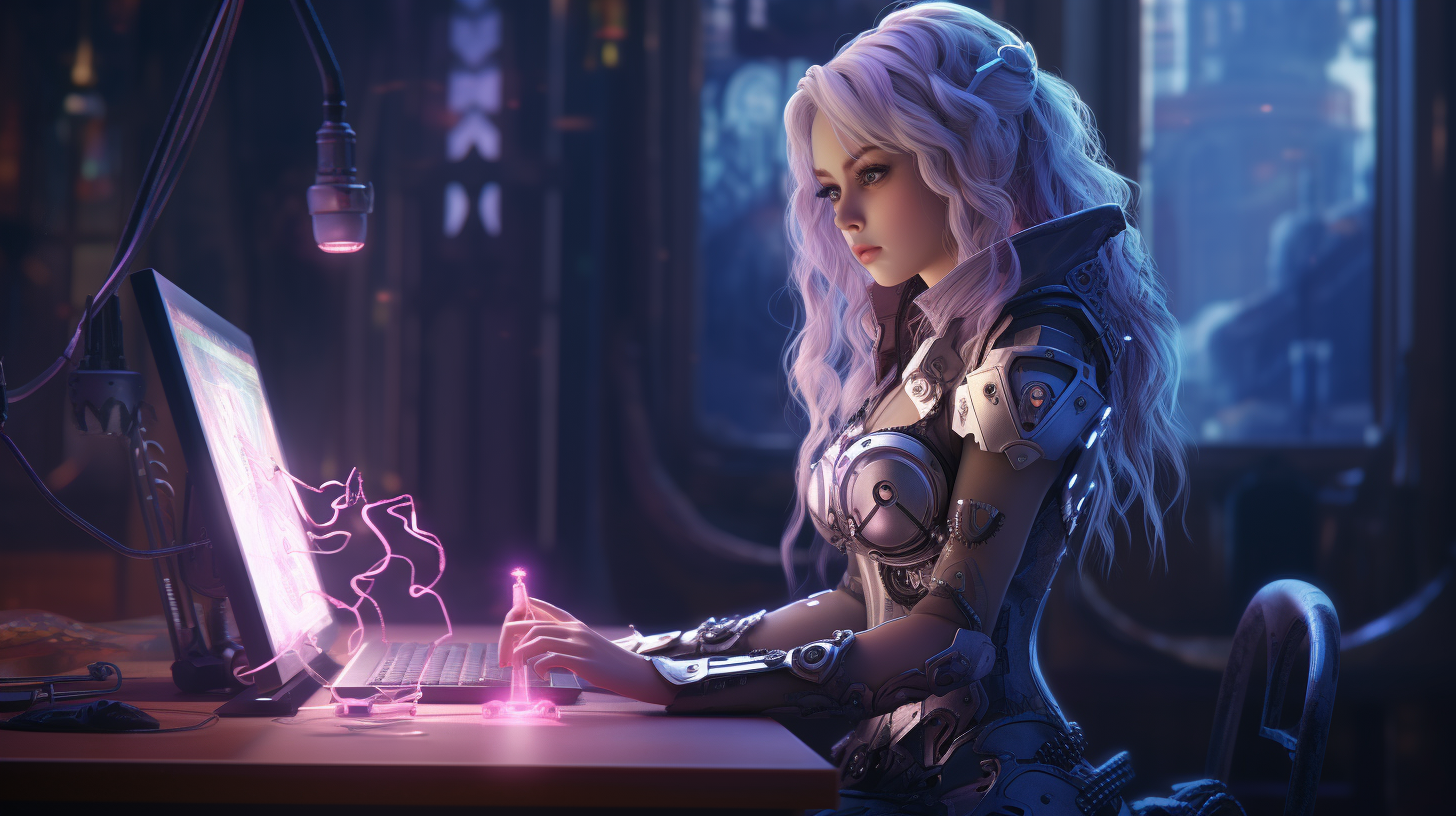
JavaScript and Progressive Web Apps (PWAs)
Progressive Web Apps (PWAs) represent a significant evolution in web technology, merging the best of web and mobile applications. They provide a compelling user experience by using state-of-the-art web capabilities, allowing them to work offline, load quickly, and feel like native mobile apps.
At their core, PWAs are built on standard web technologies—HTML, CSS, and JavaScript—enabling them to run on any device with a standards-compliant browser. This universality ensures that developers can reach a broader audience without the need for multiple codebases. PWAs deliver an app-like experience directly through the web, eliminating barriers associated with traditional app installations.
A key characteristic of PWAs is their ability to be installed on a user’s device, providing offline functionality and integrating into the device’s home screen. That is achieved through the use of Web App Manifests and Service Workers. The manifest file outlines how the app should appear on the device, detailing its name, icon, and display mode, while service workers manage caching strategies and network requests, enhancing the app’s performance and reliability.
One of the most powerful aspects of PWAs is their responsiveness. They adapt seamlessly to different screen sizes and orientations, ensuring a consistent experience across devices. That is accomplished through CSS media queries and flexible layouts that allow content to flow dynamically based on the user’s screen.
Another important factor is the use of HTTPS. PWAs require a secure context, making them safe from man-in-the-middle attacks and ensuring that all communications between the client and server are encrypted. This is vital for building trust with users and is a fundamental aspect of providing a secure web experience.
In practice, creating a PWA involves a few essential steps. Developers start by creating a typical web application, then enhance it by implementing a service worker. A simple service worker might look like this:
navigator.serviceWorker.register('/service-worker.js') .then(function(registration) { console.log('Service Worker registered with scope:', registration.scope); }) .catch(function(error) { console.error('Service Worker registration failed:', error); });
This snippet registers a service worker, providing a foundation for implementing caching strategies and offline capabilities. The service worker can intercept network requests, which will allow you to return cached resources when the network is unavailable.
Key Features of PWAs
Key features of Progressive Web Apps (PWAs) are what truly set them apart from traditional web applications and native mobile apps. Among these features, offline capabilities stand out as a game-changer. By using service workers, PWAs can cache essential resources, allowing users to access content even when they’re not connected to the internet. This capability is particularly important in areas with unreliable connectivity.
Another distinguishing feature is the ability of PWAs to provide a seamless installation experience. When users visit a PWA, they are prompted to add the app to their device’s home screen. Upon installation, the app can launch in a standalone window, free from the typical browser UI, which enhances the user experience. This transformation is facilitated by the Web App Manifest, a JSON file that defines how the PWA appears to users, including its icon, name, and theme color. A sample manifest file looks like this:
{ "name": "My Progressive Web App", "short_name": "MyPWA", "start_url": "/index.html", "display": "standalone", "background_color": "#ffffff", "theme_color": "#000000", "icons": [ { "src": "icon-192.png", "sizes": "192x192", "type": "image/png" }, { "src": "icon-512.png", "sizes": "512x512", "type": "image/png" } ] }
Responsiveness is another critical feature, ensuring that the PWA delivers optimal user experiences across various devices, be it a smartphone, tablet, or desktop. This adaptability is derived from responsive design principles, using CSS media queries to adjust layouts and styles based on the screen size. This way, the application feels consistent and intuitive, no matter how or where it’s accessed.
Security is paramount in the context of PWAs, and that is achieved through mandatory HTTPS. Secure connections not only protect user data but also enhance the overall trustworthiness of the application. A secure context is a requirement for service workers and provides assurance to users that their interactions are protected from potential threats.
Moreover, the ability to send push notifications is a key feature that keeps users engaged with the app. By using the Push API and Notifications API, developers can deliver timely updates and information directly to users, even when the app is not actively open. This capability can significantly boost user retention and engagement. Below is a simple example of how to request permission for notifications:
Notification.requestPermission().then(function(result) { if (result === 'granted') { console.log('Notification permission granted.'); } else { console.log('Notification permission denied.'); } });
JavaScript’s Role in Building PWAs
JavaScript plays an integral role in the construction of Progressive Web Apps (PWAs), serving as the backbone for interactivity, network management, and the overall user experience. By using JavaScript in conjunction with web APIs, developers can tap into the innate capabilities of the web platform, transforming a standard web application into a dynamic, engaging PWA.
One of the primary ways JavaScript is employed within PWAs is through service workers. These scripts run in the background, independent of web pages, enabling functionalities such as caching, background sync, and push notifications. To effectively implement a service worker, developers must define the caching strategy, determining which resources to cache and how to manage updates. Here’s a basic example of a service worker that caches specified assets:
self.addEventListener('install', function(event) { event.waitUntil( caches.open('my-cache-v1').then(function(cache) { return cache.addAll([ '/', '/index.html', '/styles.css', '/script.js', '/icon-192.png', '/icon-512.png' ]); }) ); }); self.addEventListener('fetch', function(event) { event.respondWith( caches.match(event.request).then(function(response) { return response || fetch(event.request); }) ); });
This service worker example demonstrates the installation phase, where essential files are cached. During the fetch event, the service worker checks if the requested resource is available in the cache; if it isn’t, it retrieves the resource from the network. This approach ensures that users have a consistent experience, even when offline.
JavaScript also facilitates the interaction with the Web App Manifest, allowing developers to dynamically manage app details. By programmatically accessing the manifest properties, developers can customize the app appearance based on user preferences or environmental conditions. For instance, you can set up a function to update the application’s theme color based on user input:
function updateThemeColor(color) { const metaThemeColor = document.querySelector("meta[name='theme-color']"); if (metaThemeColor) { metaThemeColor.setAttribute("content", color); } } // Example usage: updateThemeColor("#FF5733"); // Changes the theme color to a warm orange
Handling push notifications is another area where JavaScript shines in the context of PWAs. After obtaining permission from the user, developers can subscribe to push notifications using the Push API. Here’s how you can easily set up a subscription:
navigator.serviceWorker.ready.then(function(registration) { return registration.pushManager.subscribe({ userVisibleOnly: true, applicationServerKey: 'YOUR_PUBLIC_VAPID_KEY' }); }).then(function(subscription) { console.log('User is subscribed:', subscription); }).catch(function(error) { console.error('Failed to subscribe the user: ', error); });
In this example, upon successful subscription, the application can send push notifications, enhancing the user’s engagement with timely updates or alerts, even if the app is not active. JavaScript’s ability to manage these complex interactions within a PWA framework showcases its versatility in state-of-the-art web application development.
JavaScript’s asynchronous nature through promises and async/await syntax also plays a critical role in ensuring responsive user interfaces. By allowing developers to execute non-blocking operations, such as fetching data from an API while keeping the user interface responsive, PWAs can deliver a seamless user experience. For instance, here’s how you can fetch data asynchronously:
async function fetchData(url) { try { const response = await fetch(url); if (!response.ok) throw new Error('Network response was not ok'); const data = await response.json(); console.log(data); // Process the received data } catch (error) { console.error('Fetch error:', error); } } // Example usage: fetchData('https://api.example.com/data');
Service Workers and Caching Strategies
Service workers are at the heart of PWAs, acting as a powerful intermediary between the web application and the network. They enable sophisticated caching strategies that enhance performance, reliability, and the overall user experience. By intercepting network requests, service workers allow developers to define how and when resources are cached and served. This flexibility very important for optimizing loading times and ensuring seamless functionality, even when the user is offline.
When a service worker is registered, it can listen for various events, including install
and fetch
. The install event is where caching strategies are typically implemented. You might want to cache an initial set of critical resources that your application requires to function correctly. An example implementation is provided below:
self.addEventListener('install', function(event) { event.waitUntil( caches.open('my-app-cache').then(function(cache) { return cache.addAll([ '/', '/index.html', '/styles.css', '/script.js', '/icon-192.png', '/icon-512.png' ]); }) ); });
During the fetch event, the service worker can control how resources are served. It can respond with cached resources when available, thereby reducing load times and improving responsiveness. If the requested resource isn’t in the cache, it can proceed to fetch it from the network. Here’s how that can be accomplished:
self.addEventListener('fetch', function(event) { event.respondWith( caches.match(event.request).then(function(response) { return response || fetch(event.request); }) ); });
This approach ensures that users have access to the application’s core functionality even without a network connection. In scenarios where the user is offline, the service worker can provide a fallback response, such as a cached offline page or a message indicating that the application is unavailable.
Caching strategies can be further refined through the use of different cache versions, dynamic caching, and cache expiration policies. For instance, developers can implement stale-while-revalidate strategies, which serve cached content immediately while at once updating it in the background. This balances the need for fast loading times and up-to-date content, providing an optimal user experience.
For example, you could enhance the fetch event handler to implement this strategy:
self.addEventListener('fetch', function(event) { event.respondWith( caches.match(event.request).then(function(response) { const fetchPromise = fetch(event.request).then(networkResponse => { caches.open('my-app-cache').then(cache => { cache.put(event.request, networkResponse.clone()); }); return networkResponse; }); return response || fetchPromise; }) ); });
This code snippet will first attempt to serve the cached version of the requested resource. If the cached version doesn’t exist, it will fetch from the network and cache the newly retrieved resource for future requests. This ensures the application remains responsive and provides users with the most current content when online.
Managing cache size and handling updates to cached resources is another essential aspect of service worker implementations. Developers must think strategies for versioning caches and managing content expiration. A common practice involves checking for updates to resources and updating caches accordingly, allowing for clean and efficient resource management.
Enhancing User Experience with PWAs
Within the scope of Progressive Web Apps (PWAs), enhancing user experience hinges on a few pivotal components: speed, reliability, and engagement. The unique capabilities of PWAs not only allow for the offline functionality that users have come to expect but also provide a framework for building engaging experiences that feel native, even when accessed through a browser.
Speed is an essential aspect that directly impacts user satisfaction. A well-optimized PWA should load quickly, irrespective of network conditions. That is where the thoughtful implementation of caching strategies through service workers becomes indispensable. By pre-caching key assets during the install phase, developers can ensure that the most critical parts of the application are available immediately, even when a user revisits the app offline. The earlier example of a service worker that caches assets is a direct illustration of this concept:
self.addEventListener('install', function(event) { event.waitUntil( caches.open('my-cache-v1').then(function(cache) { return cache.addAll([ '/', '/index.html', '/styles.css', '/script.js', '/icon-192.png', '/icon-512.png' ]); }) ); });
Once the essential resources are cached, the fetch event listener can be employed to serve these resources efficiently. By employing a strategy that prioritizes cached content while still fetching updated resources from the network, developers can create an application that feels instantaneous. The following example details how you can achieve this:
self.addEventListener('fetch', function(event) { event.respondWith( caches.match(event.request).then(function(response) { return response || fetch(event.request).then(function(networkResponse) { return caches.open('my-cache-v1').then(function(cache) { cache.put(event.request, networkResponse.clone()); return networkResponse; }); }); }) ); });
Beyond performance, reliability is another cornerstone of user experience in PWAs. By enabling offline capabilities, users can continue to interact with the application without being tethered to the internet. That’s particularly important for users in regions with unstable connectivity. The application must gracefully handle scenarios where users lose their connection, providing offline pages or messages rather than just displaying errors. A simplistic offline fallback can be integrated into your service worker like this:
self.addEventListener('fetch', function(event) { event.respondWith( fetch(event.request).catch(function() { return caches.match('/offline.html'); }) ); });
Equally important is the aspect of engagement. PWAs can utilize push notifications to keep users informed and engaged, even when the app is not actively in use. The following code snippet demonstrates how to request permission for notifications and subscribe the user:
Notification.requestPermission().then(function(result) { if (result === 'granted') { navigator.serviceWorker.ready.then(function(registration) { return registration.pushManager.subscribe({ userVisibleOnly: true, applicationServerKey: 'YOUR_PUBLIC_VAPID_KEY' }); }).then(function(subscription) { console.log('User is subscribed:', subscription); }).catch(function(error) { console.error('Failed to subscribe the user: ', error); }); } });
This permission model not only ensures that users are kept up-to-date with relevant information but also reinforces that the application is responsive to their needs and preferences. Developers can send targeted notifications based on user behavior and app usage, thereby increasing engagement rates significantly.
Furthermore, the use of animations and transitions in conjunction with service workers and caching can greatly enhance perceived performance. JavaScript can be leveraged to create smooth animations that respond to user interactions, making the application feel alive and responsive. For instance, using CSS animations triggered by JavaScript events can create feedback that users expect in a native app context:
document.querySelector('.button').addEventListener('click', function() { this.classList.add('loading'); // Simulate a network request setTimeout(() => { this.classList.remove('loading'); }, 2000); });
Deploying and Maintaining a PWA
Deploying a Progressive Web App (PWA) involves several critical steps that ensure the application functions correctly in a production environment. Once the PWA is developed, it’s essential to host it on a server that supports HTTPS, as secure contexts are mandatory for service workers and other PWA features. Popular choices for hosting PWAs include platforms like Firebase Hosting, Netlify, or traditional web servers that support SSL certificates.
After hosting, you need to ensure that your PWA is discoverable and can be installed by users. This is typically achieved by configuring the Web App Manifest correctly. The manifest file should be linked in the HTML and provide essential metadata about the app, including its name, icons, and start URL. Here’s a brief example of how to link the manifest in your HTML:
In addition to the manifest, you must also ensure the service worker is correctly registered and operational. This entails checking that the service worker file is accessible and that it registers successfully. Here’s a sample registration script that can be included in your main JavaScript file:
if ('serviceWorker' in navigator) { window.addEventListener('load', function() { navigator.serviceWorker.register('/service-worker.js').then(function(registration) { console.log('Service Worker registered successfully with scope:', registration.scope); }).catch(function(error) { console.error('Service Worker registration failed:', error); }); }); }
Once deployed, maintaining a PWA involves monitoring its performance, updating content, and managing cached assets. Regular updates are crucial to ensure that users have access to the latest features and fixes. This can often be managed through the service worker, which can listen for the ‘update’ event to handle new versions of cached resources. For instance, you might want to prompt users when a new version of the application is available:
self.addEventListener('install', function(event) { self.skipWaiting(); // Forces waiting service workers to become the active service worker }); self.addEventListener('activate', function(event) { event.waitUntil( caches.keys().then(function(cacheNames) { return Promise.all( cacheNames.map(function(cacheName) { // Delete old caches if (cacheName !== 'my-app-cache') { return caches.delete(cacheName); } }) ); }) ); });
Furthermore, developers should implement analytics to track user engagement and application performance. Tools like Google Analytics can be integrated into the PWA to gather insights on how users interact with the app. This data is invaluable for making informed decisions about future updates and enhancements.
Lastly, it’s essential to ensure that your PWA is performant and provides a good user experience across all devices. Regularly testing the application on different devices and network conditions will help identify potential issues. Tools like Lighthouse can be utilized to audit performance and accessibility, providing actionable insights for improvement.