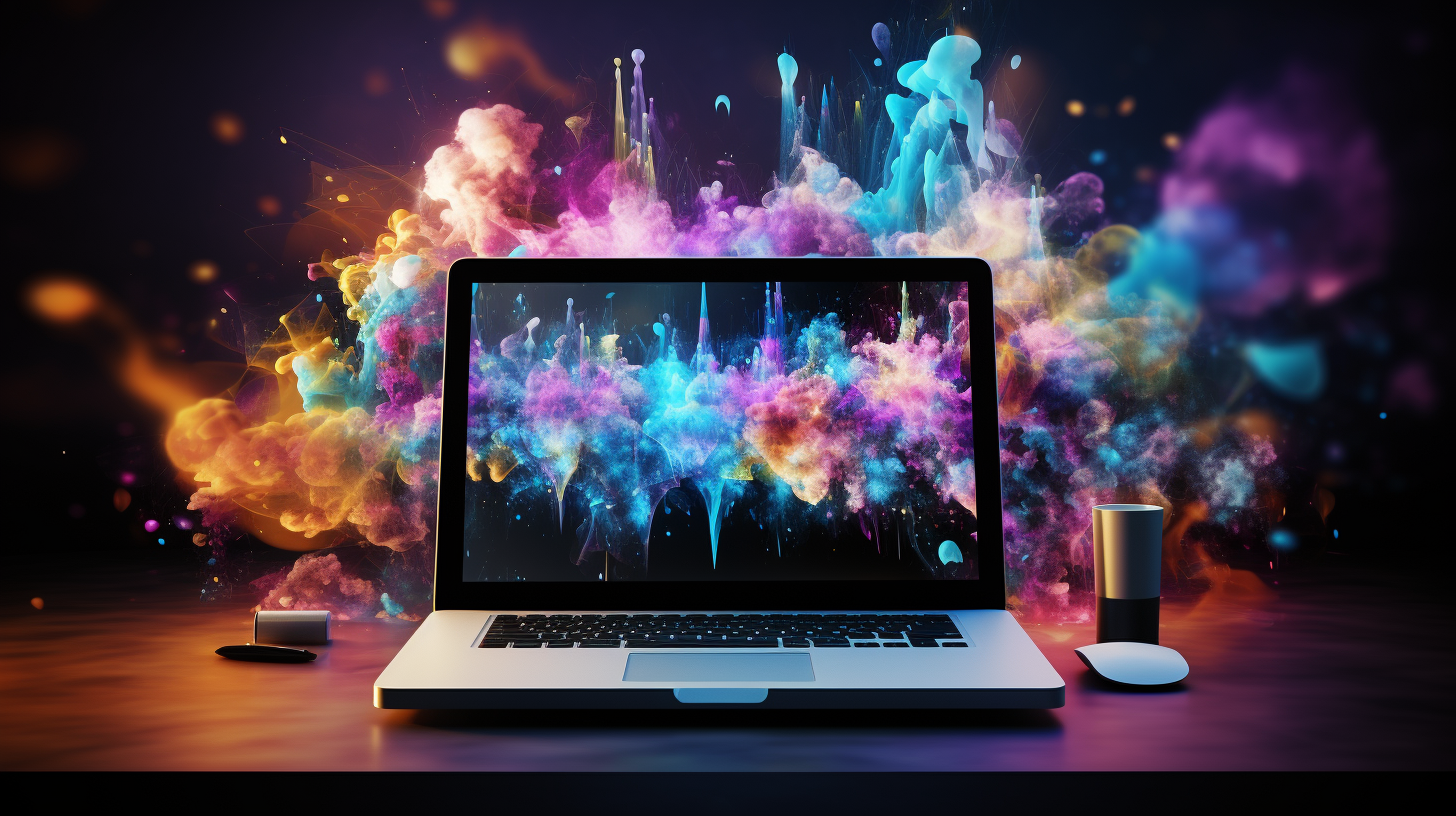
JavaScript for Freelancers: Tips and Tricks
As a freelancer in the sphere of JavaScript, mastering essential skills especially important to stand out in a competitive landscape. The foundation of your career will rest on your technical proficiency, but understanding the nuances of the language will elevate your work.
Core JavaScript Concepts are non-negotiable. Familiarity with variables, data types, functions, and control structures forms the bedrock upon which all JavaScript applications are built. It’s critical to not only memorize syntax but to grasp how these elements interact to produce dynamic applications.
// Example of variable declaration and data types
let name = 'Alice'; // String
const age = 30; // Number
let isFreelancer = true; // Boolean
// Function to greet a user
function greet(userName) {
return `Hello, ${userName}!`;
}
console.log(greet(name)); // Outputs: Hello, Alice!
Moving beyond the basics, asynchronous programming is another pivotal skill. Understanding callbacks, promises, and async/await will empower you to write efficient, responsive applications. That’s particularly important when dealing with APIs or any operations that involve waiting for a response.
// Using async/await for asynchronous operations
async function fetchData(url) {
try {
const response = await fetch(url);
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData('https://api.example.com/data');
Understanding the Document Object Model (DOM) is equally essential. Being able to manipulate the DOM with JavaScript allows you to create interactive web applications. Familiarity with methods such as getElementById, querySelector, and event handling will serve you well.
// Example of DOM manipulation
document.getElementById('myButton').addEventListener('click', function() {
alert('Button clicked!');
});
Moreover, a solid grasp of JavaScript frameworks and libraries, such as React, Vue, or Angular, can significantly enhance your marketability. These tools not only expedite development but also provide structure and scalability to your projects.
// Simple React component example
import React from 'react';
function Greeting() {
return Hello, World!
;
}
export default Greeting;
Lastly, version control systems, particularly Git, are indispensable for freelancers. Knowing how to effectively use Git allows you to track changes, collaborate with others, and maintain a history of your work.
# Example Git commands
git init # Initialize a new Git repository
git add . # Stage changes
git commit -m "Initial commit" # Commit changes
git push origin main # Push changes to the main branch
Mastering these essential JavaScript skills will not only help you deliver high-quality projects but also build a reputation as a dependable freelancer in the tech community. The combination of technical acumen and reliable practices will set you on a path to success.
Building a Strong Portfolio with JavaScript Projects
Building a solid portfolio is a fundamental step for any freelancer looking to showcase their JavaScript capabilities and attract potential clients. A well-crafted portfolio is not merely a collection of projects; it’s a narrative that reflects your skills, creativity, and problem-solving abilities. Here are several strategies to assemble an impressive portfolio that highlights your JavaScript expertise.
First and foremost, choose diverse projects that demonstrate a range of skills. Ideally, your portfolio should include examples of both simple and complex tasks. For instance, a basic project could be a to-do list application, while a more advanced project might involve integrating third-party APIs to fetch and display data.
// Simple to-do list application const tasks = []; function addTask(task) { tasks.push(task); console.log(`Task added: ${task}`); } function showTasks() { console.log('Current Tasks:'); tasks.forEach(task => console.log(task)); } addTask('Learn JavaScript'); addTask('Build portfolio'); showTasks();
Next, ensure that you include live demonstrations of your projects. A GitHub repository with code is essential, but having a live version of your application can significantly increase your portfolio’s impact. Tools like GitHub Pages, Vercel, or Netlify make it easy to deploy your JavaScript applications and showcase them in action.
// Example of deploying a React app with Vercel // In the terminal, after creating your React app: vercel login // Log in to your Vercel account vercel init // Initialize your project vercel deploy // Deploy your project
Collaboration projects can also add depth to your portfolio. Working on open-source projects or collaborating with other developers can demonstrate your ability to work within a team, which is often a key consideration for potential clients. Engage in platforms like GitHub and contribute to projects that pique your interest.
Additionally, document your thought process and the challenges you faced during development. Writing blog posts or creating video tutorials about your projects not only reinforces your understanding but also showcases your communication skills. Clients appreciate seeing how you approach problem-solving.
// Example of a problem-solving approach in a blog post // Topic: How I solved the issue of state management in React /* The challenge was managing state across multiple components. I researched various solutions and settled on using React's Context API, which allowed me to avoid prop drilling and made my components cleaner. */ // Context setup import React, { createContext, useState } from 'react'; const MyContext = createContext(); function MyProvider({ children }) { const [state, setState] = useState(initialState); return ( {children} ); }
Lastly, prioritize quality over quantity. It’s better to showcase 3-5 high-quality projects than to include a dozen mediocre ones. Each project should reflect your best work and your ability to follow best practices in code quality, such as using meaningful variable names, proper indentation, and code organization.
A strong portfolio is an essential tool for any freelance JavaScript developer. By carefully selecting projects, showcasing live demos, documenting your process, and prioritizing quality, you can create a compelling narrative that attracts clients and sets you apart in the competitive freelance marketplace.
Effective Communication with Clients
Effective communication is a cornerstone of freelance success, especially for JavaScript developers. When working with clients, bridging the gap between technical jargon and client understanding is paramount. This skill not only enhances client satisfaction but also fosters long-term relationships and repeat business.
To begin with, clarity is vital. When discussing project requirements, it’s crucial to avoid overwhelming clients with technical details. Instead, focus on the outcomes and benefits of the solutions you propose. Use simple language and analogies that relate to their business needs. For instance, if a client is unsure about the need for a specific JavaScript framework, explain how it can enhance their website’s performance or user experience without diving too deep into the technical intricacies.
Active listening plays a significant role in effective communication. Make sure to fully understand the client’s needs and expectations. This means asking clarifying questions, paraphrasing what they say to confirm understanding, and taking notes during discussions. By demonstrating that you value their input, you build trust and rapport.
Regular updates are another essential aspect of communication. Clients appreciate being kept in the loop regarding project progress. Set a schedule for updates and stick to it, whether it is weekly or bi-weekly. Use this time to highlight achievements, outline upcoming tasks, and discuss any obstacles you may be facing. This transparency can alleviate client anxieties and reinforce their confidence in your abilities.
Moreover, the use of collaborative tools can enhance communication. Platforms like Slack, Trello, or Asana can help streamline discussions and project management. For instance, using Trello to track tasks allows clients to see progress in real time, minimizing the need for constant updates while still keeping them informed.
When it comes to technical discussions, using visuals can significantly aid understanding. Diagrams, flowcharts, and wireframes can effectively convey concepts that might be complex if explained through text alone. For example, if you’re explaining the structure of a web application, a simple flowchart can show how components interact with each other, making the information more digestible.
// Example of a simple flowchart for a web application structure // Client-side rendering function App() { return (); } // Server-side interaction async function fetchData() { const response = await fetch('/api/data'); const data = await response.json(); return data; }
Lastly, always be open to feedback. Encourage clients to share their thoughts on your work and communication style. This not only helps you improve but also shows clients that their opinions are valued. Be prepared to adapt your communication approach based on their preferences, whether they prefer detailed technical reports or high-level summaries.
In short, effective communication encompasses clarity, active listening, regular updates, collaborative tools, the use of visuals, and openness to feedback. By honing these skills, you can elevate your freelance JavaScript career, ensuring that your clients feel heard, informed, and satisfied throughout the project lifecycle.
Time Management Strategies for Developers
Time management is an essential skill for freelancers, particularly for JavaScript developers who often juggle multiple projects, deadlines, and client expectations. Mastering the art of time management can significantly enhance productivity and lead to a more satisfying work experience. Here are some effective strategies to help you manage your time better and maximize your efficiency.
First, start by setting clear goals and priorities. Break your workload into manageable tasks and prioritize them based on their deadlines and importance. Using the Eisenhower Matrix can help you categorize tasks into four quadrants: urgent and important, important but not urgent, urgent but not important, and neither urgent nor important. This method allows you to focus on what truly matters, ensuring that you dedicate your energy to high-impact activities.
// Example of prioritizing tasks const tasks = [ { name: 'Fix bug in project A', urgent: true, important: true }, { name: 'Client meeting', urgent: true, important: false }, { name: 'Update documentation', urgent: false, important: true }, { name: 'Research new technologies', urgent: false, important: false } ]; const prioritizeTasks = (tasks) => { return tasks.sort((a, b) => (b.urgent === a.urgent ? b.important - a.important : b.urgent - a.urgent)); }; console.log(prioritizeTasks(tasks));
Establishing a routine can also bolster your time management. Create a daily schedule that allocates specific blocks of time for focused work, meetings, and breaks. This structured approach can help minimize distractions and improve your concentration. Utilize techniques like the Pomodoro Technique, which involves working in short, focused bursts followed by brief breaks. This method not only enhances productivity but also helps prevent burnout.
// Example of a Pomodoro timer implementation function startPomodoro(duration) { let timeLeft = duration * 60; // Convert minutes to seconds const timerId = setInterval(() => { if (timeLeft <= 0) { clearInterval(timerId); console.log('Pomodoro completed!'); } else { console.log(`Time left: ${Math.floor(timeLeft / 60)}:${timeLeft % 60}`); timeLeft--; } }, 1000); } startPomodoro(25); // Start a 25-minute Pomodoro
Another key strategy is to leverage productivity tools and apps. Tools like Trello, Asana, or Notion can help you organize tasks, set deadlines, and track progress, while time-tracking apps such as Toggl or Clockify allow you to monitor how much time you spend on different tasks. Understanding where your time goes can reveal patterns and help you adjust your strategies for better efficiency.
// Example of using a simple object to track time spent on tasks const timeTracker = { tasks: {}, addTask(taskName) { this.tasks[taskName] = { timeSpent: 0 }; }, logTime(taskName, seconds) { if (this.tasks[taskName]) { this.tasks[taskName].timeSpent += seconds; } }, getReport() { console.log(this.tasks); } }; timeTracker.addTask('Project A'); timeTracker.logTime('Project A', 120); timeTracker.getReport();
Furthermore, learn to say no when necessary. As a freelancer, it’s tempting to take on every project that comes your way, but overcommitting can lead to stress and subpar work. Assess each opportunity carefully, and be honest with yourself about your current bandwidth. Saying no to lower-priority projects can free up time for the work that truly matters.
Lastly, regularly review your progress and adjust your strategies. Set aside time at the end of each week to reflect on what you accomplished, what challenges you faced, and how you can improve your time management skills. This reflective practice can aid in identifying inefficiencies and help you stay on track with your goals.
By implementing these time management strategies, you can enhance your productivity, meet deadlines more effectively, and ultimately create a more balanced freelance lifestyle as a JavaScript developer.
Staying Updated with JavaScript Trends and Tools
> 0) {
console.log(`Time left: ${Math.floor(timeLeft / 60)}:${timeLeft % 60}`);
timeLeft–;
} else {
clearInterval(timerId);
console.log(‘Pomodoro session complete! Take a short break.’);
}
}, 1000);
}
startPomodoro(25); // Starts a 25-minute Pomodoro session
Another key aspect of effective time management is the use of tools and resources. Leverage project management tools like Trello, Asana, or Notion to keep track of your tasks and deadlines. These platforms enable you to visualize your progress and ensure nothing slips through the cracks. Additionally, using time-tracking apps like Toggl can provide insights into how you allocate your time across various tasks, highlighting areas for improvement.
Delegation is also an invaluable strategy for freelancers. Recognize when you have too much on your plate and don’t hesitate to delegate tasks to others, whether by outsourcing specific work or collaborating with fellow developers. This allows you to focus on high-value tasks that require your unique expertise, ultimately enhancing your productivity.
Lastly, don’t forget the importance of regular reflection and adjustment. At the end of each week, take time to evaluate what worked and what didn’t. Identify any time-wasting activities and strategize on how to eliminate or minimize them. Adapting your approach based on your experiences will lead to continuous improvement in your time management skills.
Staying Updated with JavaScript Trends and ToolsIn the fast-paced realm of JavaScript development, staying updated with the latest trends and tools is not just an option; it is a necessity. As a freelancer, your ability to adapt to new technologies can significantly influence your marketability and the quality of your work. Here’s how you can keep your skills sharp and stay ahead in the game.
First and foremost, follow reputable sources of information. Websites like MDN Web Docs, JavaScript Weekly, and Smashing Magazine provide a wealth of resources, tutorials, and articles that cover the latest developments in JavaScript. Subscribing to newsletters, podcasts, and YouTube channels that focus on JavaScript can also provide regular insights into new tools, libraries, and best practices.
Engaging with the community is another effective way to stay informed. Platforms like Twitter, Stack Overflow, and Reddit can be invaluable for networking and discovering emerging trends. Participating in discussions, asking questions, and sharing your knowledge can lead to learning opportunities and collaborations that keep you in the loop.
Moreover, consider attending conferences and meetups, whether in-person or virtually. Events like JSConf, React Conf, or local JavaScript meetups offer a chance to learn from industry leaders and fellow developers, providing insights that you might not find in articles. These gatherings often feature workshops and presentations that focus on cutting-edge tools and frameworks.
Experimenting with new technologies in your own projects is an excellent way to deepen your understanding. For example, exploring frameworks like Svelte or libraries like D3.js can broaden your skill set and reveal new ways to approach problems. By integrating these tools into your workflow, you’ll not only gain hands-on experience but also discover practical applications that can benefit your freelance work.
Additionally, think contributing to open-source projects. This not only allows you to apply new skills but also helps you understand real-world applications of trends and technologies. Engaging with existing projects on GitHub can expose you to best practices, code reviews, and contributions from other developers, enriching your learning experience.
Lastly, keep an eye on the job market to understand what skills are in demand. Websites like LinkedIn, Glassdoor, and job boards specific to tech can provide insights into which frameworks, libraries, and tools employers are seeking. By aligning your learning path with market needs, you ensure that your skills remain relevant and attractive to potential clients.
Staying updated with JavaScript trends and tools is an ongoing journey that requires commitment and curiosity. By engaging with the community, experimenting with new technologies, attending events, and following credible sources, you can maintain a competitive edge in your freelance career.