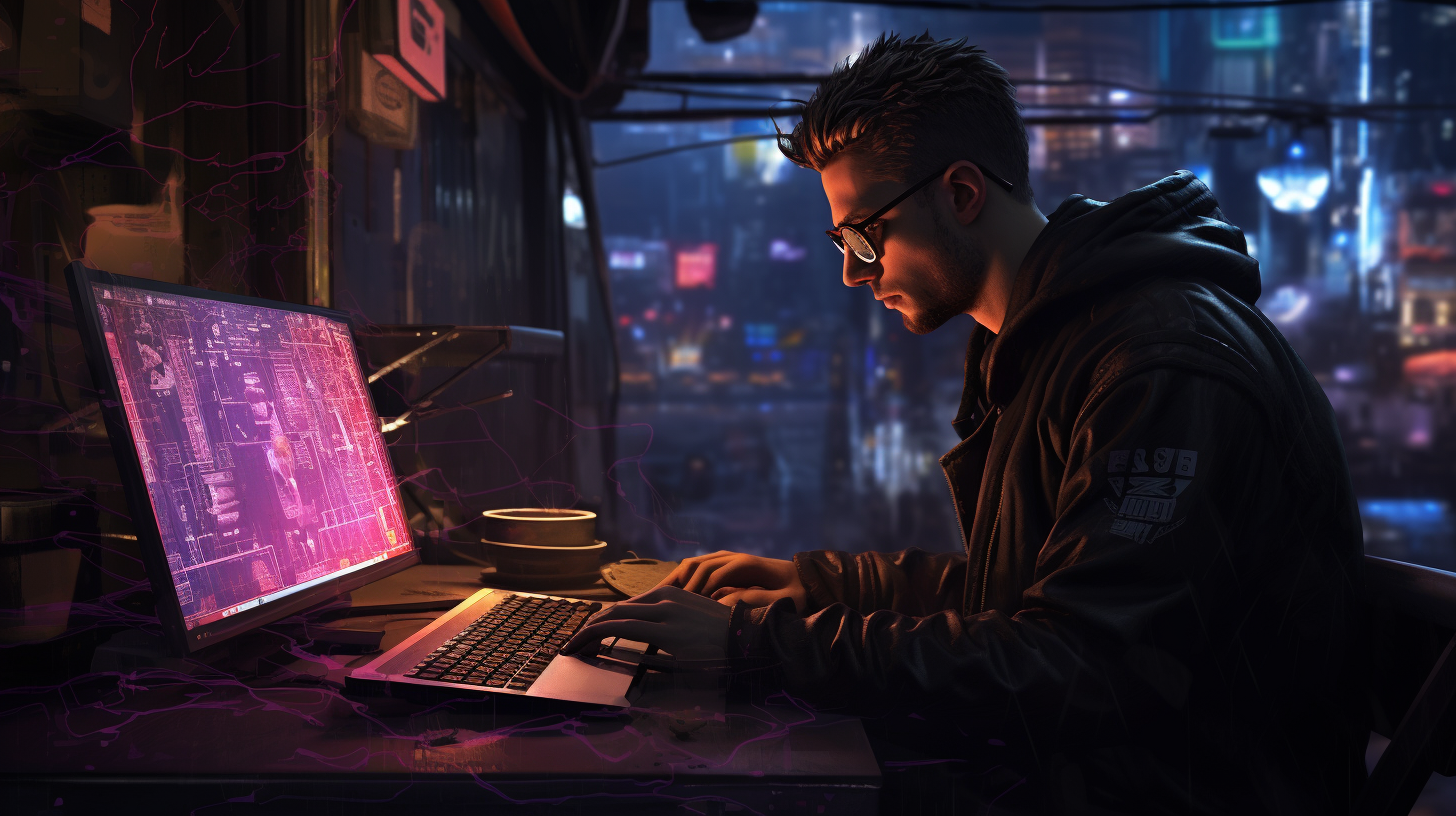
Swift and File Management
In Swift, understanding the file system structure is essential for effective file management. The file system is organized in a hierarchical manner, with directories containing files or other directories, forming a tree-like structure. This design allows for efficient organization and retrieval of files. Swift provides a robust API for interacting with the file system, primarily through the FileManager
class.
Each user has a home directory, typically located at /Users/username
on macOS. This directory contains several standard folders, such as Documents, Downloads, and Desktop, where users can store their files. Understanding these directories especially important for application development, especially for apps that require user data storage.
Swift’s FileManager
class offers methods to navigate the file system, allowing developers to access and manipulate files and directories. The FileManager.default
instance is often used to perform operations like checking for file existence, creating directories, and enumerating contents. Here’s an example of how to explore directories:
let fileManager = FileManager.default let homeDirectory = fileManager.homeDirectoryForCurrentUser do { let contents = try fileManager.contentsOfDirectory(at: homeDirectory, includingPropertiesForKeys: nil) for file in contents { print("File: (file.lastPathComponent)") } } catch { print("Error reading contents of directory: (error)") }
This code snippet retrieves the contents of the user’s home directory and prints out the names of the files and directories contained within it. By using the methods provided by FileManager
, developers can efficiently traverse and manage the file system.
Moreover, Swift provides a way to work with file URLs using the URL
structure. That is particularly useful when dealing with file paths, as it allows for more robust and type-safe manipulations. For example:
let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first! let fileURL = documentsDirectory.appendingPathComponent("example.txt") print("File URL: (fileURL)")
In this code, we obtain the URL for the user’s Documents directory and create a file URL for a hypothetical file named example.txt. The use of URL
provides benefits like better handling of path components and improved readability.
Understanding the file system structure in Swift not only helps in navigating through files and directories but also in creating applications that can effectively store and retrieve user data. This foundational knowledge is key to implementing more advanced file operations as we delve deeper into the file management capabilities of Swift.
Reading and Writing Files
Reading and writing files in Swift is a fundamental aspect of file management that enables applications to persist data and interact with user-generated content. The FileManager class, along with the FileHandle class, provides a simpler API for performing file operations such as creating, reading, writing, and deleting files.
To write data to a file, you can use the `Data` type, which is a versatile representation of binary data. Here’s a simple example of how to create a text file and write data into it:
let fileManager = FileManager.default let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first! let fileURL = documentsDirectory.appendingPathComponent("example.txt") let content = "Hello, Swift File Management!" do { try content.write(to: fileURL, atomically: true, encoding: .utf8) print("File written successfully to: (fileURL)") } catch { print("Error writing to file: (error)") }
In this example, we first obtain the URL for the Documents directory, then append a filename to create a complete file URL. Using the `write(to:atomically:encoding:)` method, we write a simple string to the file. If the operation is successful, a confirmation message is printed; if it fails, the error is caught and printed.
Reading data from a file is equally simpler. You can use the `String` initializer that accepts a URL to read the contents of a text file. Here’s how you can read the contents of the file we just wrote:
do { let readContent = try String(contentsOf: fileURL, encoding: .utf8) print("Read content: (readContent)") } catch { print("Error reading from file: (error)") }
In this example, the `String(contentsOf:encoding:)` initializer is used to read the contents of the file located at the specified URL. If successful, the contents are printed to the console.
It’s important to note that file operations can fail for various reasons, such as lack of permissions, non-existent files, or issues with the file system. Therefore, it’s crucial to handle potential errors using do-catch blocks to ensure robustness in your applications.
Reading and writing files in Swift is made easy through the combination of the FileManager class and the String and Data types. These tools allow developers to efficiently manage file operations, enabling seamless integration of file handling capabilities into their applications.
Handling Directories and File Paths
Handling directories and file paths in Swift is a critical aspect of effective file management. The FileManager class serves as the primary interface for these operations, allowing developers to navigate through the directory structure, create or delete directories, and manipulate file paths seamlessly.
When working with directories, it’s essential to understand how to create and manage them. For instance, you may want to create a folder for storing application-specific data. Here’s how you can do that:
let fileManager = FileManager.default let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first! let appDirectory = documentsDirectory.appendingPathComponent("MyAppData") do { try fileManager.createDirectory(at: appDirectory, withIntermediateDirectories: true, attributes: nil) print("Directory created successfully at: (appDirectory)") } catch { print("Error creating directory: (error)") }
In this example, we use the `createDirectory(at:withIntermediateDirectories:attributes:)` method to create a new directory called “MyAppData” within the Documents directory. The `withIntermediateDirectories` parameter allows for creating any intermediate directories needed to complete the path. Error handling is essential here to ensure that the directory creation is successful.
Once directories are established, you may need to navigate or enumerate through them. The ability to retrieve a list of contents within a directory can be crucial for many applications. Here’s an example:
do { let contents = try fileManager.contentsOfDirectory(at: appDirectory, includingPropertiesForKeys: nil) print("Contents of directory:") for file in contents { print(" - (file.lastPathComponent)") } } catch { print("Error reading contents of directory: (error)") }
In this snippet, we utilize `contentsOfDirectory(at:includingPropertiesForKeys:)` to fetch and display all files and directories within “MyAppData.” This method returns an array of URLs, allowing us to leverage the powerful URL methods for further operations.
Another significant aspect of handling directories is managing file paths. Swift’s `URL` type allows for robust path manipulations that ensure correctness and safety. Ponder this scenario where you need to append a file name to a directory path:
let fileURL = appDirectory.appendingPathComponent("data.txt") print("Full path to the file: (fileURL)")
This code demonstrates how to append a file name to a directory URL. Using `appendingPathComponent(_:)`, we ensure that the file path is constructed correctly, avoiding common pitfalls associated with string manipulation.
Lastly, it is essential to understand how to remove directories when they’re no longer needed. Deleting a directory is simpler but must be handled with care, especially if it contains files. Here’s how to remove a directory:
do { try fileManager.removeItem(at: appDirectory) print("Directory removed successfully at: (appDirectory)") } catch { print("Error removing directory: (error)") }
With the `removeItem(at:)` method, you can delete the directory. However, if the directory contains files, you will encounter an error. Hence, it’s often prudent to check for contents before attempting deletion.
By using the capabilities of Swift’s FileManager and URL types, developers can effectively manage directories and file paths, creating a solid foundation for robust file management in their applications.
Managing File Attributes and Metadata
Managing file attributes and metadata in Swift especially important for applications that rely on detailed information about files and directories. The FileManager class, along with the URL and AttributesOfItem methods, provides a rich interface for accessing and manipulating file attributes. Attributes include file size, creation date, modification date, and permissions, all of which can be essential for tasks such as data synchronization, version control, and user experience enhancements.
To retrieve the attributes of a file, you can use the attributesOfItem(atPath:)
method provided by FileManager. This method returns a dictionary containing various attributes associated with the specified file. Here’s an example of how to obtain file attributes:
let fileManager = FileManager.default let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first! let fileURL = documentsDirectory.appendingPathComponent("example.txt") do { let attributes = try fileManager.attributesOfItem(atPath: fileURL.path) print("File attributes:") for (key, value) in attributes { print("(key): (value)") } } catch { print("Error retrieving file attributes: (error)") }
In this example, we first construct the file URL for “example.txt” and then attempt to retrieve its attributes. If successful, the attributes are printed, giving insight into the file’s properties. The keys in the attributes dictionary are defined in FileAttributeKey
, which includes options like size
, creationDate
, modificationDate
, and posixPermissions
.
Understanding when files were created and last modified can be pivotal in scenarios such as file backup systems, where only the most recent files need to be backed up. This can be accomplished using the creation and modification date attributes:
if let creationDate = attributes[.creationDate] as? Date, let modificationDate = attributes[.modificationDate] as? Date { print("Creation Date: (creationDate)") print("Modification Date: (modificationDate)") }
In this code, we safely unwrap the creation and modification dates from the attributes dictionary, allowing us to utilize this information in our application logic.
Another important aspect of file attributes is managing permissions. Permissions control the access level of the file, specifying who can read, write, or execute it. The posixPermissions
attribute can be examined and modified to ensure proper access control. Here’s how you can check and modify file permissions:
if let permissions = attributes[.posixPermissions] as? NSNumber { print("Current Permissions: (permissions.intValue)") // Example: Grant read and write permissions to the owner let newPermissions: NSNumber = 0o600 // Owner read/write, group/others none try fileManager.setAttributes([.posixPermissions: newPermissions], ofItemAtPath: fileURL.path) print("Updated Permissions to: (newPermissions.intValue)") }
This snippet first retrieves the current permissions and then sets new permissions for the file. The setAttributes(_:ofItemAtPath:)
method allows you to update various attributes, including permissions, ensuring that your application respects user privacy and data security.
Managing file attributes and metadata in Swift not only helps developers understand their files better but also enables the creation of more robust applications. By using the capabilities of FileManager, you can ensure that your applications make informed decisions based on file characteristics, leading to improved functionality and user experiences.
Error Handling in File Operations
Error handling in file operations is a critical aspect of writing robust Swift applications that interact with the file system. Given the inherent complexities of file management, such as varying file permissions, potential non-existent paths, and unpredictable file system states, it is important to implement effective error handling strategies. Swift provides powerful mechanisms for dealing with errors, primarily through the use of the `do-catch` syntax, which allows developers to gracefully handle exceptions that may arise during file operations.
When performing file management tasks, you should anticipate that errors can occur and use `do-catch` blocks to catch these exceptions. Here’s an example of how to handle potential errors when reading a file:
let fileManager = FileManager.default let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first! let fileURL = documentsDirectory.appendingPathComponent("nonexistent.txt") do { let content = try String(contentsOf: fileURL, encoding: .utf8) print("File content: (content)") } catch let error as NSError { print("Error reading file: (error.domain) - (error.localizedDescription)") }
In this code snippet, we attempt to read a file that may not exist. If the file operation fails, the error is caught, and relevant information is printed, including the error domain and a localized description. This practice not only informs developers of the issue but can also be helpful for debugging.
Different types of errors can arise during file operations, and it’s crucial to distinguish between them for appropriate handling. Swift’s `Error` protocol allows creating custom error types, which can provide more context about the specific issue encountered. For instance, ponder defining an enum for file-related errors:
enum FileError: Error { case fileNotFound case insufficientPermissions case unknown var localizedDescription: String { switch self { case .fileNotFound: return "The specified file was not found." case .insufficientPermissions: return "You do not have permission to access the file." case .unknown: return "An unknown error has occurred." } } }
Now, when performing file operations, you can throw these custom errors based on the condition encountered. Here’s an example of a function that attempts to read a file and throws an error if the file is not found:
func readFile(at path: String) throws -> String { let fileURL = URL(fileURLWithPath: path) guard FileManager.default.fileExists(atPath: fileURL.path) else { throw FileError.fileNotFound } return try String(contentsOf: fileURL, encoding: .utf8) } do { let content = try readFile(at: "/path/to/file.txt") print("File content: (content)") } catch let error as FileError { print("File error: (error.localizedDescription)") } catch { print("An unexpected error occurred: (error)") }
This approach enhances error handling by allowing the developer to cater to specific error types in a more customized manner. Additionally, you can always include a fallback catch-all to handle any unforeseen errors.
Another common source of errors during file operations is permission issues. For instance, trying to write to a read-only file or attempting to access a directory without the necessary permissions can lead to failures. It’s important to manage these situations proactively by checking permissions before attempting operations:
let fileURL = documentsDirectory.appendingPathComponent("readonly.txt") do { let attributes = try fileManager.attributesOfItem(atPath: fileURL.path) if let permissions = attributes[.posixPermissions] as? NSNumber, permissions.intValue & 0o200 == 0 { throw FileError.insufficientPermissions } try "New Content".write(to: fileURL, atomically: true, encoding: .utf8) } catch { print("Error during file operation: (error.localizedDescription)") }
This snippet checks for write permissions before attempting to write to a file, ensuring that your application behaves predictably even under restrictive file system conditions.
Implementing effective error handling in file operations is paramount for building reliable Swift applications. Using `do-catch` blocks, defining custom error types, and proactively checking for permissions can significantly enhance the robustness of your file management code. With proper error handling, you can create applications that not only function correctly but also provide clear feedback when something goes wrong, ultimately improving the user experience.