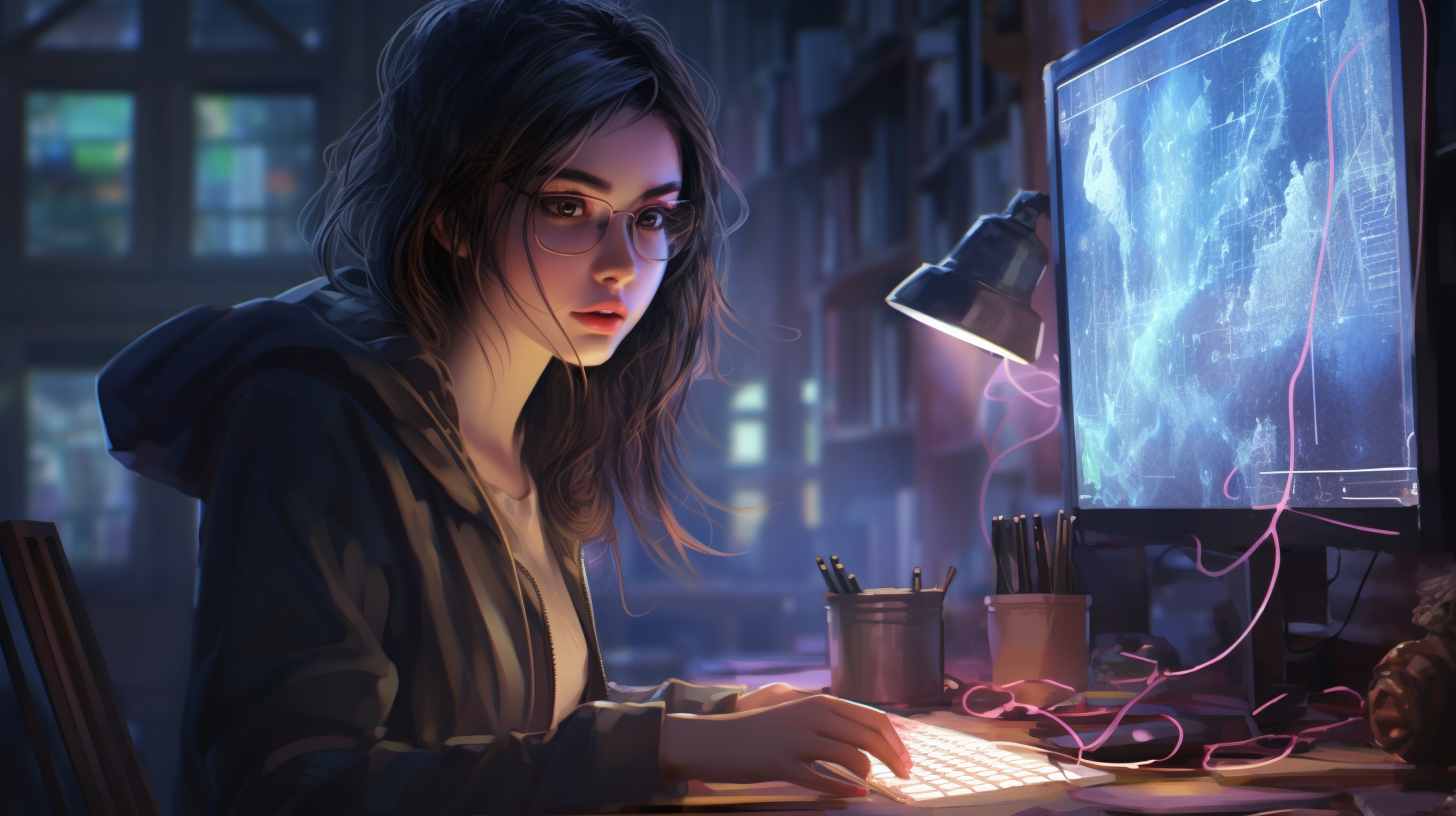
Writing Maintainable Bash Scripts
When embarking on the journey of writing maintainable Bash scripts, the structure of your script is paramount. A well-structured script not only enhances readability but also simplifies debugging and future modifications, so that you can navigate through your code with ease.
Indentation and Spacing
Just as in any programming language, the use of proper indentation and spacing in Bash scripts is essential. Consistent indentation aids in visually separating code blocks, which is vital for clarity. A simple rule of thumb is to use a tab for each level of indentation or four spaces, but be consistent throughout your script.
if [ "$condition" ]; then # Execute block of code echo "Condition met" else # Execute alternative block echo "Condition not met" fi
Use Meaningful Variable Names
Choosing descriptive variable names is an important aspect of script readability. Avoid cryptic abbreviations; instead, opt for names that convey the purpose of the variable. This practice makes it easier for you and others to understand the context of the code at a glance.
# Bad variable names x=5 y=10 # Good variable names number_of_files=5 max_connections=10
Organizing Your Code into Sections
Dividing your script into logical sections improves readability. Each section can serve a distinct purpose, such as initialization, main logic, and cleanup, and can be easily navigated with comments that clearly delineate these areas.
# Initialization initialize_variables() { # Set up variables } # Main logic process_files() { # Process files } # Cleanup cleanup() { # Perform cleanup }
Commenting Your Code
Comments are invaluable for explaining why certain decisions were made in your script. They’re not just for others; they’re for your future self, who might not remember the thought process behind a particular block of code. Keep comments concise and relevant to the surrounding code.
# This function processes input files and outputs results process_input_files() { # Loop through each file for file in "${input_files[@]}"; do # Process each file process_file "$file" done }
Consistent Style and Conventions
Establishing a consistent style across your scripts enhances maintainability. This includes conventions for naming functions, variable casing (e.g., snake_case vs camelCase), and even the placement of braces. Consider adopting a style guide that you and your team can adhere to, ensuring uniformity across all scripts.
# Function names in snake_case function_name() { # Function implementation } # Variable names in UPPER_SNAKE_CASE MAX_CONNECTIONS=100
By following these best practices for structuring your Bash scripts, you set the stage for code that is not only functional but also elegant and maintainable. As with any craft, the initial investment in time and thought pays dividends in the quality of your output and the ease with which you can manage and extend your scripts in the future.
Error Handling and Debugging Techniques
Error handling and debugging are critical components of writing maintainable Bash scripts. When scripts fail, understanding why they fail is essential for both fixing the immediate issue and preventing similar problems in the future. By implementing robust error handling techniques and debugging practices, you can significantly enhance the reliability of your scripts.
Exit Status
In Bash, every executed command returns an exit status. A zero exit status indicates success, while any non-zero exit status indicates an error. Checking the exit status of commands can help catch errors right where they occur. Use the special variable $?
to access the exit status of the last executed command.
some_command if [ $? -ne 0 ]; then echo "Error: some_command failed." exit 1 fi
For better readability, you can wrap this logic in a function that handles error checking for you:
check_command() { "$@" if [ $? -ne 0 ]; then echo "Error: Command '$*' failed." exit 1 fi }
With this function, you can easily check any command:
check_command some_command
Using Trap for Cleanup
Sometimes scripts may exit unexpectedly, leaving behind temporary files or locked resources. The trap
command allows you to specify actions to be taken when a script exits, whether normally or due to an error. That’s useful for cleanup operations.
trap 'cleanup; exit' EXIT
Define your cleanup function to handle necessary tasks:
cleanup() { echo "Cleaning up..." # Remove temporary files or restore state }
Debugging Tools
Bash provides several built-in tools to assist with debugging. One of the most effective methods is to run your script with the -x
option, which prints each command and its arguments as they’re executed. This can be done by adding the following line to your script:
set -x
Conversely, if you want to disable this debugging output at any point, you can use:
set +x
Using Echo for Tracing Execution
In addition to using set -x
, strategically placing echo
statements in your script can help trace the execution flow. That’s especially useful for tracking the value of variables or the entry and exit of functions.
my_function() { echo "Entering my_function with parameters: $*" # Function logic echo "Exiting my_function" }
By employing these error handling and debugging techniques, you not only make your scripts more robust but also easier to troubleshoot. This proactive approach to scripting will save you time and effort in the long run, allowing you to focus on the logic rather than the pitfalls of Bash programming.
Using Functions for Code Reusability
Functions in Bash scripts are not merely a convenience; they’re a powerful tool for enhancing code reusability and maintainability. By encapsulating functionality into discrete units, functions allow you to avoid redundancy and promote a cleaner structure. This section delves into why and how to effectively utilize functions in your Bash scripts.
Defining Functions
Functions in Bash are defined using the function_name() { ... }
syntax. A well-defined function encapsulates code that performs a specific task, enabling you to call that function multiple times throughout your script without rewriting the same code.
greet_user() { local username="$1" echo "Hello, $username!" }
In the example above, the function greet_user
takes a single argument, username
, and prints a greeting. This function can be invoked several times with different usernames, thus eliminating repetitive code.
Parameters and Return Values
Functions can accept parameters, allowing for flexible and dynamic code. You can access parameters within the function using $1
, $2
, and so on, for the first, second, etc., parameters. Although Bash does not support return values in a traditional sense, you can use the echo
command to return output from a function.
calculate_sum() { local sum=$(( $1 + $2 )) echo "$sum" } result=$(calculate_sum 5 10) echo "The sum is: $result"
Here, the calculate_sum
function adds two numbers and echoes the result. By capturing the echoed output into a variable, you can easily utilize the result later in your script.
Function Scope
Understanding scope very important when working with functions. Variables declared within a function using local
are limited to that function’s scope, preventing any unintended interference with variables outside the function.
set_global() { global_var="I am global" } set_local() { local local_var="I am local" } set_global set_local echo "$global_var" # This will print: I am global echo "$local_var" # This will not print anything; it's out of scope
The variable global_var
is accessible outside its function, while local_var
remains confined within set_local
, showcasing the importance of proper variable scoping.
Using Functions for Complex Logic
Functions can encapsulate complex logic, making your scripts easier to read and manage. As your script grows in complexity, breaking down large tasks into smaller, manageable functions can significantly improve maintainability.
process_data() { for file in "$@"; do echo "Processing $file" # Further processing logic... done } data_files=("file1.txt" "file2.txt") process_data "${data_files[@]}"
In this example, the process_data
function processes multiple files passed as arguments. This separation of concerns enhances clarity, as each function handles a specific piece of your overall logic.
Documenting Functions
Each function should be accompanied by comments that explain its purpose, parameters, and return values. This documentation is invaluable not only for others who may read your code but also for your future self. A clear explanation of what a function does can save time and reduce errors when revisiting the code after some time.
# Function to calculate the factorial of a number # Arguments: # $1 - The number to calculate the factorial for # Returns: # The factorial of the number factorial() { local num="$1" if [ "$num" -le 1 ]; then echo 1 else echo $(( num * $(factorial $(( num - 1 )) ) )) fi }
In the above snippet, the factorial
function computes the factorial of a given number. The accompanying comments clarify what the function does and how to use it, fostering better understanding and easier maintenance.
Embracing functions in your Bash scripts not only promotes code reusability but also enhances readability and maintainability. By breaking down complex logic into smaller, manageable pieces, you create a scripting environment this is not only easier to navigate but also more resilient to changes over time.
Documenting Your Code Effectively
Documenting your code is one of the most essential practices when writing maintainable Bash scripts. While it may seem tedious, clear documentation can save considerable time and effort for both yourself and others who may work on your scripts in the future. Proper documentation serves as a guide, helping to navigate through the intricacies of your code and ensuring that the intent and functionality are easily understood.
Inline Comments
Inline comments are crucial for explaining specific lines or blocks of code. They should be used judiciously to clarify complex logic or to annotate decisions that may not be immediately apparent. Strive to answer the “why” behind your code, rather than the “what,” as the latter is often clear from the code itself.
# Loop through each file and process for file in "${input_files[@]}"; do process_file "$file" # Process each file individually done
In this example, the comment explains the purpose of the loop and clarifies what happens within it. Such comments can be tremendously helpful for future readers of your code.
Function Documentation
Documenting functions is equally important. Each function should have a comment block at the beginning that outlines its purpose, expected parameters, and return values. By maintaining a consistent format for this documentation throughout your script, you create a reliable reference that can be invaluable during maintenance or updates.
# Function to fetch data from a URL # Arguments: # $1 - The URL to fetch data from # Returns: # The content retrieved from the URL fetch_data() { local url="$1" curl -s "$url" }
This structured comment block clearly details what the function does, what inputs it requires, and what outputs to expect, promoting a thorough understanding of its usage.
Documentation Tools
Think using tools to automate or enhance your documentation process. Tools such as bashdoc
or shdoc
can help generate documentation from specially formatted comments in your scripts. By adopting such tools, you can ensure that your documentation stays current with the code.
README Files
In addition to inline comments and function documentation, think maintaining a README file alongside your scripts. This file should provide an overview of your script’s functionality, usage instructions, and dependencies. A well-crafted README is especially useful for more extensive projects, serving as an entry point for new users or contributors.
# Sample README content # My Bash Script # # This script processes data files and generates reports. # # Usage: # ./my_script.sh [options] input_file # # Options: # -h, --help Show this help message # -o, --output Specify output file
Version Control Comments
When making changes to your scripts, document the rationale behind significant modifications in your version control system. Utilize commit messages effectively to provide context for your changes. This practice will facilitate future understanding of the evolution of your codebase.
# Example commit message # Fixed bug in input validation; added error handling for empty inputs
By adhering to these documentation practices, you cultivate a more maintainable and understandable codebase. Whether you are working alone or as part of a team, comprehensive documentation serves not just as a roadmap for your current work, but as a legacy for those who will navigate your code in the future.