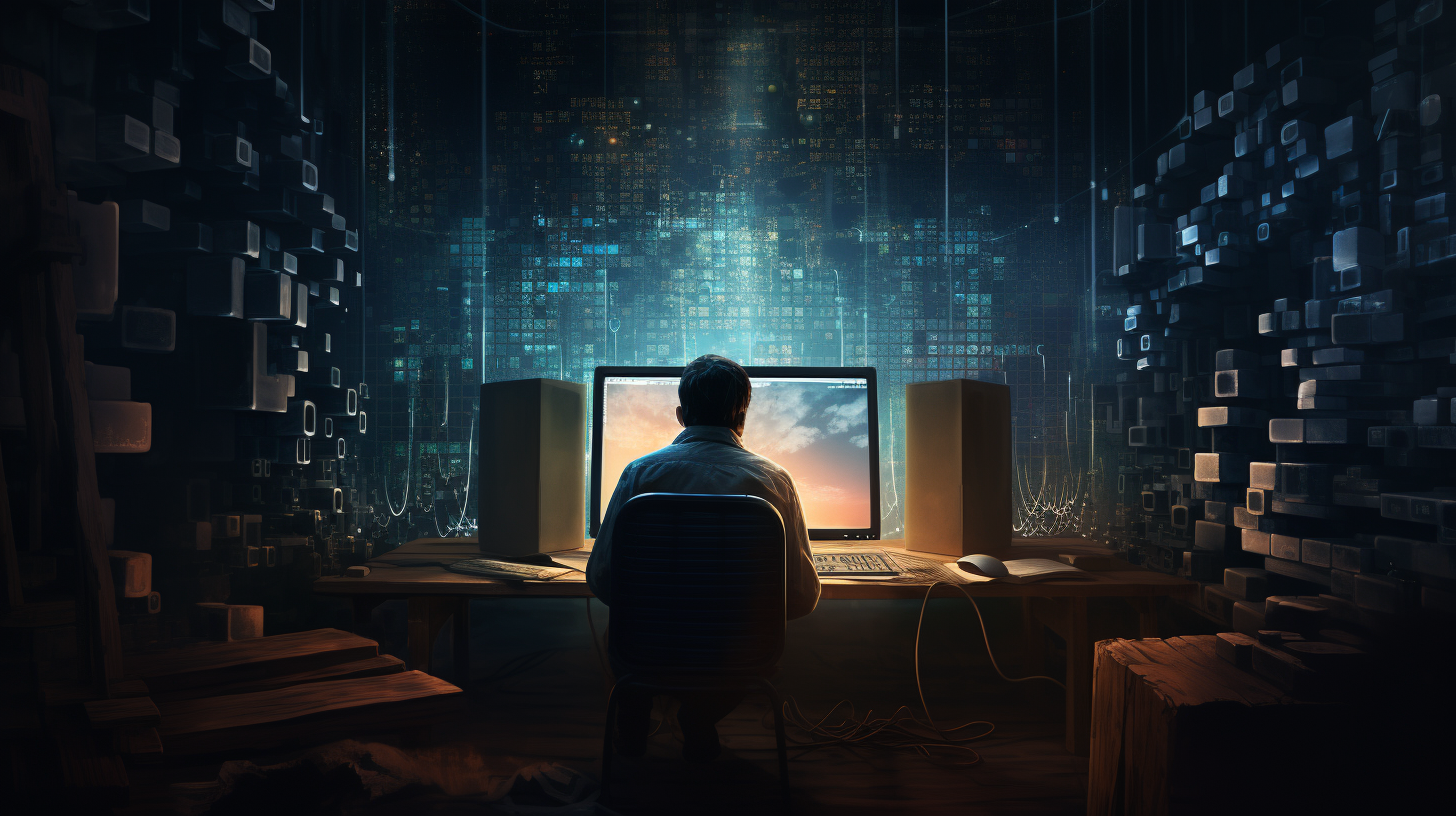
JavaScript for Interactive Storytelling
JavaScript is a versatile language that breathes life into static web pages, transforming them into immersive interactive experiences. When it comes to interactive storytelling, understanding the basics of JavaScript is important. It allows creators to manipulate the Document Object Model (DOM), respond to user inputs, and control the flow of narratives dynamically.
At its core, JavaScript enables developers to define behaviors of characters, transitions between scenes, and the overall pacing of the story. By using functions, events, and objects, storytellers can weave complex interactions that respond to user engagement.
To get started, familiarize yourself with the basic syntax and structures of JavaScript. Here’s an example of a simple function that changes a character’s dialogue when the user clicks a button:
function changeDialogue() { const dialogueElement = document.getElementById('characterDialogue'); dialogueElement.innerText = 'Hello, traveler! What brings you here?'; } document.getElementById('nextButton').addEventListener('click', changeDialogue);
In this snippet, we define a function called changeDialogue that modifies the inner text of a paragraph element to reflect the character’s speech. The event listener attached to a button allows the user to trigger the dialogue change, creating an interactive element that propels the story forward.
Variables and data types are fundamental in JavaScript, enabling you to store and manipulate information. For instance, you could create an object to represent a character:
const character = { name: 'Elysia', age: 27, dialogue: 'Welcome to the realm of magic!', speak: function() { console.log(this.dialogue); } };
Here, we have defined a character object containing attributes and a method. The speak method utilizes the this keyword to access the character’s dialogue, demonstrating how objects can encapsulate behavior and properties.
Control structures like loops and conditionals are also essential. They help dictate story paths based on user choices. For example, a simple conditional statement could dictate which path the narrative takes based on a user’s response:
const userChoice = 'fight'; if (userChoice === 'fight') { console.log('You prepare for battle!'); } else { console.log('You choose to run away.'); }
Creating Interactive Characters and Dialogues
To create truly interactive characters and dialogues in JavaScript, you’ll want to leverage the power of event-driven programming alongside object-oriented design. By representing characters as objects and using methods to dictate their behavior, you can make each interaction feel unique and responsive to user actions.
Consider extending our character model with multiple dialogues and choices. This can be achieved by adding an array of dialogue options for our character and a method to handle user choices:
const character = { name: 'Elysia', age: 27, dialogues: [ 'Welcome to the realm of magic!', 'Beware, not all who wander are lost.', 'Do you seek adventure or knowledge?' ], currentDialogue: 0, speak: function() { console.log(this.dialogues[this.currentDialogue]); }, nextDialogue: function() { this.currentDialogue = (this.currentDialogue + 1) % this.dialogues.length; this.speak(); } };
In this code, we have added an array called dialogues to store multiple lines of dialogue. The method nextDialogue cycles through the dialogues, allowing for a more dynamic interaction. Each time a user clicks a button, the character can speak a new line, enhancing the storytelling experience.
Next, let’s implement event listeners that facilitate user interactions, allowing them to choose how they want to engage with the character. For example, we can provide options for the user to respond:
document.getElementById('nextButton').addEventListener('click', () => { character.nextDialogue(); }); document.getElementById('choiceButton').addEventListener('click', (event) => { const userResponse = event.target.dataset.response; if (userResponse === 'adventure') { console.log('You chose adventure! Let the journey begin.'); } else if (userResponse === 'knowledge') { console.log('You seek knowledge! The ancient texts await.'); } });
Here, we add an event listener to a button that cycles through the character’s dialogues. Another button listens for user responses that determine the story path. Using data attributes, we can easily identify the user’s choice and react accordingly.
By implementing these interactive elements, you can create a rich tapestry of dialogue where every user interaction influences the narrative. This not only heightens engagement but also allows for a more personalized storytelling experience. You can take this further by expanding the dialogue system to include more complex branching paths based on user choices, leading to different endings or character developments.
Building Dynamic Story Paths with Conditional Logic
Building dynamic story paths in your interactive narrative requires a solid understanding of conditional logic. By using JavaScript’s control flow constructs, you can create a branching narrative that responds to user actions, shaping the story in real-time based on their decisions. This allows for a richer user experience, where every choice feels impactful and consequential.
Conditional statements, particularly if, else if, and else, are your allies in managing different story outcomes. Consider a scenario where the user must choose between multiple quests. Each choice can lead to a distinct path in your story:
const questChoice = prompt("Choose your quest: 'dragon' or 'treasure'"); if (questChoice === 'dragon') { console.log('You prepare to face the fearsome dragon!'); } else if (questChoice === 'treasure') { console.log('You venture forth in search of hidden treasure!'); } else { console.log('Invalid choice! Please select either "dragon" or "treasure".'); }
In this example, the user’s input determines which narrative path they will take. This approach can be further enhanced by creating functions for each quest, encapsulating the logic and making your code cleaner and more manageable:
function startDragonQuest() { console.log('You approach the dragon's lair, heart pounding.'); // Additional logic for the dragon quest } function startTreasureQuest() { console.log('You navigate through the dense forest towards the treasure.'); // Additional logic for the treasure quest } if (questChoice === 'dragon') { startDragonQuest(); } else if (questChoice === 'treasure') { startTreasureQuest(); } else { console.log('Invalid choice! Please select either "dragon" or "treasure".'); }
As your storytelling grows more intricate, you might introduce an array of choices that can further complicate the narrative. Using nested conditionals, you can allow for deeper branching paths based on previous decisions:
const questChoice = prompt("Choose your quest: 'dragon', 'treasure', or 'puzzle'"); if (questChoice === 'dragon') { const actionChoice = prompt("Do you want to 'fight' or 'flee'?"); if (actionChoice === 'fight') { console.log('You bravely battle the dragon!'); } else if (actionChoice === 'flee') { console.log('You retreat to safety, heart racing.'); } } else if (questChoice === 'treasure') { const treasureChoice = prompt("Do you want to 'dig' or 'search'?"); if (treasureChoice === 'dig') { console.log('You start digging and uncover a chest!'); } else if (treasureChoice === 'search') { console.log('You search the area and find a hidden map!'); } } else if (questChoice === 'puzzle') { console.log('You encounter a mystical puzzle that guards the secrets of the land.'); } else { console.log('Invalid choice! Please choose a valid quest.'); }
This code snippet illustrates how each decision can lead to different subsequent choices, crafting a more engaging and complex storyline. By layering conditional statements, you create a web of possibilities that the user can navigate, significantly enhancing their investment in the narrative.
Moreover, think using arrays or objects to manage the conditions and outcomes—this allows for easier updates and expansions as your story evolves. For example, you could maintain an object mapping quests to their corresponding functions:
const quests = { dragon: startDragonQuest, treasure: startTreasureQuest, puzzle: () => console.log('You encounter a mystical puzzle that guards the secrets of the land.') }; const questChoice = prompt("Choose your quest: 'dragon', 'treasure', or 'puzzle'"); if (quests[questChoice]) { quests[questChoice](); } else { console.log('Invalid choice! Please choose a valid quest.'); }
Enhancing Engagement with Multimedia Elements
To elevate the user experience in interactive storytelling, integrating multimedia elements is pivotal. JavaScript can seamlessly interact with various forms of media—images, audio, and video—enriching the narrative and immersing users in the story. By enhancing the visual and auditory aspects, you can create a more engaging environment that captivates the audience and deepens their emotional connection to the narrative.
First, let’s explore how to incorporate images that correspond with the storyline. Using JavaScript, you can dynamically change images based on user choices or narrative progression. For instance, ponder a scenario where different scenes are depicted through images, and the image changes based on user decisions:
const scenes = { start: 'images/start.jpg', forest: 'images/forest.jpg', castle: 'images/castle.jpg' }; function changeScene(scene) { const sceneElement = document.getElementById('sceneImage'); sceneElement.src = scenes[scene]; } // Example usage: document.getElementById('forestButton').addEventListener('click', () => changeScene('forest')); document.getElementById('castleButton').addEventListener('click', () => changeScene('castle'));
In this example, we define an object called scenes that holds the paths to different images representing various story locations. The changeScene function dynamically updates the source of an img element based on the current scene, effectively transporting the user to different parts of the narrative through visuals. This approach can significantly enhance storytelling by visually contextualizing user choices.
Next, sound effects and background music can further enrich the immersive experience. JavaScript’s Web Audio API allows for complex audio manipulations, but for simpler implementations, the HTML5 audio element is often sufficient. By playing audio clips based on narrative events, you can evoke emotions and set the atmosphere:
const audio = new Audio('audio/background-music.mp3'); function playBackgroundMusic() { audio.loop = true; audio.play(); } function stopBackgroundMusic() { audio.pause(); audio.currentTime = 0; // Reset to the beginning } // Start the music when the story begins playBackgroundMusic(); // Stop the music when the user makes a choice document.getElementById('choiceButton').addEventListener('click', stopBackgroundMusic);
This code snippet demonstrates how to initiate background music that loops throughout the storytelling experience. You can easily modify the audio source based on different scenes or user interactions to create a dynamic soundscape that complements the narrative.
To take interactivity a step further, ponder using video clips that can highlight key moments in the story. By embedding video elements, you can visually represent pivotal scenes that enhance the emotional weight of the narrative:
function showVideo(videoSrc) { const videoElement = document.getElementById('storyVideo'); videoElement.src = videoSrc; videoElement.play(); } // Example usage document.getElementById('videoButton').addEventListener('click', () => { showVideo('videos/key_moment.mp4'); });
In this example, the showVideo function changes the source of a video element and plays it, allowing for a cinematic experience that can be triggered by user actions. This adds another layer of storytelling, enabling users to experience the narrative in a more dynamic way.
Incorporating multimedia elements requires careful consideration of timing and user controls. You want to ensure that media does not distract or overwhelm the user but rather enhances their journey through the story. Balancing these elements is key; for each multimedia asset, ask how it serves the narrative and how it can engage users without detracting from the interactive experience.
Implementing User Feedback and Choices
Implementing user feedback and choices is a cornerstone of interactive storytelling in JavaScript. The essence of engaging narratives lies in how effectively they respond to the reader’s actions, allowing users to feel a sense of agency in shaping the outcome of the story. By collecting user input and implementing it into the narrative flow, you can create a rich, personalized experience that resonates deeply with your audience.
To utilize user feedback, you can leverage various input methods such as prompts, forms, and buttons. For instance, using a simple prompt can set the stage for initial user engagement:
const userName = prompt("What is your name, brave adventurer?");
This single line of code invites users to enter their name, which can subsequently be incorporated into the narrative to create a more intimate storyline:
console.log(`Welcome, ${userName}, to the world of adventure!`);
Next, consider allowing users to make choices that drive the narrative forward. A simple structure involving buttons can facilitate this interaction. For example, you could present users with a series of choices, each leading to a different narrative path:
document.getElementById('choice1').addEventListener('click', () => { console.log('You have chosen to enter the dark forest.'); }); document.getElementById('choice2').addEventListener('click', () => { console.log('You decide to climb the mountain instead.'); });
In this code, two buttons are set up with event listeners that log different statements depending on the user’s selection. This can significantly enhance the storytelling experience by allowing users to influence the story’s direction based on their choices.
Moreover, you can introduce more complex decision-making scenarios by storing the user’s choices in variables or objects. This way, you can reference past decisions later in the narrative, creating a cohesive and intertwined story:
const userChoices = { journeyStart: null, encounter: null }; document.getElementById('forestButton').addEventListener('click', () => { userChoices.journeyStart = 'forest'; console.log('You have entered the forest.'); }); document.getElementById('mountainButton').addEventListener('click', () => { userChoices.journeyStart = 'mountain'; console.log('You have chosen the mountain.'); });
As the story evolves, you can build upon the user’s previous choices. For instance, if they initially chose to enter the forest, you might provide narrative options that are unique to that path:
if (userChoices.journeyStart === 'forest') { console.log('As you venture deeper, you hear a rustling sound. What do you do?'); } else if (userChoices.journeyStart === 'mountain') { console.log('The wind howls as you scale the rocky terrain. What’s your next move?'); }
This structure not only enhances the narrative depth but also makes the user feel that their decisions are consequential, impacting the unfolding story. Each choice paves the way for unique interactions, developing a more intricate web of possibilities.
Additionally, providing feedback on user actions very important. Consider displaying messages or updating the interface to reflect the consequences of their choices. This could involve changing sections of a story or updating visible elements on the page:
function displayMessage(message) { const messageElement = document.getElementById('storyMessage'); messageElement.innerText = message; } // Usage example document.getElementById('choice1').addEventListener('click', () => { displayMessage('You bravely peek into the dark forest.'); });
Incorporating such feedback loops not only informs the user of their current status within the narrative but also reinforces their sense of agency. By clearly articulating the outcomes of their choices, you heighten engagement and investment in the story.
Lastly, remember to allow for flexibility in user input. The more varied the options you provide, the richer the storytelling will become. Use arrays or objects to manage different paths and outcomes, and implement randomization or unexpected events to keep the narrative fresh and exciting:
const unexpectedEvents = [ 'A sudden storm erupts!', 'You stumble upon a hidden treasure!', 'A wise old sage appears before you.' ]; const randomEvent = unexpectedEvents[Math.floor(Math.random() * unexpectedEvents.length)]; console.log(randomEvent);
This code snippet randomly selects an unexpected event to incorporate into the narrative, adding an element of surprise and spontaneity that can lead to unique storytelling experiences each time the user engages.
Case Studies: Successful Interactive Storytelling Projects
const caseStudies = [ { title: "Zelda: Breath of the Wild", description: "This game utilizes an open-world format where player choices significantly impact the game's narrative and exploration. The dynamic weather system and various character interactions allow users to experience different outcomes based on their decisions." }, { title: "80 Days", description: "An interactive novel where players take on the role of Phileas Fogg, making choices that affect the route taken around the world. The game features a rich narrative with multiple endings based on the player's decisions." }, { title: "Life is Strange", description: "This episodic graphic adventure game allows players to rewind time and alter the choices they've made. The consequences of these choices ripple through the narrative, leading to various endings and character developments." }, { title: "The Stanley Parable", description: "A first-person exploration game that plays with narrative structure and player choice. The game features multiple endings based on the player's decisions, often providing humorous and thought-provoking commentary on the nature of choice in games." } ]; caseStudies.forEach(study => { console.log(`Title: ${study.title}`); console.log(`Description: ${study.description}`); });
Examining successful interactive storytelling projects reveals the profound impact that JavaScript and user interactivity can have on narrative experiences. Each case study illustrates unique ways in which developers have leveraged user choices, multimedia elements, and innovative programming techniques to create engaging, dynamic stories.
In “Zelda: Breath of the Wild,” players navigate an expansive world filled with choices that affect not only their immediate encounters but the overarching narrative as well. The game’s environment reacts to player decisions, fostering a sense of agency. Weather conditions can change gameplay mechanics, while NPC interactions can lead to multiple outcomes, showcasing how interactivity enriches the storytelling experience.
“80 Days” offers a fascinating exploration of narrative branching. Players are tasked with circumnavigating the globe within a set time limit, making critical decisions along the way. Each choice affects the route taken and the events encountered, leading to a high number of possible endings. This game exemplifies how JavaScript can effectively manage and track player choices, crafting a narrative that feels personalized and impactful.
“Life is Strange” stands out for its use of time manipulation as a gameplay mechanic. Players are faced with choices that lead to different outcomes, but the ability to rewind time allows them to explore consequences dynamically. This intricate relationship between choice and consequence demonstrates the potential of JavaScript in creating layered narratives that invite players to engage deeply with the story.
Finally, “The Stanley Parable” cleverly subverts traditional narrative expectations. Players navigate a seemingly linear path, but their decisions lead to unexpected and often humorous endings. The game’s commentary on choice and narrative flow serves as a reminder of the power of storytelling in games, highlighting how JavaScript can facilitate complex interactions that challenge conventional storytelling paradigms.