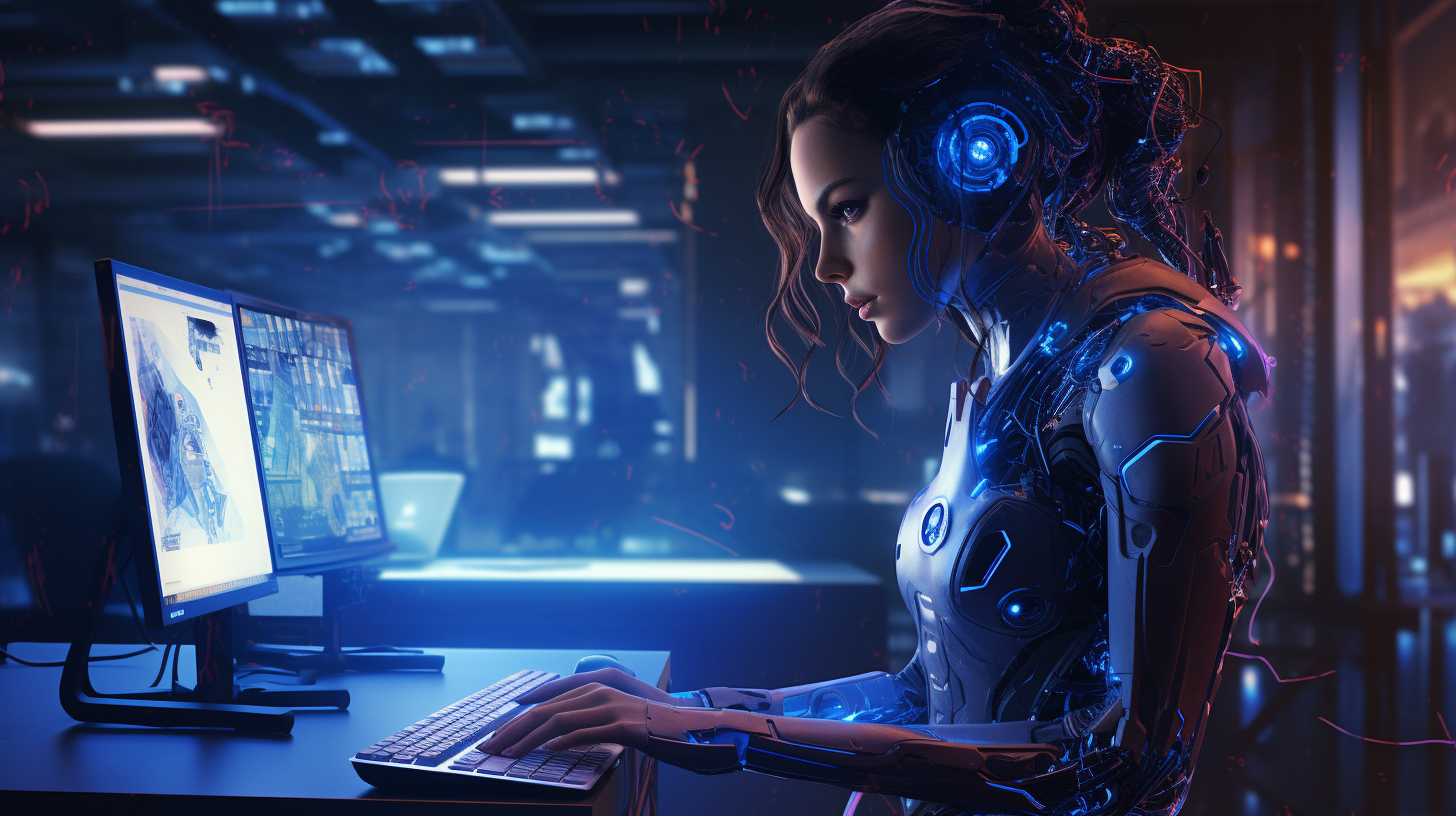
Closures in Swift
In Swift, closures are self-contained blocks of functionality that can be passed around and used in your code. They can capture and store references to any constants and variables from the context in which they are defined, which makes them incredibly powerful and flexible. You can consider of closures as similar to blocks in C or Objective-C, or lambdas in other programming languages. They are essentially anonymous functions that you can define inline, which allows for cleaner, more concise code.
Closures can be used for a variety of purposes, including callbacks, event handling, and custom sorting. One of their key features is that they can capture values, which means that they can remember the context in which they were created. This ability to capture values makes closures particularly useful for asynchronous operations, where you might want to perform some action once a task completes without needing to pass all the required data explicitly.
Think the following simple example that demonstrates a closure capturing a value. In this scenario, we define a closure that adds a number to a fixed constant:
let addToFive: (Int) -> Int = { number in return number + 5 } let result = addToFive(10) // result will be 15
In the example above, the closure addToFive
takes an integer as a parameter and adds 5 to it. The closure is defined inline, which allows for clarity and immediacy in understanding what the closure does.
Closures in Swift can also capture references to variables from their surrounding context. This is especially useful in situations where you need to maintain state in asynchronous operations. Here’s an example that demonstrates value capturing:
func makeIncrementer(incrementAmount: Int) -> () -> Int { var total = 0 let incrementer: () -> Int = { total += incrementAmount return total } return incrementer } let incrementByTwo = makeIncrementer(incrementAmount: 2) print(incrementByTwo()) // prints 2 print(incrementByTwo()) // prints 4
In this example, the makeIncrementer
function returns a closure that increments a total by a specified amount every time it is called. The variable total
is captured by the closure, allowing it to maintain its state across multiple invocations.
Understanding closures especially important for effective Swift programming, as they provide a powerful way to encapsulate functionality and maintain state. Their ability to capture values from their context opens up numerous possibilities for writing cleaner, more modular code.
Syntax and Structure of Closures
To dive deeper into the syntax and structure of closures in Swift, it’s essential to understand the components that make up a closure declaration. A closure in Swift can be defined with or without parameters, return types, and can also have an implicit return when it’s a single expression. Let’s break down the syntax step-by-step.
The basic structure of a closure can be expressed as follows:
{ (parameters) -> returnType in // closure body }
Here, the closure begins with an opening curly brace, followed by an optional list of parameters enclosed in parentheses. After the parameters, you specify the return type, using the arrow operator (->) to denote the type that the closure will return. The keyword in separates the parameters and return type from the body of the closure, where the actual functionality is defined.
For example, a closure that takes two integers and returns their sum might look like this:
let sumClosure: (Int, Int) -> Int = { (a: Int, b: Int) in return a + b }
Here, sumClosure is a closure that takes two Int parameters and returns an Int result. The closure captures the values of a and b, adding them together and returning the result. However, Swift allows for a more concise syntax when the type can be inferred:
let sumClosure: (Int, Int) -> Int = { a, b in return a + b }
In this case, we omitted the type annotations for the parameters since Swift can infer their types from the closure’s declaration. If the body of the closure is a single expression, we can eliminate the return keyword entirely:
let sumClosure: (Int, Int) -> Int = { a, b in a + b }
Additionally, when using closures as function parameters, we can also use shorthand argument names. Swift provides implicit names for parameters in closures, which are denoted by the dollar sign followed by the parameter index. For instance, the same closure written using shorthand argument names would look like this:
let sumClosure: (Int, Int) -> Int = { $0 + $1 }
This version of the closure uses $0 and $1 to refer to the first and second parameters, respectively, further simplifying the syntax.
Closures can also capture and store references to variables and constants from their surrounding context, which allows for even more versatile implementations. Here’s a closure that captures a variable from its environment:
func makeMultiplier(factor: Int) -> (Int) -> Int { return { number in number * factor } } let double = makeMultiplier(factor: 2) print(double(5)) // prints 10
In this example, the closure returned by makeMultiplier captures the factor variable. When we call the double closure with an argument of 5, it multiplies that argument by the captured factor, yielding an output of 10.
This elegant syntax and structure not only enhance the readability of Swift code but also enable developers to leverage the power of closures in various programming paradigms, from functional programming to callbacks in asynchronous operations. Understanding how to articulate and utilize closures effectively especially important for mastering Swift and writing clean, efficient code.
Capturing Values and Closure Expressions
Closures in Swift are not just anonymous functions; they come with a powerful feature known as value capturing. This means that closures can capture and remember the values of variables and constants from the context in which they are defined. This ability makes closures particularly useful in many programming scenarios, especially when dealing with asynchronous operations or callbacks where maintaining state is critical.
When a closure captures a variable, it retains a reference to that variable. This allows the closure to use the variable even if it’s outside its immediate scope. To illustrate this concept, think the following example:
func createCounter() -> () -> Int { var count = 0 let increment: () -> Int = { count += 1 return count } return increment } let counter = createCounter() print(counter()) // prints 1 print(counter()) // prints 2 print(counter()) // prints 3
In this example, the function createCounter
returns a closure that increments a counter each time it’s called. The variable count
is captured by the closure, allowing it to maintain its state between calls. Each call to counter()
produces a new value, demonstrating how closures can effectively encapsulate state.
Another notable aspect of closures in Swift is how they can be expressed as closure expressions. Closure expressions provide a concise way to define closures without the need for a full function definition. This can lead to cleaner code, especially when dealing with short closures that are used as arguments in function calls. Here’s an example of a closure expression used to sort an array:
let numbers = [5, 3, 1, 4, 2] let sortedNumbers = numbers.sorted { $0 < $1 } print(sortedNumbers) // prints [1, 2, 3, 4, 5]
In this case, the closure expression { $0 < $1 }
is used to determine the ordering of the numbers. The implicit parameters $0
and $1
refer to the first and second elements being compared, respectively. This syntax is both succinct and expressive, allowing developers to write compact and understandable sorting logic.
Closures can also capture more complex data types, not just simple variables. For example, consider a scenario where you want to keep track of the average of a series of numbers. A closure can capture the total and count of numbers to compute the average:
func makeAverageCalculator() -> (Int) -> Double { var total = 0 var count = 0 return { number in total += number count += 1 return Double(total) / Double(count) } } let average = makeAverageCalculator() print(average(10)) // prints 10.0 print(average(20)) // prints 15.0 print(average(30)) // prints 20.0
In this example, the closure returned by makeAverageCalculator
captures both total
and count
. Each time a new number is processed, the closure updates these captured variables and calculates the average based on the current total and count.
Thus, the capturing behavior of closures in Swift is not just a technical detail; it fundamentally changes how we can structure our code. By allowing closures to retain access to their surrounding context, Swift enables developers to write more modular and maintainable code, making it easier to manage state across various operations. Understanding this feature is key to using the full potential of closures in Swift programming.
Using Closures as Function Parameters and Return Types
When working with closures in Swift, one of the most powerful features is their ability to be passed around as function parameters and even returned from functions. This flexibility allows developers to write highly abstracted and reusable code, using the power of closures as first-class citizens in the language. Using closures as function parameters enables a variety of programming paradigms, including functional programming styles, callback mechanisms, and event handling.
To illustrate the concept of using closures as function parameters, consider a scenario where we want to perform a specific operation on an array of integers. We can define a function that takes an array and a closure as parameters. The closure will dictate how each element in the array should be processed. Here’s an example:
func processArray(array: [Int], operation: (Int) -> Int) -> [Int] { var processedArray: [Int] = [] for number in array { let processedNumber = operation(number) processedArray.append(processedNumber) } return processedArray } let numbers = [1, 2, 3, 4, 5] let doubledNumbers = processArray(array: numbers) { $0 * 2 } print(doubledNumbers) // prints [2, 4, 6, 8, 10]
In this example, the function processArray
takes two parameters: an array of integers and a closure that defines an operation to be performed on each element of the array. We can easily customize the behavior of this function by passing different closures. Here, we passed a closure that doubles each number, demonstrating how closures provide a mechanism for flexible code reuse.
Moreover, closures can also be returned from functions, which enhances their utility even further. This allows for the creation of factory functions that generate closures tailored to specific configurations. Here’s an example that encapsulates a simple arithmetic operation:
func makeOperation(operation: String) -> (Int, Int) -> Int { switch operation { case "add": return { $0 + $1 } case "subtract": return { $0 - $1 } case "multiply": return { $0 * $1 } case "divide": return { $0 / $1 } default: return { _, _ in 0 } } } let addition = makeOperation(operation: "add") print(addition(4, 5)) // prints 9 let multiplication = makeOperation(operation: "multiply") print(multiplication(4, 5)) // prints 20
In this case, the makeOperation
function returns a closure based on the specified operation type. Each closure encapsulates the logic for a particular arithmetic operation, allowing for easy adjustments and configurations based on context.
Using closures as return types provides a powerful abstraction mechanism, allowing for more dynamic and flexible programming patterns. This can lead to cleaner and more maintainable code, especially in scenarios involving callbacks or asynchronous operations.
Using closures in this way showcases their versatility and highlights the importance of understanding their application as both function parameters and return types. By effectively using closures, developers can create highly modular code that’s both easy to understand and maintain.