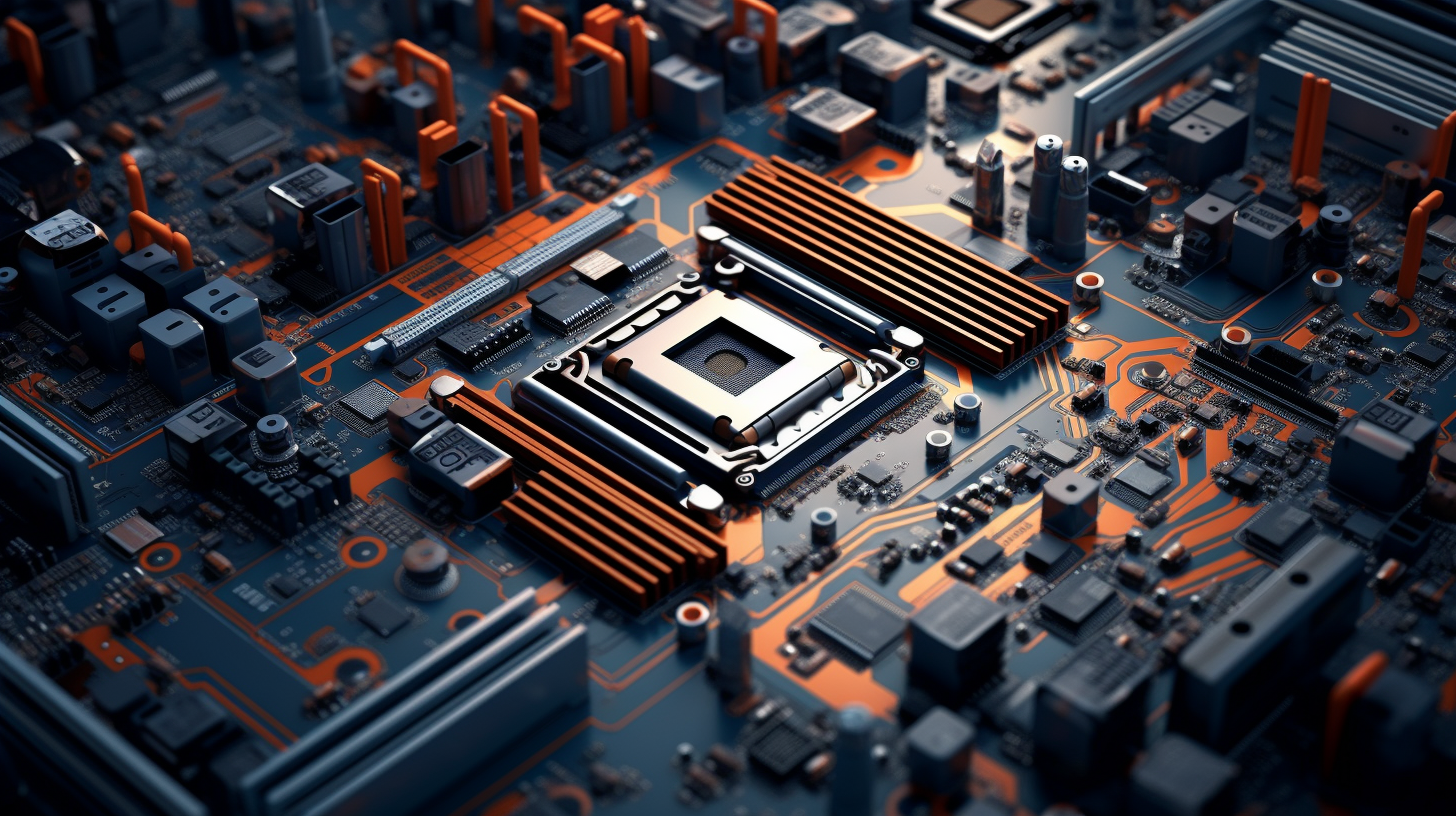
Building Interactive Web Applications with JavaScript
JavaScript is a versatile and powerful language that serves as the backbone of interactive web applications. Understanding the core concepts is essential for any developer venturing into web development. At its heart, JavaScript is an event-driven, functional, and imperative language. It allows developers to create dynamic content, control multimedia, animate images, and much more, all directly in the user’s browser.
One of the fundamental aspects of JavaScript is its ability to manipulate data types, primarily through variables. Variables act as containers for data values. In JavaScript, you can declare variables using var
, let
, or const
. Each has its own scope and usage implications:
// Declaring variables var name = "John"; // function-scoped variable let age = 30; // block-scoped variable const city = "New York"; // immutable block-scoped variable
Another cornerstone of JavaScript is its control structures, which enable you to dictate the flow of the program. You can use if
statements, loops, and switch cases to manage complex logic. Here’s a basic example using an if
statement:
if (age >= 18) { console.log(name + " is an adult."); } else { console.log(name + " is a minor."); }
Functions in JavaScript allow for code reuse and organization. You can define a function using the function
keyword, or you can use arrow functions for a more concise syntax:
// Traditional function function greet() { console.log("Hello, " + name); } // Arrow function const greetArrow = () => { console.log("Hello, " + name); };
JavaScript also embraces the concept of objects and arrays, which are essential for managing complex data. An object is a collection of properties, while an array is an ordered list of values. Here’s an example that combines both:
const person = { name: "John", age: 30, hobbies: ["reading", "traveling", "gaming"] }; console.log(person.name); // Accessing object property console.log(person.hobbies[1]); // Accessing array element
Enhancing User Experience with DOM Manipulation
Enhancing user experience in web applications often hinges on the ability to manipulate the Document Object Model (DOM). The DOM is a representation of the structure of an HTML document, allowing JavaScript to interact with and modify the content and structure of a web page dynamically. Through DOM manipulation, developers can create rich user interfaces that respond to user actions, making applications feel more interactive and engaging.
To begin with, selecting elements from the DOM is an important step. JavaScript provides several methods to identify and select DOM elements, with document.getElementById, document.querySelector, and document.querySelectorAll being the most commonly used. For example:
// Selecting a single element by ID const header = document.getElementById('main-header'); // Selecting the first element that matches a CSS selector const firstItem = document.querySelector('.item'); // Selecting all elements that match a CSS selector const items = document.querySelectorAll('.item');
Once elements are selected, you can manipulate them in various ways. This includes changing their inner content, modifying their styles, or even creating new elements. For instance, if you want to change the text of a header, you can do so using the innerHTML or textContent properties:
// Changing the header text header.textContent = 'Welcome to My Web Application!';
Style adjustments can also be made directly through JavaScript. By accessing the style property of an element, developers can dynamically change CSS attributes:
// Changing the background color and text color header.style.backgroundColor = 'blue'; header.style.color = 'white';
Adding and removing classes is another powerful technique for enhancing user experience. You can use the classList property, which provides methods such as add, remove, and toggle. This allows you to apply or remove CSS styles conditionally:
// Adding a class header.classList.add('highlight'); // Removing a class header.classList.remove('highlight'); // Toggling a class header.classList.toggle('active');
Creating new elements and appending them to the DOM can further enrich user interactions. By using document.createElement and appendChild, developers can craft a more dynamic environment:
// Creating a new paragraph const newParagraph = document.createElement('p'); newParagraph.textContent = 'This is a newly created paragraph.'; // Appending the new paragraph to the body document.body.appendChild(newParagraph);
Event handling is another vital aspect of DOM manipulation. JavaScript allows developers to respond to user actions such as clicks, key presses, and form submissions. By attaching event listeners to DOM elements, you can define what should happen when a specific event occurs:
// Adding a click event listener to a button const button = document.querySelector('.my-button'); button.addEventListener('click', () => { alert('Button clicked!'); });
Implementing Asynchronous Operations with AJAX
Asynchronous operations are crucial in modern web applications, allowing for efficient data retrieval without blocking the user interface. AJAX (Asynchronous JavaScript and XML) is a technique that enables web applications to send and receive data asynchronously, meaning that web pages can request small chunks of data from the server instead of reloading the entire page. This results in a smoother user experience, as users can interact with the application while requests are processed in the background.
At the core of AJAX is the XMLHttpRequest object, which provides a way to send HTTP requests and receive responses from a server. However, with the advent of the Fetch API, developers have a more state-of-the-art and flexible approach to handle asynchronous requests. The Fetch API uses promises to facilitate easier handling of responses. Here is a simple example of how to use the Fetch API to get data from a server:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON from the response
})
.then(data => {
console.log(data); // Handle the data received from the server
})
.catch(error => {
console.error('There was a problem with the fetch operation:', error);
});
In this example, we initiate a GET request to the specified URL. The first `.then()` method checks if the response was successful. If not, it throws an error. If the response is good, we parse it as JSON, and in the next `.then()`, we can handle the data as needed. The `.catch()` at the end allows for error handling in case the request fails.
For more complex interactions, such as sending data to the server, you can use the POST method. Here’s how you can send JSON data using Fetch:
const postData = {
name: "Luke Douglas",
email: "[email protected]"
};
fetch('https://api.example.com/submit', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(postData) // Convert the JavaScript object to a JSON string
})
.then(response => response.json())
.then(data => {
console.log('Success:', data); // Handle the success response
})
.catch(error => {
console.error('Error:', error); // Handle any errors
});
In this example, we create a JavaScript object called `postData` containing the data we want to send. We then call `fetch()` with the URL, specifying the method as ‘POST’, setting the appropriate headers, and including the body as a JSON string. The response is then handled similarly to the previous example.
Asynchronous operations not only improve user experience but can also lead to performance enhancements. By loading data dynamically, applications can reduce the amount of data transferred and minimize the time users spend waiting for responses. That is especially important for mobile users or those on slower connections.
It is important to note that while AJAX facilitates asynchronous communication, developers should always ponder error handling and user feedback. For instance, implementing loading indicators or success messages can help guide users during data retrieval processes. Here’s a simple way to show a loading message while data is being fetched:
const loadingMessage = document.createElement('div');
loadingMessage.textContent = 'Loading...';
document.body.appendChild(loadingMessage);
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
loadingMessage.remove(); // Remove the loading message once data is loaded
})
.catch(error => {
console.error('Error:', error);
loadingMessage.textContent = 'Error loading data.'; // Update message on error
});
In this example, a loading message is displayed while the fetch request is being processed and removed once the data has been successfully retrieved. This practice not only improves user experience but also keeps users informed about the state of the application.
Using JavaScript Frameworks for Efficient Development
In the context of web development, using JavaScript frameworks can significantly enhance the efficiency and effectiveness of building applications. Frameworks like React, Angular, and Vue.js provide structured ways to implement complex functionalities, ultimately improving developer productivity and maintainability of code. These frameworks abstract away many of the mundane tasks associated with DOM manipulation and state management, allowing developers to focus more on the application’s logic and user experience.
One of the key benefits of using a JavaScript framework is the component-based architecture it promotes. This design principle encourages developers to build reusable components, which can lead to more organized and modular code. For instance, in React, a simple button component might look like this:
const Button = ({ label, onClick }) => { return ( <button onClick={onClick}> {label} </button> ); };
Here, the `Button` component can be reused throughout the application with different labels and click handlers, promoting DRY (Don’t Repeat Yourself) principles. This modular approach not only saves time but also enhances code clarity, making it easier to manage and scale projects.
Moreover, frameworks provide powerful state management solutions, allowing developers to handle data and application state efficiently. For example, React has a built-in state management feature using the `useState` hook:
import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); return ( <div> <p>Current count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); };
In this example, the `Counter` component manages its internal state using `useState`. The `setCount` function is called every time the button is clicked, updating the `count` and re-rendering the component with the new value. This reactive data flow is one of the standout features of modern JavaScript frameworks.
Furthermore, routing is another area where frameworks shine. React Router, for instance, allows for easy management of navigation and views within a single-page application (SPA). Here’s a simple example of defining routes:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; const App = () => { return ( <Router> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </Switch> </Router> ); };
This code snippet demonstrates how components can be rendered based on the current URL, making the development of SPAs intuitive and manageable. The `Router` component wraps around the application’s structure, enabling seamless transitions between different views.
JavaScript frameworks also come with an ecosystem of libraries and tools designed to complement their functionality. For instance, state management libraries like Redux or MobX can be integrated into React applications for more complex state management needs. Similarly, Vue has Vuex, which serves the same purpose within its ecosystem.
However, it’s essential to choose the right framework based on the project requirements and your team’s familiarity with the technology. While React is known for its flexibility and component-based architecture, Angular offers a more opinionated structure with built-in support for forms, HTTP requests, and routing. On the other hand, Vue provides a gentle learning curve while still offering powerful features.
Best Practices for Debugging and Optimizing JavaScript Code
When developing web applications, ensuring that your JavaScript code is both functional and optimized is paramount. Debugging and optimizing your code can greatly enhance performance, maintainability, and user experience. There are several best practices that developers should adopt to streamline this process.
Using Console for Debugging
The console is an invaluable tool for debugging JavaScript code. It allows developers to log information, identify errors, and monitor the execution flow of their programs. Using console.log
to output variable states or error messages can provide immediate feedback during development. For example:
console.log('Current value of count:', count);
Moreover, state-of-the-art browsers come equipped with robust developer tools that include breakpoints, watch expressions, and call stacks. This allows you to pause execution at a certain point in your code and inspect the current state of the application, which is essential for diagnosing issues.
Using Try-Catch for Error Handling
Effective error handling especially important in any application. Wrapping potentially problematic code in a try-catch
block allows developers to gracefully handle exceptions and prevent the application from crashing:
try { let result = riskyFunction(); } catch (error) { console.error('An error occurred:', error); }
This approach not only helps catch errors but also provides an opportunity to log meaningful error messages, which can be useful for troubleshooting.
Performance Optimization Techniques
Optimizing JavaScript performance is essential for ensuring smooth interactions within web applications. One common practice is to minimize DOM manipulations. Since interacting with the DOM can be slow, batching updates or using document fragments can yield significant performance improvements:
const fragment = document.createDocumentFragment(); items.forEach(item => { const newItem = document.createElement('li'); newItem.textContent = item; fragment.appendChild(newItem); }); document.querySelector('ul').appendChild(fragment);
Another optimization technique involves debouncing or throttling functions, particularly those linked to events such as scrolling or resizing. This limits the number of times a function is executed within a certain timeframe, thereby reducing strain on the browser:
function debounce(func, delay) { let timeoutId; return function(...args) { if (timeoutId) clearTimeout(timeoutId); timeoutId = setTimeout(() => { func.apply(this, args); }, delay); }; } window.addEventListener('resize', debounce(() => { console.log('Window resized'); }, 300));
Using Proper Data Structures
Choosing the right data structures can also lead to better performance. For instance, using maps or sets can provide faster lookup times compared to arrays when managing collections of data. Understanding the time complexity of operations on various data structures is key to making informed decisions:
const mySet = new Set(); mySet.add('apple'); mySet.add('banana'); console.log(mySet.has('apple')); // true
Minimizing Global Scope Pollution
Global variables can create conflicts and make debugging more challenging. Encapsulating code within functions or using closures can help mitigate this issue. This practice not only keeps the global namespace clean but also enhances code maintainability:
(function() { let privateVar = 'I am private'; console.log(privateVar); })();
Employing Linting Tools
Finally, using linting tools like ESLint can enforce coding standards and identify potential issues before the code is executed. These tools can help catch errors related to syntax and style, thereby improving code quality:
// An example of ESLint warning let x = 10; // Unexpected var, use let or const instead
It is a solid overview, but an important aspect that could enhance the discussion is the importance of understanding how JavaScript handles asynchronous operations beyond just the basics of AJAX and the Fetch API. Incorporating concepts like Promises, async/await, and the event loop would provide a deeper understanding of JavaScript’s concurrency model. This would help developers grasp how to write more efficient and readable asynchronous code, which especially important for modern web applications. Additionally, some examples demonstrating error handling in async functions would be a beneficial inclusion.