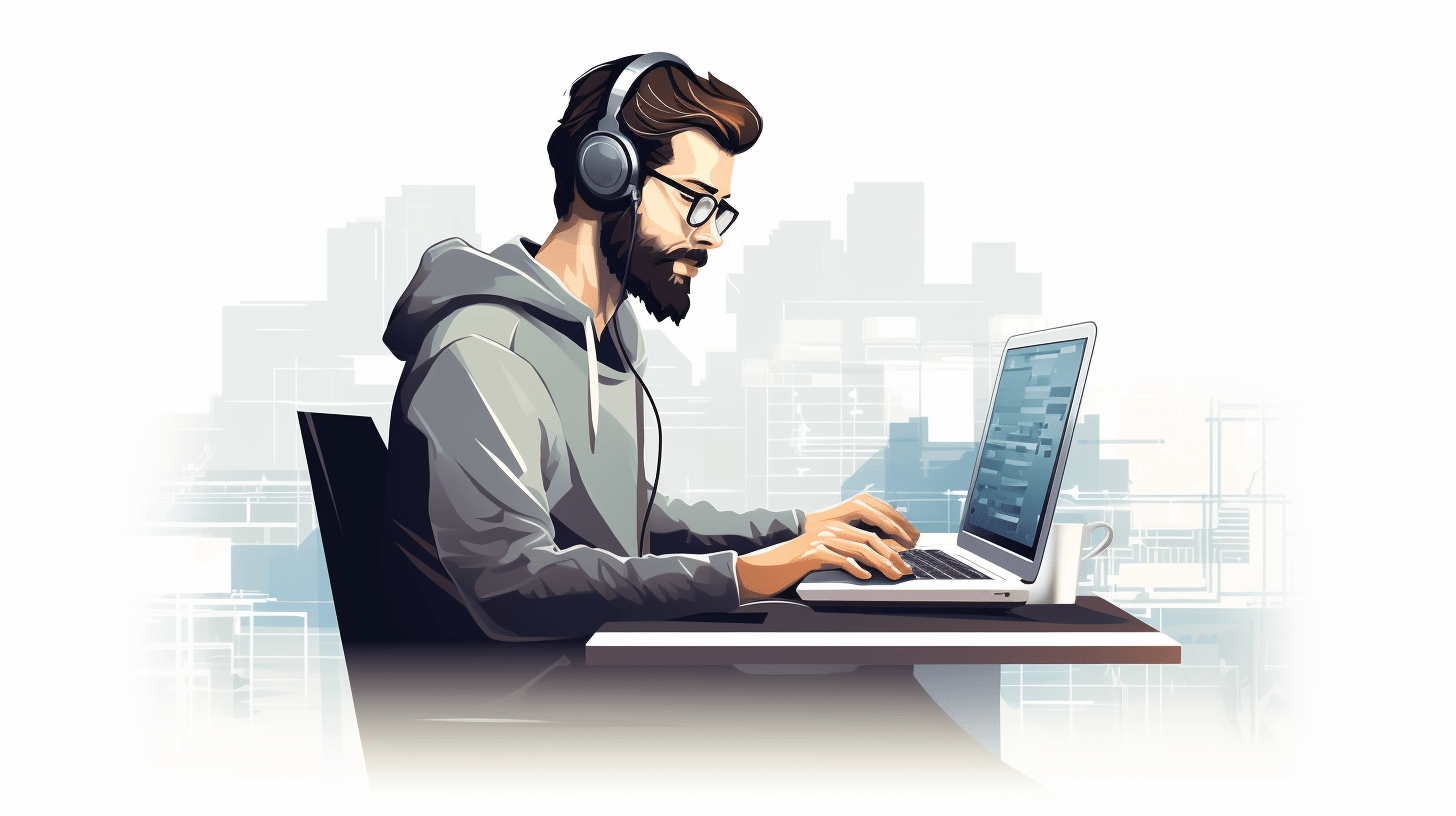
Time and Date Manipulation in Bash
Bash offers a variety of built-in commands and utilities for manipulating dates and times, allowing developers to perform a wide range of operations with relative ease. Two of the most commonly used commands for handling date and time in Bash are date
and time
.
The date
command retrieves the current date and time, and it can also format the output according to specified templates. For example, to display the current date and time in a standard format, you would use:
date
To customize the output format, you can use various format specifiers. For instance, the following command prints the date in “YYYY-MM-DD” format:
date +"%Y-%m-%d"
Here are some common format specifiers you can use with the date
command:
- %Y – Year (e.g., 2023)
- %m – Month (01 to 12)
- %d – Day of the month (01 to 31)
- %H – Hour (00 to 23)
- %M – Minute (00 to 59)
- %S – Second (00 to 59)
Another useful command in Bash is time
, which is primarily used to measure the duration of time taken by a command to execute. For example:
time ls -l
This command will execute ls -l
, and the output will include the real time taken, user CPU time, and system CPU time.
For more advanced date manipulations, the date
command can also handle arithmetic operations. You can calculate dates in the past or future using the -d
option. For example, to find out what the date will be 10 days from now, you can use:
date -d "+10 days"
Similarly, to find out the date 30 days ago, you can write:
date -d "-30 days"
Formatting Dates and Times
When it comes to formatting dates and times in Bash, the versatility of the date
command becomes particularly evident. By combining various format specifiers, you can create output tailored to your specific needs, whether for logging, reporting, or user interfaces.
For example, if you want to display the current date and time in a more verbose manner, such as “Friday, March 10, 2023, 14:30:00”, you can achieve this with the following command:
date +"%A, %B %d, %Y, %H:%M:%S"
In this command:
- %A gives the full weekday name.
- %B provides the full month name.
- %d is the day of the month.
- %Y is the four-digit year.
- %H, %M, and %S provide the hour, minute, and second, respectively.
If you need to output the date in a more compact format, such as “03/10/23”, you can use:
date +"%m/%d/%y"
For applications that require logging timestamps, the ISO 8601 format is often preferred for its unambiguous representation. To display the current date and time in this format, you can execute:
date +"%Y-%m-%dT%H:%M:%S%z"
Here, %z appends the timezone offset, making the output much more informative.
Furthermore, you can format dates from specific timestamps or manipulate strings representing dates. For instance, if you have a date string in a peculiar format, you can convert it to a standard one using:
date -d "03-10-2023" +"%Y-%m-%d"
Additionally, there are scenarios where you may want to format previous or future dates. For example, to display the date that was a week ago in a human-readable way:
date -d "last week" +"%A, %B %d, %Y"
Calculating Time Intervals
Bash provides powerful tools for calculating time intervals, so that you can perform a variety of operations that can streamline your scripts and automate various tasks. These calculations can be particularly useful in scenarios such as logging, scheduling, and conditional operations based on elapsed time.
To begin with, the date
command plays a central role in calculating time intervals. You can easily compute the difference between two dates or determine durations by using the built-in capabilities of the command. For instance, you can use the date
command to capture timestamps and manipulate them as needed.
Suppose you want to calculate the number of days between two dates. You can do this by converting both dates into seconds since the epoch and then performing arithmetic on these values. Here’s a practical example:
start_date="2023-03-01" end_date="2023-03-10" start_seconds=$(date -d "$start_date" +%s) end_seconds=$(date -d "$end_date" +%s) difference=$(( (end_seconds - start_seconds) / 86400 )) # 86400 seconds in a day echo "Difference in days: $difference"
In this example, we first define two date variables. Using the date -d
command, we convert these dates into seconds since the epoch (1 January 1970). The difference is then calculated by subtracting the start date in seconds from the end date, and we divide by the number of seconds in a day (86400) to get the result in days.
Moreover, you can also compute time intervals relative to the current date. For instance, if you want to find out how many days are left until a specific future date:
future_date="2023-12-25" current_seconds=$(date +%s) future_seconds=$(date -d "$future_date" +%s) days_left=$(( (future_seconds - current_seconds) / 86400 )) echo "Days left until $future_date: $days_left"
This snippet captures the current timestamp in seconds and compares it to the timestamp of the future date. The result gives you a simple count of how many days remain until that date.
Another useful application of time interval calculations is in performance measurement. By capturing timestamps before and after a command execution, you can determine the duration of that command. Here’s a simple way to measure the execution time of a command:
start_time=$(date +%s) # Replace 'your_command_here' with the command you want to time your_command_here end_time=$(date +%s) duration=$(( end_time - start_time )) echo "Execution time: $duration seconds"
This script captures the start time, executes a command, and then captures the end time, so that you can compute the total execution duration. This technique is particularly valuable for performance tuning and optimization in scripts where execution time is critical.
Timezone Management in Bash
Timezone management in Bash is important for developing scripts that operate across different geographical locations or for applications that need to ponder local time settings. The Linux operating system provides a robust mechanism for handling time zones, enabling you to work with both the system’s local time and UTC (Coordinated Universal Time).
The system’s default time zone can be viewed or changed through the timedatectl
command, which provides information about the current time zone, local time, and UTC. To check the current time zone, simply run:
timedatectl
To set the time zone, you might use:
sudo timedatectl set-timezone America/New_York
In Bash, you can also use the TZ
environment variable to temporarily set the time zone for a specific command or script execution. This is particularly useful when you need to display or log dates in different time zones without changing the system-wide setting.
For example, to display the current time in Tokyo, you can do the following:
TZ="Asia/Tokyo" date
This command sets the TZ
variable to the Tokyo time zone, and the subsequent date
command will output the current time in that time zone. Similarly, if you want to see the time in London, just replace the time zone string:
TZ="Europe/London" date
When working with multiple time zones, you can also convert between them directly in your scripts. This is particularly handy for scheduling tasks that must occur at the same instant across various locations.
Suppose you have a UTC time you want to convert to a different time zone. Here’s how you can do it:
utc_time="2023-03-10 12:00:00" TZ="America/New_York" date -d "$utc_time UTC"
In this snippet, we first define the UTC time, and then use the date command with the -d
option to interpret it as UTC and convert it to the specified time zone. The output will reflect the equivalent local time in New York.
Timezone conversions can also be used in calculations. If you need to find the time difference between two locations, you can capture their respective times and compute the difference. Consider two cities:
time_in_tokyo=$(TZ="Asia/Tokyo" date +"%s") time_in_new_york=$(TZ="America/New_York" date +"%s") time_difference=$((time_in_tokyo - time_in_new_york)) echo "Time difference in seconds: $time_difference"
This example retrieves the current time in both Tokyo and New York in seconds since the epoch, and computes the difference. The result will inform you how many seconds ahead or behind one city is compared to the other.
Scheduling Tasks with Cron
# Example of using cron for scheduling a task # Open the crontab editor crontab -e # Schedule a script to run every day at 2 AM 0 2 * * * /path/to/your/script.sh
Scheduling tasks with Cron in Bash is a powerful way to automate repetitive tasks, ensuring that scripts execute at specific intervals or times without manual intervention. Cron is a time-based job scheduler in Unix-like operating systems, allowing users to run scripts or commands at scheduled times. After all, who wants to remember to check their logs or run maintenance scripts daily when a simple cron job can do it for you?
To begin using Cron, you can edit the crontab file using the command:
crontab -e
This command opens the current user’s crontab file in the default text editor. Each line in this file represents a scheduled task, formatted in a specific way:
* * * * * command_to_execute
The asterisks correspond to five fields that define when the command should run:
- (0-59)
- (0-23)
- (1-31)
- (1-12)
- (0-7) where both 0 and 7 represent Sunday
For instance, if you want to run a script located at /path/to/your/script.sh
every day at 2 AM, you would add the following line to your crontab:
0 2 * * * /path/to/your/script.sh
This line specifies that at minute 0 of hour 2 (2:00 AM), this script will be executed every day, regardless of the day of the month or the month itself.
Cron also supports more complex scheduling. For example, if you want to run a script every hour on the hour, you can use:
0 * * * * /path/to/your/script.sh
Additionally, you might want to run a script every 15 minutes:
*/15 * * * * /path/to/your/script.sh
Beyond basic scheduling, you can use special strings for common intervals:
- Run once at startup
- Run once a day (equivalent to
0 0 * * *
) - Run once a week (equivalent to
0 0 * * 0
) - Run once a month (equivalent to
0 0 1 * *
) - Run once a year (equivalent to
0 0 1 1 *
)
For example, to run a script at reboot, simply add:
@reboot /path/to/your/script.sh
It’s crucial to ensure that your scripts are executable and that you specify the complete path to the script or command you want to run. Crontab runs commands in a minimal environment, so it’s best practice to use full paths for any commands or scripts.
To view your current cron jobs, simply run:
crontab -l
And if you want to remove all scheduled jobs, you can execute:
crontab -r