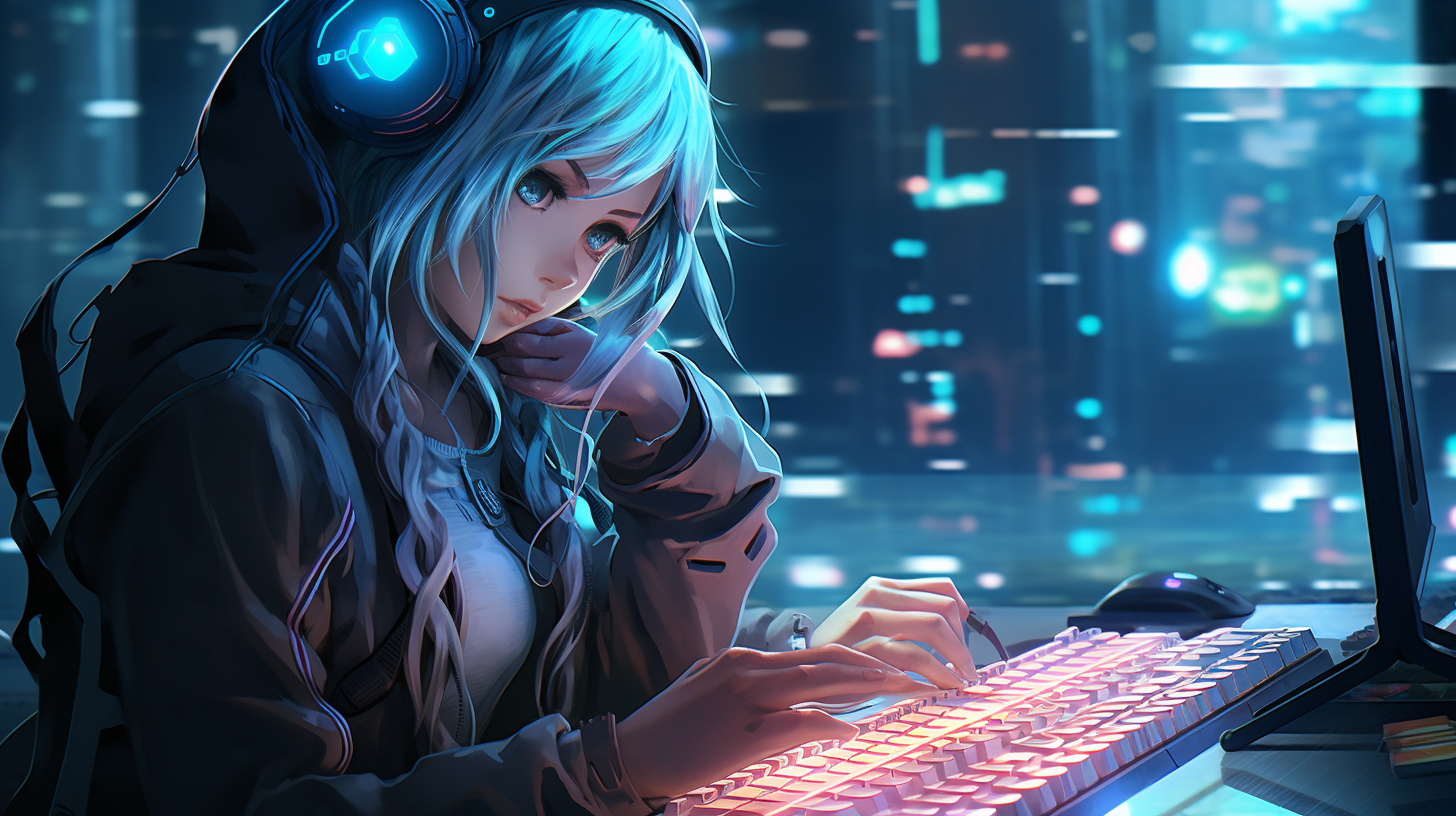
Java Hibernate: ORM Basics
Object-Relational Mapping, or ORM, represents a bridge between the world of object-oriented programming and relational database management systems. In essence, ORM allows developers to interact with a database using Java objects, abstracting away the complexities of raw SQL queries. This paradigm shift not only enhances productivity but also promotes cleaner and more maintainable code.
At the core of ORM is the concept of mapping, where Java classes are represented as database tables, and the attributes of these classes correspond to columns within those tables. This relationship enables developers to manipulate database records as simpler objects, effectively masking the underlying SQL operations.
For example, ponder a simple Java class that represents a Book entity:
public class Book { private Long id; private String title; private String author; // Getters and Setters public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } }
In this scenario, the Book class could map to a books table in the database, where each instance of Book represents a row in that table. The properties of the class, such as title and author, correspond directly to columns in the database.
ORM frameworks like Hibernate take this mapping concept further by providing utilities for managing the lifecycle of these objects. This includes operations such as saving, retrieving, updating, and deleting records from the database without writing explicit SQL code. Instead, developers can use methods provided by the framework to handle these operations, leading to a more streamlined development process.
Ponder the following Hibernate code snippet for saving a Book object to the database:
Session session = sessionFactory.openSession(); Transaction transaction = null; try { transaction = session.beginTransaction(); Book book = new Book(); book.setTitle("Effective Java"); book.setAuthor("Joshua Bloch"); session.save(book); transaction.commit(); } catch (Exception e) { if (transaction != null) { transaction.rollback(); } e.printStackTrace(); } finally { session.close(); }
This code illustrates how Hibernate abstracts the complexities of database interactions. The developer focuses on the Book object and its attributes, while Hibernate manages the SQL generation and execution behind the scenes.
Moreover, ORM enhances portability across various database systems. By using Hibernate’s mapping capabilities, changing the underlying database often requires minimal adjustments to the codebase, as the ORM layer handles many of the differences between database dialects.
Object-Relational Mapping simplifies database interactions and promotes a more object-oriented approach to data management in Java applications. Hibernate, as a powerful ORM framework, provides the tools necessary to implement this paradigm effectively, allowing developers to concentrate on building robust applications without getting bogged down by the intricacies of SQL.
Key Features of Hibernate
Hibernate boasts a myriad of features that elevate it to a preferred choice among Java developers for ORM solutions. These features not only streamline the development process but also enhance application performance and maintainability.
1. Automatic Schema Generation: Hibernate can automatically create and update database schemas based on your entity mappings. This feature is particularly useful during the development phase, allowing developers to focus on coding without worrying about the underlying database structure. By configuring Hibernate’s property hibernate.hbm2ddl.auto
, you can manage schema generation easily.
hibernate.hbm2ddl.auto = update
2. HQL (Hibernate Query Language): Hibernate introduces HQL, an object-oriented query language that allows developers to write queries against the entity model rather than the database tables. HQL supports polymorphic queries and is designed to work seamlessly with the Java object model, making it intuitive for Java developers.
String hql = "FROM Book WHERE author = :authorName"; Query query = session.createQuery(hql); query.setParameter("authorName", "Joshua Bloch"); List results = query.list();
3. Caching Mechanism: Hibernate includes a powerful caching mechanism that can significantly improve performance by minimizing database access. Hibernate supports both first-level and second-level caching. The first-level cache is session-specific and works automatically, while the second-level cache is shared across sessions and can be configured with various caching providers like Ehcache or Infinispan.
4. Lazy Loading: Hibernate uses lazy loading to optimize data retrieval. This means that associated data is not loaded until it’s explicitly accessed, which can enhance performance and reduce unnecessary database queries. This feature is particularly beneficial when dealing with large datasets or complex object graphs.
Book book = session.get(Book.class, bookId); String title = book.getTitle(); // Only now is this book object initialized
5. Support for Inheritance: Hibernate supports various inheritance strategies, allowing developers to map superclass and subclass relationships in a seamless manner. You can use strategies like SINGLE_TABLE
, TABLE_PER_CLASS
, and JOINED
to manage these relationships effectively.
@Entity @Inheritance(strategy = InheritanceType.JOINED) public class Publication { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String title; } @Entity public class Book extends Publication { private String author; }
6. Transaction Management: Hibernate simplifies transaction management by integrating seamlessly with Java Transaction API (JTA) and allowing for programmatic transaction management through the Transaction
interface. This integration ensures that your database operations are performed atomically and can be rolled back in case of errors.
Transaction transaction = session.beginTransaction(); try { // Perform some operations transaction.commit(); // Commit the transaction } catch (Exception e) { transaction.rollback(); // Rollback in case of an error }
7. Event Listeners and Interceptors: Hibernate provides a flexible event listener mechanism that allows developers to intercept and react to various lifecycle events of entities. This feature is useful for implementing custom logic during entity state transitions, such as pre-insert or post-update operations.
8. Multi-Tenancy Support: For applications requiring multi-tenancy, Hibernate provides built-in support that allows a single instance of the application to serve multiple tenants. That’s achieved through various strategies, enabling developers to isolate data for different tenants efficiently.
In summary, the key features of Hibernate reduce boilerplate code, increase productivity, and allow Java developers to focus on business logic rather than the intricacies of database interactions. With its robust capabilities, Hibernate lays a solid foundation for scalable and maintainable Java applications.
Setting Up Hibernate in Your Java Application
Setting up Hibernate in your Java application is a simpler process, which involves configuring the necessary dependencies, creating a configuration file, and initializing the Hibernate session factory. Hibernate’s flexibility allows it to integrate seamlessly into both standalone applications and web applications, making it an ideal choice for a variety of project types.
To begin, you must include Hibernate core and any additional libraries required for your project in your build tool. For Maven users, you can add the following dependencies to your pom.xml
file:
org.hibernate hibernate-core 5.6.9.Final javax.persistence javax.persistence-api 2.2 org.slf4j slf4j-api 1.7.32 org.slf4j slf4j-simple 1.7.32
Once your dependencies are configured, the next step involves creating a hibernate.cfg.xml
configuration file, which contains essential information about the database connection, dialect, and other property settings. This file is typically placed in the src/main/resources
directory. Below is a sample configuration for connecting to a MySQL database:
org.hibernate.dialect.MySQLDialect com.mysql.cj.jdbc.Driver jdbc:mysql://localhost:3306/mydb root password update true true
In this configuration, adjust the hibernate.url
, hibernate.username
, and hibernate.password
properties to match your database credentials. The hibernate.hbm2ddl.auto
property set to update
allows Hibernate to automatically create or update the database schema based on your entity mappings.
With the configuration file in place, you can now initialize the Hibernate SessionFactory
. That’s typically done in a utility class:
import org.hibernate.SessionFactory; import org.hibernate.cfg.Configuration; public class HibernateUtil { private static final SessionFactory sessionFactory = buildSessionFactory(); private static SessionFactory buildSessionFactory() { try { return new Configuration().configure().buildSessionFactory(); } catch (Throwable ex) { System.err.println("Initial SessionFactory creation failed." + ex); throw new ExceptionInInitializerError(ex); } } public static SessionFactory getSessionFactory() { return sessionFactory; } }
After setting up the Hibernate utility class, you can obtain a session from the SessionFactory
and begin using Hibernate to manage your entities. For example:
import org.hibernate.Session; public class Main { public static void main(String[] args) { Session session = HibernateUtil.getSessionFactory().openSession(); try { session.beginTransaction(); // Perform operations with the session session.getTransaction().commit(); } catch (Exception e) { if (session.getTransaction() != null) { session.getTransaction().rollback(); } e.printStackTrace(); } finally { session.close(); } } }
This setup provides a solid foundation for using Hibernate in your Java applications. With Hibernate initialized, you can now focus on implementing your data access logic, confident that the ORM will handle the intricacies of database interactions efficiently and effectively.
Basic CRUD Operations with Hibernate
Performing basic CRUD (Create, Read, Update, Delete) operations with Hibernate is intuitive and allows developers to concentrate on their Java objects rather than the underlying SQL. Each of these operations can be executed using Hibernate’s session management, which takes care of transactional integrity and object state management.
To begin with the Create operation, you simply need to create an instance of your entity class and call the save()
method provided by the Hibernate Session
object. Here’s a sample implementation:
Session session = HibernateUtil.getSessionFactory().openSession(); Transaction transaction = null; try { transaction = session.beginTransaction(); Book book = new Book(); book.setTitle("Clean Code"); book.setAuthor("Robert C. Martin"); session.save(book); // Persist the book instance transaction.commit(); // Commit the transaction } catch (Exception e) { if (transaction != null) { transaction.rollback(); // Rollback in case of an error } e.printStackTrace(); } finally { session.close(); // Ensure session is closed }
Next, for the Read operation, you can retrieve objects by their primary key using the get()
or load()
methods. Here’s how you can fetch a book by its ID:
Long bookId = 1L; Session session = HibernateUtil.getSessionFactory().openSession(); try { Book book = session.get(Book.class, bookId); // Fetch the book object if (book != null) { System.out.println("Book Title: " + book.getTitle()); } else { System.out.println("No book found with ID: " + bookId); } } finally { session.close(); // Ensure session is closed }
For the Update operation, you first retrieve the object you wish to update, modify its properties, and then call the update()
method. Here’s an example:
Long bookId = 1L; Session session = HibernateUtil.getSessionFactory().openSession(); Transaction transaction = null; try { transaction = session.beginTransaction(); Book book = session.get(Book.class, bookId); // Fetch the book if (book != null) { book.setTitle("Clean Code - Updated Edition"); // Update title session.update(book); // Update this book object in the database } transaction.commit(); // Commit the transaction } catch (Exception e) { if (transaction != null) { transaction.rollback(); // Rollback in case of an error } e.printStackTrace(); } finally { session.close(); // Ensure session is closed }
Finally, the Delete operation allows you to remove an object from the database. This can be achieved by calling the delete()
method:
Long bookId = 1L; Session session = HibernateUtil.getSessionFactory().openSession(); Transaction transaction = null; try { transaction = session.beginTransaction(); Book book = session.get(Book.class, bookId); // Fetch this book if (book != null) { session.delete(book); // Remove this book instance from the database } transaction.commit(); // Commit the transaction } catch (Exception e) { if (transaction != null) { transaction.rollback(); // Rollback in case of an error } e.printStackTrace(); } finally { session.close(); // Ensure session is closed }
These operations encapsulate the fundamental interactions with your database using Hibernate. By abstracting away the complexities of SQL statements, Hibernate allows you to focus on your application’s logic, making CRUD operations simpler and efficient.
Advanced Hibernate Concepts and Best Practices
When diving deeper into Hibernate, advanced concepts and best practices become essential for optimizing your application and using the full power of this ORM framework. These practices not only improve performance but also enhance maintainability and scalability. Here are some key advanced concepts and best practices to consider when working with Hibernate.
1. Batch Processing
Batch processing is a technique used to optimize the performance of bulk operations. Instead of executing each operation individually, you can batch multiple operations together, greatly reducing the overhead of database calls. Hibernate allows you to set a batch size, enabling it to send multiple inserts or updates in a single round trip to the database. Here’s how you can implement batch processing:
Session session = HibernateUtil.getSessionFactory().openSession(); Transaction transaction = null; try { transaction = session.beginTransaction(); for (int i = 0; i < 1000; i++) { Book book = new Book(); book.setTitle("Book " + i); book.setAuthor("Author " + i); session.save(book); if (i % 50 == 0) { // Flush and clear every 50 inserts session.flush(); session.clear(); } } transaction.commit(); } catch (Exception e) { if (transaction != null) { transaction.rollback(); } e.printStackTrace(); } finally { session.close(); }
2. Optimistic Locking
In scenarios where multiple users may be trying to update the same data, it’s crucial to manage concurrent modifications correctly. Optimistic locking is a strategy where the application assumes that multiple transactions can complete without affecting each other. You can implement optimistic locking in Hibernate by adding a version field to your entity:
@Entity public class Book { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Version private Long version; // Version field for optimistic locking private String title; private String author; // Getters and Setters }
With this setup, Hibernate checks the version before performing updates. If the version has changed since it was last read, it throws an OptimisticLockException, so that you can handle the conflict appropriately.
3. Connection Pooling
Connection pooling is another best practice that can greatly enhance performance. Instead of opening and closing connections for every database operation, a connection pool maintains a pool of database connections that can be reused. This reduces the overhead associated with connection management. Hibernate works well with connection pool libraries like HikariCP or C3P0. Here’s a simple way to configure HikariCP with Hibernate:
hibernate.hikari.dataSourceClassName = com.zaxxer.hikari.HikariDataSource hibernate.hikari.maximumPoolSize = 10 hibernate.hikari.minimumIdle = 5 hibernate.hikari.idleTimeout = 30000
4. Query Optimization
Using Hibernate’s HQL or Criteria API can be powerful, but it’s essential to optimize your queries for performance. Use projections to fetch only the necessary fields instead of the entire entity when you don’t need the full object. Here’s an example using HQL:
String hql = "SELECT title, author FROM Book WHERE author = :authorName"; Query query = session.createQuery(hql); query.setParameter("authorName", "Joshua Bloch"); List
5. Using Second-Level Cache
Hibernate’s second-level cache can significantly improve your application’s performance by reducing the number of database queries. This cache is shared across sessions and can store entities, collections, and query results. To enable it, you need to configure a caching provider such as Ehcache:
true org.hibernate.cache.ehcache.EhCacheRegionFactory
With second-level caching enabled, entities will be cached after their initial retrieval, preventing repeated database hits for the same data.
6. Profiling and Monitoring
Finally, effective monitoring and profiling are critical for identifying performance bottlenecks. Tools like Hibernate’s statistics feature can help track query execution time and the number of cache hits. You can enable statistics as follows:
SessionFactory sessionFactory = new Configuration() .configure() .buildSessionFactory(); sessionFactory.getStatistics().setStatisticsEnabled(true);
By using these advanced concepts and best practices, you can optimize your Java applications using Hibernate, ensuring they’re not only efficient but also scalable and maintainable. Properly managing entities, optimizing queries, and implementing caching strategies are pivotal steps towards achieving an effective data management strategy.