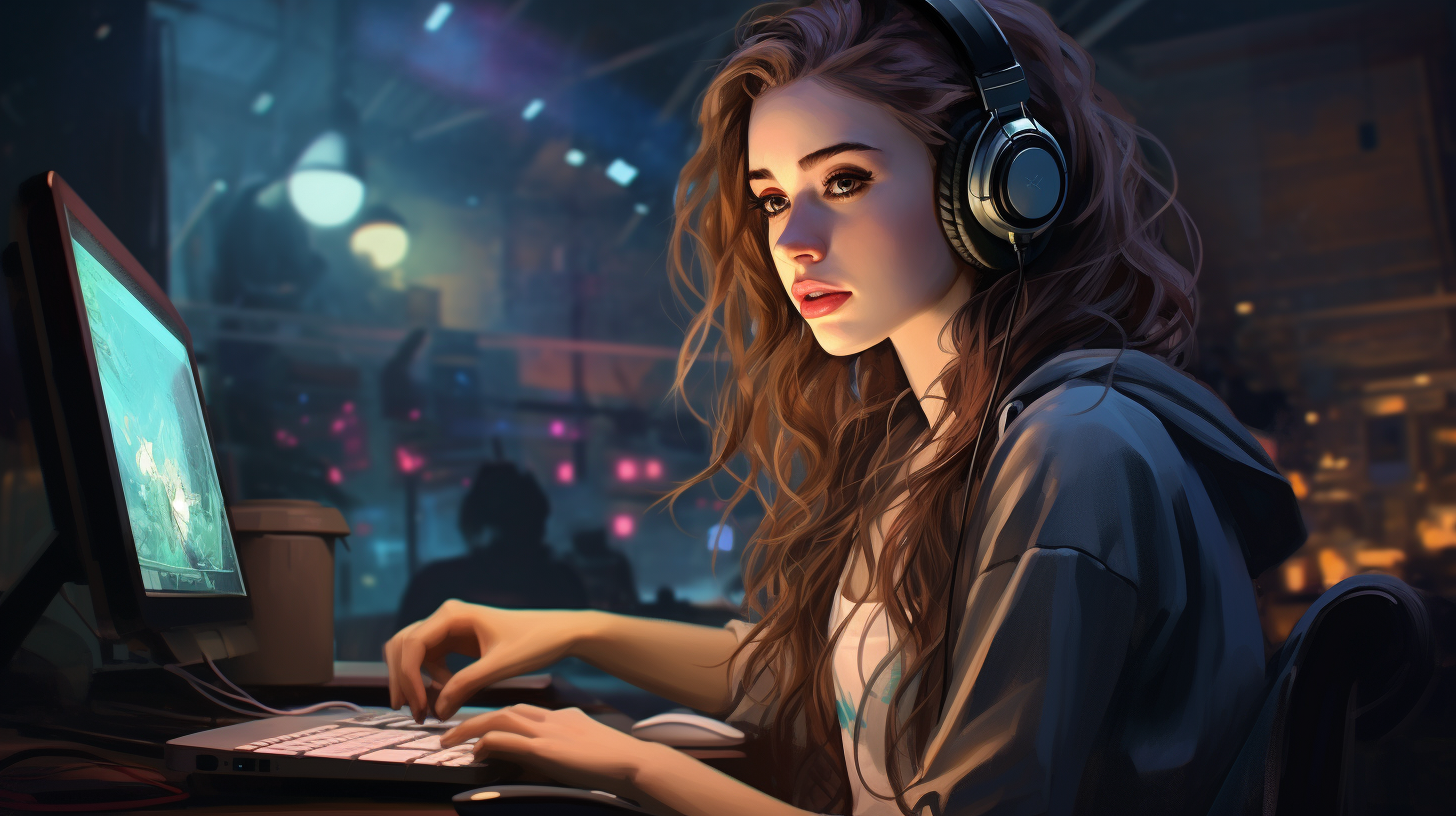
SQL Transactions for Data Integrity
In the context of database management, SQL transactions represent a critical component that ensures data integrity and consistency. A transaction is a sequence of one or more SQL operations that are executed as a single unit of work. The primary goal of a transaction is to guarantee that either all the operations are executed successfully, or none at all, which is fundamental in maintaining a reliable database state.
To grasp the idea of transactions, consider the analogy of a bank transfer. When transferring money from one account to another, two primary actions occur: debiting the sender’s account and crediting the recipient’s account. If any part of this process fails, such as an interruption or an error in communication, it’s crucial that neither account is altered, preserving the financial integrity.
SQL transactions are initiated with the BEGIN TRANSACTION
command and concluded with either COMMIT
or ROLLBACK
. The former confirms the changes made during the transaction, while the latter discards any modifications, reverting the database to its previous state.
BEGIN TRANSACTION; -- Debit the sender's account UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Credit the recipient's account UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT;
In this example, if the debit operation is successful but the credit operation fails (perhaps due to a constraint violation), the transaction is rolled back, ensuring that the sender’s account does not reflect a transaction that was not completed.
Transactions also promote data integrity through isolation, allowing multiple transactions to occur at once without interfering with one another. This isolation ensures that the results of one transaction remain hidden from others until the transaction is complete.
ACID Principles Explained
At the heart of SQL transactions lies the ACID principles, a set of properties that guarantee reliable processing of database transactions. ACID is an acronym that stands for Atomicity, Consistency, Isolation, and Durability. Each of these properties plays a vital role in ensuring that transactions are processed reliably, maintaining the integrity of the database throughout the transaction lifecycle.
Atomicity ensures that a transaction is treated as a single unit, which means that all operations within the transaction must succeed or fail collectively. If any part of the transaction encounters an error, the entire transaction is rolled back, leaving the database unchanged. This property is critical for operations where partial completion could lead to data inconsistency. For example, consider the following SQL code snippet:
BEGIN TRANSACTION; -- Attempt to withdraw funds from the account UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Attempt to deposit funds into another account UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; -- This will only be reached if both updates succeed
If the first update fails, the second won’t be applied, and the database will remain in its previous state.
Consistency ensures that a transaction brings the database from one valid state to another, maintaining all predefined rules, such as data integrity constraints. When a transaction is executed, it must not violate any database rules, and it should ensure that all data remains accurate and valid. For instance, if a transaction involves updating an account balance, it should ensure that the balance never falls below zero:
BEGIN TRANSACTION; -- Check for sufficient funds before proceeding IF (SELECT balance FROM accounts WHERE account_id = 1) >= 100 THEN UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; ELSE ROLLBACK; -- Insufficient funds, revert changes END IF;
The execution of this transaction either succeeds in maintaining account integrity or is rolled back to preserve consistency.
Isolation ensures that at once executed transactions do not affect each other’s execution. Each transaction is executed in isolation, preventing the outcomes of one transaction from being visible to another until it is complete. This very important in a multi-user database environment, where multiple transactions might be trying to read or write to the same data. SQL databases implement various isolation levels (such as READ COMMITTED, READ UNCOMMITTED, REPEATABLE READ, and SERIALIZABLE) to balance performance with data integrity.
Lastly, Durability guarantees that once a transaction has been committed, it will remain so, even in the event of a system failure. This means that the changes made by the transaction are permanently recorded in the database, typically through mechanisms like write-ahead logging or ensuring that all changes are saved to persistent storage before the transaction is considered complete. The following example illustrates a committed transaction’s resilience:
BEGIN TRANSACTION; UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; -- Changes will survive even if the server crashes after this point
Types of SQL Transactions
SQL transactions can be categorized into various types, primarily focusing on how they are executed and their impact on the database. Understanding these types is essential for effective transaction management, as each serves different use cases and requirements. The three primary types of SQL transactions are implicit, explicit, and distributed transactions.
Implicit Transactions are those that the database management system (DBMS) automatically manages. When a single SQL statement is executed, the DBMS treats it as a transaction if it involves data modification (like INSERT, UPDATE, or DELETE) and automatically commits it once the statement is executed successfully. This can simplify transaction control for simpler operations where multiple statements are not necessary.
-- Implicit transaction example UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- This update is automatically committed if successful
However, implicit transactions can be limiting when complex operations require multiple steps or error handling, making explicit transactions preferable in many scenarios.
Explicit Transactions, in contrast, allow developers to define the transaction boundaries manually, giving them greater control over error handling and rollback capabilities. That’s achieved using the BEGIN TRANSACTION, COMMIT, and ROLLBACK commands. Explicit transactions are particularly useful in complex scenarios, such as the aforementioned bank transfer example, where precise control over multiple operations is essential.
-- Explicit transaction example BEGIN TRANSACTION; UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; -- Only committed if both updates succeed
In this case, if an error occurs during either update, the entire transaction can be rolled back, ensuring the database remains in a consistent state.
Distributed Transactions extend the idea of transactions across multiple databases or systems. These transactions are crucial in environments where data integrity needs to be maintained across disparate data sources. Distributed transactions leverage a two-phase commit protocol to ensure that all participating databases either commit or roll back the transaction collectively. This complexity is necessary but introduces additional overhead, as coordination between multiple systems is required.
-- Distributed transaction example (pseudo-code) BEGIN DISTRIBUTED TRANSACTION; UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Database A UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; -- Database B COMMIT; -- Both databases must agree to commit the changes
With distributed transactions, it is vital to ensure that all systems involved are capable of participating in the transaction, and the proper transaction manager is in place to handle the coordination process efficiently.
Implementing Transactions in SQL
Implementing transactions in SQL is simpler, yet it requires a rigorous approach to ensure that the intended data integrity and consistency are achieved. When executing transactions in SQL, it is crucial to follow a systematic way to encapsulate your SQL statements within transaction control commands. This process involves using the BEGIN TRANSACTION, COMMIT, and ROLLBACK commands to define the transaction boundaries and manage the execution flow.
The fundamental syntax for implementing a transaction is as follows:
BEGIN TRANSACTION; -- Your SQL operations here COMMIT; -- or ROLLBACK; if needed
In a practical scenario, let’s consider a situation where you need to transfer funds between two accounts. The implementation should be robust enough to handle potential errors, ensuring that if any part of the transaction fails, all changes are rolled back. Here’s how you might implement such a transaction:
BEGIN TRANSACTION; -- Step 1: Attempt to withdraw from the sender's account UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Step 2: Check if the withdrawal was successful IF @@ROWCOUNT = 0 BEGIN ROLLBACK; -- Rollback if no rows were affected PRINT 'No account found or insufficient funds.'; END ELSE BEGIN -- Step 3: If withdrawal was successful, proceed to deposit into the receiver's account UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; -- Step 4: Ensure the deposit was also successful IF @@ROWCOUNT = 0 BEGIN ROLLBACK; -- Rollback if the deposit fails PRINT 'Deposit failed. Transaction rolled back.'; END ELSE BEGIN COMMIT; -- Both operations succeeded, commit the transaction PRINT 'Transaction completed successfully.'; END END
This example demonstrates a clear transaction flow where each operation is checked for success before proceeding to the next step. If any operation fails, an immediate rollback is triggered, ensuring that the database remains consistent.
Additionally, it’s important to ponder error handling in your transaction implementations. In SQL Server, for instance, you can make use of the TRY…CATCH construct to handle exceptions that may occur during transaction execution:
BEGIN TRY BEGIN TRANSACTION; -- Perform multiple operations UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; -- Commit if all operations succeed END TRY BEGIN CATCH ROLLBACK; -- Rollback if an error occurs PRINT 'An error occurred: ' + ERROR_MESSAGE(); END CATCH;
This approach encapsulates the transaction logic within a try block. Should any error arise during the execution of the SQL statements, control is passed to the catch block, where the transaction can be rolled back, and an error message is reported. This mechanism not only simplifies error handling but also enhances the robustness of your transaction implementations.
Handling Errors and Rollbacks
In the context of SQL transactions, handling errors and rollbacks is a vital aspect that ensures data integrity in the face of unforeseen issues. When a transaction is executed, it operates under the assumption that everything will proceed smoothly. However, real-world scenarios often present challenges such as connection failures, constraint violations, or even unexpected data states that can lead to transaction failures. This is where robust error handling and rollback mechanisms come into play.
When an error occurs during the execution of a transaction, the primary goal is to revert any changes made during that transaction to maintain the database’s consistency. This capability is especially crucial in financial applications, where even the smallest error can lead to significant discrepancies. The SQL standard provides mechanisms to handle errors gracefully, allowing developers to roll back transactions at the first sign of trouble.
To illustrate the error handling process, think this enhanced example of a bank transfer transaction, which incorporates error checking and rollback procedures:
BEGIN TRANSACTION; -- Step 1: Attempt to withdraw from the sender's account UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Check if the withdrawal was successful IF @@ROWCOUNT = 0 BEGIN ROLLBACK; -- Rollback if no rows were affected or insufficient funds PRINT 'Withdrawal failed. No account found or insufficient funds.'; RETURN; END -- Step 2: Attempt to deposit into the receiver's account UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; -- Check if the deposit was successful IF @@ROWCOUNT = 0 BEGIN ROLLBACK; -- Rollback if the deposit fails PRINT 'Deposit failed. Transaction rolled back.'; RETURN; END COMMIT; -- If both operations succeeded, commit the transaction PRINT 'Transaction completed successfully.';
In this example, each SQL operation is followed by a check to see if the previous operation was successful, indicated by the @@ROWCOUNT system variable. If an error occurs, such as an attempt to withdraw from a nonexistent account or when insufficient funds are present, the transaction is rolled back immediately, and a message is printed to inform the user of the failure.
Moreover, SQL Server and other RDBMS platforms offer advanced error handling techniques using the TRY…CATCH construct. This structure allows for a more comprehensive error management system, which can capture exceptions and execute rollback statements in a controlled manner. Here’s how you can implement it:
BEGIN TRY BEGIN TRANSACTION; -- Perform multiple operations UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; -- Commit if all operations succeed PRINT 'Transaction completed successfully.'; END TRY BEGIN CATCH ROLLBACK; -- Rollback if an error occurs PRINT 'An error occurred: ' + ERROR_MESSAGE(); END CATCH;
In this structure, if any error occurs during the execution of the transaction commands in the TRY block, control is passed to the CATCH block, where a rollback is executed. The ERROR_MESSAGE() function provides valuable feedback on the nature of the error, facilitating debugging and enhancing user experience.
Best Practices for Transaction Management
Managing SQL transactions is not merely a technical necessity; it’s also an art that requires a keen understanding of best practices to ensure data integrity and application reliability. Here are several best practices to think when working with transactions in SQL, designed to optimize performance and maintain consistency.
1. Keep Transactions Short and Focused
One of the cardinal rules of transaction management is to keep transactions as brief as possible. Long-running transactions can lead to locking issues and reduced concurrency, as they hold resources for prolonged periods. By minimizing the scope of a transaction, you reduce the likelihood of deadlocks and improve overall system responsiveness. For instance:
BEGIN TRANSACTION; -- Only execute necessary operations UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; COMMIT;
In this example, the transaction focuses solely on updating the balance, avoiding unnecessary operations that could extend the transaction duration.
2. Use Appropriate Isolation Levels
SQL databases support various isolation levels that govern how transactions interact with each other. Selecting the right isolation level is critical for balancing performance and data integrity. For instance:
SET TRANSACTION ISOLATION LEVEL SERIALIZABLE; BEGIN TRANSACTION; -- Your SQL operations here COMMIT;
While SERIALIZABLE provides the highest level of isolation, it can significantly impact system performance. Consider using READ COMMITTED for most interactions, reserving higher levels for situations requiring stricter consistency.
3. Handle Errors Gracefully
As previously discussed, error handling especially important in transaction management. Implementing robust error handling mechanisms ensures that transactions can recover gracefully from unexpected situations. Using TRY…CATCH blocks can help to encapsulate transaction logic and manage errors effectively:
BEGIN TRY BEGIN TRANSACTION; -- Perform multiple operations UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT; END TRY BEGIN CATCH ROLLBACK; -- Rollback if an error occurs PRINT 'Error occurred: ' + ERROR_MESSAGE(); END CATCH;
By implementing this structure, you ensure that your transactions are resilient and maintain integrity despite potential failures.
4. Avoid User Interaction Inside Transactions
When designing applications that utilize SQL transactions, it’s best practice to avoid any user interactions during a transaction. User prompts can introduce delays, leading to prolonged locks and potential deadlocks. Instead, gather all necessary inputs before starting the transaction, allowing for smoother execution:
DECLARE @amount INT = 100; DECLARE @sender_account_id INT = 1; DECLARE @recipient_account_id INT = 2; BEGIN TRANSACTION; UPDATE accounts SET balance = balance - @amount WHERE account_id = @sender_account_id; UPDATE accounts SET balance = balance + @amount WHERE account_id = @recipient_account_id; COMMIT;
This approach ensures that user input does not interfere with transaction timing, thus enhancing performance.
5. Implement Logging and Monitoring
To better manage transactions, implement logging and monitoring strategies. Keeping track of transaction states and outcomes allows you to analyze performance and detect anomalies quickly. SQL Server provides system views that can be queried for transaction logs:
SELECT * FROM fn_dblog(NULL, NULL);
This query retrieves the transaction log, which can be invaluable for auditing and diagnosing transaction-related issues in your database.
6. Test Transactions Thoroughly
Before deploying transaction-heavy applications, thorough testing is essential. Simulate various failure scenarios to ensure your transaction logic handles errors gracefully and maintains data integrity. Writing comprehensive unit tests for your transaction logic can help identify potential pitfalls before they become problems in production.