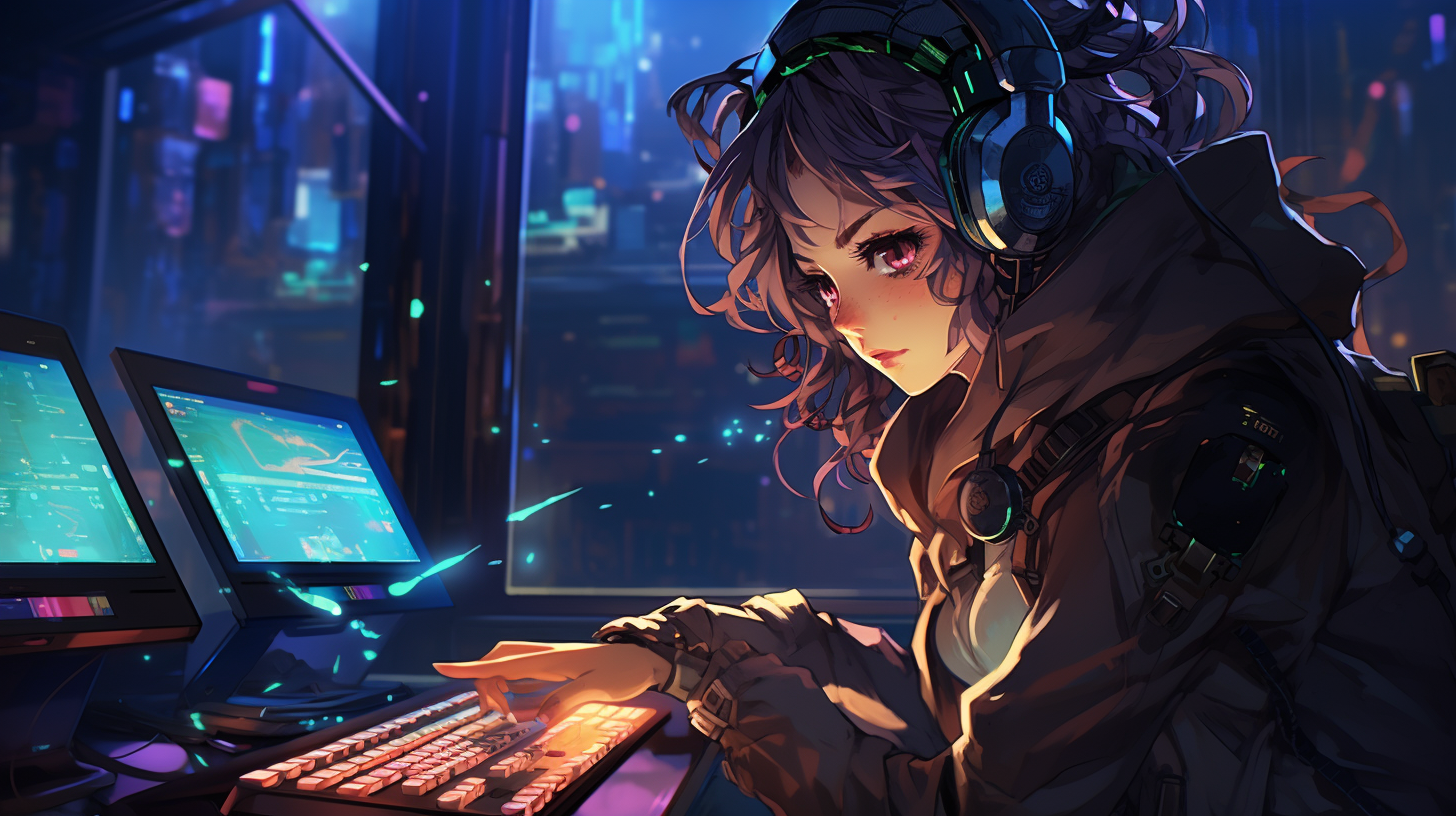
Java Annotations: Creating Custom Annotations
Java annotations are a powerful feature of the Java programming language that allows developers to add metadata to their code. This metadata can provide information to the compiler, be available at runtime through reflection, or even be processed by tools and libraries. At their core, annotations help make the code more expressive and facilitate various functionalities without changing the actual logic of the program.
Annotations are defined using the @interface
keyword and can be applied to classes, methods, fields, parameters, and even other annotations. This flexibility enables a wide range of use cases, from simple tagging to complex processing by frameworks. For instance, the built-in annotations in Java, such as @Override
, @Deprecated
, and @SuppressWarnings
, illustrate how annotations can guide the compiler and improve code readability.
The processing of annotations can occur at different stages:
- Some annotations are processed during compilation, allowing tools to generate code or perform checks.
- Annotations can be retained at runtime, making them accessible for reflection, which is particularly useful for frameworks like Spring and Hibernate.
To define an annotation, one specifies its retention policy, which determines how long the annotation is kept. That is done using the @Retention
annotation. For example, if an annotation is only needed at compile time, it can be declared with RetentionPolicy.SOURCE
. If it should be retained in the compiled class files but not available during runtime, RetentionPolicy.CLASS
is used. Lastly, if an annotation should be available at runtime, RetentionPolicy.RUNTIME
is the appropriate choice.
import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; @Retention(RetentionPolicy.RUNTIME) public @interface MyCustomAnnotation { String value(); }
This example shows how to define a custom annotation named MyCustomAnnotation
that retains its information at runtime. The value
method inside the annotation can be used to pass additional information when the annotation is applied.
Annotations can also have elements (methods) and default values. It very important to understand that they do not have a body; instead, they define attributes that can be configured during their application.
import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; @Retention(RetentionPolicy.RUNTIME) public @interface MyCustomAnnotation { String value() default "default value"; int number() default 0; }
In this example, MyCustomAnnotation
includes two elements: value
and number
, each with a default value. This allows developers to use the annotation without specifying all attributes explicitly, which can help reduce boilerplate code and improve usability.
Using annotations in Java promotes cleaner code, enriches the language’s capabilities, and aids in building robust applications. Understanding how to create and use custom annotations empowers developers to leverage this feature effectively in their projects.
Defining Custom Annotations
When defining custom annotations, it is also essential to think the target of the annotation. The @Target annotation specifies where the custom annotation can be applied, such as on methods, classes, fields, or parameters. This adds another layer of control and helps prevent misuse of the annotation.
Here’s an example of how to define an annotation that can only be used on methods:
import java.lang.annotation.ElementType; import java.lang.annotation.Target; @Target(ElementType.METHOD) @Retention(RetentionPolicy.RUNTIME) public @interface MethodAnnotation { String description() default "No description provided"; }
In this case, the @MethodAnnotation can only be applied to methods, and it includes a description element with a default value.
Another important aspect of defining annotations is the use of meta-annotations. These are annotations that can be applied to other annotations. For instance, @Documented indicates that whenever the annotation is used, it should be included in the Javadoc, enhancing its visibility in the generated documentation.
Here’s how you can combine meta-annotations with a custom annotation:
import java.lang.annotation.Documented; import java.lang.annotation.ElementType; import java.lang.annotation.Target; @Documented @Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) public @interface DocumentedClassAnnotation { String author(); String version(); }
This example defines a custom annotation called DocumentedClassAnnotation that can only be applied to classes and includes metadata about the author and version. Because it is annotated with @Documented, this information will be available in the Javadoc.
When creating custom annotations, it is also worth noting that they can extend existing annotations. That’s useful when you want to add additional functionality or constraints while still inheriting the behaviors of the original annotation.
Here’s an example of an annotation that extends another:
import java.lang.annotation.ElementType; import java.lang.annotation.Target; @Target(ElementType.FIELD) @interface FieldAnnotation { String value(); } @Target(ElementType.FIELD) @Retention(RetentionPolicy.RUNTIME) public @interface ExtendedFieldAnnotation extends FieldAnnotation { boolean required() default false; }
In this example, ExtendedFieldAnnotation inherits from FieldAnnotation and adds a required attribute, enabling users to specify whether the field is mandatory.
Defining custom annotations in Java involves specifying retention policies, targets, and optional elements, while also considering the use of meta-annotations to enhance documentation and utility. This understanding forms the foundation for effectively using annotations to enhance code organization and readability.
Using Custom Annotations in Code
Once you have defined your custom annotations, the next step is to utilize them in your code effectively. The application of custom annotations can significantly enhance code clarity and functionality. By annotating classes, methods, fields, and other elements, you can embed valuable information that can later be retrieved and processed.
To use a custom annotation, simply apply it to the desired element in your code. Here’s a simpler example of how to use the previously defined MyCustomAnnotation:
@MyCustomAnnotation(value = "Example value") public class AnnotatedClass { @MyCustomAnnotation(value = "Method annotation") public void annotatedMethod() { // Method logic goes here } }
In this example, the AnnotatedClass is marked with the MyCustomAnnotation, and the annotatedMethod is also annotated. This indicates that both the class and method have additional metadata associated with them, which can be useful for documentation or processing in frameworks.
To process these annotations at runtime, Java provides reflection capabilities. Using reflection, you can inspect the annotations applied to a class or method and retrieve their values. Here’s how you could implement a simple processor that inspects the annotations:
import java.lang.reflect.Method; public class AnnotationProcessor { public static void main(String[] args) { Class<AnnotatedClass> obj = AnnotatedClass.class; if (obj.isAnnotationPresent(MyCustomAnnotation.class)) { MyCustomAnnotation annotation = obj.getAnnotation(MyCustomAnnotation.class); System.out.println("Class annotation value: " + annotation.value()); } for (Method method : obj.getDeclaredMethods()) { if (method.isAnnotationPresent(MyCustomAnnotation.class)) { MyCustomAnnotation annotation = method.getAnnotation(MyCustomAnnotation.class); System.out.println("Method " + method.getName() + " annotation value: " + annotation.value()); } } } }
In this AnnotationProcessor class, reflection is used to check if the AnnotatedClass has the MyCustomAnnotation applied. If it’s present, it retrieves and prints the annotation’s value. It similarly checks each method in the class for the annotation, demonstrating how you can dynamically interact with the annotations embedded in your code.
Custom annotations can also be used in conjunction with various frameworks that utilize them for configuration or behavior modification. For instance, in Spring, annotations play an important role in defining beans and configuring dependency injection. By annotating your classes and methods, you can leverage a wide array of functionalities intrinsic to these libraries.
For example, think a scenario in a Spring application where you are marking a method for transactional behavior:
import org.springframework.transaction.annotation.Transactional; public class TransactionalService { @Transactional public void performTransactionalOperation() { // Code that should run within a transaction } }
In this snippet, the performTransactionalOperation method is annotated with @Transactional, indicating that the method should execute within a transactional context. Spring’s transaction management then processes this annotation at runtime, ensuring proper transaction handling without cluttering the business logic with transaction management code.
Using custom annotations in your Java code not only allows for clearer and more organized code but also opens up avenues for powerful integrations with various frameworks. By applying and processing annotations thoughtfully, developers can create expressive and maintainable applications that adhere to contemporary best practices.
Best Practices for Custom Annotations
When creating custom annotations, adhering to best practices very important for ensuring that they serve their intended purpose without causing confusion or misuse. One of the primary considerations is to keep annotations focused and concise. Each annotation should encapsulate a single responsibility or a specific piece of metadata. This not only enhances readability but also simplifies the logic associated with processing the annotations.
For example, instead of creating a monolithic annotation that combines multiple attributes, it is often more beneficial to define several smaller, purpose-specific annotations. This way, the developer can mix and match annotations as needed, leading to clearer code. Ponder the following example:
@Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) public @interface Entity { String tableName(); } @Target(ElementType.FIELD) @Retention(RetentionPolicy.RUNTIME) public @interface Column { String name(); boolean nullable() default true; }
In this case, the @Entity
annotation is used to specify a database table name, while the @Column
annotation can be applied to class fields to define column attributes. This separation allows for greater flexibility and clearer intent.
Another best practice is to provide meaningful defaults for annotation elements when applicable. This can make the annotations easier to use, as developers may not always need to specify every attribute. However, it’s essential to strike a balance; overly broad defaults can lead to ambiguous usages. Think the following example:
@Target(ElementType.METHOD) @Retention(RetentionPolicy.RUNTIME) public @interface ScheduledTask { String cron() default "0 0 * * *"; // Default to run hourly String description() default "Scheduled task"; }
In this annotation, cron
and description
both have reasonable defaults. This allows a developer to use @ScheduledTask
without specifying the cron expression, while still conveying the purpose of the task.
Documentation is another critical aspect of custom annotations. Using @Documented
to indicate that an annotation is intended to be part of the public API enhances its visibility in generated documentation. Additionally, providing clear and simple documentation for each annotation can significantly help other developers understand how and when to use them. Here’s an example:
@Documented @Target(ElementType.METHOD) @Retention(RetentionPolicy.RUNTIME) public @interface LogExecutionTime { String value() default "Execution Time:"; }
For the @LogExecutionTime
annotation, ensuring proper documentation will guide developers on its intended use, such as logging method execution durations for performance monitoring.
Modifying existing frameworks or libraries with custom annotations should be approached with caution. When extending or using annotations from third-party libraries, ensure that your annotations are compatible and do not introduce unexpected behaviors. Where possible, stick to established conventions within the community to maintain code consistency.
Finally, think the lifecycle of your annotations. When designing annotations that will be processed at runtime, ensure they are properly retained using RetentionPolicy.RUNTIME
. Also, assess if there are performance implications or potential memory leaks associated with retaining annotations, especially in large systems. For example:
@Target(ElementType.TYPE) @Retention(RetentionPolicy.RUNTIME) public @interface Cacheable { String cacheName(); }
The @Cacheable
annotation might be used to mark classes whose instances should be cached, but if it is retained unnecessarily, it could lead to excessive memory usage. Being mindful of the retention policy is imperative for both performance and resource management.
By following these best practices, developers can create custom annotations that are not only functional but also enhance the overall quality and maintainability of the codebase. Thoughtfully designed annotations facilitate cleaner and more expressive code, ultimately leading to better software architecture.