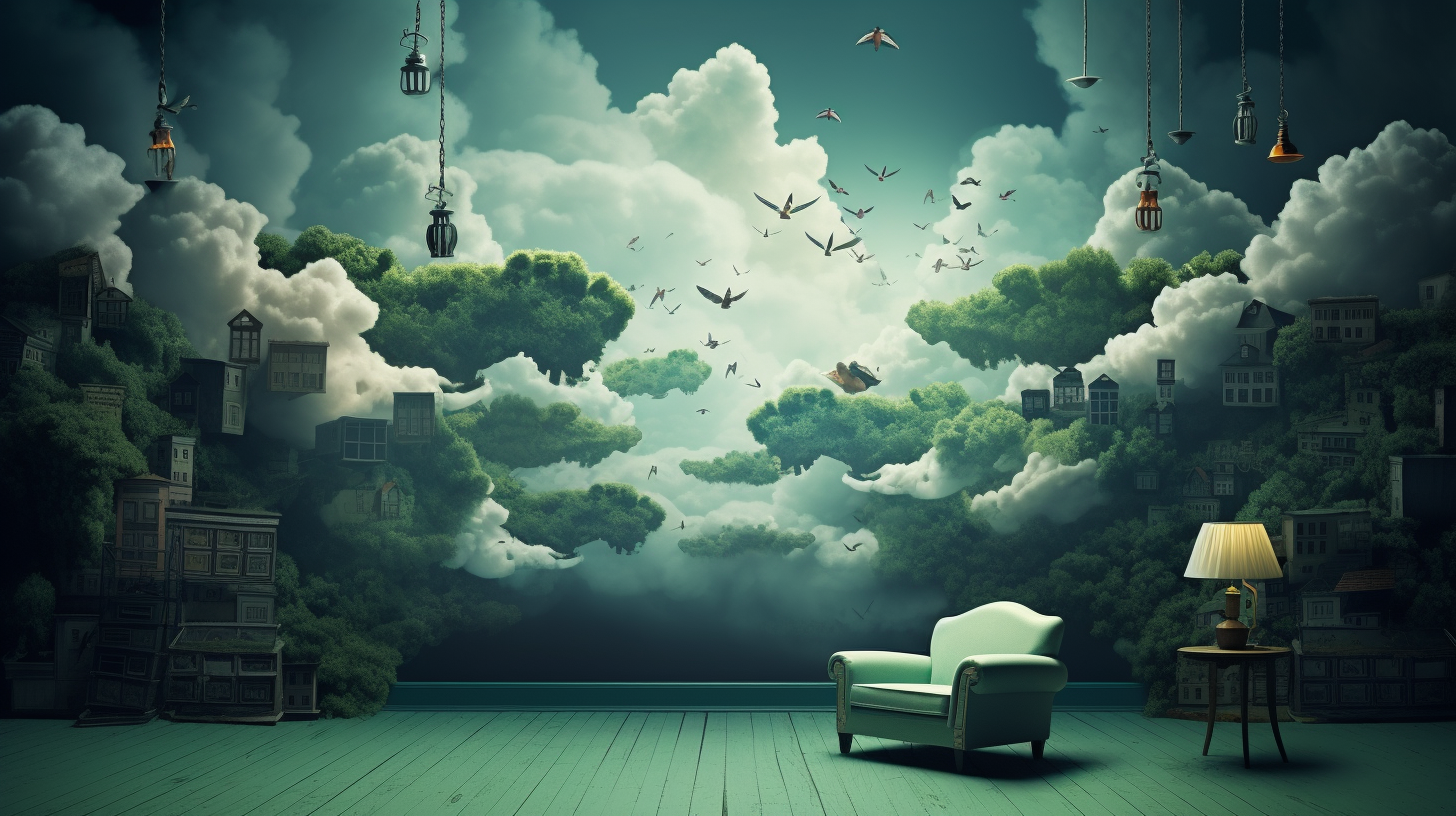
Understanding Java Syntax
Java syntax is a structured framework that dictates how programs written in Java must be organized. Understanding the key components of this syntax is essential for writing efficient and effective Java code. At its core, Java syntax consists of several fundamental elements, including keywords, data types, variables, operators, and control structures.
Keywords are reserved words that have a predefined meaning in the Java language. They cannot be used for any other purpose, such as naming variables or methods. Examples include class
, public
, static
, and void
. These keywords play crucial roles in defining the structure of a Java program.
public class Example { public static void main(String[] args) { // Main method } }
Data types specify the type of data that can be stored in a variable. Java is statically typed, meaning that the type of a variable must be declared before it can be used. The basic data types in Java are:
int
for integersdouble
for floating-point numberschar
for single charactersboolean
for true/false values
Variables are containers for storing data. Each variable in Java must be declared with a specific data type. The declaration consists of the data type followed by the variable name. For example:
int number = 10; double price = 99.99; char letter = 'A';
Operators allow for the manipulation of variables and values. Java supports arithmetic operators like +
, -
, *
, and /
, as well as relational operators like ==
, !=
, and >
. Understanding these operators is vital for performing calculations and comparisons within your program:
int a = 5; int b = 10; int sum = a + b; // sum will hold the value 15 boolean isEqual = (a == b); // isEqual will be false
Control structures, including conditionals and loops, enable you to dictate the flow of execution in your program. The if
statement is perhaps the most common control structure:
if (number > 0) { System.out.println("Number is positive"); } else { System.out.println("Number is non-positive"); }
Additionally, loops such as for
, while
, and do-while
allow you to execute a block of code multiple times:
for (int i = 0; i < 5; i++) { System.out.println("Iteration: " + i); }
Comments in Java are also a key component of syntax. They provide a way to annotate your code and explain logic to future readers, including yourself. There are single-line comments using //
and multi-line comments enclosed within /*
and */
:
// That is a single-line comment /* This is a multi-line comment that spans multiple lines */
Understanding these components is essential for navigating the complexities of Java programming. Each element interacts with others, and mastering them is the first step toward becoming a proficient Java developer.
Data Types and Variables
Data types in Java are categorized into two main groups: primitive types and reference types. Primitive types are the foundational data types provided by the language, while reference types refer to objects and arrays created from classes.
The primitive data types in Java include:
- Used for integers, it can store values ranging from -2,147,483,648 to 2,147,483,647.
- That is used for decimal numbers and can represent fractional values with double precision.
- A single 16-bit Unicode character, enclosed in single quotes.
- Represents a value of either true or false.
Here’s a practical illustration of these primitive types:
int age = 30; double salary = 75000.50; char grade = 'A'; boolean isEmployed = true;
In addition to primitive types, Java allows the creation of reference types through classes. A class can encapsulate data and methods that operate on that data. For instance:
class Employee { String name; int id; Employee(String name, int id) { this.name = name; this.id = id; } } Employee emp1 = new Employee("Vatslav Kowalsky", 12345);
In the example above, the Employee
class is defined with two fields: name
and id
. An object emp1
of this class is created using the new
keyword, which allocates memory for the object.
Variables in Java are not just holders of data; they also have scope and lifetime, determined by where they’re declared. A variable declared inside a method is local to that method, while a variable declared at the class level can be accessed by all methods within that class.
For example, think the following Java program that demonstrates variable scope:
public class ScopeExample { int instanceVariable = 5; // Instance variable public void method() { int localVariable = 10; // Local variable System.out.println("Local Variable: " + localVariable); System.out.println("Instance Variable: " + instanceVariable); } public static void main(String[] args) { ScopeExample example = new ScopeExample(); example.method(); // System.out.println(localVariable); // This would result in a compile-time error } }
In the example above, the local variable localVariable
is only accessible within the method()
. Attempting to access it outside its scope results in a compile-time error.
Java also supports type casting, allowing you to convert one data type to another. That’s particularly useful when performing operations with different types. Implicit casting occurs when converting a smaller type to a larger type, while explicit casting must be done when going from a larger to a smaller type:
int num = 10; double decimalNum = num; // Implicit casting double largeDecimal = 9.78; int smallInt = (int) largeDecimal; // Explicit casting
Understanding data types and how they interact with variables is fundamental to writing robust Java applications. The precision and range of primitive types, combined with the flexibility of reference types, empower Java developers to manage and manipulate data effectively, providing a solid base for building complex systems.
Control Flow Statements
Control flow statements are essential for making decisions in a Java program and determining the execution path based on certain conditions. These statements allow developers to create dynamic and responsive applications that can handle various scenarios. The primary control flow statements in Java include conditional statements such as if, else if, and switch, as well as looping constructs like for, while, and do-while.
The if statement serves as the backbone of decision-making in Java. It evaluates a boolean expression, and if the expression evaluates to true, the block of code within the if statement is executed. If the condition is false, the code inside the block is skipped. Here’s an example:
int score = 85; if (score >= 90) { System.out.println("Grade: A"); } else if (score >= 80) { System.out.println("Grade: B"); } else { System.out.println("Grade: C or lower"); }
In this instance, the program evaluates the value of score
and prints the appropriate grade based on the conditions specified. The else if clause allows for multiple conditions to be checked sequentially. If none of the conditions are met, the else block executes.
The switch statement is another powerful control flow tool, particularly useful when dealing with multiple potential values for a single variable. It checks the value of an expression and executes the corresponding case block. Here’s an example:
int day = 3; switch (day) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; case 3: System.out.println("Wednesday"); break; default: System.out.println("Not a valid day"); }
In this example, the current day is checked using the switch statement. When the value of day
matches a case, that block of code runs. The break
statement very important here, as it prevents the program from falling through to subsequent cases after a match is found.
On the other hand, looping constructs allow for repeated execution of a block of code as long as a specified condition holds true. The most common looping structures are for, while, and do-while.
The for loop is typically used when the number of iterations is known. It consists of three components: initialization, condition, and increment/decrement. Here’s an example:
for (int i = 0; i < 5; i++) { System.out.println("Iteration: " + i); }
This loop will print the value of i
from 0 to 4, executing the code block five times.
The while loop, in contrast, continues executing as long as the specified condition remains true. This type of loop is useful when the number of iterations is not predetermined. For example:
int count = 0; while (count < 5) { System.out.println("Count: " + count); count++; }
In this example, the loop runs until count
reaches 5, effectively printing the current count on each iteration.
The do-while loop is similar to the while loop, but it guarantees that the block of code will be executed at least once, as the condition is checked after the execution. Here’s how it looks:
int number = 0; do { System.out.println("Number: " + number); number++; } while (number < 5);
Regardless of the condition, the code inside the do block will execute a minimum of once, making it particularly useful in scenarios where the initial action must occur before any conditions are evaluated.
Understanding these control flow statements equips developers with the necessary tools to build complex logical structures within their applications. Mastery of these constructs allows for the creation of sophisticated and responsive programs that can adapt to a wide range of inputs and conditions, ensuring a more dynamic user experience.
Methods and Function Definitions
In Java, methods are the cornerstone of functionality and code organization. They encapsulate behavior and provide a structured way to perform operations on data. A method in Java consists of a name, a return type, parameters, and a body, which contains the code that defines what the method does. The syntax for defining a method is simpler and follows a consistent pattern.
A typical method declaration begins with the access modifier, followed by the return type, method name, and parentheses that may include parameters. The general syntax looks like this:
accessModifier returnType methodName(parameterType parameterName) { // method body }
For example, ponder a method that calculates the square of a number:
public int square(int number) { return number * number; }
In this example, the method square
is declared with the access modifier public
, meaning it can be accessed from other classes. The return type is int
, indicating that it will return an integer value. The method takes one parameter of type int
, named number
.
When you want to call this method, you can do so by specifying the method name with the appropriate arguments. For instance:
int result = square(5); System.out.println("Square: " + result);
Methods can also have multiple parameters. Here’s an example of a method that adds two numbers:
public int add(int a, int b) { return a + b; }
You can invoke this method as follows:
int sum = add(10, 20); System.out.println("Sum: " + sum);
Java also supports method overloading, which allows multiple methods to have the same name but different parameter lists. This feature enhances code readability and allows for flexibility in method usages. For instance:
public int add(int a, int b) { return a + b; } public double add(double a, double b) { return a + b; }
In this case, both methods named add
are defined, one for integers and another for doubles. The Java compiler determines which method to invoke based on the argument types provided.
In addition to return types, methods can also be void, indicating that they do not return a value. Such methods are typically used for performing actions rather than computing a value. For instance:
public void printGreeting(String name) { System.out.println("Hello, " + name + "!"); }
You can call the printGreeting
method like this:
printGreeting("Alice");
Method definitions can also include variable-length arguments using the varargs feature. This allows you to pass a variable number of arguments to a method. The syntax for varargs is as follows:
public void displayNumbers(int... numbers) { for (int number : numbers) { System.out.println(number); } }
When invoking the displayNumbers
method, you can pass any number of integers:
displayNumbers(1, 2, 3, 4, 5);
This flexibility makes methods powerful tools for creating reusable code blocks. Understanding how to define and utilize methods effectively especially important for building well-structured Java applications. It promotes code modularity and aids in maintaining and debugging code more efficiently, ultimately enhancing the developer’s productivity.
Object-Oriented Principles in Java
Object-oriented programming (OOP) forms the backbone of Java, providing a paradigm that models real-world entities through the use of classes and objects. This approach emphasizes the encapsulation of data and behavior, inheritance of properties, and polymorphism, all of which are fundamental principles in Java. Understanding these principles is essential for writing modular and reusable code.
At the heart of OOP in Java is the idea of a class. A class serves as a blueprint for creating objects, encapsulating both data (attributes) and methods (functions) that operate on that data. For instance, think the following class definition for a simple Car object:
class Car { // Attributes String color; String model; int year; // Constructor Car(String color, String model, int year) { this.color = color; this.model = model; this.year = year; } // Method void displayInfo() { System.out.println("Car Model: " + model + ", Color: " + color + ", Year: " + year); } }
In the Car class, the attributes represent the state of the object, while the displayInfo method provides behavior. The constructor initializes the Car object with specified values. To create an instance of Car and utilize its methods, you would do the following:
public class Main { public static void main(String[] args) { Car myCar = new Car("Red", "Toyota", 2021); myCar.displayInfo(); } }
In this example, the myCar object is instantiated using the Car constructor, and the displayInfo method is called to print its details.
Inheritance is another critical aspect of OOP in Java, allowing a new class to inherit attributes and methods from an existing class. This promotes code reuse and establishes a hierarchical relationship between classes. For example, if we wanted to create a subclass called ElectricCar that extends the Car class, it could look like this:
class ElectricCar extends Car { int batteryCapacity; ElectricCar(String color, String model, int year, int batteryCapacity) { super(color, model, year); // Call to the parent class constructor this.batteryCapacity = batteryCapacity; } void displayInfo() { super.displayInfo(); // Call to the parent class method System.out.println("Battery Capacity: " + batteryCapacity + " kWh"); } }
In this code, the ElectricCar class inherits from the Car class. It adds a new attribute, batteryCapacity, and overrides the displayInfo method to include this new information. The use of the super
keyword allows access to the parent class’s constructor and methods.
Polymorphism, the ability for different classes to be treated as instances of the same class through a common interface, adds further flexibility to Java programming. This can be achieved through method overriding and interfaces. Here’s an example demonstrating method overriding:
class SportsCar extends Car { SportsCar(String color, String model, int year) { super(color, model, year); } void displayInfo() { System.out.println("This is a sports car!"); super.displayInfo(); } }
In this situation, the SportsCar class overrides the displayInfo method to provide specific details for sports cars. When an object of SportsCar is created and the method is called, it will execute the overridden version:
SportsCar mySportsCar = new SportsCar("Blue", "Ferrari", 2022); mySportsCar.displayInfo();
Encapsulation, another OOP principle, ensures that an object’s internal state is hidden from outside interference and misuse. That is typically accomplished by declaring attributes as private and providing public getter and setter methods to access and modify them. This approach allows for controlled access to the data while maintaining data integrity.
class Person { private String name; private int age; // Getter public String getName() { return name; } // Setter public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age > 0) { // Basic validation this.age = age; } } }
This Person class encapsulates the name and age attributes, allowing controlled access through its getter and setter methods. It also performs simple validation in the setAge method to ensure that the age remains a positive value.
By embracing the principles of object-oriented programming, Java developers can create highly organized, modular, and maintainable code. Mastery of these concepts empowers programmers to design robust applications that are adaptable to future needs and challenges, fostering a more efficient and effective coding experience.
Common Syntax Errors and Debugging Tips
Common syntax errors in Java can be a source of frustration, particularly for those new to the language. Understanding these errors is important for rapid debugging and for writing efficient, error-free code. One of the most prevalent syntax errors is the missing semicolon at the end of a statement. In Java, each statement must end with a semicolon. Neglecting to do so results in a compilation error. For example:
int number = 10 // Missing semicolon System.out.println(number);
In this case, the compiler will throw an error indicating that it expected a semicolon at the end of the first line.
Another common mistake is mismatched parentheses or braces. Java uses parentheses for method calls and conditionals, and braces for defining code blocks. It is essential that these are balanced; otherwise, it will lead to compilation errors. For instance:
if (number > 0 { // Mismatched parentheses System.out.println("Positive Number"); }
In this example, the opening parenthesis is not properly closed, which results in a syntax error.
Variable declaration and initialization also play a significant role in avoiding syntax errors. Declaring a variable without specifying its type or using an undeclared variable will lead to compilation issues. For instance:
String name; System.out.println(name); // Error: Variable name might not have been initialized
Notice how in this snippet, name is declared but never initialized, leading to a potential error when it’s accessed.
Control flow statements, including if-else and loops, require that the conditions they evaluate are boolean expressions. Using non-boolean conditions will also result in an error. For example:
int a = 5; if (a) { // Incorrect use of non-boolean condition System.out.println("This won't work"); }
In this case, the compiler cannot evaluate the condition since ‘a’ is an integer, not a boolean expression.
Debugging syntax errors in Java can be made easier by using an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse, which provide real-time syntax checking and suggestions for corrections. Compilers will often provide line numbers where errors occur, giving you a good starting point for troubleshooting.
Additionally, employing systematic code reviews and keeping code organized can significantly reduce the occurrence of syntax errors. Commenting out sections of code when introducing changes can also help identify the source of errors more easily.
By familiarizing yourself with common syntax errors and using the tools at your disposal, you can streamline the debugging process and enhance your overall coding experience in Java.
It’s a solid walkthrough on Java syntax. However, one critical aspect that could enhance the discussion is the importance of understanding Java’s exception handling mechanisms. Exception handling plays a vital role in writing robust applications, as it allows developers to manage runtime errors gracefully and maintain program stability. Including a section on try-catch blocks, the `finally` clause, and custom exceptions would provide a more comprehensive picture of effective Java programming practices.