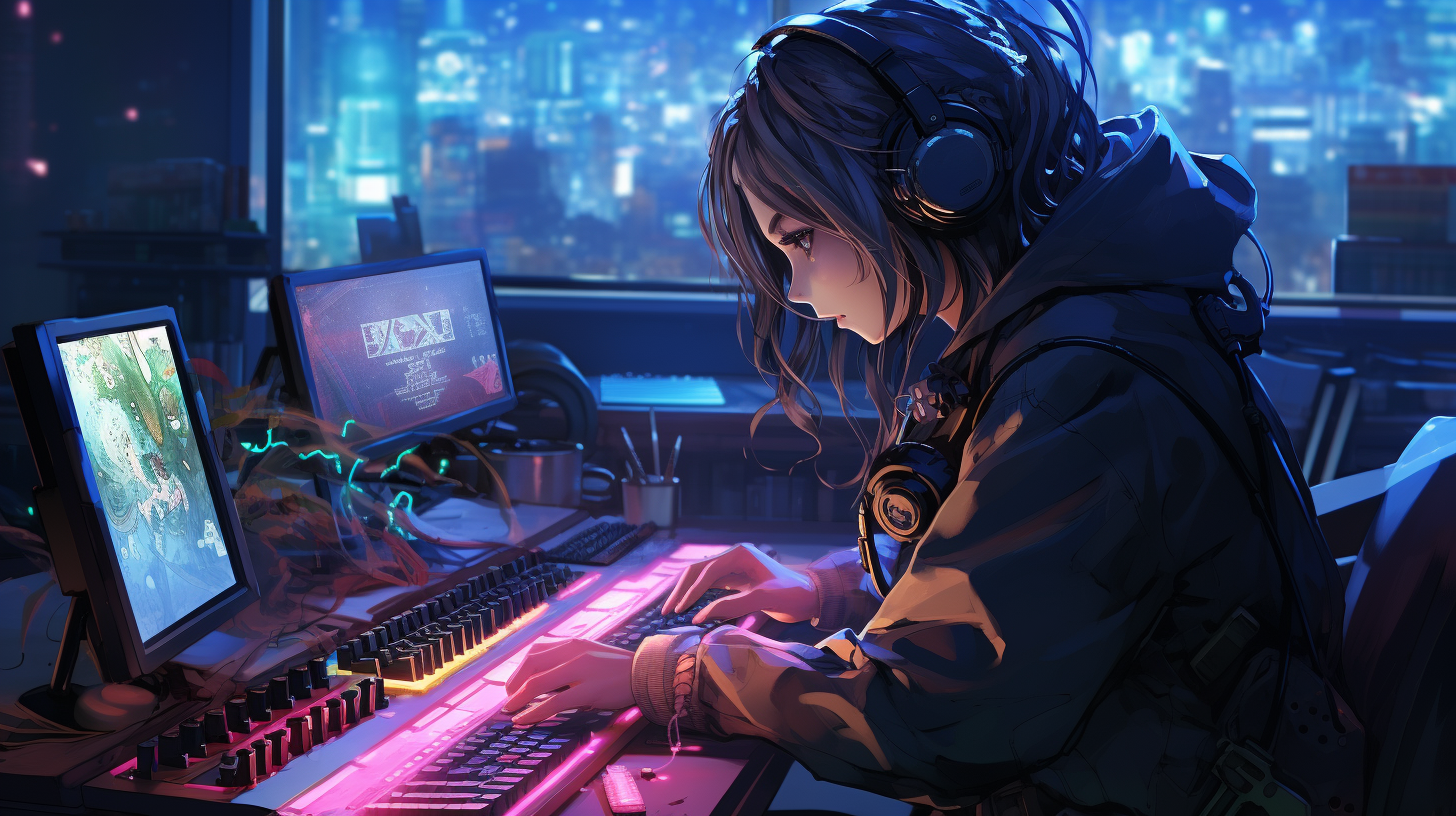
SQL Error Handling with TRY…CATCH
The TRY…CATCH construct in SQL Server is a powerful feature that allows developers to handle exceptions and errors in a controlled manner. This mechanism separates the normal flow of execution from error handling, enabling cleaner and more maintainable code.
When using TRY…CATCH, the code inside the TRY block is executed normally. If an error occurs during the execution of this block, control is transferred to the CATCH block. This transition allows for the capturing of error details and the execution of alternative logic to manage the error, rather than allowing the application to fail abruptly.
The basic syntax of TRY…CATCH is as follows:
BEGIN TRY -- SQL statements for normal processing END TRY BEGIN CATCH -- SQL statements for error handling END CATCH
Here’s a practical example illustrating the use of TRY…CATCH when attempting to insert a record into a table:
BEGIN TRY INSERT INTO Employees (FirstName, LastName, Age) VALUES ('John', 'Doe', 30); END TRY BEGIN CATCH DECLARE @ErrorMessage NVARCHAR(4000); DECLARE @ErrorSeverity INT; DECLARE @ErrorState INT; SELECT @ErrorMessage = ERROR_MESSAGE(), @ErrorSeverity = ERROR_SEVERITY(), @ErrorState = ERROR_STATE(); RAISERROR (@ErrorMessage, @ErrorSeverity, @ErrorState); END CATCH
In this example, if the INSERT operation fails—perhaps due to a constraint violation—the control passes to the CATCH block. Here, the error message is captured using the built-in error functions, which will allow you to log it or raise it again, providing detailed feedback about the error that occurred.
Using TRY…CATCH not only improves the robustness of your SQL code but also enhances the user experience by providing meaningful error messages instead of generic ones. This approach fosters better debugging and issue resolution, making it a vital part of any SQL developer’s toolkit.
Common Errors and Exceptions Handled by TRY…CATCH
In SQL Server, the TRY…CATCH construct is capable of handling a variety of common errors and exceptions that developers are likely to encounter during database operations. Understanding these errors is important for effective error handling and ensuring that applications behave predictably, even when things go awry.
Common errors that can be managed within the TRY…CATCH block include:
1. Syntax Errors: These occur when there is a mistake in the SQL syntax. However, syntax errors are typically caught at compile time rather than at runtime, meaning they won’t trigger the CATCH block. Instead, they need to be corrected before execution.
2. Runtime Errors: These are the errors that occur during the execution of SQL commands. Examples include:
- Division by zero
- Data type conversion errors
- Null reference errors
- Foreign key constraint violations
- Unique constraint violations
For instance, consider an operation that divides a number by another. If the denominator is zero, a runtime error is thrown:
BEGIN TRY DECLARE @result INT; SET @result = 10 / 0; -- This will cause a division by zero error END TRY BEGIN CATCH SELECT ERROR_MESSAGE() AS ErrorMessage; END CATCH
In this example, the division by zero triggers the CATCH block, and the error message is captured and displayed.
3. Deadlocks: A deadlock occurs when two or more processes are waiting for each other to release resources, creating a cycle of dependencies. SQL Server automatically detects deadlocks and terminates one of the processes to resolve the situation. While the deadlock itself cannot be caught directly, the error raised can be handled in the CATCH block:
BEGIN TRY -- Code that may cause a deadlock END TRY BEGIN CATCH IF ERROR_NUMBER() = 1205 -- Deadlock error number BEGIN PRINT 'A deadlock occurred. Please retry the transaction.'; END END CATCH
4. Custom Application Errors: Developers can raise custom errors using the RAISERROR statement, which can be caught in the CATCH block. That is particularly useful for validating business rules or triggering specific handling logic:
BEGIN TRY -- Check for some condition IF (SELECT COUNT(*) FROM Employees) > 100 BEGIN RAISERROR('Employee count exceeds limit of 100.', 16, 1); END END TRY BEGIN CATCH SELECT ERROR_MESSAGE() AS ErrorMessage; END CATCH
By effectively capturing and managing these common errors, SQL developers can create robust applications that handle exceptions gracefully. The implementation of TRY…CATCH is integral to achieving a seamless user experience, as it allows for the identification, reporting, and resolution of errors in a systematic manner.
Implementing Custom Error Messages
Implementing custom error messages within the TRY…CATCH construct can significantly enhance the clarity and usefulness of the feedback provided to users and developers alike. Instead of relying solely on the generic error messages generated by SQL Server, you can create tailored messages that are more descriptive and informative, helping to pinpoint the issue at hand and guiding the user towards a resolution.
To implement custom error messages, you can use the RAISERROR function within the CATCH block. This function allows you to specify a custom message, along with a severity level and state, which can be particularly useful for differentiating between types of errors or for providing specific instructions based on the error context.
Here’s an example of how to implement custom error messages:
BEGIN TRY -- Attempting to insert a new employee record INSERT INTO Employees (FirstName, LastName, Age) VALUES ('Jane', 'Doe', 25); END TRY BEGIN CATCH -- Declare variables to hold error information DECLARE @ErrorMessage NVARCHAR(4000); DECLARE @ErrorSeverity INT; DECLARE @ErrorState INT; -- Capture the error details SELECT @ErrorMessage = ERROR_MESSAGE(), @ErrorSeverity = ERROR_SEVERITY(), @ErrorState = ERROR_STATE(); -- Implementing a custom error message IF @ErrorSeverity >= 16 BEGIN RAISERROR('Custom Error: %s', 16, 1, @ErrorMessage); END ELSE BEGIN PRINT 'Non-critical error: ' + @ErrorMessage; END END CATCH
In the above example, if the INSERT operation fails due to a violation such as a unique constraint, the error details are captured and evaluated. If the error severity is 16 or higher, indicating a serious issue, a custom error message is raised with the original error message embedded within it. This allows developers to have meaningful insights into what went wrong while also being able to display less critical error messages for lower severity errors.
Moreover, integrating custom error messages not only aids in debugging but also enhances the user experience by providing specific guidance on how to resolve the issue. For instance, if an operation fails due to a foreign key constraint violation, the custom error message can inform the user to check the related records, making the troubleshooting process more efficient.
To summarize, the use of custom error messages within the TRY…CATCH structure in SQL Server is a vital practice that empowers developers to convey precise and actionable information regarding errors. This practice ultimately leads to more robust applications and a better experience for users who may encounter issues during database operations.
Best Practices for Error Handling in SQL Queries
When it comes to error handling in SQL queries, adopting best practices plays an important role in ensuring that your code is not only robust but also maintainable. The goal is to create a system where errors are anticipated, caught, and handled in a structured manner, minimizing disruptions in application functionality and improving overall user experience.
One of the best practices is to limit the scope of your TRY…CATCH blocks. It’s advisable to keep the code within the TRY block as compact as possible. This ensures that the error handling is focused and easier to debug. For example, if you have multiple statements, ponder placing them in separate TRY blocks, especially if they’re independent operations:
BEGIN TRY -- First operation INSERT INTO Employees (FirstName, LastName, Age) VALUES ('Alice', 'Smith', 28); END TRY BEGIN CATCH -- Handle error for first operation PRINT 'Error inserting employee: ' + ERROR_MESSAGE(); END CATCH; BEGIN TRY -- Second operation INSERT INTO Departments (DepartmentName) VALUES ('HR'); END TRY BEGIN CATCH -- Handle error for second operation PRINT 'Error inserting department: ' + ERROR_MESSAGE(); END CATCH;
Another important practice involves logging errors for further analysis. Simply displaying an error message may not be enough. Capturing error details in a log table can be invaluable for diagnosing issues after the fact. Here’s how you might implement logging within the CATCH block:
BEGIN TRY -- Operation that may fail DELETE FROM Employees WHERE EmployeeID = 999; -- Assume this ID does not exist END TRY BEGIN CATCH -- Log error details INSERT INTO ErrorLog (ErrorMessage, ErrorTime, ErrorSeverity) VALUES (ERROR_MESSAGE(), GETDATE(), ERROR_SEVERITY()); END CATCH;
Additionally, you should ensure that appropriate error messages are provided. This not only aids developers during debugging but also helps users understand the issues. Avoid vague error messages; instead, provide context and actionable information. Using custom messages that inform users of what went wrong and how to correct it can enhance user satisfaction.
Including a transaction control mechanism is also a vital best practice. If your operations span multiple statements that need to be treated atomically, wrap them in a transaction with COMMIT and ROLLBACK statements. This approach guarantees that your data remains consistent and valid:
BEGIN TRY BEGIN TRANSACTION; INSERT INTO Employees (FirstName, LastName, Age) VALUES ('Bob', 'Jones', 34); INSERT INTO EmployeeDetails (EmployeeID, Address) VALUES (SCOPE_IDENTITY(), '123 Main St'); COMMIT TRANSACTION; END TRY BEGIN CATCH ROLLBACK TRANSACTION; -- Revert all changes on error PRINT 'Transaction failed: ' + ERROR_MESSAGE(); END CATCH;
It’s also wise to avoid using the RAISERROR function to handle errors that are not severe enough to warrant escalated attention. Only raise errors when necessary, as flooding the application with too many error messages can lead to confusion. Instead, let the CATCH block manage non-critical issues gracefully.
Lastly, think implementing a global error handler. By centralizing error management, you can streamline how errors are processed across your application, ensuring consistency and reducing the overhead of repetitive error handling logic.
By following these best practices, SQL developers can create resilient applications capable of gracefully handling errors, thus maintaining smooth operations and a pleasant user experience even in the face of unexpected issues.
The article provides a solid overview of the TRY…CATCH mechanism in SQL Server, but it could expand on the implications of error handling in performance tuning. Specifically, it should mention how excessive use of error handling, particularly in high-frequency code, can lead to performance degradation. Furthermore, explaining the importance of transaction scoping and the potential impact of unhandled exceptions on resource management and locked resources would offer a more comprehensive understanding for developers seeking to optimize their SQL interactions.