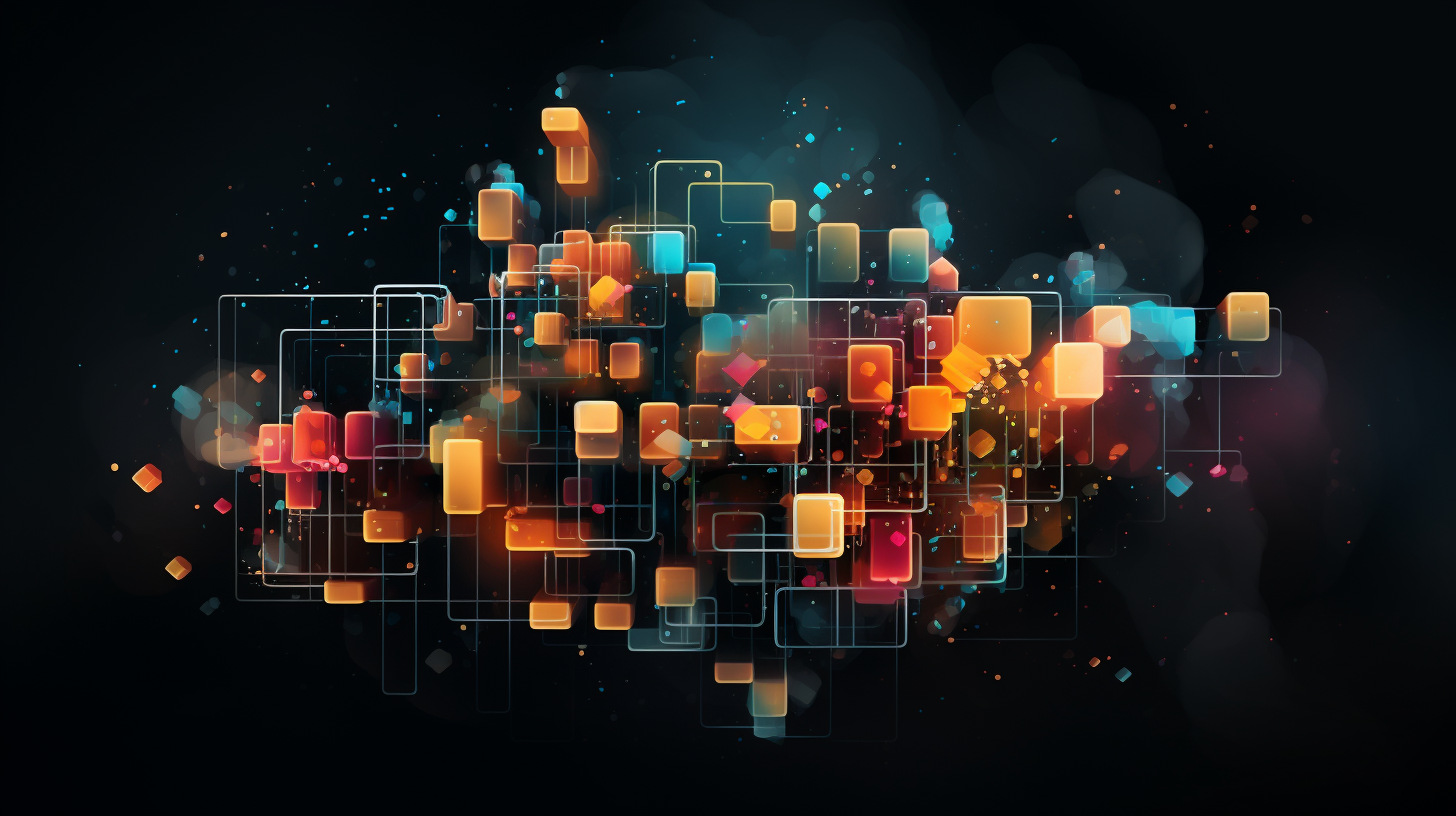
Python and XML: Working with XML Data
XML, or Extensible Markup Language, serves as a powerful tool for data representation and interchange. It provides a structured way to encode documents in a format that is both human-readable and machine-readable. The foundation of XML lies in its use of elements, attributes, and a hierarchical structure.
At its core, an XML document is defined by a set of nested elements, each enclosed within opening and closing tags. The tags describe the data contained within, making the structure of the document self-descriptive. Think the following simple XML snippet:
Tove Jani ReminderDon't forget me this weekend!
In this example, the root element is note, which contains several child elements: to, from, heading, and body. Each element can hold text, and elements can also contain attributes, providing further detail about the data. For instance:
Tove Jani ReminderDon't forget me this weekend!October 1, 2023
In this expanded example, the date element includes an attribute sent that supplies additional information about when the note was sent. This flexibility allows XML to represent complex data structures clearly and concisely.
XML documents must adhere to strict syntax rules to ensure they’re well-formed. These rules include:
- Every opening tag must have a corresponding closing tag.
- Tags must be properly nested.
- Element names are case-sensitive.
- Attribute values must be enclosed in quotes.
A well-formed XML document ensures that parsers can accurately read and process the data without ambiguity. To illustrate the potential pitfalls, the following is an example of a poorly formed XML document:
Tove Jani ReminderDon't forget me this weekend!
This document is invalid because the to tag is not correctly closed. Understanding these structural and syntactical rules is important for anyone looking to work with XML data.
When dealing with XML, it is also important to recognize the idea of namespaces, which help avoid naming conflicts by qualifying element names with a URI. This becomes particularly relevant in applications where multiple XML standards might overlap. For example:
Tove Jani ReminderDon't forget me this weekend!
In this case, the to element is defined within a specific namespace, ensuring clarity in its context and usage. Mastering the XML structure and syntax is not just an academic exercise; it is essential for effective data manipulation and integration in various applications.
Parsing XML in Python with ElementTree
Parsing XML in Python can be accomplished efficiently using the built-in ElementTree module, which provides a simple and intuitive way to navigate the structure of an XML document. This module allows you to read XML data from a file or string and interact with its elements in a simpler manner.
To begin parsing an XML document, you first need to import the ElementTree module and load your XML data. Here’s a basic example that demonstrates how to parse an XML string:
import xml.etree.ElementTree as ET xml_data = '''<note> <to>Tove</to> <from>Jani</from> <heading>Reminder</heading> <body>Don't forget me this weekend!</body> </note>''' # Parse the XML string root = ET.fromstring(xml_data) # Accessing elements to = root.find('to').text from_ = root.find('from').text heading = root.find('heading').text body = root.find('body').text print(f'To: {to}') print(f'From: {from_}') print(f'Heading: {heading}') print(f'Body: {body}')
In this snippet, we define a multi-line string that contains XML data. The `ET.fromstring()` function is then used to parse this string and create an ElementTree object. From there, we can navigate the XML tree by using the `find()` method to retrieve the text content of each element.
For larger XML files, you might prefer to parse the data directly from a file. This can be achieved using the `ET.parse()` method, which allows you to work with files more conveniently. Here’s how you can do it:
# Load XML data from a file tree = ET.parse('note.xml') root = tree.getroot() # Accessing elements to = root.find('to').text from_ = root.find('from').text heading = root.find('heading').text body = root.find('body').text print(f'To: {to}') print(f'From: {from_}') print(f'Heading: {heading}') print(f'Body: {body}')
In this example, we use `ET.parse()` to load the XML document named `note.xml`. The `getroot()` method retrieves the root element, from which we can similarly access child elements.
ElementTree also provides powerful features to iterate through elements. You can loop through elements using the `iter()` method, which allows for more complex data structures. The following example demonstrates how to iterate through all child elements:
for elem in root.iter(): print(f'{elem.tag}: {elem.text}')
This loop will print out each element’s tag and its text, which can be incredibly useful for debugging or processing XML data dynamically.
When working with XML, it’s essential to handle potential errors gracefully. Parsing XML can throw exceptions if the data is not well-formed. The following example shows how to handle such cases:
try: tree = ET.parse('invalid_note.xml') except ET.ParseError as e: print(f'Error parsing XML: {e}')
In this code, we use a try-except block to catch `ParseError` exceptions, allowing us to take corrective action or log the error without crashing the program.
With these foundational techniques, you can effectively work with XML data in Python using the ElementTree module. This flexibility opens doors to processing and manipulating various types of XML data, paving the way for more complex applications and integrations.
Manipulating XML Data Using lxml
When it comes to manipulating XML data in Python, the lxml library stands out due to its performance and ease of use. Unlike the built-in ElementTree module, lxml offers a more extensive set of features that can handle more complex XML structures, including support for XPath and XSLT. This makes it an excellent choice for developers needing to execute more sophisticated XML operations.
To begin using lxml, you’ll need to install it using pip if it is not already available in your environment:
pip install lxml
Once installed, you can start manipulating XML documents. Here’s a basic example that demonstrates how to parse an XML string and modify its elements:
from lxml import etree # Sample XML data xml_data = '''<note> <to>Tove</to> <from>Jani</from> <heading>Reminder</heading> <body>Don't forget me this weekend!</body> </note>''' # Parse the XML string root = etree.fromstring(xml_data) # Modify an element root.find('body').text = "Don't forget me next weekend!" # Add a new element etree.SubElement(root, 'date').text = 'October 1, 2023' # Serialize the modified XML back to string modified_xml = etree.tostring(root, pretty_print=True).decode() print(modified_xml)
In this example, we parse a simple XML string into an lxml Element, modify the text of the element, and add a new element. The `etree.tostring()` method is then used to convert the manipulated XML back into a string format, which can be printed or stored as needed.
lxml’s capabilities extend beyond basic modifications. For example, you can utilize XPath to query elements efficiently. XPath is a powerful language for selecting nodes from an XML document, and lxml makes it easy to apply. Here’s how you can use XPath to retrieve elements:
# Using XPath to find elements to_node = root.xpath('/note/to') print(f'To: {to_node[0].text}') # Access the text of the selected node # Finding all elements with a specific tag all_elements = root.xpath('//*') for elem in all_elements: print(f'{elem.tag}: {elem.text}') # Print all elements with their text
In this snippet, we use XPath expressions to locate the element and print its text. The second XPath expression retrieves all elements in the document, demonstrating the flexibility of XPath for querying XML structures.
Another powerful feature of lxml is its ability to transform XML documents using XSLT (Extensible Stylesheet Language Transformations). XSLT allows you to convert XML data into different formats or even into other XML structures. This capability is particularly useful for generating reports or web pages from XML data. Here’s a simple example of how to apply XSLT with lxml:
xslt_data = '''<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"> <xsl:template match="/note"> <message> <to><xsl:value-of select="to"/></to> <from><xsl:value-of select="from"/></from> <body><xsl:value-of select="body"/></body> </message> </xsl:template> </xsl:stylesheet>''' # Load the XSLT xslt_root = etree.fromstring(xslt_data) transform = etree.XSLT(xslt_root) # Apply the transformation result = transform(root) print(str(result))
In this example, we define a simple XSLT stylesheet that transforms the original note into a message format. After loading the XSLT, we apply it to the parsed XML, and the result is printed out as a new XML structure.
Overall, lxml provides an incredibly powerful framework for XML manipulation in Python, offering advanced capabilities for parsing, modifying, querying, and transforming XML data. Its rich feature set makes it perfect for developers handling complex XML documents and needing efficient processing capabilities.
Serializing and Writing XML Files in Python
import xml.etree.ElementTree as ET # Creating a new XML document note = ET.Element("note") to = ET.SubElement(note, "to") from_ = ET.SubElement(note, "from") heading = ET.SubElement(note, "heading") body = ET.SubElement(note, "body") # Assigning text to the elements to.text = "Tove" from_.text = "Jani" heading.text = "Reminder" body.text = "Don't forget me this weekend!" # Serializing the XML to a string xml_string = ET.tostring(note, encoding='unicode', method='xml') print(xml_string)
In this example, we’re creating a new XML document from scratch using the `ElementTree` module. We define the root element as `note` and add child elements using `SubElement`. After assigning values to each element, we serialize the entire structure back into a string format using `ET.tostring()`, specifying the encoding as ‘unicode’ to ensure the output is human-readable.
When it comes to writing XML data to a file, the `ElementTree` module provides a simpler method. The `write()` function allows you to save the XML structure directly to a file. Here’s how to do that:
# Writing XML to a file tree = ET.ElementTree(note) with open("note.xml", "wb") as xml_file: tree.write(xml_file, encoding='utf-8', xml_declaration=True)
In this snippet, we create an `ElementTree` object from our note element and use the `write()` method to output the XML data to a file called `note.xml`. The `encoding` parameter is set to ‘utf-8’, and `xml_declaration=True` adds the XML declaration at the top of the file, ensuring proper encoding specifications.
For more advanced serialization, including pretty-printing the XML, you may prefer to use the `lxml` library, which provides more options for formatting the output. Here’s how to write and format an XML file with `lxml`:
from lxml import etree # Creating the XML structure with lxml note = etree.Element("note") to = etree.SubElement(note, "to") from_ = etree.SubElement(note, "from") heading = etree.SubElement(note, "heading") body = etree.SubElement(note, "body") # Assigning text to the elements to.text = "Tove" from_.text = "Jani" heading.text = "Reminder" body.text = "Don't forget me this weekend!" # Writing the XML to a file with pretty print with open("note_lxml.xml", "wb") as xml_file: xml_file.write(etree.tostring(note, pretty_print=True, encoding='utf-8', xml_declaration=True))
Here, we utilize the `lxml` library to create an XML structure and write it to a file named `note_lxml.xml`. By setting `pretty_print=True` in the `tostring()` method, the output is formatted for readability, making it easier to inspect the resulting XML structure.
These techniques for serializing and saving XML data in Python allow for flexibility depending on your needs. Whether you are creating new XML documents or saving existing data structures, Python’s libraries make the process efficient and intuitive.