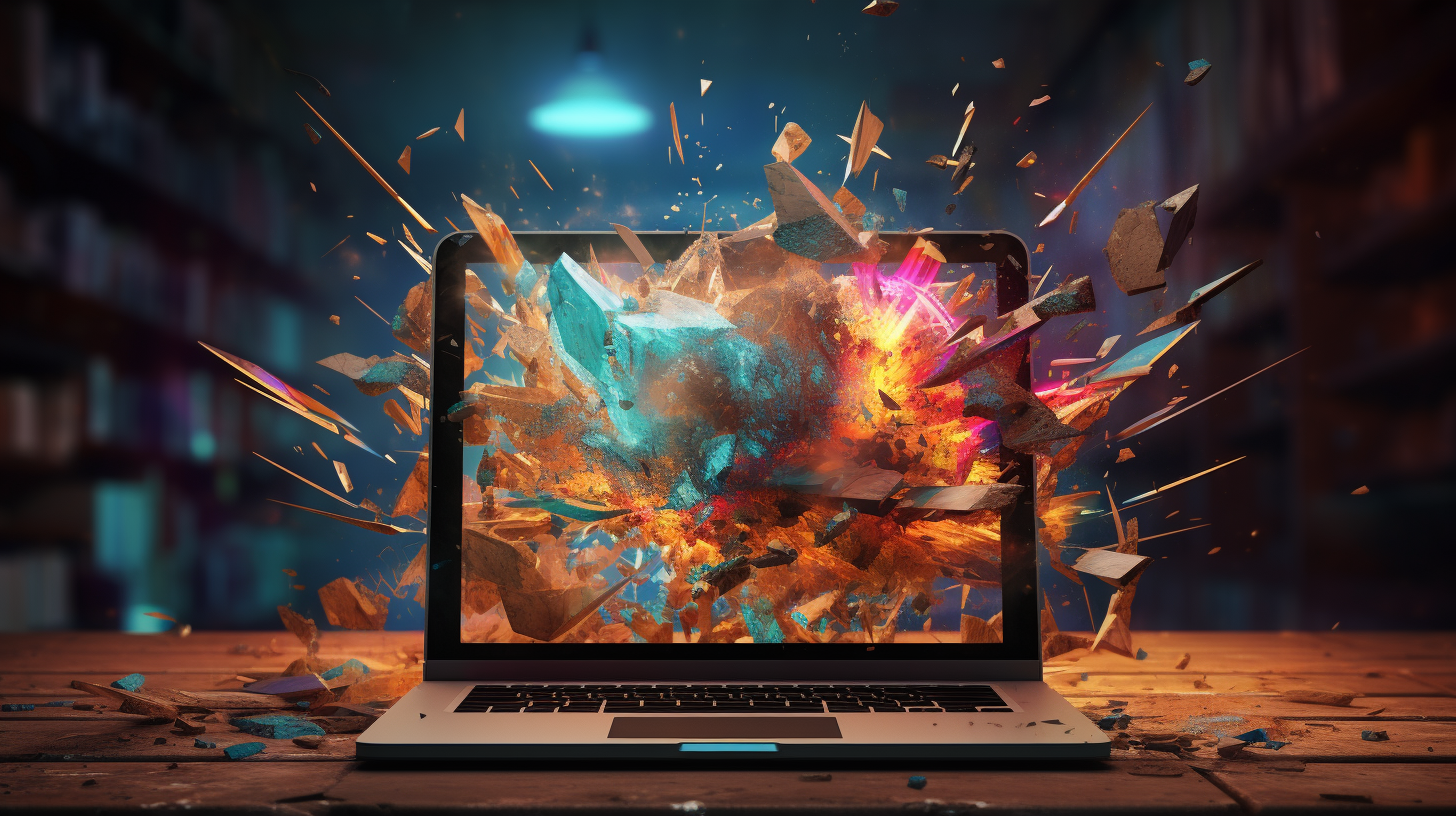
Bash Scripting for System Health Checks
When it comes to assessing the health of a system, certain key metrics are pivotal in providing a comprehensive overview. Monitoring these metrics helps in identifying potential issues before they escalate into critical problems. Here are some of the most vital system health metrics to keep an eye on:
CPU Usage: High CPU usage can indicate that processes are consuming too many resources, which may lead to performance degradation. It is essential to track both average and peak CPU usage over time.
top -b -n1 | grep "Cpu(s)"
Memory Usage: Memory is a finite resource, and monitoring its usage is important. You want to ensure that your applications have enough RAM to operate effectively without causing excessive swapping to disk.
free -h
Disk Space: Insufficient disk space can halt applications or lead to data loss. It’s vital to monitor disk usage to ensure that you have enough free space available for data and logs.
df -h
Network Throughput: Network performance can significantly affect application responsiveness, especially in distributed systems. Monitoring bandwidth and error rates can help identify bottlenecks.
ifstat -S
System Load Average: The load average indicates how many processes are actively competing for CPU time. A load average consistently higher than the number of CPU cores can signify that the system is overloaded.
uptime
By regularly monitoring these metrics, one can gain invaluable insights into system performance and readiness, which in turn informs both immediate actions and long-term planning. Each of these elements plays a critical role in ensuring system stability and efficiency.
Creating Basic Health Check Scripts
Creating basic health check scripts in Bash is a fundamental skill for system administrators and developers alike. These scripts can automate the monitoring of vital system metrics, allowing for timely intervention when issues arise. Below are the key components and examples that demonstrate how to build these scripts effectively.
First, let’s focus on the basic structure of a health check script. A good script begins with a shebang line that defines the interpreter to be used, followed by the commands to monitor various system health metrics. Below is a simple template for a health check script:
#!/bin/bash # Health Check Script # Function to check CPU usage check_cpu() { echo "Checking CPU Usage:" top -b -n1 | grep "Cpu(s)" } # Function to check Memory usage check_memory() { echo "Checking Memory Usage:" free -h } # Function to check Disk space check_disk() { echo "Checking Disk Space:" df -h } # Function to check Network throughput check_network() { echo "Checking Network Throughput:" ifstat -S } # Function to check Load Average check_load() { echo "Checking Load Average:" uptime } # Execute the health checks check_cpu check_memory check_disk check_network check_load
Each function in the script performs a specific health check. When executed, it will print the results of each metric to the terminal. This modular design allows for easy adjustments or expansions to the script as new requirements emerge.
Make sure to give execute permissions to your script before running it:
chmod +x health_check.sh
Then, you can run the script using:
./health_check.sh
In more complex scenarios, you might want to log the results of these checks to a file for future reference. This can be accomplished by redirecting the output to a log file:
./health_check.sh >> health_check.log
Furthermore, incorporating conditional checks into your script can enhance its functionality. For example, you can alert if CPU usage exceeds a specified threshold:
check_cpu() { echo "Checking CPU Usage:" cpu_usage=$(top -b -n1 | grep "Cpu(s)" | awk '{print $2 + $4}') threshold=80.0 if (( $(echo "$cpu_usage > $threshold" | bc -l) )); then echo "Warning: CPU usage is above ${threshold}%" else echo "CPU usage is within normal limits." fi }
This way, your script not only monitors system health but also provides proactive alerts, which can be crucial for maintaining system performance.
The ability to create basic health check scripts in Bash is invaluable for maintaining system integrity. By using simple functions and conditional logic, you can build robust monitoring tools that keep your systems running smoothly.
Automating Health Checks with Cron Jobs
To ensure that your Bash health check scripts run regularly without requiring manual intervention, you can automate their execution using Cron jobs. Cron is a time-based job scheduler in Unix-like operating systems, which will allow you to schedule tasks at specific intervals. This capability is especially useful for system health checks, enabling continuous monitoring without the need for constant supervision.
First, you need to open the Crontab configuration file for editing, which is done using the following command:
crontab -e
This will open the current user’s Crontab in your default text editor. Each line in this file represents a scheduled task, formatted to specify when and how often the task should run. The general syntax for a Cron job is as follows:
* * * * * /path/to/your/script.sh
The five asterisks represent the following time fields:
- 0-59
- 0-23
- 1-31
- 1-12
- 0-7 (both 0 and 7 represent Sunday)
For instance, if you want to run your health check script every hour, at the top of the hour, you would write:
0 * * * * /path/to/your/health_check.sh
This configuration instructs Cron to execute the script at minute 0 of every hour. You can customize the timing to suit your monitoring needs. Here are a few examples of common scheduling patterns:
*/5 * * * * /path/to/your/health_check.sh
0 0 * * * /path/to/your/health_check.sh
0 3 * * 0 /path/to/your/health_check.sh
After adding your desired Cron job to the file, save and exit the editor. Cron will automatically pick up the changes and start executing your health check script according to the specified schedule.
It’s also advisable to redirect the output of your Cron jobs to a log file. This way, you can review the results of each run and catch any potential issues. Modify your Cron job as follows:
0 * * * * /path/to/your/health_check.sh >> /path/to/your/health_check.log 2>&1
In this example, the standard output and error output are both redirected to the log file, allowing for comprehensive logging of the script’s execution.
By automating your health checks with Cron jobs, you create a safety net for your systems, ensuring that vital checks are performed regularly without the need for constant oversight. This not only enhances system reliability but also aids in timely detection and remediation of issues, preserving the integrity of your operational environment.
Logging and Reporting System Status
Logging and reporting system status is a critical aspect of maintaining a healthy operational environment. By capturing the output of health checks and storing it in a log file, administrators can not only review past performance but also identify trends over time, which may indicate potential issues before they escalate. This process transforms health checks from a simple monitoring tool into a powerful source of insight.
Integrating logging into your health check scripts is simpler. You can redirect the output of each health check directly into a log file, allowing for easy accumulation of data over time. Below is an enhanced version of the previously discussed health check script, now with logging capabilities:
#!/bin/bash LOGFILE="/var/log/health_check.log" # Function to log messages log() { echo "$(date '+%Y-%m-%d %H:%M:%S') - $1" >> "$LOGFILE" } # Function to check CPU usage check_cpu() { cpu_usage=$(top -b -n1 | grep "Cpu(s)" | awk '{print $2 + $4}') log "CPU Usage: $cpu_usage%" threshold=80.0 if (( $(echo "$cpu_usage > $threshold" | bc -l) )); then log "Warning: CPU usage is above ${threshold}%" else log "CPU usage is within normal limits." fi } # Function to check Memory usage check_memory() { memory_usage=$(free -h) log "Memory Usage: $memory_usage" } # Function to check Disk space check_disk() { disk_usage=$(df -h) log "Disk Space: $disk_usage" } # Function to check Network throughput check_network() { network_usage=$(ifstat -S 1 1) log "Network Throughput: $network_usage" } # Function to check Load Average check_load() { load_average=$(uptime) log "Load Average: $load_average" } # Execute the health checks check_cpu check_memory check_disk check_network check_load
In this version, the `log` function captures the current date and time along with the message passed to it. All logs are appended to a specified log file, providing a chronological record of system health status. This approach allows you to review logs at your convenience, making it easier to spot irregularities and trends.
It’s also beneficial to implement log rotation to manage the size of your log files. Tools like `logrotate` can help automate this process, ensuring that your logs remain manageable while retaining historical data. A simple configuration for `logrotate` might look like this:
/var/log/health_check.log { daily rotate 7 compress missingok notifempty }
This configuration tells `logrotate` to rotate the log file daily, keep the last seven days of logs, and compress older logs to save space. By maintaining clear logs, you ensure that your system’s health history is both accessible and manageable, facilitating better decision-making and timely responses.
Moreover, reporting can be enhanced by summarizing the log information into a more digestible format. You might want to create a summary report that condenses the key findings from your logs into a single output, which can be sent via email or displayed on a monitoring dashboard. A simple script could parse the log file and extract relevant data:
#!/bin/bash LOGFILE="/var/log/health_check.log" SUMMARYFILE="/var/log/health_check_summary.log" echo "Health Check Summary - $(date)" > "$SUMMARYFILE" echo "=====================================" >> "$SUMMARYFILE" grep "Warning" "$LOGFILE" >> "$SUMMARYFILE" || echo "No warnings found." >> "$SUMMARYFILE" echo "" >> "$SUMMARYFILE" echo "Full log can be found at $LOGFILE" >> "$SUMMARYFILE"
This script generates a summary of warnings recorded in the health check log, providing a quick overview of any issues that require attention. Such summaries are invaluable when time is of the essence and immediate action may be warranted.
In short, effective logging and reporting of system health not only aids in troubleshooting and performance tuning but also serves as a foundational element of proactive system management. By employing these strategies, you can enhance your system’s reliability and ensure that you’re always one step ahead of potential issues.
Troubleshooting and Error Handling in Scripts
When developing health check scripts, it’s essential to incorporate robust troubleshooting and error handling techniques. These practices ensure that your scripts can gracefully handle unexpected situations, providing clear feedback when something goes awry. Bash scripts may encounter various types of errors, from syntax errors to runtime exceptions, and preparing for these scenarios is vital for maintaining system reliability.
One of the first steps in error handling is to enable strict mode at the beginning of your script. This mode allows you to catch potential issues early in the execution process. You can enable strict mode using the following command:
set -euo pipefail
Here’s what each option does:
- Exit immediately if a command exits with a non-zero status, which helps catch failures early.
- Treat unset variables as an error when substituting, preventing the use of undefined variables.
- Return the exit status of the last command in a pipeline that returned a non-zero status instead of the last command’s status, making it easier to catch errors in pipelines.
Incorporating this setup into your health check scripts will significantly reduce the chances of subtle bugs or unnoticed failures. Once strict mode is enabled, the next step is to implement error handling within each function. Use conditional statements to check the success of commands and handle them appropriately. Here’s an improved version of the CPU check function that includes error handling:
check_cpu() { echo "Checking CPU Usage:" cpu_usage=$(top -b -n1 | grep "Cpu(s)" | awk '{print $2 + $4}') || { echo "Error: Unable to retrieve CPU usage" exit 1 } echo "CPU Usage: $cpu_usage%" threshold=80.0 if (( $(echo "$cpu_usage > $threshold" | bc -l) )); then echo "Warning: CPU usage is above ${threshold}%" else echo "CPU usage is within normal limits." fi }
In this example, if the command to retrieve CPU usage fails, an error message is printed, and the script exits with a status code of 1. This allows you to quickly identify and respond to issues without the script continuing to operate in a potentially erroneous state.
Additionally, logging errors to a separate log file can provide insights into recurring issues. You can modify the logging function to handle errors differently from standard log messages:
log_error() { echo "$(date '+%Y-%m-%d %H:%M:%S') - ERROR: $1" >> "$LOGFILE.error" }
This way, all error messages are directed to a dedicated error log, ensuring that they’re easy to track and analyze. Here’s how you might implement error logging in the memory check function:
check_memory() { memory_usage=$(free -h) || { log_error "Unable to retrieve memory usage" return 1 } log "Memory Usage: $memory_usage" }
The use of return codes is another effective method for signaling success or failure within your functions. By convention, a return code of 0 indicates success, while any non-zero value indicates an error. You can check the return value of each function when executing them in your main script:
check_cpu || exit 1 check_memory || exit 1 check_disk || exit 1 check_network || exit 1 check_load || exit 1
This approach prevents the script from continuing past a failed health check, so that you can address issues before proceeding. In scenarios where you may want to perform additional checks even if one fails, you can collect errors and report them at the end of the script instead.
Effective troubleshooting and error handling in Bash scripts enhance the reliability and maintainability of your health checks. By employing strict mode, conditional checks, specialized error logging, and proper return codes, you can create robust scripts that not only monitor system health but also respond gracefully to issues as they arise. This ensures that your systems remain healthy and operational, even in the face of unexpected challenges.