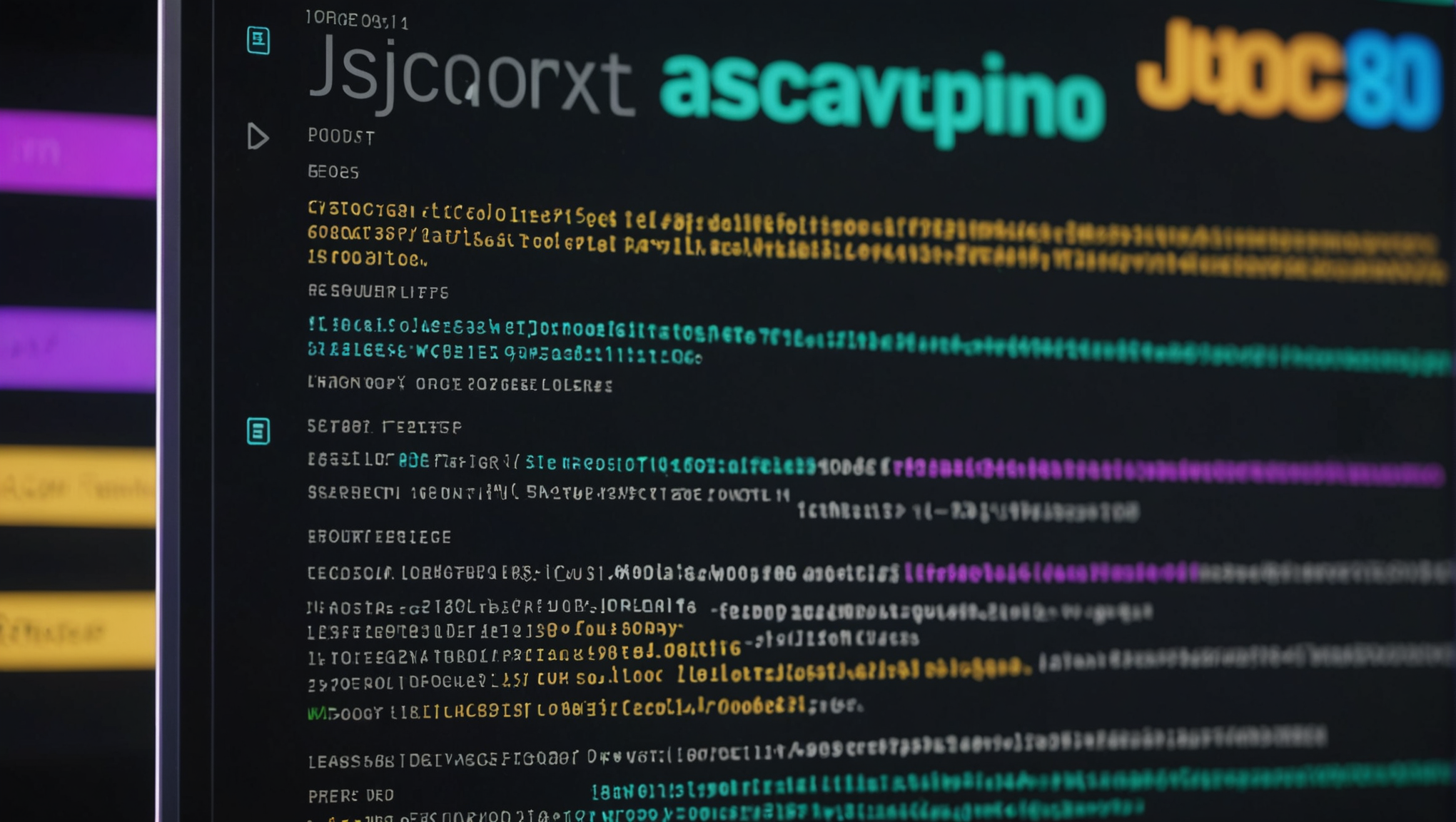
JavaScript and Text Editors: Choosing the Right One
In the sphere of JavaScript development, text editors serve as the primary interface between the developer and the code. Unlike integrated development environments (IDEs) that provide a plethora of built-in tools and functionalities, a text editor offers a more streamlined approach, allowing developers to focus solely on writing and manipulating code. The choice of a text editor can significantly impact a developer’s productivity and the overall quality of the software being created.
The primary function of a text editor is to facilitate the writing and editing of code. It’s where developers spend most of their time crafting algorithms, designing components, and debugging errors. A well-chosen text editor can enhance the coding experience by providing features that aid in code readability, structure, and organization. Text editors can range from simple applications with basic functionalities to powerful tools equipped with plugins and extensions that cater to a developer’s specific needs.
One of the core roles of a text editor in JavaScript development is syntax highlighting. This feature differentiates keywords, operators, and other elements of the language visually, making it easier for developers to read and comprehend code at a glance. For example, a JavaScript function can be visually distinguished from variables and comments, as shown in the following snippet:
function calculateSum(a, b) { return a + b; // Return the sum of a and b }
Another significant aspect is code completion, which helps speed up coding by suggesting possible completions for partially typed words or symbols. This feature reduces the likelihood of typos and allows developers to focus more on logic rather than syntax. For example, when typing a function call, a text editor may suggest the available methods associated with a particular object, thus providing a helpful shortcut to commonly used functions.
Moreover, text editors often include debugging tools that allow developers to step through their code, set breakpoints, and inspect variables. Even without a full-fledged IDE, many modern text editors can integrate with browsers or tools like Node.js to provide a debugging environment that suits JavaScript developers.
Collaboration is another critical function of text editors, especially in team settings. Many editors support version control systems like Git, enabling multiple developers to work on the same codebase efficiently. The ability to manage code versions, branches, and merges especially important for maintaining a clean and organized project history.
Lastly, the importance of customization should not be overlooked. A text editor that allows developers to personalize their environment—whether through themes, key bindings, or extensions—can lead to a more enjoyable and efficient coding experience. Tailoring the workspace to fit individual preferences helps minimize distractions and enhances focus, which is essential in the intricate world of JavaScript development.
Key Features to Look for in a JavaScript Text Editor
When considering a text editor for JavaScript development, there are several key features that can make a significant difference in your coding workflow and overall productivity. These features not only enhance the user experience but also streamline the development process, allowing developers to write cleaner, more efficient code.
1. Syntax Highlighting
As previously mentioned, syntax highlighting is a fundamental feature of any text editor aimed at programming. Effective syntax highlighting enhances code readability by color-coding different elements such as keywords, variables, strings, and comments. This visual distinction helps prevent errors and makes it easier to spot issues at a glance. For example, in the following code snippet, the function, parameters, and comments are visually distinct:
function calculateSum(a, b) { return a + b; // Return the sum of a and b }
2. Code Completion and IntelliSense
Code completion is another invaluable feature. It saves time and reduces the chance of typographical errors by suggesting completions for partially typed code. This predictive capability, often referred to as IntelliSense, can be a game-changer when working with complex libraries or frameworks. For instance, when you begin typing a method associated with an object, the editor can prompt you with a list of potential methods, allowing for faster coding:
const person = { name: 'John', age: 30, greet: function() { console.log('Hello, ' + this.name); } }; // Code completion assists here person.greet(); // Suggested method from 'person' object
3. Integrated Debugging
Debugging tools embedded within the text editor can significantly enhance a developer’s ability to troubleshoot issues. Modern JavaScript text editors often provide the means to set breakpoints, step through code, and inspect variables in real-time. Such functionality allows for a more seamless debugging experience without the need to switch between multiple tools or environments. Here’s a simple illustration of how a debugging session might look:
function divide(a, b) { if (b === 0) { throw new Error('Division by zero'); } return a / b; } // Setting a breakpoint here to inspect values console.log(divide(10, 2));
4. Version Control Integration
With collaborative development becoming increasingly common, support for version control systems like Git especially important. Text editors that seamlessly integrate Git functionalities allow developers to commit changes, create branches, and manage merges directly within the editor. This integration fosters a smoother workflow and helps maintain a clean project history. Here’s a simple Git command example that can be executed in a terminal integrated into the editor:
git commit -m "Fix: Corrected division by zero error"
5. Extensibility and Customization
The ability to extend a text editor’s functionality through plugins or extensions can greatly enhance the coding environment. Many popular editors allow developers to install a variety of plugins that cater to specific needs, such as linting, formatting, or even theming. Being able to customize key bindings, themes, and layouts can lead to a more personalized and efficient coding experience. Ponder the following code snippet that demonstrates the use of ESLint for maintaining code quality:
// Sample ESLint configuration module.exports = { "env": { "browser": true, "es2021": true }, "extends": "eslint:recommended", "parserOptions": { "ecmaVersion": 12 }, "rules": { "no-console": "warn" } };
A text editor is not merely a tool for writing code; it is a vital component of a developer’s toolkit. By understanding the key features to look for, developers can select a text editor that not only meets their immediate needs but also supports their long-term growth and efficiency in JavaScript development.
Popular Text Editors for JavaScript: A Comparative Overview
As we delve deeper into the landscape of JavaScript text editors, it’s essential to examine some of the most popular options available today. Each text editor offers a unique blend of features, user experience, and extensibility, catering to different developers’ needs and preferences. Below, we will explore a few notable text editors that have garnered widespread adoption within the JavaScript community.
Visual Studio Code (VS Code) stands out as one of the most popular choices among JavaScript developers. Its robust ecosystem of extensions allows for an impressive range of functionality, from debugging to Git integration. VS Code offers built-in support for JavaScript, TypeScript, and has an intuitive user interface that promotes productivity. With features like IntelliSense for smart completions based on variable types and function definitions, developers can write code more efficiently. The integrated terminal adds another layer of convenience, allowing developers to run scripts and commands without leaving the editor.
const greeting = 'Hello, world!'; console.log(greeting); // Output: Hello, world!
Sublime Text is another favorite, known for its speed and efficiency. Its minimalist design appeals to many developers, while its extensive package ecosystem allows for significant customization. Sublime Text supports multiple cursors and powerful search and replace functionalities, making it particularly useful for editing large files or performing bulk changes. However, unlike VS Code, Sublime Text’s free version periodically prompts users to purchase a license, which some developers may find off-putting.
const numbers = [1, 2, 3, 4, 5]; const doubled = numbers.map(num => num * 2); // Doubling each number console.log(doubled); // Output: [2, 4, 6, 8, 10]
Atom, developed by GitHub, embraces a hackable nature that invites developers to modify its source code. This editor is particularly favored by those who enjoy customizing their development environment. Atom provides a built-in package manager, allowing users to easily install new packages or themes to imropve their workflow. Additionally, its collaborative editing feature, Teletype, enables real-time collaboration, making it easier for teams to work together on projects.
const fruits = ['apple', 'banana', 'cherry']; fruits.forEach(fruit => console.log(fruit)); // Output: apple, banana, cherry
Brackets is an editor designed specifically for web development. Its live preview feature is a standout, allowing developers to see updates to their HTML and CSS in real-time as they code. While Brackets is primarily focused on front-end technologies, its support for JavaScript is commendable. The inline editors enable quick edits without losing context, enhancing productivity for front-end developers working heavily with JavaScript.
function welcomeUser(user) { return `Welcome, ${user}!`; // Template literals for cleaner syntax } console.log(welcomeUser('Alice')); // Output: Welcome, Alice!
WebStorm, developed by JetBrains, is a commercial IDE that offers extensive support for JavaScript and related technologies. While it comes with a higher price tag, its powerful features justify the cost for many professional developers. WebStorm includes integrated debugging, testing, and version control support, all within a cohesive environment. Its intelligent coding assistance, including smart refactorings and advanced navigation, allows for a more streamlined coding experience.
const user = { name: 'Bob', age: 25 }; const { name, age } = user; // Destructuring assignment for cleaner code console.log(`Name: ${name}, Age: ${age}`); // Output: Name: Bob, Age: 25
The selection of a text editor can greatly influence a developer’s efficiency and enjoyment while coding in JavaScript. Each of these editors presents unique advantages and features, and the best choice ultimately depends on individual preferences, workflow requirements, and project needs. Whether you lean towards the extensive capabilities of Visual Studio Code or the simplicity of Sublime Text, choosing the right editor can elevate your JavaScript development experience.
Customization and Extensions: Enhancing Your Text Editor Experience
In the ever-evolving landscape of JavaScript development, customization and extensions play an important role in tailoring the text editor to meet specific needs and enhance productivity. The beauty of modern text editors lies in their flexibility; they can be transformed from basic code-writing tools into powerful environments that cater to individual workflows and preferences. This adaptability not only improves user experience but also allows developers to harness additional features that are critical in today’s fast-paced coding world.
One of the primary ways to imropve a text editor is through its extension system. Most popular editors provide a marketplace or repository where developers can browse and install a variety of plugins that add new functionalities. For instance, tools like ESLint can be integrated to enforce coding standards and detect potential errors in real-time, which helps maintain code quality. Here’s an example of an ESLint configuration:
// Sample ESLint configuration module.exports = { "env": { "browser": true, "es2021": true }, "extends": "eslint:recommended", "parserOptions": { "ecmaVersion": 12 }, "rules": { "no-console": "warn" } };
Additionally, many editors support snippets—predefined templates of code that can be quickly inserted into the codebase. These snippets can drastically reduce the time spent on common tasks such as defining functions or creating boilerplate code. For example, a snippet for creating a JavaScript function might look like this:
// Snippet for a JavaScript function function myFunction(param1, param2) { // Your code here return param1 + param2; }
Customization goes beyond just installing plugins. Developers can often define their own keybindings to optimize their workflow according to their habits. For instance, remapping common actions to shortcuts that feel natural can create a more fluid coding experience. Here’s an example of a modified keybinding configuration that could be set in an editor:
// Example keybindings configuration { "key": "ctrl+alt+f", "command": "editor.action.formatDocument" }
Theming is another aspect that contributes to a comfortable coding experience. Most text editors allow users to choose from a variety of themes or even create their own. A visually appealing and easy-to-read color scheme can reduce eye strain and help maintain focus during long coding sessions. Here’s a simple example that illustrates how one might define a custom theme for a more soothing editing environment:
// Custom theme configuration example { "name": "My Cool Theme", "type": "dark", "colors": { "editor.background": "#2E2E2E", "editor.foreground": "#FFFFFF", "editorCursor.foreground": "#FFCC00" } }
Beyond individual productivity enhancements, extensions can also facilitate collaboration among team members. Tools like Live Share allow multiple developers to work in the same coding session, providing real-time collaboration capabilities that can be invaluable for brainstorming and debugging as a group. Imagine a scenario where developers can navigate through code together, share insights, and resolve issues on the fly.
The true power of a text editor for JavaScript development lies in its ability to be customized and extended. By using plugins, personal configurations, and collaborative tools, developers can create a coding environment that not only suits their individual preferences but also enhances their overall productivity. Investing time into personalizing your text editor can yield significant returns in efficiency and satisfaction, making the coding experience not just productive, but also highly enjoyable.
Setting Up Your Text Editor for Optimal JavaScript Coding
Setting up your text editor for optimal JavaScript coding is a vital step that can essentially transform your development experience. The right configuration ensures that you can harness the full potential of your text editor, increasing efficiency and reducing friction in your workflow. Here’s how to optimize your editor for JavaScript development.
First and foremost, ensure that your editor is properly configured for JavaScript syntax. Many contemporary text editors like Visual Studio Code, Sublime Text, and Atom provide out-of-the-box support for JavaScript, but it is often beneficial to customize the settings further. Look for options to enable JavaScript-specific features such as linting and syntax highlighting. For instance, in VS Code, you can install the ESLint extension to automatically check your code for potential errors and enforce coding standards. Here’s a sample configuration for ESLint that you might include in your project:
// Sample ESLint configuration module.exports = { "env": { "browser": true, "es2021": true }, "extends": "eslint:recommended", "parserOptions": { "ecmaVersion": 12 }, "rules": { "no-console": "warn" } };
Next, consider configuring your editor’s code formatting options. Clean, consistent code is essential in collaborative environments. Many editors support extensions for code formatting, such as Prettier, which can automatically format your JavaScript code according to specified guidelines every time you save. This not only keeps your code tidy but also minimizes merge conflicts in team settings. A typical Prettier configuration in a project would look like this:
// Sample Prettier configuration module.exports = { semi: true, singleQuote: true, trailingComma: 'all', };
Moreover, harness the power of snippets to streamline your coding process. Snippets are predefined pieces of code that can be inserted quickly, which will allow you to avoid repetitive typing. You can create your own snippets tailored to your most common patterns. For example, if you frequently create a function to handle events, a snippet could look like this:
// Snippet for an event handler function function handleEvent(event) { event.preventDefault(); // Your code here }
Incorporating keyboard shortcuts tailored to your workflow can also drastically enhance your productivity. Most text editors allow you to remap shortcuts based on your preferences. For example, you might create a shortcut that formats your document or opens a terminal window. Here’s how you might set a custom keybinding for formatting in VS Code:
// Example keybindings configuration { "key": "ctrl+shift+f", "command": "editor.action.formatDocument" }
Finally, don’t overlook the importance of themes and visual preferences. A well-chosen theme can reduce eye strain and improve focus during long coding sessions. Most editors let you choose from a library of themes or create your own. For example, a simple dark theme configuration might look like this:
// Dark theme configuration example { "name": "My Dark Theme", "type": "dark", "colors": { "editor.background": "#1E1E1E", "editor.foreground": "#F8F8F2", "editorCursor.foreground": "#FF79C6" } };
By integrating these configurations and enhancements into your text editor, you create an environment tailored to your specific JavaScript development needs. This thoughtful setup can lead to a more enjoyable and productive coding experience, so that you can focus on what truly matters: writing great code.