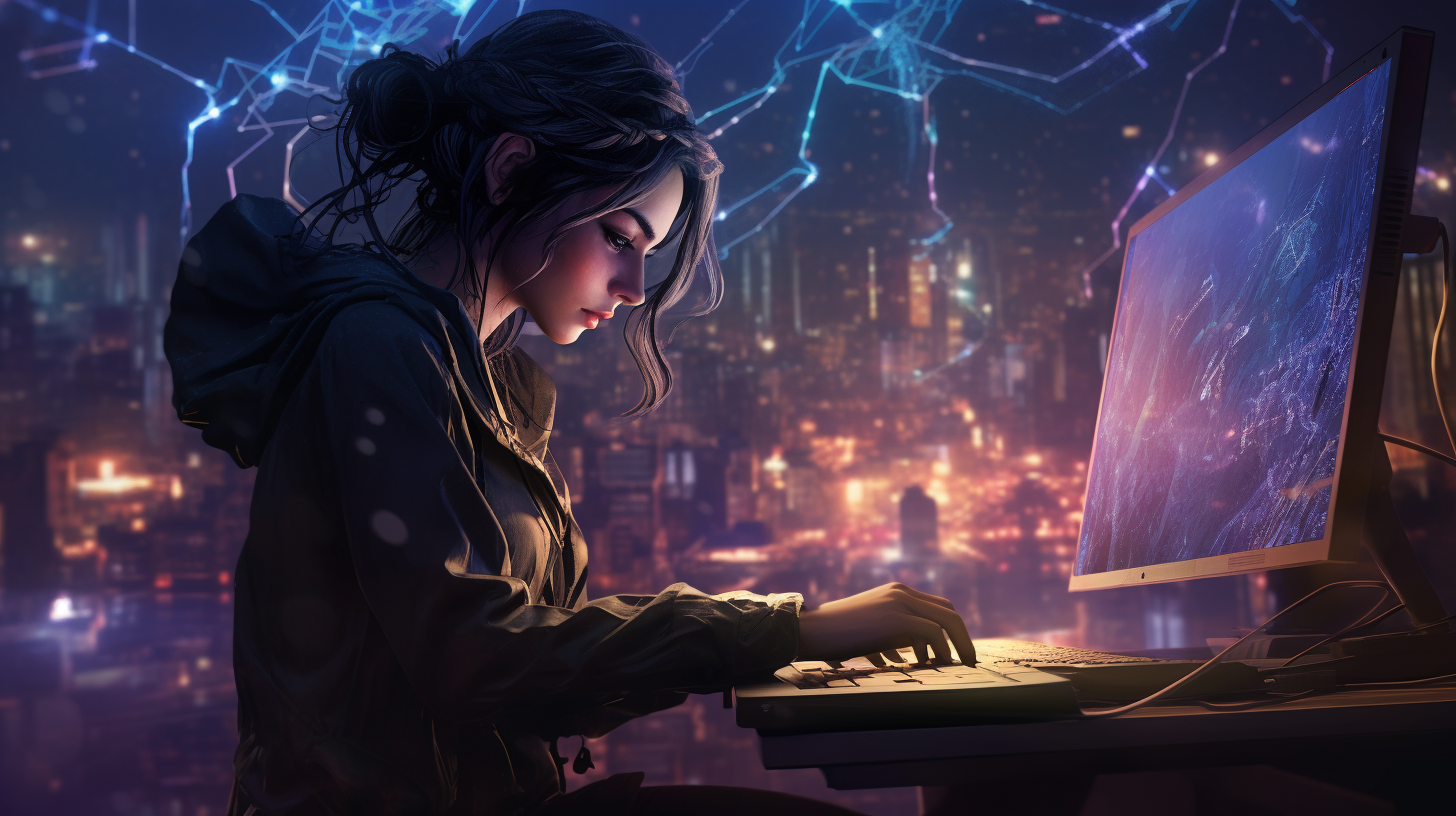
JavaScript and Online Surveys and Polls
JavaScript forms the backbone of interactive web applications, enabling developers to create engaging and responsive online surveys. Understanding the fundamentals of JavaScript is important for crafting surveys that not only capture user data but also enhance user interaction. At its core, JavaScript is a versatile programming language that allows you to manipulate HTML elements, respond to user events, and manage data dynamically.
One fundamental idea of JavaScript is the use of variables. Variables store data values and can be changed during the execution of a script. Here’s a simple example:
let userName = "Luke Douglas"; let userAge = 25;
In this example, we define two variables: userName
and userAge
. These variables can be used throughout the survey to personalize questions or store responses.
Another essential aspect to grasp is the use of functions, which allow you to encapsulate reusable code. A function can be triggered by events such as clicks or submissions, making it a powerful tool for creating interactive surveys. Here’s how you can define and call a function:
function submitSurvey() { alert("Thank you for your submission!"); }
To invoke this function when the user clicks a button, you would set an event listener:
document.getElementById("submitButton").addEventListener("click", submitSurvey);
Events are the lifeblood of user interaction in JavaScript. They allow your application to respond to user actions, such as clicks, key presses, or mouse movements. The addEventListener
method is a way to monitor these events and execute code accordingly. It is crucial to ensure that your survey captures user input efficiently and effectively.
Furthermore, JavaScript provides various data structures, such as arrays and objects, which are particularly useful for managing survey questions and responses. For instance, you can store a list of survey questions in an array:
const questions = [ "What is your favorite color?", "How often do you use our service?", "Would you recommend us to a friend?" ];
Iterating over this array allows you to dynamically generate survey questions in the HTML:
questions.forEach(question => { let questionElement = document.createElement("p"); questionElement.textContent = question; document.getElementById("surveyContainer").appendChild(questionElement); });
Using these fundamental concepts enables the creation of interactive surveys that respond to user input seamlessly. By effectively using variables, functions, events, and data structures, you can build a rich user experience that encourages participation and enhances data collection.
Creating Dynamic Polls with JavaScript
Creating dynamic polls with JavaScript involves more than just presenting questions to users; it also requires a thoughtful approach to managing user interactions and responses. To achieve this, developers can use various JavaScript features to manipulate the Document Object Model (DOM) and provide real-time feedback to users.
One effective way to create a dynamic poll is by using HTML forms combined with JavaScript to handle user input. By dynamically generating form elements based on a predefined set of questions, you can keep your code organized and easily maintainable. Here’s an example of how to create a poll that allows users to select their preferred options:
const pollQuestions = [ { question: "What is your favorite programming language?", options: ["JavaScript", "Python", "Java", "C#"] }, { question: "How do you prefer to learn programming?", options: ["Online courses", "Books", "YouTube", "Practice"] } ]; pollQuestions.forEach((poll) => { let questionElement = document.createElement("div"); let questionText = document.createElement("h3"); questionText.textContent = poll.question; questionElement.appendChild(questionText); poll.options.forEach((option) => { let optionLabel = document.createElement("label"); let optionInput = document.createElement("input"); optionInput.type = "radio"; optionInput.name = poll.question; optionInput.value = option; optionLabel.appendChild(optionInput); optionLabel.appendChild(document.createTextNode(option)); questionElement.appendChild(optionLabel); }); document.getElementById("pollContainer").appendChild(questionElement); });
In this snippet, we first define an array of poll questions, each with its associated options. The forEach method is then used to iterate through each question and create corresponding HTML elements. For each option, we generate a radio button, allowing users to select only one answer per question.
To improve interactivity, ponder adding a “Submit” button that will process the user’s selections. Using the same principles, we can easily capture user responses when the button is clicked:
function collectResponses() { const responses = {}; pollQuestions.forEach((poll) => { const selectedOption = document.querySelector(`input[name="${poll.question}"]:checked`); responses[poll.question] = selectedOption ? selectedOption.value : "No response"; }); console.log(responses); alert("Thank you for participating! Your responses have been recorded."); } document.getElementById("submitButton").addEventListener("click", collectResponses);
This function, collectResponses, iterates through the poll questions to gather user selections. The results are stored in an object, where each question corresponds to its selected answer. Using console.log helps in debugging by which will allow you to view the collected responses. Finally, an alert gives users confirmation of their submission.
Dynamic polls not only enrich the user experience but also enhance data collection by ensuring that responses are captured in real-time and can be easily managed. By combining these JavaScript techniques, developers can build interactive polls that engage users and streamline the feedback process.
Using APIs for Survey Data Collection
APIs (Application Programming Interfaces) are essential tools that enable developers to connect their applications with external services, making them invaluable for collecting survey data. When it comes to online surveys, using APIs allows for efficient data submission, retrieval, and analysis, providing a seamless flow of information between the client-side application and server-side resources.
One of the most common use cases for APIs in survey applications is to send user responses to a server for storage. In a typical scenario, once a user completes a survey, the collected data should be sent to a backend service for processing and analysis. JavaScript’s fetch API provides a simpler way to handle such HTTP requests. Here’s an example illustrating how to submit survey responses to a server:
const submitSurveyData = async (responses) => { try { const response = await fetch('https://yourapi.com/submit', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(responses) }); if (!response.ok) { throw new Error('Network response was not ok'); } const data = await response.json(); console.log('Success:', data); alert('Your responses have been submitted successfully!'); } catch (error) { console.error('Error:', error); alert('There was an issue submitting your responses.'); } };
In this code snippet, the submitSurveyData function takes an object of user responses as an argument. It utilizes the fetch API to send a POST request to the specified endpoint. The response from the server is then checked for success, and appropriate actions are taken based on the outcome. This asynchronous approach ensures that the application remains responsive while waiting for the server’s reply.
To call this function after collecting survey responses, you would modify your collectResponses function like this:
function collectResponses() { const responses = {}; pollQuestions.forEach((poll) => { const selectedOption = document.querySelector(`input[name="${poll.question}"]:checked`); responses[poll.question] = selectedOption ? selectedOption.value : "No response"; }); console.log(responses); submitSurveyData(responses); }
By implementing API calls in this manner, you streamline the process of data collection, allowing for real-time analytics and user feedback. Furthermore, you can enhance your surveys by integrating other APIs that provide additional functionalities, such as analytics, user authentication, or even third-party survey platforms.
Another critical aspect of using APIs for survey data collection is the ability to retrieve previous survey results for display or analysis. For this, you would similarly use the fetch API to perform a GET request. Here’s a basic example of how to retrieve survey results:
const fetchSurveyResults = async () => { try { const response = await fetch('https://yourapi.com/results'); if (!response.ok) { throw new Error('Network response was not ok'); } const results = await response.json(); console.log('Survey Results:', results); // Code to display results in the UI would go here } catch (error) { console.error('Error:', error); } };
This fetchSurveyResults function allows you to asynchronously retrieve existing survey results, which can then be processed or displayed in the user interface. By managing both data submission and retrieval through APIs, you create a robust system that not only collects user input but also provides insights into survey performance and user engagement.
Using APIs for survey data collection enhances the capabilities of JavaScript applications, allowing for efficient management of user responses. By using the fetch API for both sending and receiving data, developers can create a seamless and interactive experience for users, ultimately leading to better data insights and enhanced survey effectiveness.
Enhancing User Experience with JavaScript Frameworks
Enhancing user experience in online surveys is a critical component that can significantly influence participation rates and the quality of data collected. By integrating JavaScript frameworks, developers can create more responsive, interactive, and visually appealing surveys, thereby fostering a more engaging environment for users. Frameworks like React, Vue, and Angular provide robust tools for managing state, rendering components, and handling user interactions efficiently.
One of the core advantages of using JavaScript frameworks is the ability to manage the state of your application in a more organized manner. For instance, in a React application, you can utilize component state to control user inputs dynamically. Here’s an example of how to implement a simple survey form using React:
import React, { useState } from 'react'; const SurveyForm = () => { const [responses, setResponses] = useState({}); const handleInputChange = (event) => { const { name, value } = event.target; setResponses({...responses, [name]: value}); }; const handleSubmit = (event) => { event.preventDefault(); console.log('Submitted Responses:', responses); alert('Thank you for your submission!'); // You could call an API here to send responses }; return (Daily Weekly Monthly); }; export default SurveyForm;
In this example, the SurveyForm component maintains the user responses in the local state using the useState hook. Event handlers manage input changes and form submission, ensuring that the data is captured dynamically. This structure not only streamlines the development process but also enhances the user experience by providing immediate feedback on their interactions.
Furthermore, frameworks like Vue.js allow for similar constructs with a more simpler syntax. Vue’s reactivity system ensures that the UI updates automatically as the underlying data changes. Here’s a Vue example illustrating how to improve user interaction:
export default { data() { return { responses: { favoriteColor: '', serviceFrequency: '' } }; }, methods: { handleSubmit() { console.log('Submitted Responses:', this.responses); alert('Thank you for your submission!'); // Optionally, call an API to send this data } } }Survey
Daily Weekly Monthly
In this Vue.js example, the v-model directive binds input fields directly to the responses object, streamlining data capture while ensuring the UI remains responsive to user input. The handleSubmit method is triggered upon form submission, and like the React example, you can easily extend it to integrate API calls for data submission.
Additionally, enhancing the visual aspects of surveys through animated transitions and feedback can significantly improve user engagement. Libraries like GSAP or Animate.css can be used alongside frameworks to create eye-catching effects that draw users in and keep them invested in the survey process. These effects can be as simple as fading in questions or as complex as animating responses as they’re submitted.
Ultimately, the integration of JavaScript frameworks into online surveys enriches user experience by providing dynamic, responsive, and visually appealing interfaces. The ability to maintain application state effectively, coupled with the ease of integrating animations and API interactions, empowers developers to craft surveys that not only capture valuable data but also enhance user satisfaction and engagement. By focusing on the user experience, developers can create surveys that make participants feel valued and motivated to provide their insights.
Analyzing and Visualizing Survey Results
Analyzing and visualizing survey results is an important step in understanding user feedback and making informed decisions. By using JavaScript’s capabilities, developers can create powerful tools to analyze collected data, derive insights, and present results in a visually appealing manner. This involves not only statistical analysis but also the use of graphs and charts to make data consumption easier for stakeholders.
JavaScript libraries such as Chart.js, D3.js, and Google Charts are particularly effective for visualizing data. These libraries allow developers to create dynamic charts that can respond to user interactions, providing a more engaging experience. For example, Chart.js is a simple yet flexible tool for creating various types of charts that are easy to integrate into web applications. Here’s how you can create a basic bar chart to visualize survey results using Chart.js:
const ctx = document.getElementById('surveyResultsChart').getContext('2d'); const surveyResults = { labels: ['JavaScript', 'Python', 'Java', 'C#'], // Languages from survey datasets: [{ label: 'Favorite Programming Language', data: [30, 20, 25, 15], // Corresponding response counts backgroundColor: ['rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', 'rgba(75, 192, 192, 0.2)'], borderColor: ['rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', 'rgba(75, 192, 192, 1)'], borderWidth: 1 }] }; const surveyResultsChart = new Chart(ctx, { type: 'bar', data: surveyResults, options: { scales: { y: { beginAtZero: true } } } });
This example demonstrates how to set up a bar chart to display the favorite programming languages based on survey responses. The data array in the datasets object represents the number of responses for each programming language. The chart is rendered in a canvas element identified by ‘surveyResultsChart’, which can be added to your HTML as follows:
Once the chart is rendered, users can interact with it to see detailed information about the survey results. This interactivity can include tooltips that display exact values on hover, or drill-down capabilities that allow users to explore more detailed segments of the data.
For a more advanced analysis, you can also incorporate D3.js, which provides a more granular control over the visualization process. D3 allows for the creation of complex, interactive visualizations that can respond to data changes in real-time. Here’s a simple example of creating a pie chart using D3.js:
const data = [30, 20, 25, 15]; const colors = d3.scaleOrdinal(d3.schemeCategory10); const width = 400, height = 400, radius = Math.min(width, height) / 2; const pie = d3.pie(); const arc = d3.arc().innerRadius(0).outerRadius(radius); const svg = d3.select('body') .append('svg') .attr('width', width) .attr('height', height) .append('g') .attr('transform', `translate(${width / 2}, ${height / 2})`); const arcs = svg.selectAll('.arc') .data(pie(data)) .enter() .append('g') .attr('class', 'arc'); arcs.append('path') .attr('d', arc) .style('fill', (d, i) => colors(i));
This D3.js example creates a pie chart representing survey responses. The pie function computes the angles for the different segments of the pie based on the input data. This visualization can also be enhanced with animations and transitions to make it more engaging.
In addition to visual representation, analyzing survey results often involves statistical calculations, such as determining averages, medians, and response distributions. JavaScript can handle these calculations easily with built-in methods or libraries like Math.js, which provides advanced mathematical functions. For example, calculating the average response from an array can be done with:
const responses = [30, 20, 25, 15]; const average = responses.reduce((sum, value) => sum + value, 0) / responses.length; console.log('Average Response:', average);
By combining these analysis techniques with effective visualization tools, developers can provide stakeholders with deep insights into user feedback. This not only enhances the understanding of customer sentiments but also drives strategic decision-making based on data-driven insights. Ultimately, the ability to analyze and visualize survey results empowers organizations to adapt and evolve their services in response to user needs.
Best Practices for Implementing Online Surveys
Implementing online surveys effectively requires a solid understanding of best practices that can enhance user engagement and ensure data integrity. These best practices encompass several areas, including survey design, user experience, data handling, and privacy considerations. By adhering to these guidelines, developers can create surveys that are not only effective in gathering data but also enjoyable for users to complete.
One of the foremost principles in survey design is simplicity. A well-structured survey should avoid convoluted language and complex questions. Instead, using clear and simple phrasing encourages users to provide accurate responses. For instance, when prompting users about their preferences, questions should be straightforward:
const surveyQuestions = [ "What is your preferred method of communication?", "How satisfied are you with our service?" ];
In addition to simplicity, maintaining a logical flow throughout the survey is important. Grouping related questions together can help users navigate the survey more easily. JavaScript can facilitate this by dynamically displaying questions based on previous answers, creating a personalized survey experience. For example:
function showFollowUpQuestion(selectedOption) { if (selectedOption === 'Yes') { document.getElementById('followUpQuestion').style.display = 'block'; } else { document.getElementById('followUpQuestion').style.display = 'none'; } }
In this example, the follow-up question appears only if the user selects ‘Yes’ to a previous question, thus reducing cognitive load and keeping the user engaged.
Another essential best practice is to ensure that your survey is mobile-friendly. With the increasing use of mobile devices for internet access, it is imperative that surveys are accessible and responsive regardless of the device used. Using CSS frameworks like Bootstrap alongside JavaScript can streamline this process:
<div class="form-group"> <label for="email">Email address</label> <input type="email" class="form-control" id="email" placeholder="Enter your email"> </div>
Moreover, using progress indicators can enhance user experience. These indicators inform users about their progress through the survey, thereby reducing abandonment rates. JavaScript can dynamically update a progress bar based on user responses:
function updateProgressBar(currentStep, totalSteps) { const progressBar = (currentStep / totalSteps) * 100; document.getElementById('progressBar').style.width = progressBar + '%'; }
Privacy and data protection are also paramount. Users should be informed about how their data will be used and stored, and consent should be obtained before data collection. Implementing a clear privacy policy and using secure data handling practices will foster trust and encourage more users to participate. For instance, using HTTPS for API calls ensures that data is transmitted securely:
fetch('https://secureapi.com/survey', { method: 'POST', body: JSON.stringify(userResponses), headers: { 'Content-Type': 'application/json', } });
Lastly, testing and iterating on your survey design is vital. Conducting A/B tests to compare different versions of your survey can provide insights into what works best for your target audience. Using analytics tools to track user behavior during the survey can yield valuable data that can inform future improvements.
By adhering to these best practices, developers can create online surveys that not only gather meaningful insights but also provide a positive experience for users. This ultimately leads to higher response rates and more reliable data, empowering organizations to make informed decisions based on user feedback.