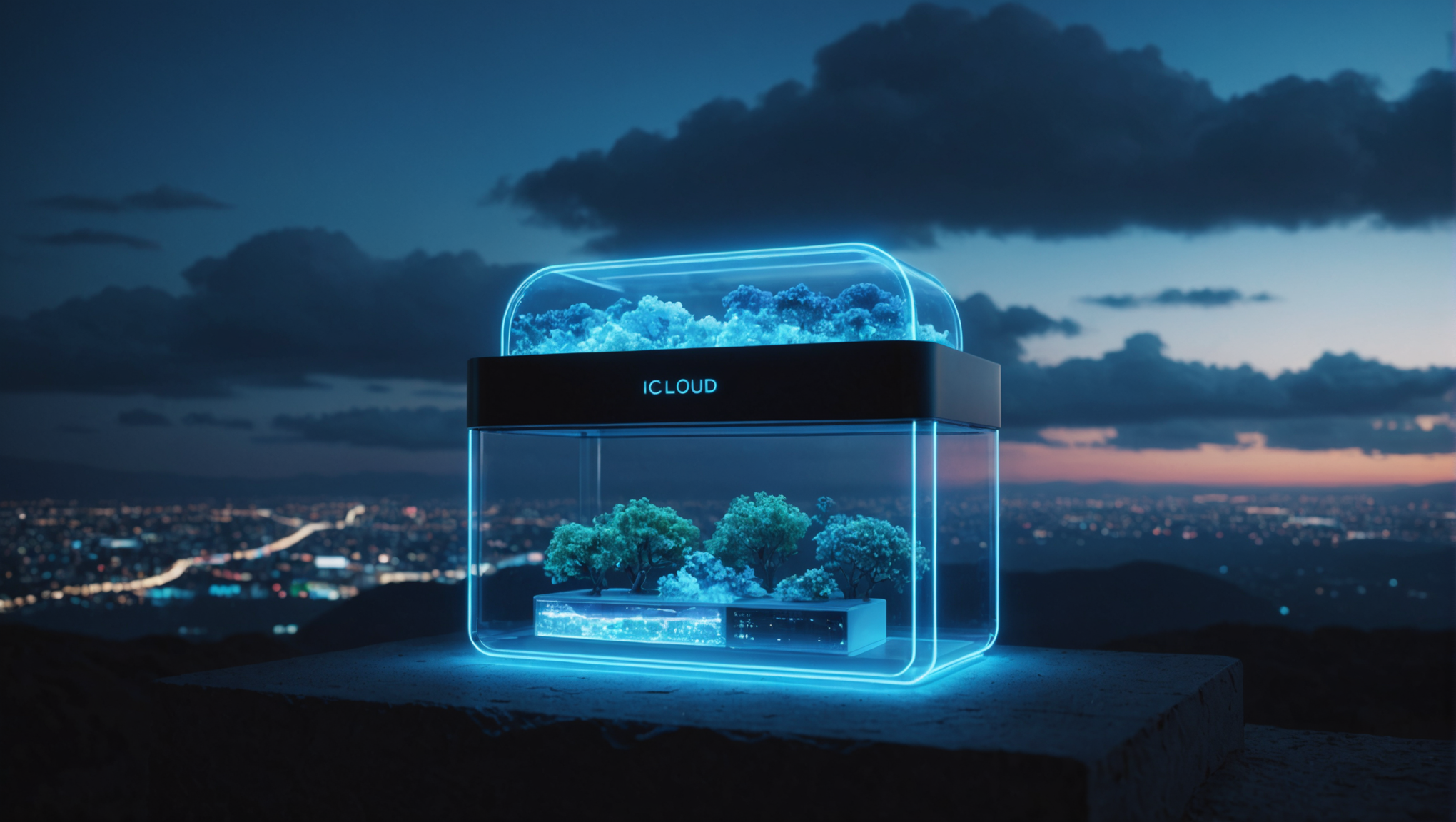
Swift and CloudKit
CloudKit is a powerful framework that facilitates seamless integration between your iOS or macOS application and the cloud. At its core, CloudKit is built on a client-server architecture that allows developers to store, retrieve, and manage data in a secure and efficient manner. Understanding this architecture is important for effectively using CloudKit in your Swift projects.
CloudKit operates on a three-tier structure: the client, the CloudKit server, and the iCloud storage. The client is your application that interacts with the CloudKit framework, sending requests for data and receiving responses. The CloudKit server acts as the intermediary, handling requests from multiple clients and managing the communication between them and the iCloud storage.
When a client makes a request to the CloudKit server, it can perform operations like querying records, saving data, or deleting entries. The server processes these requests and accesses the underlying iCloud storage, which is where all your data is actually stored. This separation of concerns allows developers to write clean and maintainable code while relying on Apple’s infrastructure to manage data integrity, security, and scalability.
CloudKit distinguishes between two primary types of databases: the public database and the private database. The public database is accessible to all users of the app, allowing for shared data storage that can be useful in social applications or collaborative tools. Conversely, the private database is user-specific, enabling the storage of personal data that should not be visible to others. That is particularly important for maintaining user privacy and security.
Another important concept in CloudKit architecture is the record, which is the fundamental data structure used to store information. Records consist of fields, which can hold various data types, including strings, numbers, dates, and more. When you interact with CloudKit in your Swift code, you typically create and manipulate these records to perform your desired operations.
Here’s a simple example of how to define a record in Swift:
let record = CKRecord(recordType: "Note") record["title"] = "My First Note" as CKRecordValue record["content"] = "This is a note saved in CloudKit." as CKRecordValue
In addition to managing records, CloudKit provides features for handling subscriptions, notifications, and user authentication. Subscriptions allow clients to receive real-time updates when changes occur in the database, ensuring that users always have access to the latest information.
By understanding this architecture, you can make informed decisions about how to structure your data, optimize your queries, and ensure your application scales effectively as user demand grows. CloudKit, with its robust architecture, empowers developers to create dynamic and responsive applications that can harness the power of the cloud.
Setting Up CloudKit in Your Swift Project
To set up CloudKit in your Swift project, you need to follow several key steps that will integrate the framework into your development environment and ensure that your app can communicate effectively with iCloud. The process involves creating an iCloud container, configuring your app’s capabilities, and setting up the necessary entitlements.
First, you need to create an iCloud container in the Apple Developer portal. This is essential, as it acts as the storage space for your app’s data in the cloud. Here’s how to do it:
1. Log in to your Apple Developer account. 2. Navigate to the "Certificates, Identifiers & Profiles" section. 3. Select "Identifiers" and click on the "+" button to create a new identifier. 4. Choose "App IDs," and fill in the necessary details for your application. 5. Ensure that the "iCloud" service is enabled and that you create a new iCloud container with a unique identifier, typically in the format of "iCloud.com.yourcompany.yourapp". 6. Save the changes.
Once the container is created, you must configure your Xcode project to use this container. Open your project in Xcode and follow these steps:
1. Select your project in the Project Navigator. 2. Choose your app target. 3. Go to the "Signing & Capabilities" tab. 4. Click the "+" button to add a capability, and select "iCloud." 5. In the iCloud settings, check the "CloudKit" option and select the container you created earlier from the dropdown menu.
Having set up the iCloud container, you need to ensure that your app has the correct entitlements. Xcode automatically manages these entitlements when you configure the iCloud capability, but it’s always good to verify they’re present in your app’s entitlements file.
Next, you’ll want to establish a connection to CloudKit in your code. Typically, this involves initializing the CloudKit database you wish to work with—either the public or private database. Here’s a quick snippet demonstrating how to access the private database:
let privateDatabase = CKContainer.default().privateCloudDatabase
In this example, `CKContainer.default()` retrieves the default container associated with your app, and `privateCloudDatabase` accesses the private database for data storage. If you want to use the public database instead, you can replace `privateCloudDatabase` with `publicCloudDatabase`.
After you have the database reference, you’re ready to implement various operations such as saving records or retrieving data. However, before you can do that, ensure that you handle user authentication appropriately, especially when working with the private database. It’s essential to check the user’s authentication status and prompt them to log in if necessary.
Setting up CloudKit in your Swift project is a simpler process that requires careful configuration of both the iCloud container and your app’s capabilities. With these foundations in place, you’ll be equipped to leverage CloudKit’s powerful features to build a responsive and cloud-integrated application.
Core Features of CloudKit
CloudKit offers a rich set of core features that enhance the integration of cloud services within your Swift application. Understanding these features is vital for developers looking to harness the full potential of CloudKit, ensuring that they can create applications that are not only functional but also scalable and responsive to user needs.
One of the most prominent features of CloudKit is its robust data storage capabilities. The framework allows you to create, read, update, and delete records in a structured way, making it easier for developers to manage data. Each record is associated with a specific record type, which defines the schema and fields that can be stored. This flexibility allows you to model your application’s data in a way that aligns with its requirements.
Another critical feature is the ability to perform queries on your data. CloudKit provides a powerful querying system that enables developers to retrieve records based on specific criteria. You can execute simple queries or more complex ones involving sorting and filtering. Here’s an example of how to query for notes that contain a specific keyword in their title:
let predicate = NSPredicate(format: "title CONTAINS[c] %@", "Note") let query = CKQuery(recordType: "Note", predicate: predicate) privateDatabase.perform(query, inZoneWith: nil) { (results, error) in if let error = error { print("Error querying notes: (error.localizedDescription)") } else if let results = results { for record in results { print("Found note: (record["title"] ?? "No title")") } } }
CloudKit also supports notifications through subscriptions. You can set up subscriptions to receive push notifications when changes occur in the database, allowing your application to respond to real-time updates. This capability is particularly beneficial for collaborative applications where multiple users may be interacting with the same data. Here’s how to create a subscription for record changes:
let subscription = CKQuerySubscription( recordType: "Note", predicate: NSPredicate(value: true), options: .firesOnRecordCreation ) let notificationInfo = CKSubscription.NotificationInfo() notificationInfo.alertBody = "A new note has been added!" notificationInfo.shouldBadge = true subscription.notificationInfo = notificationInfo privateDatabase.save(subscription) { (savedSubscription, error) in if let error = error { print("Error saving subscription: (error.localizedDescription)") } else { print("Subscription saved successfully!") } }
User authentication is another core feature that sets CloudKit apart. It allows you to handle user accounts and their associated data securely. By managing user authentication seamlessly, CloudKit lets you focus on building your application without worrying about the complexities of user management. You can check the user’s authentication status and retrieve their records from the private database efficiently:
CKContainer.default().fetchUserRecordID { (recordID, error) in if let error = error { print("Error fetching user record ID: (error.localizedDescription)") } else if let recordID = recordID { print("User Record ID: (recordID)") } }
Finally, CloudKit’s data migration features allow you to manage schema changes effortlessly. As your application evolves, you may need to update your record types or fields. CloudKit handles these changes gracefully, allowing you to maintain a seamless experience for users while keeping your data structure current.
The core features of CloudKit provide developers with the tools necessary to build powerful, scalable, and real-time applications. Understanding these features and how to implement them effectively in your Swift projects will significantly enhance your app’s capabilities and improve user experience.
Performing CRUD Operations with CloudKit
// Create a new record in the private database let noteRecord = CKRecord(recordType: "Note") noteRecord["title"] = "My CloudKit Note" as CKRecordValue noteRecord["content"] = "This note is stored in CloudKit for easy access." as CKRecordValue privateDatabase.save(noteRecord) { (savedRecord, error) in if let error = error { print("Error saving record: (error.localizedDescription)") } else { print("Record saved successfully: (savedRecord?.recordID.recordName ?? "No Record ID")") } } // Fetching records from the private database let fetchRecordID = CKRecord.ID(recordName: "YOUR_RECORD_ID_HERE") privateDatabase.fetch(withRecordID: fetchRecordID) { (fetchedRecord, error) in if let error = error { print("Error fetching record: (error.localizedDescription)") } else if let fetchedRecord = fetchedRecord { print("Fetched record title: (fetchedRecord["title"] ?? "No title")") } } // Updating an existing record fetchedRecord["content"] = "Updated content for the CloudKit note." as CKRecordValue privateDatabase.save(fetchedRecord) { (updatedRecord, error) in if let error = error { print("Error updating record: (error.localizedDescription)") } else { print("Record updated successfully: (updatedRecord?.recordID.recordName ?? "No Record ID")") } } // Deleting a record privateDatabase.delete(withRecordID: fetchRecordID) { (deletedRecordID, error) in if let error = error { print("Error deleting record: (error.localizedDescription)") } else { print("Record deleted successfully: (deletedRecordID.recordName)") } }
Performing CRUD (Create, Read, Update, Delete) operations with CloudKit is simpler yet powerful. When you create a record, you define what information it will hold and what type it belongs to within your CloudKit database. In the example above, a new note is created and saved to the private database. Once this record is saved, you can handle any potential errors gracefully, ensuring a robust user experience.
Reading records involves fetching them using their unique identifiers. In the aforementioned code, a record is retrieved based on its ID, so that you can access and utilize its fields. That is the foundation for displaying user-specific data in your app.
Updating records follows a similar pattern. After fetching a record, you can modify its fields and save it back to the database. This pattern provides a clean and efficient method for keeping your data up-to-date, while also handling any issues that may arise during the save operation.
Finally, deleting records is just as simple. By providing the record’s ID, you can remove it from the cloud database, allowing for easy data management. This is particularly useful for applications where users may wish to delete their data or for cleaning up outdated information.
Combining these CRUD operations allows developers to build dynamic applications that can adapt to user interactions in real-time. The seamless integration with CloudKit lets you focus on writing efficient code while Apple handles the complexities of cloud storage and synchronization.
As you work with CloudKit in your Swift projects, you’ll find that mastering these CRUD operations will enable you to create applications that not only meet user needs but also leverage the full potential of cloud computing.
Best Practices for CloudKit Integration
When integrating CloudKit into your Swift applications, following best practices can significantly enhance performance, maintainability, and user experience. Here are several key strategies that you should ponder to ensure efficient and effective CloudKit integration.
1. Optimize Data Structure
Design your data structure thoughtfully. Each record type should align with the entities in your application, and you should avoid overly complex hierarchies. Use meaningful field names and ponder the types of queries you will perform. This will help in keeping the data model intuitive and efficient.
2. Use Batch Operations
When performing multiple read or write operations, utilize batch requests to minimize the number of network calls. This leads to reduced latency and improved performance. Here’s an example of how to save multiple records in a single operation:
let recordsToSave: [CKRecord] = [record1, record2, record3] privateDatabase.save(recordsToSave) { (savedRecords, error) in if let error = error { print("Error saving records: (error.localizedDescription)") } else { print("Records saved successfully: (savedRecords?.map { $0.recordID.recordName } ?? [])") } }
3. Implement Caching Strategies
Leverage CloudKit’s caching capabilities to reduce load times and improve user experience. Use local storage to cache frequently accessed data, which minimizes the number of calls to the CloudKit server. For instance, you could cache user preferences or recently accessed records. Here’s a simple example of how to cache data:
let userDefaults = UserDefaults.standard userDefaults.set(recordData, forKey: "cachedRecordData")
4. Handle Rate Limiting
Be aware that CloudKit imposes rate limits on the number of requests your app can make. Implement exponential backoff strategies for retrying failed requests to handle this gracefully. A simple implementation could look like this:
func performCloudKitOperation(retryCount: Int = 0) { privateDatabase.save(noteRecord) { (savedRecord, error) in if let error = error as? CKError { switch error.code { case .limitExceeded: let delay = pow(2.0, Double(retryCount)) DispatchQueue.global().asyncAfter(deadline: .now() + delay) { self.performCloudKitOperation(retryCount: retryCount + 1) } default: print("Error saving record: (error.localizedDescription)") } } else { print("Record saved successfully: (savedRecord?.recordID.recordName ?? "No Record ID")") } } }
5. Regularly Update Your Records
To maintain data freshness, especially in collaborative applications, regularly update your records and utilize subscriptions to listen for changes. This ensures that users are always presented with the latest information. For instance:
let subscription = CKQuerySubscription(recordType: "Note", predicate: NSPredicate(value: true), options: .firesOnRecordUpdate) privateDatabase.save(subscription) { (savedSubscription, error) in if let error = error { print("Error saving subscription: (error.localizedDescription)") } else { print("Subscription saved successfully!") } }
6. Ensure User Privacy
Respect user privacy and security when handling their data. Always inform users when collecting personal information and provide options for data management. Use the private database for user-specific data to ensure that sensitive information is kept secure. Always check for user authentication status before accessing private data.
7. Monitor for Errors and Debugging
Implement comprehensive error handling across all your CloudKit operations. Use logging to capture errors and provide user-friendly messages when issues occur. This not only aids in debugging but also enhances user experience. For example:
privateDatabase.fetch(withRecordID: fetchRecordID) { (fetchedRecord, error) in if let error = error { print("Error fetching record: (error.localizedDescription)") // Handle different error cases appropriately } else { // Process fetched record } }
Incorporating these best practices into your CloudKit integration will not only improve the performance of your application but also enhance the overall user experience. As you develop your Swift applications, think these strategies as guiding principles to help you navigate the complexities of cloud integration.
Handling Errors and Debugging CloudKit Applications
Handling errors effectively in CloudKit especially important for creating robust applications that provide a seamless user experience. When your app interacts with CloudKit, various issues may arise, such as network problems, authentication failures, or data conflicts. Understanding how to manage these errors and debug your CloudKit applications will make a significant difference in their reliability and usability.
CloudKit utilizes the CKError
class to encapsulate the various errors that can occur during operations. Each error has a specific code that indicates the nature of the problem, allowing developers to implement targeted error handling. Here’s a fundamental approach to handling errors when saving a record:
privateDatabase.save(noteRecord) { (savedRecord, error) in if let error = error as? CKError { switch error.code { case .networkUnavailable: print("Network is unavailable. Please check your internet connection.") case .notAuthenticated: print("User is not authenticated. Please log in.") case .limitExceeded: print("Operation limit exceeded. Try again later.") default: print("An error occurred: (error.localizedDescription)") } } else { print("Record saved successfully: (savedRecord?.recordID.recordName ?? "No Record ID")") } }
In this snippet, we check if the error
is of type CKError
and handle it based on its code. This approach allows you to provide meaningful feedback to users, guiding them on how to resolve issues instead of leaving them confused.
Debugging CloudKit applications also requires a good understanding of the asynchronous nature of its APIs. Since CloudKit operations are performed asynchronously, it is vital to ensure proper handling of callbacks. Using logging can provide insights into the operational flow and help identify where things might be going wrong. For instance:
privateDatabase.fetch(withRecordID: fetchRecordID) { (fetchedRecord, error) in if let error = error { print("Error fetching record: (error.localizedDescription)") // Additional logging can be added here for debugging } else if let fetchedRecord = fetchedRecord { print("Fetched record: (fetchedRecord)") } }
Implementing additional logging can help you trace requests and responses, making it easier to identify issues. You can use tools like OSLog for structured logging, which can help in organizing and filtering log messages.
Moreover, while developing your application, ponder enabling CloudKit’s debugging options in your Apple Developer account. These options can provide more detailed error messages that help clarify issues during development.
When dealing with conflicts in records—such as when two users attempt to update the same record simultaneously—CloudKit provides mechanisms to handle versioning. It’s advisable to implement conflict resolution strategies to determine how your application should respond in these situations. For instance:
privateDatabase.fetch(withRecordID: fetchRecordID) { (fetchedRecord, error) in if let error = error as? CKError, error.code == .conflict { print("Conflict detected. Please resolve the conflict before saving.") // Implement your conflict resolution strategy here } }
Handling errors and debugging your CloudKit applications is an ongoing process that requires careful consideration of both the specific errors that may arise and how to inform users appropriately. By adopting a structured approach to error handling, using logging, and planning for conflict resolution, developers can create resilient applications that maintain user trust, even when problems occur.