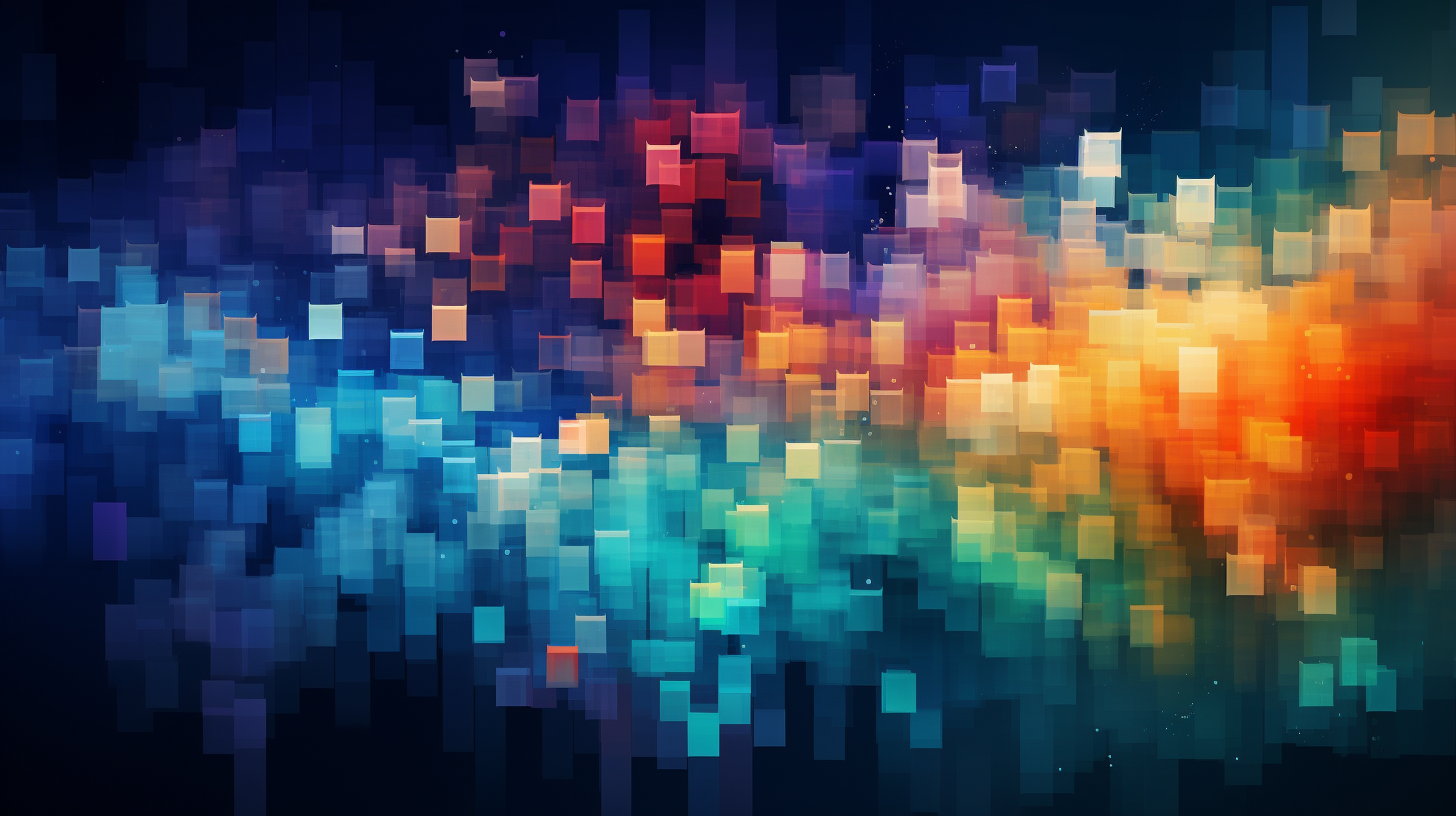
Performance Tuning in Bash Scripts
When it comes to performance tuning in Bash scripts, the first step is to understand the metrics that gauge their efficiency and speed. Performance metrics can provide insight into where bottlenecks occur and how resources are being utilized. The primary metrics to focus on include execution time, memory usage, and CPU load.
Execution Time: The time it takes for a script to execute is often the most critical metric. You can measure this using the built-in time
command, which will give you a breakdown of real time, user time, and system time.
time ./your_script.sh
The output will look something like this:
real 0m0.123s user 0m0.089s sys 0m0.034s
Here, real
indicates the actual elapsed time, while user
and sys
refer to the CPU time spent in user mode and kernel mode, respectively. Keeping an eye on these values helps you identify if your script is spending too much time in a specific area.
Memory Usage: Monitoring memory consumption is just as crucial as measuring execution time. You can use the ps
command or the top
command to assess the memory usage of your script while it runs. Employing the following command can reveal the memory footprint:
ps -o pid,vsz,rss,cmd -p $(pgrep -f your_script.sh)
Here, vsz
denotes the virtual memory size, and rss
indicates the resident set size, which is the non-swapped physical memory the task is using. Large discrepancies between these numbers can indicate excessive memory allocation.
CPU Load: Understanding the CPU load during script execution is essential, especially if your script is intended to run on a shared server. This can be monitored using the top
command, or you can observe the CPU load with:
vmstat 1
The vmstat
command will provide a snapshot of system performance, including CPU idle time, which can indicate how much of your CPU is being consumed by your script.
By examining these metrics—execution time, memory usage, and CPU load—you can develop a comprehensive understanding of your Bash script’s performance. This, in turn, allows you to make targeted optimizations that can lead to significant improvements in efficiency and resource utilization.
Optimizing Variable Usage and Data Structures
In the quest for optimized Bash scripts, the careful management of variable usage and data structures can yield remarkable efficiency gains. Bash, while powerful, has its nuances, particularly regarding how it handles variables and arrays. An understanding of these subtleties is key to enhancing script performance.
One of the cardinal rules in Bash scripting is to minimize variable scope and reuse. Each variable declaration incurs overhead, so prefer reusing variables rather than declaring new ones unnecessarily. For instance, instead of creating separate variables for interim calculations, you can reuse a single variable:
count=0 for value in 1 2 3 4 5; do count=$((count + value)) done echo $count
In this snippet, the variable count
efficiently holds the cumulative sum throughout the loop, avoiding the overhead of multiple variable declarations.
Additionally, it’s vital to select the right data structures. Bash supports arrays, which can be used to store collections of values, but they should be used judiciously. Indexed arrays allow for a compact representation, which can be advantageous over individual scalar variable declarations, particularly in loops:
scores=(90 85 88 92 78) sum=0 for score in "${scores[@]}"; do sum=$((sum + score)) done echo "Total score: $sum"
In the above example, using an array scores
not only simplifies the code but also enhances performance by minimizing variable declarations and maintaining a clear structure.
When it comes to associative arrays (or hash tables), they provide an efficient way to manage key-value pairs. If you’re dealing with look-up operations frequently, employing associative arrays can significantly reduce the time complexity:
declare -A fruit_colors fruit_colors=(["apple"]="red" ["banana"]="yellow" ["grape"]="purple") for fruit in "${!fruit_colors[@]}"; do echo "$fruit is ${fruit_colors[$fruit]}" done
Here, the associative array fruit_colors
streamlines the process of mapping fruits to their colors, allowing for quick references that would otherwise require more cumbersome constructs.
Moreover, initialization matters. When dealing with large datasets, initialize arrays with a predefined size if possible to avoid dynamic resizing, which can be costly in terms of performance. A well-structured initialization can look like this:
declare -a results results=() # Initialize an empty array for i in {1..1000}; do results[i]=$((i * 2)) done
In this scenario, the array results
is prepped for efficient usage, leading to a smoother execution.
Lastly, always remember to quote your variables unless you’re sure they will not contain spaces or special characters. Unquoted variables can lead to unintended word splitting or globbing, which can introduce subtle bugs and performance hits. For instance:
file_list=$(ls) for file in $file_list; do echo "Processing: $file" done
This should be rewritten for safety and efficiency:
file_list=$(ls) for file in $file_list; do echo "Processing: $file" done
By following these practices—minimizing scope, choosing the right data structures, initializing correctly, and quoting variables—you’ll harness the full power of Bash for your scripting needs, achieving not just function but elegance in your code.
Efficient Looping and Iteration Techniques
When it comes to optimizing loops and iterations in Bash scripts, the efficiency of your looping constructs can significantly influence overall performance. Loops often represent the core of your logic, and how you implement them can either enhance or hinder execution speed. A few well-considered techniques can lead to more efficient looping mechanisms that will make your scripts run smoother and faster.
One of the fundamental approaches to improving loop efficiency is to choose the right type of loop for the job. For example, when iterating over a list of items, the use of a simple for
loop can be both simpler and effective:
for item in item1 item2 item3; do echo "Processing $item" done
However, when you need to iterate over a range of numbers, using a C-style for
loop can yield better readability and performance:
for ((i=0; i<10; i++)); do echo "Number $i" done
This C-style loop avoids the overhead of expanding a sequence of numbers into a list, allowing for faster execution.
Another significant optimization technique involves reducing unnecessary computations within the loop. For instance, if you find yourself calculating the same value repeatedly, think moving that calculation outside the loop:
factor=2 for ((i=1; i<=10; i++)); do result=$((i * factor)) echo "Result for $i: $result" done
In this example, the multiplication factor is stored outside the loop, preventing the need to redefine it during each iteration, thus reducing overhead.
Using while
loops can also be an efficient alternative, especially when the number of iterations isn’t predetermined. This method is particularly useful when working with file input or data streams:
count=1 while [ $count -le 5 ]; do echo "Count is $count" ((count++)) done
Moreover, when processing large files or datasets, ponder using read loops to handle input more efficiently. Instead of loading the entire file into memory, read it line by line:
while IFS= read -r line; do echo "Line: $line" done < input_file.txt
This method conserves memory and allows your script to handle very large files without performance degradation.
Another optimization technique involves breaking out of a loop early when a condition is met. This can save unnecessary iterations and improve performance:
for ((i=0; i<10; i++)); do if [ $i -eq 5 ]; then echo "Breaking at $i" break fi echo "Current index: $i" done
In this snippet, the loop is terminated as soon as the desired condition is met, avoiding further iterations.
Additionally, parallel processing using background jobs can also enhance performance in scenarios where tasks are independent. For example:
process_files() { for file in *.txt; do echo "Processing $file" # Simulate a processing task sleep 1 done } process_files & # Run in background wait # Wait for all background jobs to finish
Running tasks in the background allows multiple processes to execute concurrently, significantly reducing overall execution time.
Lastly, be cautious with nested loops. If not handled judiciously, they can lead to performance bottlenecks. Aim to refactor nested loops into single loops when possible, or explore using functions to encapsulate and manage complexity, enhancing both performance and maintainability.
By applying these efficient looping and iteration techniques, you can ensure that your Bash scripts are not only functional but also optimized for performance, enabling them to handle tasks with speed and grace.
Minimizing External Command Calls
for ((i=0; i<10; i++)); do echo "Iteration $i" done
This form of the loop not only makes it explicit that you’re working with integers, but also allows for more complex conditions and increments with clear syntactical structure.
Another technique that can enhance performance is reducing the overhead associated with looping constructs. When manipulating arrays, instead of using a traditional for loop with an index, you can directly iterate over the elements of the array, which simplifies the code and can improve speed:
array=(one two three four five) for element in "${array[@]}"; do echo "Element: $element" done
Using the built-in method to access array elements avoids the need for index calculations, making your code cleaner and potentially faster.
When needing to loop through a sequence of numbers, the `seq` command is often used, but it’s worth noting that using brace expansion can be more efficient:
for i in {1..10}; do echo "Number: $i" done
This avoids spawning an external process, using Bash’s built-in capabilities.
In terms of performance, ensuring that your loops do not invoke external commands unnecessarily can be a game-changer. Each external command call can introduce latency, so it is best to do as much processing within the loop as possible. For instance, instead of calling `grep` in a loop, filter the data in memory:
data=(apple banana cherry date) for item in "${data[@]}"; do [[ $item == *"a"* ]] && echo "Contains 'a': $item" done
Here, using a built-in conditional instead of invoking an external command like `grep` saves time and resources.
Furthermore, to improve overall script performance, batch processing can be beneficial. If you find yourself performing the same operation multiple times, ponder accumulating results and processing them in one go. For example:
results=() for i in {1..100}; do results+=($((i * 2))) done for result in "${results[@]}"; do echo "Result: $result" done
This avoids repeated operations and minimizes the number of times data is accessed.
Lastly, consider the impacts of subshells when using pipelines within loops. Each pipeline creates a new subshell, which can lead to increased overhead. Instead, align your commands to work together within the main shell environment whenever possible:
while read -r line; do echo "Processing: $line" done < inputfile.txt
In this example, using input redirection directly into the loop prevents the need for a separate subshell, streamlining performance.
By applying these efficient looping techniques, you can significantly reduce the execution time of your Bash scripts, thus enhancing their performance and responsiveness while keeping your code clean and maintainable.
Using Built-in Bash Functions
In the sphere of Bash scripting, using built-in functions is not merely a suggestion; it’s an essential practice that can lead to substantial performance improvements. Bash is equipped with several built-in functions that not only enhance the speed of your scripts but also simplify code complexity. By using these built-ins effectively, you can avoid the overhead associated with external commands and streamline your script’s execution.
One of the most powerful built-in functions in Bash is printf, which serves as a robust replacement for the traditional echo. While echo is often sufficient for simple output, printf provides greater control over formatting, leading to more efficient string handling:
printf "Value: %.2fn" 123.456
In this example, printf formats the number to two decimal places, resulting in predictable output without the pitfalls of echo. This not only enhances readability but also minimizes the risk of errors in output formatting.
Another built-in function that can dramatically boost performance is let. This command allows arithmetic operations to be executed without the need for additional subshells, avoiding the performance cost associated with invoking a new process:
let "count = count + 1"
Instead of using the more cumbersome $(()) syntax for arithmetic, let provides a cleaner and more efficient approach, particularly in tight loops where every millisecond counts.
When dealing with string manipulations, the built-in string manipulation features of Bash are invaluable. For instance, extracting substrings or performing substitutions can be done directly without calling external tools like awk or sed:
string="Hello, World!" substring=${string:7:5} # Extracts "World" echo $substring
Here, we extract a substring from a string using built-in capabilities, which is not only faster but also reduces the complexity of the code by eliminating the need for external commands.
Using mapfile is another way to improve performance when handling large amounts of data. This built-in function reads lines from input into an array, allowing for efficient bulk processing:
mapfile -t lines < input.txt for line in "${lines[@]}"; do echo "$line" done
In this scenario, mapfile replaces the need for multiple calls to read lines individually, making your script cleaner and significantly faster when processing large files.
While built-in functions enhance performance, they also contribute to code clarity. When you tap into these capabilities, your scripts become more readable and maintainable, which is important in collaborative environments. In contrast, if you rely excessively on external commands, your scripts can become convoluted and harder to debug.
To sum up, using built-in Bash functions is not just a performance optimization strategy; it is a fundamental aspect of writing efficient, clean, and maintainable scripts. By embracing the power of Bash’s built-ins, you can significantly reduce execution time, improve resource efficiency, and enhance the overall quality of your code.
Profiling and Debugging Bash Scripts for Performance
for ((i=0; i<10; i++)); do echo "Iteration $i" done
This approach avoids the overhead of command substitution and is often faster than using a traditional for loop that involves external commands.
Another useful technique is to utilize `while` loops for scenarios that require conditional checks. This can help in reducing unnecessary iterations and can be tightly controlled, optimizing performance:
count=0 while [[ $count -lt 10 ]]; do echo "Count is $count" ((count++)) done
In a case where the number of iterations isn’t predetermined, a `while` loop can be a perfect fit, allowing for a dynamic termination condition.
When dealing with large datasets, consider using `readarray` or `mapfile`, which allows you to read lines from a file directly into an array. This approach can lead to significant performance improvements, especially with large files:
readarray -t lines < input.txt for line in "${lines[@]}"; do echo "Processing: $line" done
This effectively minimizes the overhead of multiple `cat` or `grep` calls while also maintaining the simplicity of array processing.
Furthermore, ponder using process substitution. It can make your script both more efficient and more elegant by which will allow you to treat the output of a command as a file:
while read -r line; do echo "Processing: $line" done < <(ls -1)
In this example, the `ls -1` output is processed line by line with minimal overhead.
When iterating through files or directories, using `find` combined with `-exec` or `-print0` can improve performance significantly:
find /path/to/dir -type f -print0 | while IFS= read -r -d '' file; do echo "Found file: $file" done
This will handle filenames with spaces and special characters seamlessly, enhancing robustness along with performance.
Lastly, use `let` or `$(( ))` for arithmetic operations instead of `expr`, which is slower and less efficient:
let count=10 count=$((count + 5)) echo $count
By applying these techniques—selecting appropriate loop types, using built-in commands effectively, and optimizing iterations—you can significantly enhance the performance of your Bash scripts. Remember, the key is to focus on minimizing overhead while maintaining clarity and efficiency throughout your implementation.