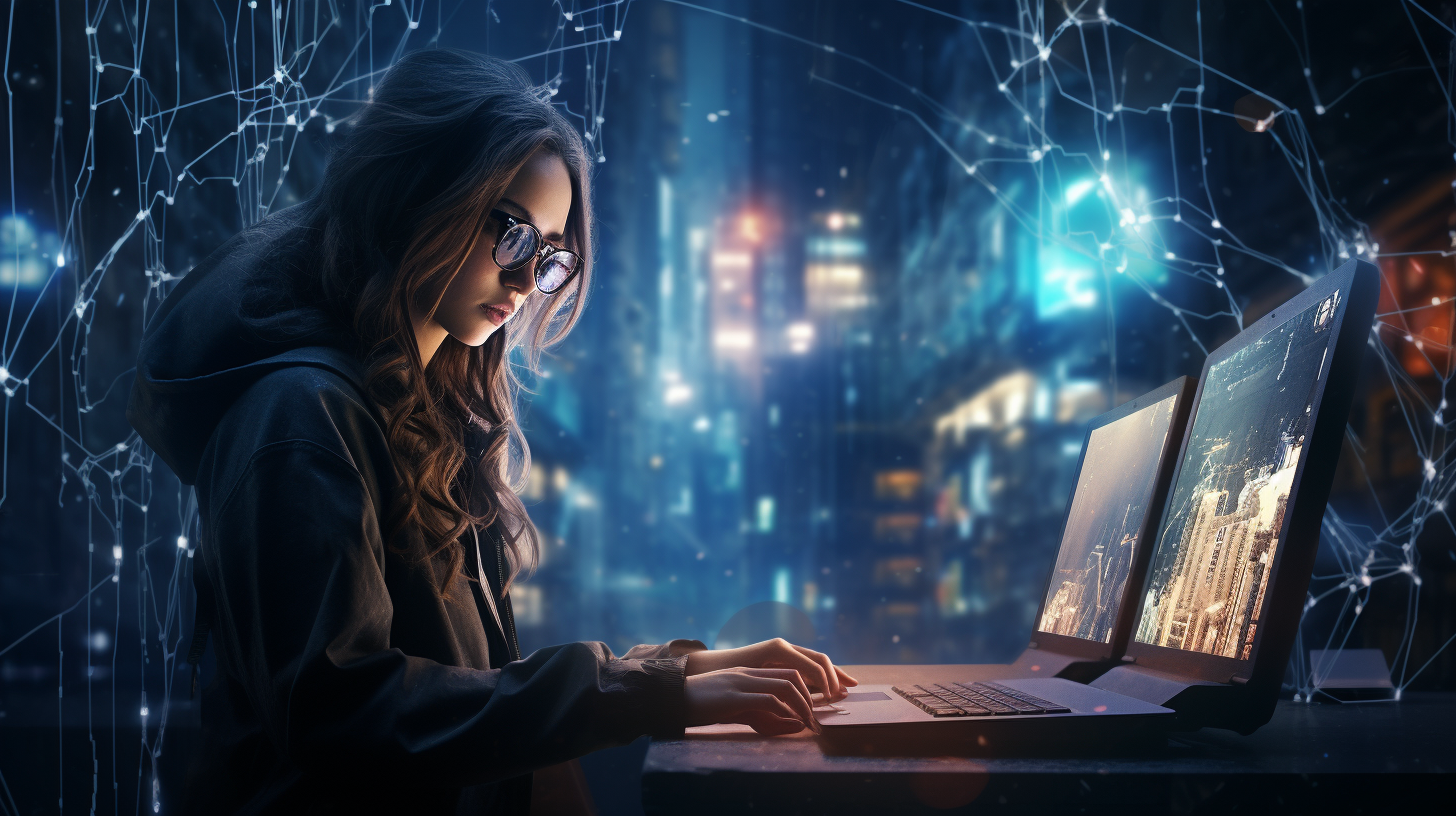
Java Regular Expressions: Pattern Matching
Java regular expressions, often referred to as regex, provide a powerful mechanism for string manipulation and pattern matching. They’re implemented in Java through the java.util.regex package, which includes the classes Pattern and Matcher. This functionality allows developers to perform complex string operations, validating input, searching, and replacing substrings based on defined patterns.
The core concept behind regular expressions is pattern matching. A regex pattern is essentially a sequence of characters that defines a search criterion. This can range from simple matches like finding a specific word, to complex patterns that can validate the structure of an email address or a phone number.
To utilize regular expressions in Java, you start by compiling a regex string into a Pattern object. This is achieved using the Pattern.compile()
method. Once a pattern is compiled, you can create a Matcher object that can be used to perform various operations such as searching, matching, and replacing characters in strings.
import java.util.regex.Pattern; import java.util.regex.Matcher; public class RegexExample { public static void main(String[] args) { String text = "The quick brown fox jumps over the lazy dog."; String regex = "quick"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(text); if (matcher.find()) { System.out.println("Found the word: " + matcher.group()); } else { System.out.println("Word not found."); } } }
In this example, we compile a regex that looks for the word “quick” within a given text. The find()
method of the Matcher class checks if the pattern exists in the text, and if it does, we can retrieve the matched substring using matcher.group()
.
Moreover, regular expressions can include special characters that allow for more sophisticated matches. For instance, the dot (.)
character matches any single character, while the asterisk (*)
matches zero or more occurrences of the preceding element. This flexibility gives developers immense power when it comes to text processing.
public class RegexDemo { public static void main(String[] args) { String text = "abc123xyz"; String regex = "[a-z]+\d+"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(text); while (matcher.find()) { System.out.println("Matched: " + matcher.group()); } } }
In this snippet, we search for a pattern that matches one or more lowercase letters followed by one or more digits. The use of brackets and quantifiers in the regex allows for a diverse range of matches, showcasing the expressiveness of regex in Java.
In summary, understanding Java regular expressions opens up a realm of possibilities for handling string data efficiently. By using the Pattern and Matcher classes, developers can create intricate patterns that facilitate precise searching and manipulation of strings, making regex an invaluable tool in Java programming.
Syntax and Components of Regular Expressions
The syntax of Java regular expressions is a blend of simple characters and special symbols that allow for intricate pattern definitions. Each component of a regex plays a unique role in defining how patterns are matched. Understanding these components especially important for effective pattern matching.
At its core, a regular expression consists of literal characters and metacharacters. Literal characters represent themselves and match exactly the characters in the target string. For example, the regex java
matches the sequence “java” in a string. In contrast, metacharacters have special meanings and behaviors that extend the matching capabilities of regex.
Some of the most commonly used metacharacters include:
- . – Matches any single character except a newline.
- * – Matches zero or more occurrences of the preceding element.
- + – Matches one or more occurrences of the preceding element.
- ? – Matches zero or one occurrence of the preceding element, making it optional.
- ^ – Asserts the start of a string.
- $ – Asserts the end of a string.
- [] – Defines a character class, matching any single character within the brackets.
- \ – Escapes a metacharacter, allowing it to be treated as a literal character.
Let’s think a practical example that illustrates the use of these components. In the following code snippet, we’ll match strings that contain a sequence of alphanumeric characters, which can be useful for validating usernames:
import java.util.regex.Pattern; import java.util.regex.Matcher; public class UsernameValidator { public static void main(String[] args) { String usernames[] = {"user123", "user_123", "123user", "user-123", "user@123"}; String regex = "^[a-zA-Z0-9]+$"; Pattern pattern = Pattern.compile(regex); for (String username : usernames) { Matcher matcher = pattern.matcher(username); if (matcher.matches()) { System.out.println(username + " is a valid username."); } else { System.out.println(username + " is NOT a valid username."); } } } }
In this example, the regex ^[a-zA-Z0-9]+$
defines a valid username as one that starts and ends with alphanumeric characters and contains only letters and numbers in between. The ^
and $
anchors ensure that the entire string meets the criteria. The +
quantifier specifies that there must be at least one character present.
Java also supports grouping and backreferences, which are powerful features for more complex pattern matching. Grouping can be achieved using parentheses ()
, so that you can apply quantifiers to entire subexpressions. Backreferences allow you to refer to previously captured groups within the same regex.
For instance, ponder the following example where we want to find repeated words:
public class RepeatedWords { public static void main(String[] args) { String text = "This is is a test test string."; String regex = "\b(\w+)\s+\1\b"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(text); while (matcher.find()) { System.out.println("Repeated word: " + matcher.group()); } } }
Here, the regex \b(\w+)\s+\1\b
identifies sequences of two consecutive identical words. The \b
asserts word boundaries, (\w+)
captures a word, \s+
matches one or more whitespace characters, and \1
refers back to the first capturing group, ensuring that the same word is repeated.
As you delve deeper into regex, you’ll find that mastering its syntax and components allows for more sophisticated pattern definitions. This proficiency in using Java regular expressions can significantly enhance your string manipulation capabilities, making it a vital skill for any Java developer.
Common Use Cases for Pattern Matching
Common use cases for Java regular expressions span a variety of applications, reflecting the versatility of regex in handling string data. From validating user inputs to extracting specific data from text, regex serves as an essential tool in a developer’s toolkit. Below are some prevalent scenarios where pattern matching shines.
Email Validation: One of the most common uses of regular expressions is validating email addresses. Email formats can be quite complex; however, a well-defined regex pattern can enforce standards effectively. Below is a code snippet that demonstrates how to validate email addresses using regex:
import java.util.regex.Pattern; import java.util.regex.Matcher; public class EmailValidator { public static void main(String[] args) { String[] emails = {"[email protected]", "invalid-email", "[email protected]", "@missingusername.com"}; String regex = "^[\w-\.]+@([\w-]+\.)+[a-zA-Z]{2,6}$"; Pattern pattern = Pattern.compile(regex); for (String email : emails) { Matcher matcher = pattern.matcher(email); if (matcher.matches()) { System.out.println(email + " is a valid email address."); } else { System.out.println(email + " is NOT a valid email address."); } } } }
In this example, the regex ^[\w-\.]+@([\w-]+\.)+[a-zA-Z]{2,6}$ checks for a valid email pattern by ensuring it has a proper structure: a local part, an ‘@’ symbol, and a domain which includes a top-level domain (TLD). The use of character classes and quantifiers ensures that the email format adheres to common standards.
Data Extraction: Regex is also extensively used for extracting specific pieces of data from strings. For instance, when dealing with log files or structured text, you might want to extract timestamps or specific identifiers. Below is an example demonstrating how to extract dates from a given text:
import java.util.regex.Pattern; import java.util.regex.Matcher; public class DateExtractor { public static void main(String[] args) { String text = "Logs: 2023-01-01 Event started, 2023-01-02 Event ended."; String regex = "\b(\d{4}-\d{2}-\d{2})\b"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(text); while (matcher.find()) { System.out.println("Extracted date: " + matcher.group()); } } }
This code uses the regex \b(\d{4}-\d{2}-\d{2})\b to find dates in the format YYYY-MM-DD. The \b ensures word boundaries so that only complete date strings are matched.
Text Replacement: Regular expressions are not limited to searching and validating; they are also powerful for replacing substrings. For instance, if you need to censor certain words in a piece of text, regex can accomplish this elegantly:
import java.util.regex.Pattern; import java.util.regex.Matcher; public class CensorWords { public static void main(String[] args) { String text = "This is a bad word example and some bad phrases."; String regex = "\bbad\b"; String replacement = "****"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(text); String result = matcher.replaceAll(replacement); System.out.println(result); } }
In the above example, we use the regex \bbad\b to find the word “bad” and replace it with “****”. The replaceAll() method allows for replacing all instances of the matched substring efficiently.
Splitting Strings: Regex can also facilitate splitting strings based on complex delimiters. For example, you might want to split a string based on one or more whitespace characters:
import java.util.regex.Pattern; public class SplitExample { public static void main(String[] args) { String text = "Split this string by spaces."; String regex = "\s+"; String[] parts = text.split(regex); for (String part : parts) { System.out.println(part); } } }
Here, the regex \s+ is used to match one or more whitespace characters, demonstrating how regex allows for flexible string manipulation.
These use cases exemplify just a fraction of what can be accomplished with Java regular expressions. As you continue to work with regex, you’ll uncover even more applications, enhancing data validation, extraction, and manipulation capabilities within your Java applications.
Best Practices for Using Regular Expressions in Java
When it comes to using regular expressions in Java, adhering to best practices can significantly improve the efficiency, readability, and maintainability of your code. Regex can be a double-edged sword; while it offers powerful capabilities for string manipulation, it can also lead to complex and hard-to-read patterns if not used judiciously. Below are some best practices to ponder when working with Java regular expressions.
1. Compile Patterns Once
Compiling a regex pattern can be an expensive operation, particularly if it’s done repeatedly within a loop. Instead of compiling the pattern every time you use it, compile it once and reuse the resulting Pattern
object. This can lead to significant performance improvements, especially in scenarios where the same pattern is applied to multiple strings.
import java.util.regex.Pattern; import java.util.regex.Matcher; public class ReusablePattern { public static void main(String[] args) { String regex = "\d+"; Pattern pattern = Pattern.compile(regex); String[] inputs = {"File 1: 100", "File 2: 200", "File 3: 300"}; for (String input : inputs) { Matcher matcher = pattern.matcher(input); while (matcher.find()) { System.out.println("Found number: " + matcher.group()); } } } }
2. Use Verbose Mode for Complex Patterns
For complex regex patterns, think using verbose mode (also known as extended mode). This allows you to write regex patterns that are easier to read by ignoring whitespace and allowing comments within the pattern itself. Although Java doesn’t natively support verbose mode like some other languages, you can achieve a similar effect by breaking your regex into smaller components and concatenating them with the |
operator.
import java.util.regex.Pattern; public class VerbosePattern { public static void main(String[] args) { String regex = "((1[89]|[2-9]\d)\d{2})" + // Match years from 1800 to 2999 "|(\d{1,2}/\d{1,2}/(\d{2}|\d{4}))"; // Match dates in MM/DD/YYYY or MM/DD/YY Pattern pattern = Pattern.compile(regex); System.out.println("Regex for dates and years: " + pattern.pattern()); } }
3. Limit the Scope of Patterns
When possible, restrict the scope of your regex patterns to minimize the risk of unintended matches. This can be done by anchoring your regex with ^
(start of string) and $
(end of string) anchors, or by using word boundaries with \b
. This practice ensures that your patterns only match the intended text, leading to more predictable outcomes.
import java.util.regex.Pattern; import java.util.regex.Matcher; public class ScopedPattern { public static void main(String[] args) { String text = "This is a sample sentence containing word."; String regex = "\bword\b"; // Matches 'word' as a separate word Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(text); if (matcher.find()) { System.out.println("Found the word: " + matcher.group()); } else { System.out.println("Word not found."); } } }
4. Use Named Groups for Clarity
Java regex supports named groups, which can make your patterns easier to understand and use. Named groups allow you to assign a name to a capturing group that can be referenced later. This can be particularly helpful when dealing with complex patterns, as it enhances readability and reduces the reliance on numeric indices.
import java.util.regex.Pattern; import java.util.regex.Matcher; public class NamedGroups { public static void main(String[] args) { String text = "Neil Hamilton, 30 years old."; String regex = "(?[A-Za-z ]+), (?\d+) years old"; Pattern pattern = Pattern.compile(regex); Matcher matcher = pattern.matcher(text); if (matcher.find()) { System.out.println("Name: " + matcher.group("name")); System.out.println("Age: " + matcher.group("age")); } } }
5. Test and Validate Your Patterns
Before deploying regex patterns in production code, it’s crucial to test and validate them thoroughly. Use online regex testers or unit tests to ensure your patterns behave as expected across a variety of input scenarios. This diligence can help you catch edge cases and avoid bugs that may arise from incorrect regex patterns.
import java.util.regex.Pattern; import java.util.regex.Matcher; public class RegexTest { public static void main(String[] args) { String regex = "\d{3}-\d{2}-\d{4}"; // Social Security Number format Pattern pattern = Pattern.compile(regex); String[] testInputs = {"123-45-6789", "123-456-789", "12-34-5678"}; for (String input : testInputs) { Matcher matcher = pattern.matcher(input); System.out.print(input + " is "); System.out.println(matcher.matches() ? "valid" : "invalid"); } } }
By following these best practices, you can harness the full potential of Java’s regex capabilities while maintaining clean and efficient code. Regular expressions can be a powerful ally in string processing, but they require careful handling to avoid pitfalls that can lead to inefficient performance and difficult-to-read code.