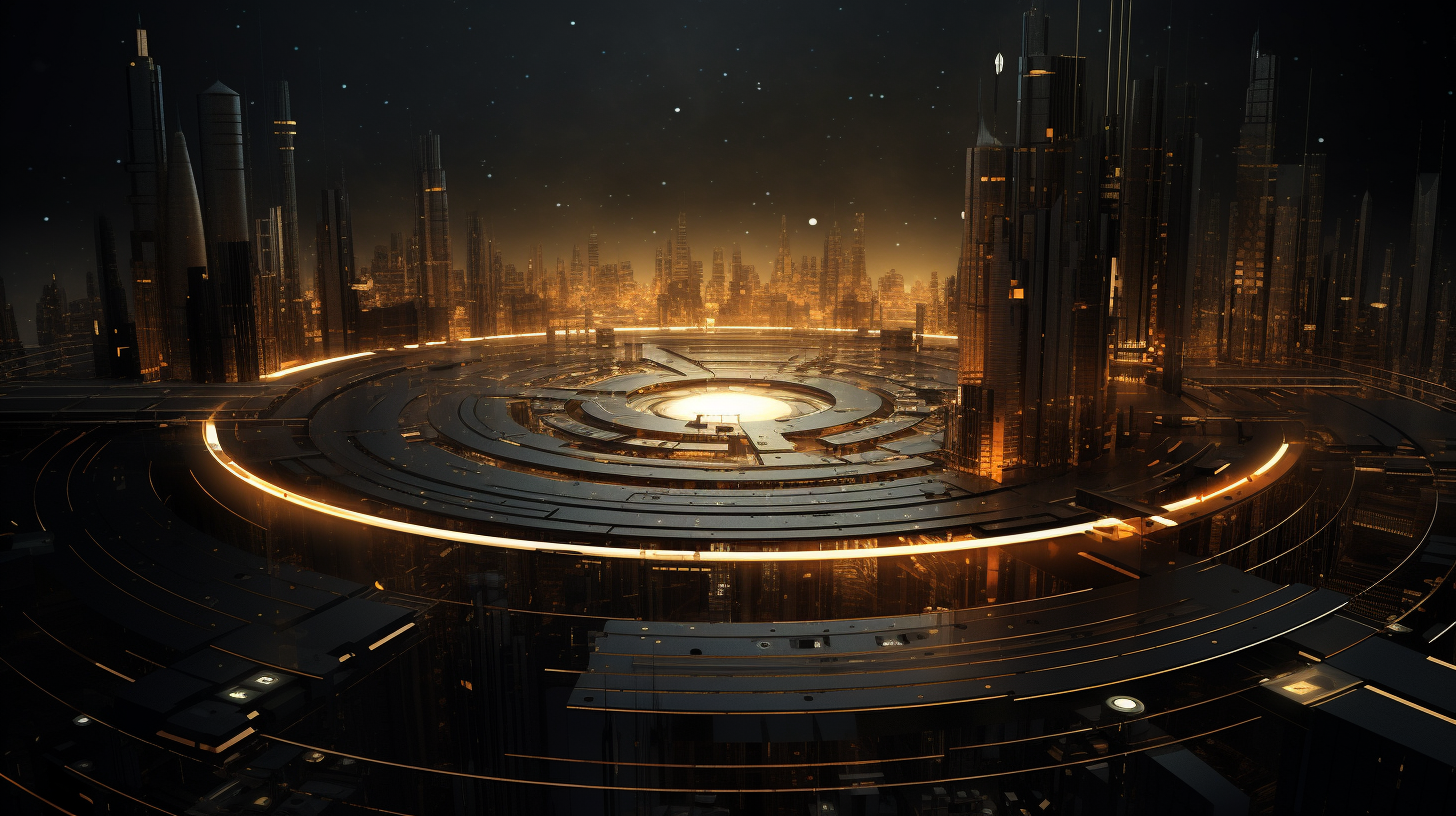
Swift and Vision Framework
The Vision framework is a powerful tool in Swift that allows developers to perform a variety of image analysis tasks with ease. Whether you are working on facial recognition, object detection, or text detection, the Vision framework provides a robust set of APIs to handle these tasks efficiently. Understanding how to leverage this framework is key to building applications that require advanced image processing capabilities.
At its core, the Vision framework utilizes a series of requests to analyze images and extract useful information. Each request is designed for a specific task, such as recognizing faces, identifying objects, or detecting text in images. The beauty of this framework lies in its high-level abstractions, which allow developers to focus on implementing features rather than getting bogged down in the complexities of image processing algorithms.
The Vision framework is built on top of Core Image and integrates seamlessly with other Apple technologies, such as Core ML, facilitating the development of AI-powered applications. When you create a vision request, you can specify the input image and the type of analysis you want to perform. The results are then provided in a structured format, making it easier to work with the data.
To get started with the Vision framework in Swift, you’ll typically follow a pattern that involves creating a VNImageRequestHandler
to process your input image and then executing one or more VNRequest
objects. Below is a Swift code example demonstrating how to detect faces in an image using the Vision framework:
import Vision import UIKit func detectFaces(in image: UIImage) { guard let cgImage = image.cgImage else { return } let request = VNDetectFaceRectanglesRequest { (request, error) in if let results = request.results as? [VNFaceObservation] { for face in results { print("Found face at: (face.boundingBox)") } } } let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([request]) } catch { print("Failed to perform face detection: (error)") } }
In this example, we import the Vision and UIKit frameworks, define a function that takes a UIImage
as input, and create a face detection request. The completion handler of the request processes the results, allowing us to capture the bounding boxes of detected faces.
An important aspect of working with the Vision framework is understanding the coordinate system it uses. The bounding boxes returned in the VNFaceObservation
are normalized to the range [0, 1], meaning that you’ll need to convert these coordinates to match your image’s dimensions if you want to draw them or perform further processing.
As you dive deeper into the Vision framework, you’ll discover a wealth of features beyond just face detection. With a solid understanding of how to set up your requests and handle results, you can unlock the full potential of image analysis in your Swift applications.
Setting Up Your Xcode Project for Vision
To set up your Xcode project for using the Vision framework, you need to follow a few specific steps that ensure your environment is ready for image analysis tasks. This involves creating a new project or modifying an existing one and integrating necessary frameworks.
First, launch Xcode and create a new project. For most applications using the Vision framework, you would typically choose the “iOS App” template. Ensure that you select Swift as your programming language. Once your project is created, the next step is to add the Vision framework to your project.
To add the Vision framework, navigate to the project settings by clicking on your project in the Project Navigator. Then, select your target and go to the “General” tab. Scroll down to the “Frameworks, Libraries, and Embedded Content” section, and click the “+” button to add a new framework. From the list, search for “Vision” and add it to your project. This ensures that you can import and utilize the APIs provided by the Vision framework.
Next, if you plan to work with images from the device’s camera or photo library, you will need to configure the necessary permissions in your project. Open the Info.plist
file and add a new entry for NSCameraUsageDescription
to request permission to access the camera. Similarly, add NSPhotoLibraryUsageDescription
if your app will be accessing the photo library. This way, your application can seamlessly interact with the user’s media.
After setting up the framework and permissions, it’s important to structure your project for image handling. Create a new Swift file where you will implement your image processing logic. Import the Vision framework at the top of your file:
import Vision
Additionally, when dealing with images, you might want to create helper functions for loading images or handling the results of your Vision requests. Here’s a simple example of how you might load an image from the photo library:
import UIKit import Photos func loadImageFromLibrary(completion: @escaping (UIImage?) -> Void) { let status = PHPhotoLibrary.authorizationStatus() if status == .authorized { let imagePicker = UIImagePickerController() imagePicker.sourceType = .photoLibrary imagePicker.delegate = self // Ensure your class conforms to UIImagePickerControllerDelegate and UINavigationControllerDelegate // Present imagePicker here } else { PHPhotoLibrary.requestAuthorization { newStatus in if newStatus == .authorized { // Retry loading the image } } } }
When you’re ready to implement Vision requests, remember to think threading. Vision tasks can be computationally intensive, and it’s crucial to avoid blocking the main thread. You can execute your requests on a background thread using GCD or operation queues. Here’s an example of how to perform a Vision request asynchronously:
func performVisionRequest(image: UIImage) { DispatchQueue.global(qos: .userInitiated).async { detectFaces(in: image) // Your previously defined function } }
By following these steps, you will have your Xcode project set up and ready for integrating the Vision framework. With the right configuration in place, you can focus on building powerful image analysis capabilities into your application.
Core Features of the Vision Framework
The Vision framework boasts several core features that empower developers to execute complex image analysis tasks with ease. At the heart of this framework are the requests, which are high-level abstractions that encapsulate specific functionalities. Each request can be tailored for tasks like object detection, text recognition, and image registration, allowing developers to efficiently extract valuable information from images.
One of the key strengths of the Vision framework is its ability to utilize a variety of request types to perform multiple analyses on a single image. For instance, you can run both face detection and text recognition requests on the same input image, collecting diverse insights with minimal effort. Below is an example demonstrating how to perform both face detection and text recognition using the Vision framework:
import Vision import UIKit func analyzeImage(_ image: UIImage) { guard let cgImage = image.cgImage else { return } // Create a face detection request let faceDetectionRequest = VNDetectFaceRectanglesRequest { (request, error) in if let results = request.results as? [VNFaceObservation] { for face in results { print("Found face at: (face.boundingBox)") } } } // Create a text detection request let textDetectionRequest = VNRecognizeTextRequest { (request, error) in if let results = request.results as? [VNRecognizedTextObservation] { for textObservation in results { if let topCandidate = textObservation.topCandidates(1).first { print("Detected text: (topCandidate.string)") } } } } let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([faceDetectionRequest, textDetectionRequest]) } catch { print("Failed to perform requests: (error)") } }
In the example above, two requests are created: one for detecting faces and another for recognizing text. When the requests are executed, they process the input image to extract and print the bounding boxes for detected faces and any recognized text.
Another notable feature of the Vision framework is its seamless integration with Core ML. This allows developers to leverage pre-trained machine learning models for more advanced image analysis tasks. For instance, you can utilize a custom Core ML model to perform object classification or image segmentation in conjunction with Vision requests. By chaining these capabilities, you can create sophisticated applications that react intelligently to visual input.
Furthermore, the Vision framework provides support for image registration, enabling you to align images taken at different times or from different perspectives. This is particularly useful in augmented reality (AR) applications, where overlaying digital content accurately onto the real world is essential. Using request configurations, you can perform transformations and adjustments to ensure that images align as expected.
Overall, the core features of the Vision framework not only simplify the process of implementing image analysis but also enhance the quality and functionality of applications. By understanding and using these features, developers can create rich and engaging user experiences that capitalize on the power of visual data.
Image Analysis Techniques with Vision
Image analysis techniques in the Vision framework are designed to be both powerful and accessible. With a variety of built-in requests for different tasks, developers can easily integrate complex image analysis capabilities into their applications. Let’s explore several key techniques provided by the Vision framework, such as object detection, text recognition, barcode detection, and more.
One of the most widely used techniques is object detection. The Vision framework provides the VNCoreMLModel
class, which allows you to utilize custom Core ML models for detecting objects in images. Here’s an example of how to set up and execute an object detection request using a Core ML model:
import Vision import CoreML import UIKit func detectObjects(in image: UIImage) { guard let cgImage = image.cgImage else { return } // Load your Core ML model guard let model = try? VNCoreMLModel(for: YourObjectDetectionModel().model) else { print("Could not load model") return } // Create a request for object detection let request = VNCoreMLRequest(model: model) { (request, error) in if let results = request.results as? [VNRecognizedObjectObservation] { for observation in results { let boundingBox = observation.boundingBox print("Detected object at: (boundingBox)") } } } let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([request]) } catch { print("Failed to perform object detection: (error)") } }
In this example, we first load a custom Core ML model and then create a VNCoreMLRequest
for object detection. The completion handler processes the results, providing bounding boxes for each detected object.
Another powerful feature is text recognition. This allows you to extract text from images using the VNRecognizeTextRequest
. Here’s an example of using this request:
import Vision import UIKit func recognizeText(in image: UIImage) { guard let cgImage = image.cgImage else { return } let request = VNRecognizeTextRequest { (request, error) in if let results = request.results as? [VNRecognizedTextObservation] { for observation in results { if let topCandidate = observation.topCandidates(1).first { print("Recognized text: (topCandidate.string)") } } } } request.recognitionLevel = .accurate // Set recognition level let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([request]) } catch { print("Failed to perform text recognition: (error)") } }
In this snippet, the VNRecognizeTextRequest
is created to recognize text from the input image. The recognition level can be set to either .accurate
or .fast
depending on the requirements for speed versus accuracy.
Additionally, the Vision framework excels in barcode detection. This functionality is important for applications that require scanning barcodes or QR codes. Below is an example of how to implement barcode detection:
import Vision import UIKit func detectBarcodes(in image: UIImage) { guard let cgImage = image.cgImage else { return } let request = VNDetectBarcodesRequest { (request, error) in if let results = request.results as? [VNBarcodeObservation] { for barcode in results { print("Detected barcode: (barcode.payloadStringValue ?? "Unknown")") } } } let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([request]) } catch { print("Failed to perform barcode detection: (error)") } }
This example demonstrates how to create a VNDetectBarcodesRequest
, which processes the image for any barcode types. The results include the detected barcode’s payload, which can be used for further processing, such as linking to URLs or retrieving product information.
Each of these techniques highlights the capabilities of the Vision framework. By combining object detection, text recognition, and barcode detection, developers can craft a wide array of applications that intelligently analyze and respond to visual data.
Moreover, using these requests in conjunction with Core ML models can elevate your applications even further, enabling advanced functionalities such as image classification and custom detection tasks tailored to specific needs.
Integrating Vision with Core ML
Integrating the Vision framework with Core ML allows developers to exploit the strengths of both technologies, creating applications that can perform sophisticated image analysis while using the power of machine learning. This integration is particularly valuable for tasks such as image classification, object detection, and scene understanding, where the combination of image processing and machine learning can yield impressive results.
To effectively integrate Vision with Core ML, you start by creating or obtaining a trained Core ML model suitable for the tasks you want to accomplish. Once you have your Core ML model ready, you can use the VNCoreMLModel class to wrap it, which allows the Vision framework to utilize the model’s capabilities for image analysis.
Here’s an example of how to set up a Core ML model for object detection using the Vision framework:
import Vision import CoreML import UIKit func detectObjectsWithCoreML(in image: UIImage) { guard let cgImage = image.cgImage else { return } // Load your Core ML model guard let model = try? VNCoreMLModel(for: YourObjectDetectionModel().model) else { print("Could not load model") return } // Create a request for object detection let request = VNCoreMLRequest(model: model) { (request, error) in if let results = request.results as? [VNRecognizedObjectObservation] { for observation in results { let boundingBox = observation.boundingBox print("Detected object at: (boundingBox)") } } } let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([request]) } catch { print("Failed to perform object detection: (error)") } }
In this example, we start by ensuring we have a valid CGImage from the UIImage. Then, we load our Core ML model and create a VNCoreMLRequest
to process the input image. The results can be accessed through the completion handler, allowing us to utilize the detected object’s bounding boxes as needed.
To enhance the performance and accuracy of the analysis, you should think adjusting the parameters and preprocessing the input images as necessary. For example, resizing images to a standard size that matches the model’s input can lead to better predictions, especially with models trained on fixed-size images.
In addition to object detection, integrating Vision with Core ML can also facilitate tasks like image segmentation, where the goal is to classify each pixel in an image. This can be particularly useful for applications in medical imaging, augmented reality, and autonomous driving, where understanding the scene at a granular level is essential.
Here’s how you might implement image segmentation using a Core ML model:
import Vision import CoreML import UIKit func segmentImageWithCoreML(in image: UIImage) { guard let cgImage = image.cgImage else { return } // Load your segmentation model guard let model = try? VNCoreMLModel(for: YourSegmentationModel().model) else { print("Could not load model") return } // Create a request for image segmentation let request = VNCoreMLRequest(model: model) { (request, error) in if let results = request.results as? [VNPixelBufferObservation] { for observation in results { // Process the segmented output print("Segmented output: (observation)") } } } let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([request]) } catch { print("Failed to perform segmentation: (error)") } }
In this example, we utilize a segmentation model with a similar approach to the object detection task. The key difference lies in the expectation of the output, which in this case is a pixel buffer that contains segmentation results for the entire image.
When integrating Vision with Core ML, it is important to keep in mind the overall architecture of your application. Make sure to handle model loading efficiently, avoid reloading the model with every request, and manage the memory of large images or buffers judiciously. These considerations will ensure that your application remains responsive while providing high-performance image analysis capabilities.
Best Practices for Performance Optimization
When working with the Vision framework in Swift, performance optimization becomes a paramount concern, especially as image analysis tasks can be computationally intensive. To ensure that your applications remain responsive while handling potentially large datasets, think the following best practices for performance optimization.
1. Use Background Processing
One of the most effective ways to optimize performance is to run Vision requests on a background thread. This prevents blocking the main thread, allowing your UI to remain responsive while processing images. Utilize Grand Central Dispatch (GCD) or operation queues for this purpose. Here’s a simpler implementation using GCD:
func performVisionRequestInBackground(image: UIImage) { DispatchQueue.global(qos: .userInitiated).async { detectFaces(in: image) // Your existing face detection function } }
This ensures that the heavy lifting of image processing is handled off the main thread, improving user experience.
2. Batch Processing
If your application needs to analyze multiple images, consider batching requests instead of processing them one by one. The Vision framework allows multiple requests to be executed at once, which can significantly improve performance. Here’s an example:
func performBatchRequests(images: [UIImage]) { for image in images { guard let cgImage = image.cgImage else { continue } let faceRequest = VNDetectFaceRectanglesRequest() let textRequest = VNRecognizeTextRequest() let handler = VNImageRequestHandler(cgImage: cgImage, options: [:]) do { try handler.perform([faceRequest, textRequest]) } catch { print("Failed to perform requests: (error)") } } }
By processing each image with multiple requests at once, you can leverage the full power of the Vision framework, thus reducing the overall time taken for analysis.
3. Image Resizing
Working with high-resolution images can drastically slow down processing times. If possible, resize images to a resolution that’s optimal for your models while maintaining sufficient quality for analysis. The Vision framework operates efficiently on smaller images, and resizing can help improve performance without sacrificing accuracy. Here’s how you can resize an image:
func resizeImage(image: UIImage, targetSize: CGSize) -> UIImage { let size = image.size let widthRatio = targetSize.width / size.width let heightRatio = targetSize.height / size.height // Determine the scale factor to maintain aspect ratio let newSize: CGSize if(widthRatio > heightRatio) { newSize = CGSize(width: size.width * heightRatio, height: size.height * heightRatio) } else { newSize = CGSize(width: size.width * widthRatio, height: size.height * widthRatio) } // Create a graphics context to perform the resizing UIGraphicsBeginImageContextWithOptions(newSize, false, 1.0) image.draw(in: CGRect(origin: .zero, size: newSize)) let newImage = UIGraphicsGetImageFromCurrentImageContext() UIGraphicsEndImageContext() return newImage! }
This function takes an image and a target size, maintaining the aspect ratio while resizing, which can help improve processing speed.
4. Optimize Vision Requests
When creating requests, think their configurations and set properties that can affect performance. For example, when using text recognition, you can choose between different recognition levels. The `.fast` option is less accurate but significantly faster than the `.accurate` option. Here’s how to set that:
let textRequest = VNRecognizeTextRequest { (request, error) in // Handle results here } textRequest.recognitionLevel = .fast // Use fast recognition for better performance
By selecting the appropriate configurations, you can strike a balance between speed and accuracy based on your application’s needs.
5. Efficiently Manage Model Loading
Loading Core ML models can be resource-intensive. Ensure that you load your models only once and reuse them across multiple requests instead of reloading them each time. Maintain a reference to your model as a class property or singleton instance:
class ModelManager { static let shared = ModelManager() let objectDetectionModel: VNCoreMLModel? private init() { objectDetectionModel = try? VNCoreMLModel(for: YourObjectDetectionModel().model) } }
Using a shared instance ensures that the model is loaded once and reused, minimizing overhead and improving performance.
6. Use Image Cache
If your application frequently processes the same images, think implementing an image caching strategy. Caching allows you to store processed outputs (such as detections) and reduce redundant computations. You can use a simple dictionary to cache results based on image identifiers or hash values.
var imageCache: [String: [VNFaceObservation]] = [:] func detectFacesWithCache(in image: UIImage, identifier: String) { if let cachedResults = imageCache[identifier] { print("Using cached results for image: (identifier)") // Use cachedResults return } // Perform face detection and cache results // ... imageCache[identifier] = detectedResults }
This way, you can save time and resources by avoiding unnecessary reprocessing of the same images.
By implementing these best practices, you can significantly enhance the performance of your application while using the Vision framework for sophisticated image analysis tasks. Balancing responsiveness with computational needs is the key to delivering a seamless user experience.