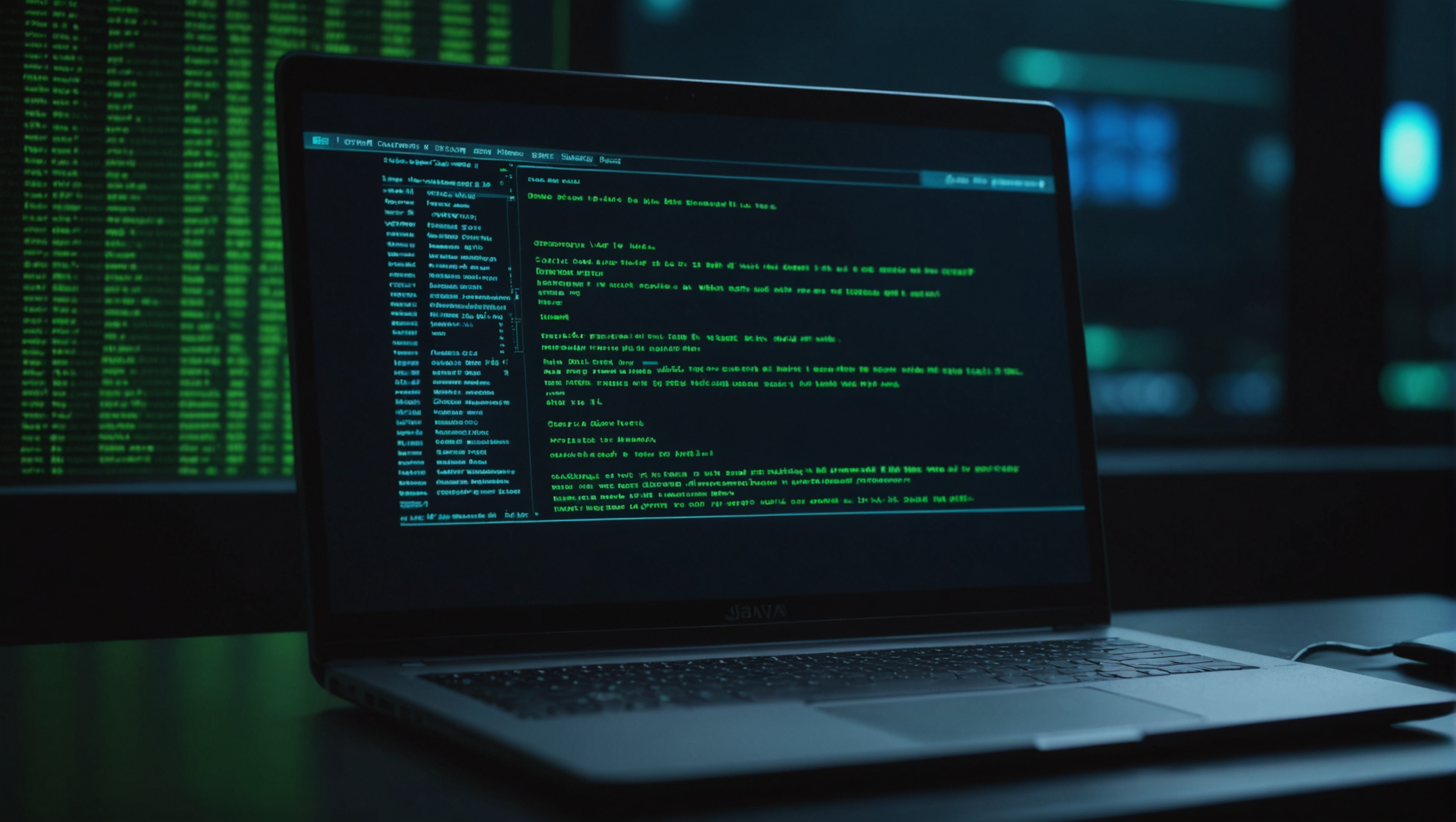
Java and Code Quality: Static Analysis Tools
Static analysis tools play a pivotal role in enhancing code quality in Java development. These tools scrutinize the source code without executing it, enabling developers to catch potential issues early in the development cycle. By identifying bugs, security vulnerabilities, and adherence to coding standards, static analysis tools contribute significantly to creating robust software.
One of the primary benefits of employing static analysis is the ability to detect issues before they escalate into more serious problems. Early detection reduces the cost and effort associated with fixing bugs later in the development process. For instance, finding a null pointer exception during the coding phase is far less costly than discovering it during production.
Static analysis also promotes better adherence to coding conventions and best practices. Tools can enforce a consistent coding style, which enhances readability and maintainability. This is particularly beneficial in teams with multiple developers, ensuring that everyone adheres to the same standards. Consistency in code makes it easier for developers to collaborate and understand each other’s work.
Another significant advantage is the enhancement of security. Static analysis tools can identify security vulnerabilities by analyzing the source code for patterns that may lead to exploits. This proactive approach can significantly reduce the risk of breaches, as it allows teams to address security flaws before the application is deployed.
Moreover, many static analysis tools offer integration with integrated development environments (IDEs), allowing developers to receive immediate feedback as they write code. This instantaneous feedback loop encourages developers to adhere to best practices from the outset rather than accumulating technical debt over time.
Consider the following Java code snippet, which demonstrates how static analysis can identify a potential null pointer exception:
public class Example { public void processData(String data) { // Potential null pointer exception if data is null System.out.println(data.length()); } }
A static analysis tool would flag the line where data.length()
is called, warning the developer that data
might be null at runtime.
Furthermore, static analysis not only helps in catching simpler coding errors but also encourages developers to think critically about the algorithms and data structures they utilize. By evaluating code complexity and identifying potential performance bottlenecks, developers can write more efficient and optimized code.
The benefits of using static analysis tools in Java are clear. From early bug detection and enforcement of coding standards to enhancing security and performance, these tools serve as invaluable allies in the quest for high-quality code. Embracing static analysis as a regular part of the development workflow can lead to significant improvements in both the quality and maintainability of Java applications.
Popular Static Analysis Tools for Java
When it comes to static analysis tools for Java, several prominent options have emerged, each with its unique features and capabilities. These tools empower developers to enforce coding standards, detect bugs, and enhance security without the need for code execution. Below are some widely-used static analysis tools that Java developers should consider incorporating into their workflow.
Checkstyle is a popular static analysis tool that focuses on enforcing coding conventions. It allows teams to define their own coding standards and check for adherence to these rules in the source code. With Checkstyle, developers can easily catch formatting issues, naming conventions, and other style violations. A typical configuration might look like this:
PMD is another powerful tool that scans Java source code for potential bugs, dead code, and inefficient practices. PMD provides a rich set of predefined rules and allows for customization, catering to specific coding guidelines or project needs. An example of how PMD can be configured to look for unused variables is:
My custom ruleset to detect unused variables.
FindBugs, now known as SpotBugs, focuses on identifying potential bugs in Java programs. It analyzes bytecode rather than source code, enabling it to detect a broader range of issues, such as concurrency problems and null pointer dereferences. The integration of SpotBugs into the build process can be done easily using Maven with the following configuration:
com.github.spotbugs spotbugs-maven-plugin 4.2.3 check
SonarQube is an integrated platform that performs continuous inspection of code quality, providing detailed reports on bugs, vulnerabilities, and code smells. SonarQube can be particularly useful for managing technical debt over time and ensuring that projects maintain a high standard of code quality. Developers can easily integrate SonarQube with CI/CD pipelines to get real-time feedback on their code changes.
Checkmarx is a static application security testing (SAST) tool designed to identify security vulnerabilities in code. By performing a comprehensive analysis of the source code, Checkmarx helps teams uncover potential security issues early in the development lifecycle. An example of a commonly detected vulnerability is the improper validation of user input, which could lead to SQL injection attacks.
Each of these static analysis tools offers unique strengths, and their integration into the Java development workflow can lead to significant improvements in code quality, maintainability, and security. By using these tools, developers can create cleaner code and reduce the likelihood of defects, ultimately contributing to the success of their projects.
Integrating Static Analysis into the Development Workflow
Integrating static analysis tools into the development workflow is essential for maximizing their effectiveness and ensuring code quality throughout the software development life cycle. The key to successful integration lies in aligning these tools with the existing processes and tools that developers use, fostering an environment where code quality is prioritized at every stage.
To begin with, it is vital to choose the right static analysis tools that fit the specific needs of the project. As mentioned earlier, tools like Checkstyle, PMD, SpotBugs, and SonarQube serve different purposes. By evaluating the requirements of the team and the project, developers can select tools that complement each other and cover a broad range of potential issues.
Once the tools are selected, the next step is to configure them properly. This involves tailoring the analysis rules to suit the project’s coding standards and best practices. For instance, with Checkstyle, teams can create a custom configuration file that enforces their specific coding conventions:
Integrating static analysis tools into the continuous integration (CI) pipeline is another crucial step. This allows the tools to run automatically whenever code is pushed to the repository, providing immediate feedback to developers. For example, using Maven, one can easily include SpotBugs in the build process, ensuring that code is analyzed for potential bugs every time changes are made:
com.github.spotbugs spotbugs-maven-plugin 4.2.3 check
Moreover, configuring the tools to report issues in a way this is easy to understand is paramount. For example, integrating SonarQube can provide a dashboard that visualizes code quality metrics, allowing developers to prioritize issues effectively:
// Example of integrating SonarQube with Maven org.sonarsource.scanner.maven sonar-maven-plugin 3.9.0.2155
Feedback loops are also critical. Developers should be encouraged to review static analysis reports regularly, allowing them to learn from the feedback and improve their coding practices over time. Setting up Git hooks can automate reminders for developers to check static analysis results before merging their code changes.
Finally, fostering a culture that values code quality is vital for long-term success. This involves educating the team about the benefits of static analysis tools and how they can enhance the overall development process. Regular training sessions and discussions about common issues identified by these tools can lead to better coding practices and increased team collaboration.
By thoughtfully integrating static analysis tools into the development workflow, teams can significantly enhance code quality, leading to more reliable, maintainable, and secure Java applications. This proactive approach ensures that developers not only write code but write high-quality code that stands the test of time.