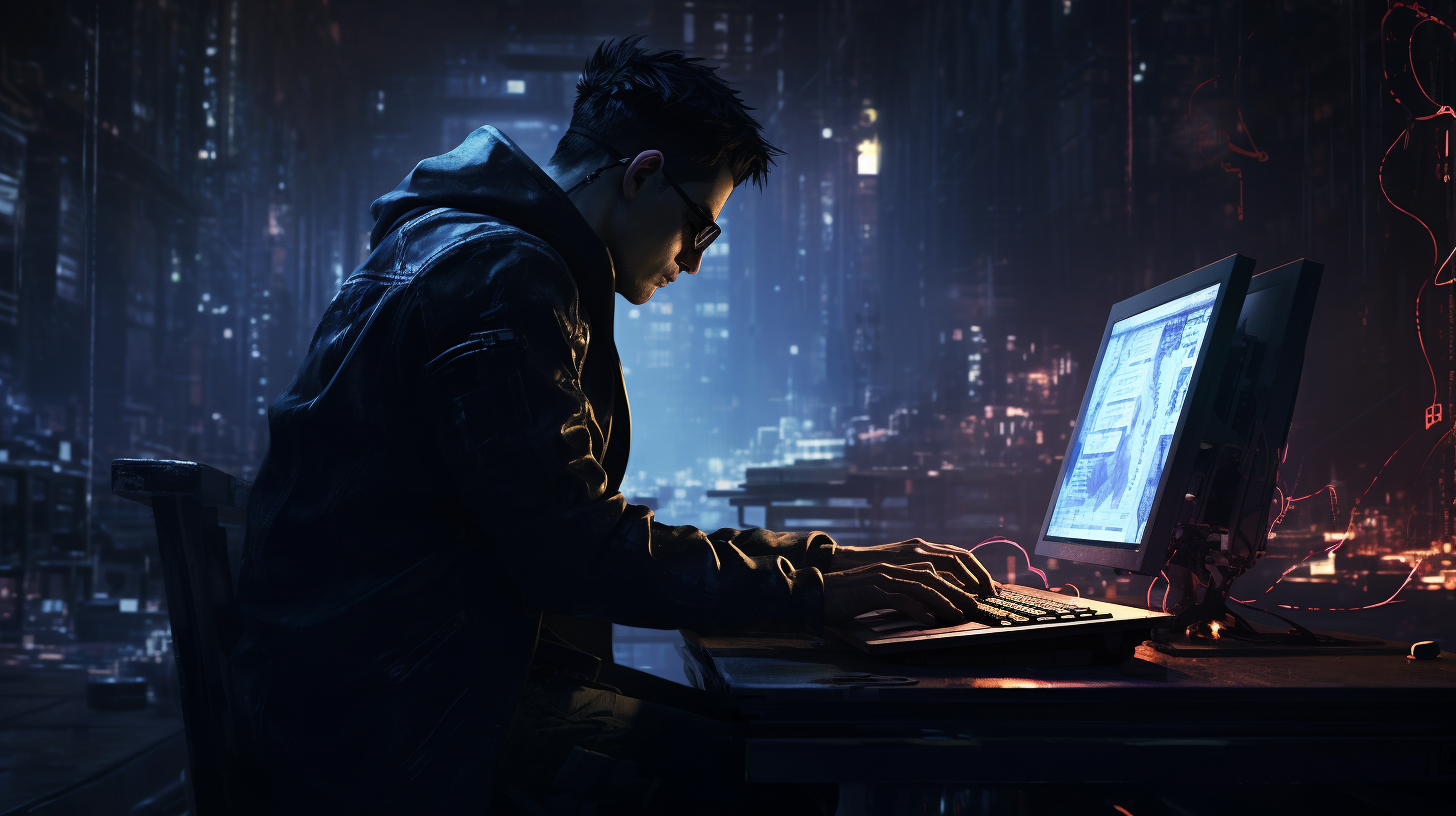
Bash and SQL Database Operations
When it comes to managing SQL databases, Bash is often overlooked as a powerful scripting language. However, its strength lies in its ability to facilitate database management tasks through automation and integration. With a few succinct commands, you can perform operations such as data retrieval, updates, and even complex transaction management.
One of the defining features of Bash is its capacity to combine shell commands with SQL operations. This synergy allows you to leverage the power of SQL while maintaining the flexibility and control that Bash provides. For instance, by using command substitution, you can capture the output of SQL queries and manipulate it directly within a Bash script.
Think the following example, where we utilize the mysql
command-line client to connect to a MySQL database and retrieve a list of users:
#!/bin/bash # Define database credentials DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # Execute SQL query to fetch users USER_LIST=$(mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "SELECT user FROM mysql.user;") # Display the result echo "List of users in the database:" echo "$USER_LIST"
In this script, we define the database credentials and employ the mysql
command to execute a SQL query. The query’s output is captured in the USER_LIST
variable, showcasing how simpler it is to integrate SQL commands within a Bash script.
Moreover, Bash shines when it comes to handling repetitive tasks. Imagine needing to back up your database regularly. You can create a simple Bash script that uses the mysqldump
utility, making it easy to automate your database backup process:
#!/bin/bash # Define backup destination BACKUP_DIR="/path/to/backup" DATE=$(date +%Y%m%d) DB_NAME="your_database" # Perform database backup mysqldump -u "$DB_USER" -p"$DB_PASS" "$DB_NAME" > "$BACKUP_DIR/$DB_NAME-$DATE.sql" echo "Backup completed for $DB_NAME on $DATE."
In this example, the script generates a timestamped backup of the specified database by using the mysqldump
command. The ability to schedule this script using cron underscores Bash’s utility in managing database operations efficiently.
In essence, while Bash is not inherently a database management tool, its scripting capabilities enable it to serve as a robust interface for interacting with SQL databases. The seamless integration of SQL commands within Bash scripts allows for powerful, automated solutions that simplify complex database management tasks.
Connecting to SQL Databases with Bash
To establish a connection to an SQL database using Bash, you’ll typically rely on command-line clients that correspond to your database management system (DBMS). For instance, MySQL uses the `mysql` client, while PostgreSQL utilizes `psql`. Each of these clients requires specific connection parameters, including the database name, user credentials, and the hostname of the database server.
When connecting to a database, you must ensure that you have the necessary permissions and that the database server is accessible. This setup often involves specifying the username, password, and database name in your connection string. Below is an example of how to connect to a MySQL database from a Bash script:
#!/bin/bash # Define database connection parameters DB_HOST="localhost" DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # Connect to MySQL and verify the connection mysql -h "$DB_HOST" -u "$DB_USER" -p"$DB_PASS" "$DB_NAME" -e "SELECT 1;" &> /dev/null # Check the exit status of the connection command if [ $? -eq 0 ]; then echo "Connection to $DB_NAME successful!" else echo "Failed to connect to $DB_NAME." fi
In this script, we define the database connection parameters and attempt to connect to the MySQL database using the `mysql` command. The command executes a simple SQL statement (`SELECT 1;`) to check if the connection is successful. The output of the command is redirected to /dev/null to avoid cluttering the terminal. After attempting the connection, we check the exit status using `$?`. A status of `0` indicates success, while any other value signals failure.
Similarly, when connecting to a PostgreSQL database, the approach is analogous but utilizes the `psql` command. Here’s how you can connect to a PostgreSQL database:
#!/bin/bash # Define database connection parameters DB_HOST="localhost" DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # Export the password for the psql command export PGPASSWORD="$DB_PASS" # Connect to PostgreSQL and verify the connection psql -h "$DB_HOST" -U "$DB_USER" -d "$DB_NAME" -c "SELECT 1;" &> /dev/null # Check the exit status of the connection command if [ $? -eq 0 ]; then echo "Connection to $DB_NAME successful!" else echo "Failed to connect to $DB_NAME." fi # Unset the password variable for security unset PGPASSWORD
In this PostgreSQL example, we export the password to the `PGPASSWORD` environment variable for the duration of the script. The `psql` command is then executed with options to specify the host, user, database, and a simple SQL command to test the connection.
By efficiently managing your database connections through Bash scripts, you can create robust automation routines that streamline interactions with your SQL databases. Whether it’s checking connections, running queries, or performing maintenance tasks, using the capabilities of Bash in conjunction with SQL command-line clients can significantly enhance your database management workflows.
Executing SQL Queries from Bash Scripts
Executing SQL queries directly from Bash scripts offers a seamless way to integrate database operations into your automation workflows. The ability to run complex SQL commands without leaving the familiar environment of the shell opens up a world of possibilities for data manipulation and management. By using command-line clients like `mysql` for MySQL databases or `psql` for PostgreSQL, you can easily embed SQL commands in your scripts, allowing for efficient data retrieval, updates, and even batch processing of multiple queries.
To execute SQL queries effectively, you simply embed your command within a Bash script, using the power of command substitution or piping. Think this simple example where you execute a query to insert a new record into a MySQL database:
#!/bin/bash # Define database credentials DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # SQL query to insert a new user INSERT_QUERY="INSERT INTO users (username, email) VALUES ('new_user', '[email protected]');" # Execute SQL query mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "$INSERT_QUERY" echo "New user inserted successfully."
In this script, we define the SQL query to insert a new user and then execute it directly using the `mysql` command. The SQL command is passed as a string to the `-e` option, making it simpler to modify or extend as needed. This pattern exemplifies how Bash can serve not just as a scripting language but as a facilitator for direct SQL operations.
Moreover, you might encounter situations where you need to execute multiple queries or handle complex logic based on query results. Here’s an example of executing a series of SQL commands that first updates a user’s email and then retrieves a list of all users after the update:
#!/bin/bash # Define database credentials DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # SQL commands to execute UPDATE_QUERY="UPDATE users SET email = '[email protected]' WHERE username = 'new_user';" SELECT_QUERY="SELECT * FROM users;" # Execute the update query mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "$UPDATE_QUERY" # Execute the select query and capture output USER_LIST=$(mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "$SELECT_QUERY") # Display the updated list of users echo "Updated user list:" echo "$USER_LIST"
In this example, we execute an update query followed by a select query to display the current state of the user table. The output of the select command is captured and printed to the console, demonstrating how you can chain SQL operations and manipulate the results within Bash.
The flexibility of Bash scripts extends to handling error checking and conditional logic based on the success or failure of your SQL commands. You can easily inspect the exit status of commands and take action accordingly. Here’s a refined example that includes error handling:
#!/bin/bash # Define database credentials DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # SQL command to create a new table CREATE_TABLE_QUERY="CREATE TABLE IF NOT EXISTS users (id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(50), email VARCHAR(100));" # Execute the command if mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "$CREATE_TABLE_QUERY"; then echo "Table 'users' created or already exists." else echo "Failed to create table 'users'." exit 1 fi
In this case, we check if the table creation command was successful and handle any errors gracefully, providing feedback that can inform further actions. This pattern of using conditional statements around SQL execution can drastically improve the robustness of your database automation scripts.
Ultimately, executing SQL queries from Bash scripts is not just about running commands; it’s about crafting a cohesive workflow that ties database management into the broader landscape of system operations. With Bash as your ally, you can automate routine tasks, enforce data integrity, and orchestrate complex data interactions with precision and ease.
Automating Database Operations with Bash Scripts
Automating database operations with Bash scripts can significantly enhance your efficiency and reduce the likelihood of human error in repetitive tasks. Whether it is scheduling regular database backups, updating records based on specific criteria, or generating reports from your datasets, Bash provides the tools needed to create scripts that run these tasks seamlessly.
One of the most common tasks you might want to automate is the regular backup of your database. In the previous example, we created a script that utilized the mysqldump
command to save a snapshot of the database. However, this script can be further enhanced with automated scheduling using cron
, which allows you to specify when and how often the script should run.
Here’s how you can set up a cron job to automate the backup process:
0 2 * * * /path/to/your/backup_script.sh
This cron entry executes backup_script.sh
every day at 2 AM. By scheduling the backup this way, you ensure that you always have a recent version of your database, ready to restore in case of an emergency.
Beyond backups, automating data updates can also dramatically streamline your workflow. Consider a scenario where you need to update user privileges in a database based on their activity. You could write a Bash script that queries for users who require updates and then applies those changes accordingly:
#!/bin/bash # Define database credentials DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # Get a list of users needing updates NEED_UPDATES=$(mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "SELECT username FROM users WHERE last_login < NOW() - INTERVAL 1 YEAR;") # Loop through users and update privileges while read -r USER; do if [ "$USER" != "username" ]; then echo "Updating privileges for user: $USER" mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "UPDATE users SET privilege_level = 'basic' WHERE username = '$USER';" fi done <<< "$NEED_UPDATES"
This script retrieves a list of users who haven’t logged in for over a year and updates their privilege levels. The loop iterates over each user, issuing an SQL command to update their privileges directly.
Furthermore, generating and sending reports is another area where Bash automation shines. By running SQL queries and formatting the output, you can create reports that provide insights into your database’s current state or performance metrics.
#!/bin/bash # Define database credentials DB_USER="your_username" DB_PASS="your_password" DB_NAME="your_database" # SQL query to generate a report on user activity REPORT_QUERY="SELECT username, COUNT(*) AS login_count FROM user_activity GROUP BY username;" # Execute the query and format the output echo "User Activity Report" > user_activity_report.txt echo "====================" >> user_activity_report.txt mysql -u "$DB_USER" -p"$DB_PASS" -D "$DB_NAME" -e "$REPORT_QUERY" >> user_activity_report.txt # Email the report mail -s "User Activity Report" [email protected] < user_activity_report.txt
In this example, the script generates a report of user activity by counting logins and saves the output to a text file. It then emails this file to a designated recipient, allowing for easy distribution of vital information.
By using the power of Bash scripts for automating database operations, you create robust and reliable processes that not only save time but also ensure the integrity and availability of your data. The combination of scheduling, conditional logic, and SQL execution creates a powerful toolkit for any database administrator looking to streamline their operations.