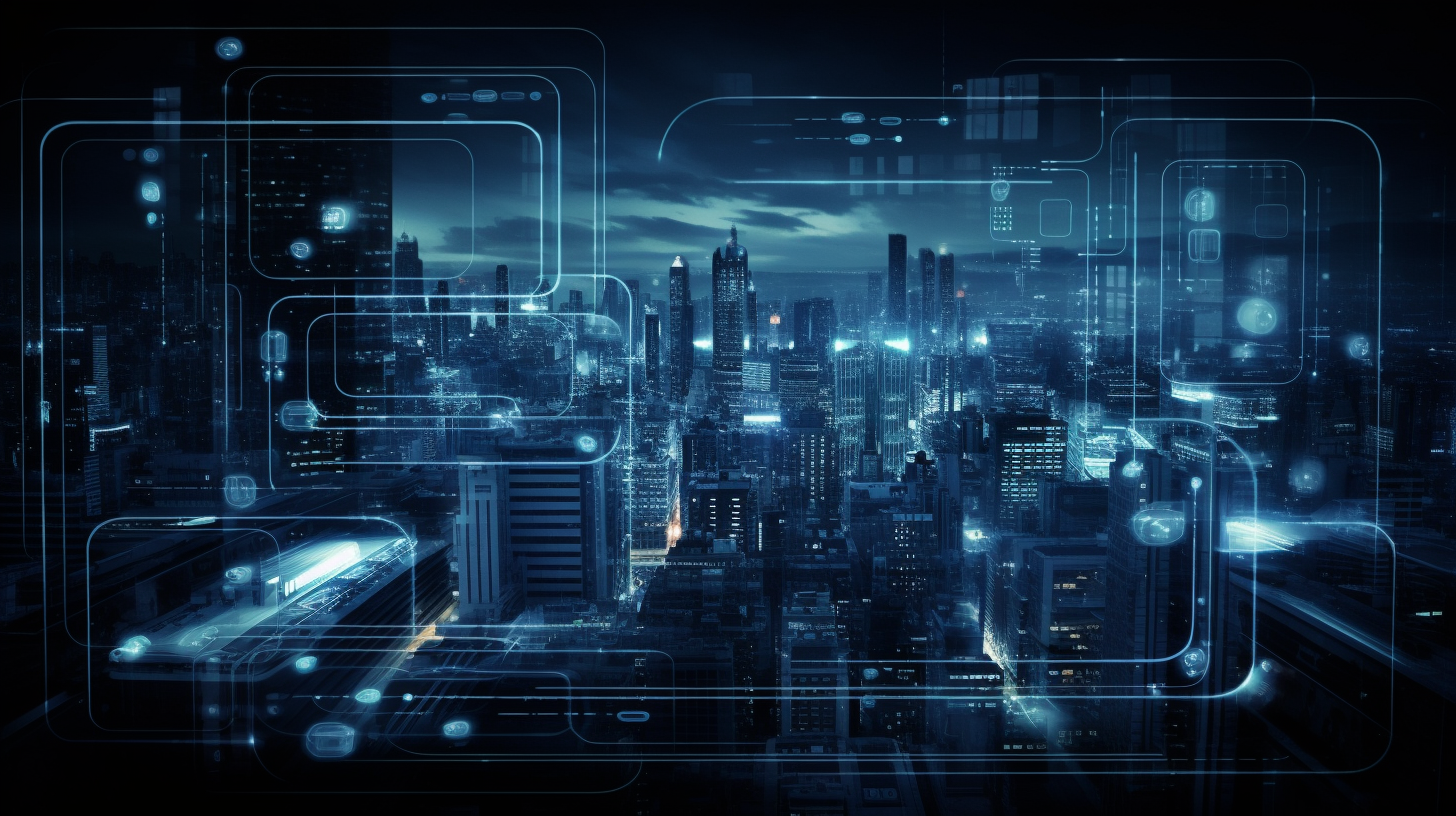
Swift and CoreHaptics
The CoreHaptics framework introduces developers to a world of tactile feedback, allowing for a more immersive user experience by using the haptic capabilities of state-of-the-art iOS devices. At its core, CoreHaptics provides a structured way to create and control haptic patterns, which are sequences of vibrations and feedback that can be finely tuned to enhance interactions within your app.
CoreHaptics is built around the concept of haptic events and patterns. Haptic events are single occurrences of feedback that can be triggered by user interactions, while haptic patterns are more complex sequences that can include multiple events. This ability to customize feedback makes it a powerful tool for engaging users, especially in gaming and interactive applications.
To utilize CoreHaptics, you need to ensure that the device supports haptics; otherwise, your haptic feedback will not function as intended. The framework allows for checking device compatibility through the CHHapticEngine
class, which serves as the main interface for handling haptic playback.
CoreHaptics provides an array of predefined haptic events, such as sharp taps, continuous vibrations, and custom patterns that can be created using various parameters. Developers can use these events to craft a unique feedback experience that resonates with user actions, creating a sense of realism and enriching the interactivity of the app.
To get started with CoreHaptics, you must import the framework into your Swift project. Here’s how you can import the framework:
import CoreHaptics
Once you’ve imported the framework, you can initialize a haptic engine, which will be responsible for managing the playback of haptic patterns. A simple initialization can look like this:
var hapticEngine: CHHapticEngine? do { hapticEngine = try CHHapticEngine() } catch { print("There was an error creating the haptic engine: (error.localizedDescription)") }
By understanding the underlying architecture of the CoreHaptics framework, developers can begin crafting experiences that not only engage users visually but also allure to their sense of touch, making applications more dynamic and enjoyable.
Setting Up CoreHaptics in Your Swift Project
After importing the CoreHaptics framework and initializing your haptic engine, the next step is to ensure that your haptic engine is started and prepared for playback. The engine must be in a running state to play any haptic patterns. This can be accomplished by calling the start()
method on your haptic engine instance. Here’s an example:
try hapticEngine?.start()
It is important to handle errors properly in case the engine fails to start. You should also ensure that your app is capable of responding to interruptions (like phone calls) that may stop the haptic engine, and prepare for it to be resumed. For this, you can implement the CHHapticEngineDelegate
protocol, which provides methods to handle such interruptions.
class HapticFeedbackManager: NSObject, CHHapticEngineDelegate { var hapticEngine: CHHapticEngine? func setupHapticEngine() { do { hapticEngine = try CHHapticEngine() hapticEngine?.delegate = self try hapticEngine?.start() } catch { print("Failed to create and start haptic engine: (error.localizedDescription)") } } func engineDidReset(_ engine: CHHapticEngine) { // Prepare the engine again after it has been reset do { try engine.start() } catch { print("Failed to restart haptic engine: (error.localizedDescription)") } } }
After setting up your haptic engine, it’s essential to check if the device supports haptic feedback. CoreHaptics provides a convenient property CHHapticEngine.capabilitiesForHardware
that can be used to verify if the hardware is capable of generating haptic feedback. You can also query additional capabilities such as the maximum number of simultaneous patterns that the device can handle:
let capabilities = CHHapticEngine.capabilitiesForHardware if capabilities.supportsHaptics { print("This device supports haptics.") } else { print("Haptic feedback is not supported on this device.") }
Once you have ensured that your haptic engine is running and the hardware supports haptics, you can begin creating haptic patterns and events. This process involves defining the individual haptic events you want to use and assembling them into a pattern that can be played back by the haptic engine.
Setting up CoreHaptics within your Swift project involves importing the framework, initializing the haptic engine, managing its state, and ensuring compatibility with the device’s hardware. These foundational steps will pave the way for crafting rich tactile experiences in your applications.
Creating Haptic Patterns and Events
Creating haptic patterns and events within the CoreHaptics framework is a key aspect of designing tactile feedback experiences that resonate with user interactions. To build haptic patterns, you will primarily work with two core components: CHHapticEvent and CHHapticPattern. These classes allow you to define the nature of the haptic feedback you want to deliver and how it will play out over time.
The CHHapticEvent class represents a single haptic event, which can be configured to generate different types of feedback. You can create events that represent single vibrations, sharp taps, or even pauses between feedback. Each event has a specified duration and intensity, allowing for fine control over the feedback experience.
To create a simple haptic event, you can use the following code:
let tapEvent = CHHapticEvent( type: .hapticTransient, // Indicates a transient event parameters: [], relativeTime: 0, // Start immediately duration: 0.1 // Duration in seconds )
In this example, hapticTransient is used to generate a quick tap feedback. The relativeTime parameter indicates when the event should start in relation to the start of the haptic pattern, while duration specifies how long the event lasts.
Once you’ve defined your haptic events, you can combine them to create a CHHapticPattern. This class allows you to sequence multiple haptic events together, crafting dynamic patterns that enhance user interactions. Here’s how to create a pattern using an array of haptic events:
let events: [CHHapticEvent] = [ CHHapticEvent(type: .hapticTransient, parameters: [], relativeTime: 0, duration: 0.1), CHHapticEvent(type: .hapticContinuous, parameters: [ CHHapticEventParameter(parameterID: .hapticIntensity, value: 1.0), CHHapticEventParameter(parameterID: .hapticSharpness, value: 0.5) ], relativeTime: 0.1, duration: 0.5), // Continuous haptic feedback CHHapticEvent(type: .hapticTransient, parameters: [], relativeTime: 0.7, duration: 0.1) ] do { let pattern = try CHHapticPattern(events: events, parameters: []) try hapticEngine?.start() let player = try hapticEngine?.makePlayer(with: pattern) try player?.start(atTime: 0) // Start playback at time zero } catch { print("Failed to create or start haptic pattern: (error.localizedDescription)") }
In this example, we create a sequence of haptic events: a transient tap, followed by a continuous feedback event that lasts half a second, and concluding with another tap. The makePlayer(with:) method is employed to instantiate a player for the defined pattern, which is then played back using start(atTime:).
Understanding how to create and manage haptic patterns effectively allows you to design richer, more engaging interactions within your applications. By combining various haptic events, you can provoke different emotional responses, making the tactile aspect of your app as compelling as its visual or auditory elements.
Customizing Haptic Feedback Experience
Customizing the haptic feedback experience is where the true power of the CoreHaptics framework lies. By adjusting parameters and creating bespoke patterns, developers can tailor feedback to suit the unique needs of their applications, enhancing the connection between users and their devices. This customization allows for a deeper interaction, making every tap, swipe, or gesture resonate with meaning.
The CoreHaptics framework provides extensive options for customization, primarily through the manipulation of haptic event parameters. Each haptic event can be finely tuned for intensity and sharpness, which directly influences how the feedback feels to the user. For example, a subtle vibration might be suitable for a confirmation action, while a stronger feedback could be used for an alert or warning.
When creating a haptic event, developers can specify parameters such as hapticIntensity and hapticSharpness. The intensity determines how strong the haptic feedback is, while sharpness affects the perceived quickness or aggressiveness of the feedback. Here’s an example of how to create a customizable haptic event:
let customHapticEvent = CHHapticEvent( type: .hapticTransient, parameters: [ CHHapticEventParameter(parameterID: .hapticIntensity, value: 0.75), // 0.0 to 1.0 CHHapticEventParameter(parameterID: .hapticSharpness, value: 0.25) // 0.0 to 1.0 ], relativeTime: 0, duration: 0.2 )
In this example, the intensity is set to 0.75, providing a strong vibration, whereas the sharpness is reduced to 0.25, creating a more subdued and less jarring feel. When crafting these events, it’s crucial to think the context of their use; for instance, a light, quick tap may be appropriate for selecting an item, while a more intense feedback might accompany a critical error.
Moreover, creating haptic patterns allows developers to sequence multiple haptic events for a richer user experience. Patterns can incorporate varying sequences of events, each with its own parameters. By doing so, developers can create complex interactions that guide users through tasks or reinforce actions. Here’s how you can create a sophisticated haptic pattern:
let hapticPatternEvents: [CHHapticEvent] = [ CHHapticEvent(type: .hapticTransient, parameters: [], relativeTime: 0, duration: 0.1), // Quick tap CHHapticEvent(type: .hapticContinuous, parameters: [ CHHapticEventParameter(parameterID: .hapticIntensity, value: 1.0), CHHapticEventParameter(parameterID: .hapticSharpness, value: 0.8) ], relativeTime: 0.1, duration: 0.5), // Strong continuous feedback CHHapticEvent(type: .hapticTransient, parameters: [], relativeTime: 0.7, duration: 0.1) // Another quick tap ]
By adjusting the duration and timing of each event, developers can create a flow that feels natural and engaging. This example illustrates a sequence that includes a quick tap, followed by a strong continuous feedback, and finishing with another quick tap. Such a combination can be especially effective in scenarios like game interactions or confirmation dialogs.
It’s also worth noting that the user’s preferences and the context of use can influence how haptic feedback is perceived. For example, in a quiet environment, users might prefer more subtle feedback, whereas in a noisy setting, a stronger vibration could be required to gain attention. Therefore, providing users with options to adjust their haptic feedback settings can greatly enhance their experience.
Lastly, testing the responsiveness and feel of the haptic feedback across various devices is essential. Different iPhones may have variations in their haptic engines, leading to discrepancies in how feedback is perceived. By meticulously crafting and testing haptic feedback patterns, developers can ensure a consistent and satisfying user experience.
Best Practices for Haptic Feedback Implementation
When implementing haptic feedback in your applications, adhering to best practices is vital to ensure that the experience is not only engaging but also enhances the overall usability of your app. Here are some key considerations for effective haptic feedback implementation using the CoreHaptics framework.
1. Use Haptics Purposefully
Haptic feedback should serve a specific purpose and not be overused, as excessive feedback can lead to annoyance rather than enhancement. Each haptic event should correspond to a meaningful action or state change within the app. For instance, using a subtle vibration for a successful action, such as saving a file, reinforces a positive interaction, while a strong feedback could signify an error.
For example, when a user successfully completes a task, you might want to provide a gentle feedback:
let successFeedback = CHHapticEvent( type: .hapticTransient, parameters: [CHHapticEventParameter(parameterID: .hapticIntensity, value: 0.6)], relativeTime: 0, duration: 0.1 )
In contrast, for an error, a sharper and more intense feedback is appropriate:
let errorFeedback = CHHapticEvent( type: .hapticTransient, parameters: [ CHHapticEventParameter(parameterID: .hapticIntensity, value: 1.0), CHHapticEventParameter(parameterID: .hapticSharpness, value: 0.9) ], relativeTime: 0, duration: 0.2 )
2. Ponder the Context
The context in which haptic feedback is delivered plays an important role in its effectiveness. Haptics should align with the visual and audio cues of your app. For instance, in a game setting, where quick responses and immersive feedback are critical, haptic patterns may need to be more aggressive and pronounced. Conversely, in productivity applications, subtle feedback may be more appropriate.
Testing haptic feedback patterns in different scenarios can help you refine their timing and intensity. Always consider user feedback, as it can provide insights into whether the haptic responses enhance or detract from the experience.
3. Ensure Accessibility
Accessibility is a fundamental aspect of app design. Some users may have sensitivities to vibrations or may not feel haptic feedback at all. Providing options to adjust haptic feedback or to disable it altogether can make your app more inclusive. You can add settings that allow users to choose their preferred haptic intensity or turn off feedback completely.
4. Test Across Devices
Different devices may have varying haptic capabilities and strengths. It is essential to test your haptic feedback on multiple device models to ensure a consistent experience. What feels strong and responsive on one device may feel weak on another. Adjust your haptic patterns accordingly to accommodate these differences.
5. Avoid Feedback Fatigue
To prevent user fatigue, vary the types of haptic feedback used throughout your app. If the same haptic pattern is used repetitively, users may start to tune it out, diminishing its effectiveness. Mixing different types of feedback—such as transient taps for selections and continuous feedback for critical notifications—can help maintain user engagement.
The implementation of haptic feedback should be a deliberate and thoughtful process. By using haptics purposefully, considering context, ensuring accessibility, testing across devices, and avoiding feedback fatigue, developers can create a tactile experience that genuinely enhances user interaction and satisfaction with their applications. By taking these best practices into account, you can leverage the full potential of the CoreHaptics framework to create immersive and responsive applications that resonate with users on a deeper level.