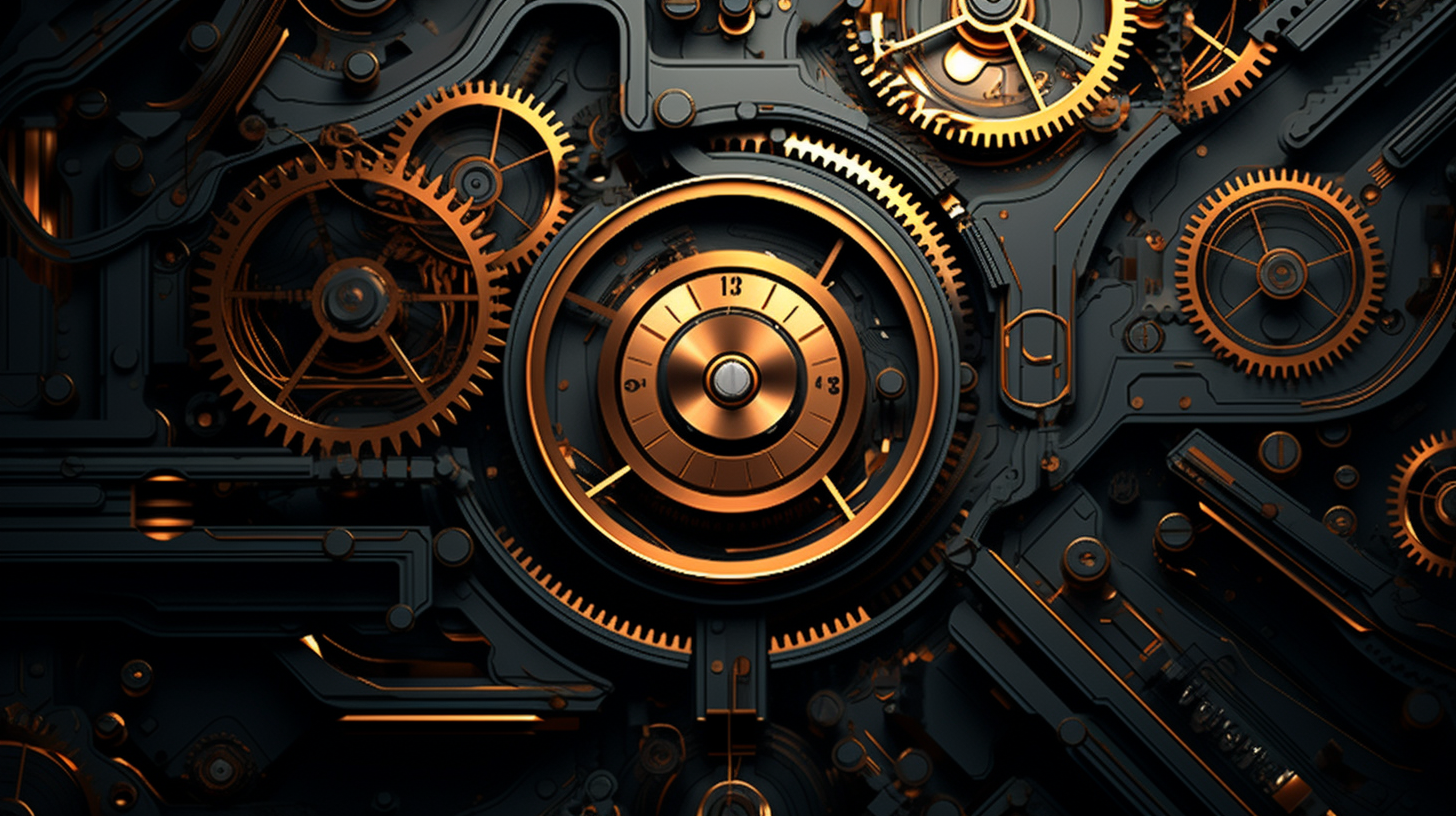
Java and Cryptography: Secure Coding
The Java Cryptography Architecture (JCA) provides a framework for implementing cryptographic operations in Java applications. It defines a set of APIs that developers can use to include cryptographic functionalities such as encryption, decryption, key generation, and secure hashing in their applications.
At the heart of the JCA is the notion of providers. A provider is a package that implements a set of cryptographic algorithms and provides the requisite functionality. Java comes with a default provider, but you can also add third-party providers to extend the cryptographic capabilities of your application.
The JCA is structured around several key components:
- Used for creating a fixed-size hash from input data. That’s useful for data integrity checks.
- Provides methods for transforming data into an unreadable format and back. Java supports symmetric and asymmetric algorithms.
- Facilitates the creation of cryptographic keys using various algorithms.
- Allows for the generation and verification of digital signatures, ensuring the authenticity and integrity of data.
To utilize these components, developers often interact with the java.security
and javax.crypto
packages. Below is an example demonstrating how to perform a simple hash operation using the SHA-256 algorithm:
import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; public class SHA256Example { public static void main(String[] args) { String input = "Hello, World!"; try { MessageDigest digest = MessageDigest.getInstance("SHA-256"); byte[] hash = digest.digest(input.getBytes()); StringBuilder hexString = new StringBuilder(); for (byte b : hash) { String hex = Integer.toHexString(0xff & b); if (hex.length() == 1) hexString.append('0'); hexString.append(hex); } System.out.println("Hash: " + hexString.toString()); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } } }
By using the JCA, developers can ensure that their applications adhere to contemporary security standards and best practices. The architecture’s flexibility allows for the integration of various algorithms and methodologies, enabling robust cryptographic solutions tailored to specific application requirements.
Common Cryptographic Algorithms in Java
Within the Java Cryptography Architecture, several common cryptographic algorithms are integral to implementing security in applications. These algorithms can be broadly categorized into three types: symmetric key algorithms, asymmetric key algorithms, and cryptographic hash functions. Understanding these algorithms very important for developers looking to secure data effectively.
Symmetric Key Algorithms
Symmetric key algorithms utilize the same key for both encryption and decryption. This means that both the sender and receiver must share the secret key securely before communication can commence. Common symmetric key algorithms in Java include AES (Advanced Encryption Standard), DES (Data Encryption Standard), and 3DES (Triple DES). Of these, AES is the most widely used due to its strength and efficiency.
import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; public class AESEncryptionExample { public static void main(String[] args) throws Exception { String data = "Confidential Data"; // Generate a key KeyGenerator keyGen = KeyGenerator.getInstance("AES"); keyGen.init(128); // Key size SecretKey secretKey = keyGen.generateKey(); // Encrypt data Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, secretKey); byte[] encryptedData = cipher.doFinal(data.getBytes()); System.out.println("Encrypted Data: " + new String(encryptedData)); // Decrypt data cipher.init(Cipher.DECRYPT_MODE, secretKey); byte[] decryptedData = cipher.doFinal(encryptedData); System.out.println("Decrypted Data: " + new String(decryptedData)); } }
Asymmetric Key Algorithms
Asymmetric key algorithms, also known as public-key algorithms, use a pair of keys for encryption and decryption: a public key for encryption and a private key for decryption. This allows anyone to encrypt data using the public key, but only the holder of the private key can decrypt it. Notable asymmetric algorithms include RSA (Rivest-Shamir-Adleman) and DSA (Digital Signature Algorithm).
import java.security.KeyPair; import java.security.KeyPairGenerator; import java.security.PrivateKey; import java.security.PublicKey; import javax.crypto.Cipher; public class RSAEncryptionExample { public static void main(String[] args) throws Exception { // Generate RSA key pair KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("RSA"); keyPairGen.initialize(2048); KeyPair keyPair = keyPairGen.generateKeyPair(); PublicKey publicKey = keyPair.getPublic(); PrivateKey privateKey = keyPair.getPrivate(); String data = "Sensitive Information"; // Encrypt data with public key Cipher cipher = Cipher.getInstance("RSA"); cipher.init(Cipher.ENCRYPT_MODE, publicKey); byte[] encryptedData = cipher.doFinal(data.getBytes()); System.out.println("Encrypted Data: " + new String(encryptedData)); // Decrypt data with private key cipher.init(Cipher.DECRYPT_MODE, privateKey); byte[] decryptedData = cipher.doFinal(encryptedData); System.out.println("Decrypted Data: " + new String(decryptedData)); } }
Cryptographic Hash Functions
Hash functions are another critical component of cryptography, generating a fixed-size hash value from variable input data. They’re commonly used for data integrity checks, password storage, and digital signatures. SHA-256 and SHA-512 are popular hash functions in Java.
import java.security.MessageDigest; public class SHA512Example { public static void main(String[] args) throws Exception { String input = "Secure Password"; MessageDigest digest = MessageDigest.getInstance("SHA-512"); byte[] hash = digest.digest(input.getBytes()); StringBuilder hexString = new StringBuilder(); for (byte b : hash) { String hex = Integer.toHexString(0xff & b); if (hex.length() == 1) hexString.append('0'); hexString.append(hex); } System.out.println("Hash: " + hexString.toString()); } }
By mastering these common cryptographic algorithms, Java developers can implement robust security measures in their applications, ensuring confidentiality, integrity, and authentication throughout their systems.
Implementing Secure Password Storage
When it comes to implementing secure password storage in Java, a multi-layered approach is essential. Simply hashing passwords is no longer deemed sufficient due to the advancement of hardware capabilities that can enable brute-force attacks. Therefore, developers need to incorporate salting, hashing with a strong algorithm, and possibly key stretching techniques to enhance security.
Salting involves adding a unique, random string (the salt) to each password before hashing. This ensures that even if two users have the same password, their hashed values will be different. Additionally, using a slow hashing algorithm designed for passwords, such as bcrypt or PBKDF2, adds an extra layer of protection by making brute-force attacks more time-consuming and resource-intensive.
Let’s take a look at an example of how to implement secure password storage using PBKDF2. Java provides a convenient way to utilize this through the SecretKeyFactory
class and the PBEKeySpec
class.
import javax.crypto.SecretKeyFactory; import javax.crypto.spec.PBEKeySpec; import java.security.SecureRandom; import java.util.Base64; public class PasswordStorageExample { private static final int SALT_LENGTH = 16; // in bytes private static final int HASH_LENGTH = 64; // in bytes private static final int ITERATIONS = 65536; // Increasing iterations makes it slower public static void main(String[] args) throws Exception { String password = "mySecurePassword"; // Create salt byte[] salt = new byte[SALT_LENGTH]; SecureRandom random = new SecureRandom(); random.nextBytes(salt); // Hash the password byte[] hash = hashPassword(password, salt); // Store the salt and hash (typically in a database) String storedSalt = Base64.getEncoder().encodeToString(salt); String storedHash = Base64.getEncoder().encodeToString(hash); // Output for demonstration purposes System.out.println("Salt: " + storedSalt); System.out.println("Hash: " + storedHash); } public static byte[] hashPassword(String password, byte[] salt) throws Exception { PBEKeySpec spec = new PBEKeySpec(password.toCharArray(), salt, ITERATIONS, HASH_LENGTH * 8); SecretKeyFactory factory = SecretKeyFactory.getInstance("PBKDF2WithHmacSHA512"); return factory.generateSecret(spec).getEncoded(); } }
In this example, we generate a random salt using the SecureRandom
class, then hash the password using the PBEKeySpec
with PBKDF2 and HMAC-SHA512. The salt and hash are stored in a format suitable for future verification.
When a user attempts to authenticate, the process involves retrieving the salt and hash from storage, hashing the input password with the same salt, and comparing the result to the stored hash. If they match, the password is correct; otherwise, it is rejected.
public static boolean verifyPassword(String inputPassword, String storedHash, String storedSalt) throws Exception { byte[] salt = Base64.getDecoder().decode(storedSalt); byte[] inputHash = hashPassword(inputPassword, salt); return Base64.getEncoder().encodeToString(inputHash).equals(storedHash); }
This verifyPassword
method illustrates the verification process, ensuring that passwords are stored securely and checked accurately without compromising security practices. By implementing these methods, developers can significantly enhance the security of password storage in their Java applications.
Best Practices for Key Management
Key management is a foundational aspect of cryptography that involves the generation, distribution, storage, and destruction of cryptographic keys. Proper key management ensures that keys are maintained securely and only accessible to authorized entities. Poor key management practices can lead to vulnerabilities, making encrypted data susceptible to unauthorized access. Here are some best practices to think when managing cryptographic keys in Java applications.
1. Use Strong Key Generation Practices
Keys should be generated using secure algorithms and libraries. Java provides the KeyGenerator class, which can be used to create strong keys with appropriate lengths based on the algorithm used. For example, when using AES, a key length of 128, 192, or 256 bits is recommended. Always ensure that the key generation process is not predictable.
import javax.crypto.KeyGenerator; public class KeyGenerationExample { public static void main(String[] args) throws Exception { KeyGenerator keyGen = KeyGenerator.getInstance("AES"); keyGen.init(256); // Specify the key size SecretKey secretKey = keyGen.generateKey(); System.out.println("Generated Key: " + Base64.getEncoder().encodeToString(secretKey.getEncoded())); } }
2. Secure Key Storage
Keys should never be hardcoded into the source code or stored in plain text. Instead, use secure storage mechanisms such as hardware security modules (HSMs), Java’s KeyStore, or encrypted storage solutions. The Java KeyStore API allows developers to securely store cryptographic keys and certificates.
import java.security.KeyStore; import javax.crypto.SecretKey; import javax.crypto.KeyGenerator; public class SecureKeyStoreExample { public static void main(String[] args) throws Exception { // Create a KeyStore KeyStore keyStore = KeyStore.getInstance("JCEKS"); keyStore.load(null, null); // Initialize the KeyStore // Generate a key KeyGenerator keyGen = KeyGenerator.getInstance("AES"); keyGen.init(256); SecretKey secretKey = keyGen.generateKey(); // Store the key in the KeyStore keyStore.setKeyEntry("mySecretKey", secretKey, "myPassword".toCharArray(), null); System.out.println("Key stored in KeyStore."); } }
3. Implement Key Rotation
Regularly rotating cryptographic keys minimizes the risk of key compromise. Rotate keys periodically or when a certain event occurs, such as a suspected breach. Ensure that old keys are securely destroyed and that any data encrypted with old keys can be decrypted with them during the transition period.
public class KeyRotationExample { public static void main(String[] args) { // Pseudocode for key rotation // Retrieve the current key // Generate a new key // Re-encrypt data with the new key // Store the new key and securely delete the old key } }
4. Implement Access Controls
Limit access to cryptographic keys to only those components of your application that need them. Use principles of least privilege and ensure that key access is logged and monitored. This minimizes the risk of unauthorized access or exposure of sensitive keys.
import javax.crypto.Cipher; import javax.crypto.SecretKey; public class AccessControlExample { public static void main(String[] args) throws Exception { // Demonstration of limited key access SecretKey secretKey = ...; // Assume this is securely retrieved // Only allow specific operations with the key Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, secretKey); // Perform encryption operation } }
5. Audit and Monitoring
Regularly audit access to cryptographic keys and monitor for any suspicious activity. Implement logging mechanisms to track key usage and possible unauthorized access attempts. This visibility can help identify and mitigate potential security incidents.
By adhering to these best practices for key management, developers can significantly enhance the security posture of their Java applications, ensuring that cryptographic keys are protected and managed effectively. Properly managed keys lay the groundwork for robust cryptographic operations and help maintain the confidentiality and integrity of sensitive data.
Secure Communication Protocols in Java
Secure communication protocols are crucial for safeguarding data transmitted over networks. In Java, developers can implement secure communication using protocols such as SSL/TLS, which are built upon cryptographic principles that ensure data integrity, confidentiality, and authentication. The javax.net.ssl package provides a robust set of classes and interfaces to facilitate the implementation of SSL/TLS in Java applications.
When establishing a secure connection using SSL/TLS, the process typically involves the following steps:
import javax.net.ssl.SSLContext; import javax.net.ssl.TrustManager; import javax.net.ssl.TrustManagerFactory; import javax.net.ssl.SSLSocket; import javax.net.ssl.SSLSocketFactory; import java.io.BufferedReader; import java.io.InputStreamReader; import java.security.KeyStore; public class SecureSocketExample { public static void main(String[] args) throws Exception { // Load the KeyStore containing trusted certificates KeyStore keyStore = KeyStore.getInstance("JKS"); keyStore.load(SecureSocketExample.class.getResourceAsStream("/keystore.jks"), "keystorePassword".toCharArray()); // Initialize the TrustManagerFactory with the KeyStore TrustManagerFactory trustManagerFactory = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm()); trustManagerFactory.init(keyStore); // Create an SSLContext and initialize it with the TrustManagers SSLContext sslContext = SSLContext.getInstance("TLS"); TrustManager[] trustManagers = trustManagerFactory.getTrustManagers(); sslContext.init(null, trustManagers, null); // Get the SSLSocketFactory from the SSLContext SSLSocketFactory sslSocketFactory = sslContext.getSocketFactory(); SSLSocket sslSocket = (SSLSocket) sslSocketFactory.createSocket("example.com", 443); // Start the handshake sslSocket.startHandshake(); // Read data from the server BufferedReader in = new BufferedReader(new InputStreamReader(sslSocket.getInputStream())); String line; while ((line = in.readLine()) != null) { System.out.println(line); } // Close the socket sslSocket.close(); } }
In this example, we first load a KeyStore containing trusted certificates. The TrustManager is initialized using this KeyStore, which allows Java to validate the identity of the server it connects to. We then create an SSLContext that will handle the secure connections.
Next, we create an SSLSocket from the SSLSocketFactory, specifying the target server and port. After starting the SSL handshake, we can begin reading data securely from the server. This process ensures that the communication is encrypted and safe from eavesdropping.
For secure client-server communication, it’s essential to manage both server and client authentication properly. In some cases, client authentication may also be required, which involves sending certificates from the client to the server. This adds an additional layer of security, ensuring that only authorized clients can connect.
Using secure communication protocols like SSL/TLS is not just about encrypting the data being transmitted; it also involves ensuring that the parties involved in the communication are who they claim to be. By integrating these protocols into Java applications, developers can significantly enhance the security of data in transit, protecting sensitive information from various threats.
Handling Cryptographic Exceptions and Errors
When working with cryptographic operations in Java, developers must also be prepared to handle various exceptions and errors that may arise during these processes. Cryptographic operations can fail for a multitude of reasons, such as incorrect key specifications, unsupported algorithms, or invalid input data. Understanding how to properly handle these exceptions very important for maintaining the reliability and security of the application.
Java provides a comprehensive set of exceptions related to cryptography in the javax.crypto
and java.security
packages. Some common exceptions include:
- Thrown when a requested cryptographic algorithm is not available in the environment.
- Raised when a specified padding scheme is not available.
- Occurs if the key is invalid, such as when it’s of the wrong type or length.
- This exception is thrown when the length of data being processed is incorrect for the specified algorithm.
- Thrown when the padding of the data does not match the expected format, indicating that the data may have been tampered with or corrupted.
Handling these exceptions appropriately can help developers provide better feedback to users and maintain the integrity of their applications. Below is an example illustrating how to implement basic cryptographic operations while handling exceptions effectively:
import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.crypto.spec.SecretKeySpec; import java.security.NoSuchAlgorithmException; import java.security.InvalidKeyException; import javax.crypto.NoSuchPaddingException; import javax.crypto.BadPaddingException; import javax.crypto.IllegalBlockSizeException; public class EncryptionExample { public static void main(String[] args) { try { // Generate a secret key KeyGenerator keyGen = KeyGenerator.getInstance("AES"); keyGen.init(128); SecretKey secretKey = keyGen.generateKey(); byte[] dataToEncrypt = "Sensitive Data".getBytes(); // Encrypt the data Cipher cipher = Cipher.getInstance("AES/ECB/PKCS5Padding"); cipher.init(Cipher.ENCRYPT_MODE, secretKey); byte[] encryptedData = cipher.doFinal(dataToEncrypt); System.out.println("Encrypted data: " + new String(encryptedData)); // Decrypt the data cipher.init(Cipher.DECRYPT_MODE, secretKey); byte[] decryptedData = cipher.doFinal(encryptedData); System.out.println("Decrypted data: " + new String(decryptedData)); } catch (NoSuchAlgorithmException e) { System.err.println("Algorithm not found: " + e.getMessage()); } catch (NoSuchPaddingException e) { System.err.println("Padding not found: " + e.getMessage()); } catch (InvalidKeyException e) { System.err.println("Invalid key: " + e.getMessage()); } catch (IllegalBlockSizeException e) { System.err.println("Illegal block size: " + e.getMessage()); } catch (BadPaddingException e) { System.err.println("Bad padding: " + e.getMessage()); } catch (Exception e) { System.err.println("An unexpected error occurred: " + e.getMessage()); } } }
In this example, we use a try-catch block to handle specific exceptions that may arise during the encryption and decryption processes. By providing clear messages for each exception, developers can quickly identify issues and react accordingly. This not only aids in debugging but also enhances the user experience by avoiding crashes or unhandled exceptions.
Moreover, logging these exceptions is a good practice, as it allows developers to track issues and identify patterns in failure scenarios. Using a logging framework like SLF4J or Log4j can help integrate these logs seamlessly into an application’s logging strategy, providing better insights into operational health.
Effective handling of cryptographic exceptions and errors is vital for ensuring the robustness of applications that rely on cryptographic functionalities. By proactively managing exceptions, developers can enhance the security and reliability of their Java applications, ultimately leading to a more resilient software ecosystem.