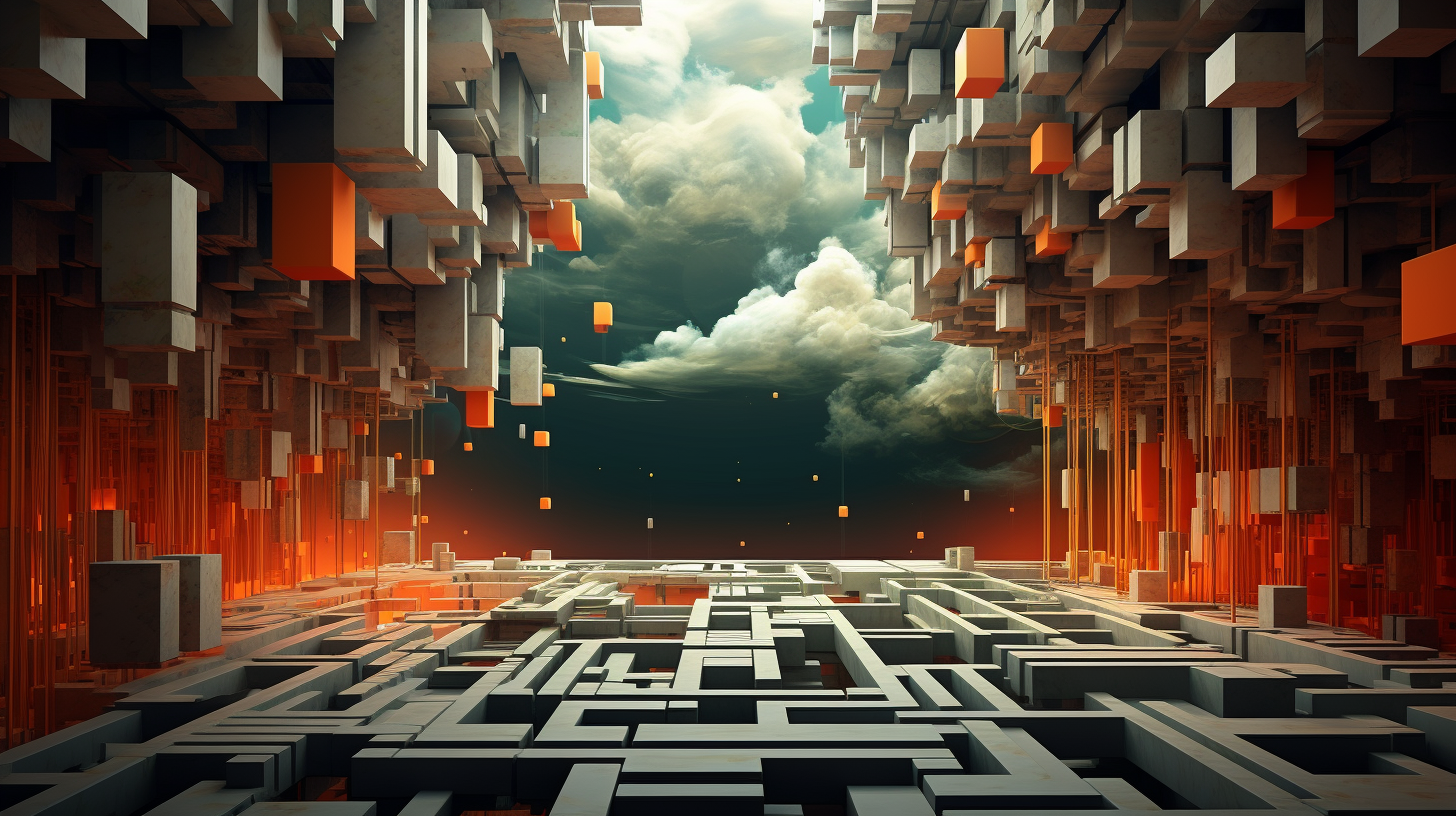
Using JavaScript for Server-Side Programming
Node.js is an open-source, cross-platform runtime environment that allows developers to execute JavaScript code server-side. It employs a non-blocking, event-driven architecture, which makes it efficient and suitable for I/O-heavy applications, such as web servers and real-time applications.
At the core of Node.js is the V8 JavaScript engine developed by Google. V8 compiles JavaScript into native machine code, enabling fast execution. This remarkable speed, combined with the ability to handle multiple connections at once, makes Node.js a popular choice for building scalable network applications.
One of the standout features of Node.js is its package ecosystem, npm (Node Package Manager). npm is the largest ecosystem of open-source libraries in the world, allowing developers to easily share and reuse code. With a simple command, you can install a package and integrate it into your project, significantly reducing development time.
Here’s how you can quickly install the Express framework, which is a minimal and flexible Node.js web application framework:
npm install express
Node.js embraces an asynchronous programming model, enabling developers to write non-blocking code. This is particularly crucial in a server environment where handling many concurrent requests efficiently is paramount.
In Node.js, callbacks, promises, and async/await are common patterns used to deal with asynchronous operations. For instance, using promises can help you manage the flow of asynchronous operations more cleanly. Here’s a simple example of reading a file asynchronously using promises:
const fs = require('fs').promises; async function readFile(filePath) { try { const data = await fs.readFile(filePath, 'utf8'); console.log(data); } catch (error) { console.error('Error reading the file:', error); } } readFile('example.txt');
Node.js encourages a modular approach to coding, where you can break your application into smaller, manageable components. This modularity not only keeps your code base organized but also enhances reusability. You can create modules using the CommonJS syntax or ES6 modules, depending on your preference and compatibility needs.
Setting Up a Server with Express
To set up a server with Express, the first step is to create a new Node.js project. This process begins by initializing a new npm project which creates a package.json file to manage your application’s dependencies and metadata. You can do this by running the following command in your terminal:
npm init -y
This command generates a default package.json file without prompting for configuration options. After initializing your project, you can proceed to install the Express framework, which provides a robust set of features for web and mobile applications.
npm install express
Once Express is installed, you can create a simple server. Open a new JavaScript file, for instance server.js, and include the following code to set up a basic Express server:
const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(port, () => { console.log(`Server is running at http://localhost:${port}`); });
In this example, we import the Express module, create an instance of an Express application, and define a route for the root URL (/). The app.get method takes two arguments: the path and a callback function. The callback receives the request and response objects and uses the res.send method to send a simple string back to the client.
Next, we call app.listen to start the server on the specified port. The callback function logs a confirmation message to the console, signaling that the server is up and running. You can run this server by executing the following command in your terminal:
node server.js
Now, if you open your web browser and navigate to http://localhost:3000, you should see the message “Hello, World!” displayed on the page. This simple example illustrates the foundational steps in setting up a server using Express.
Express also allows you to define multiple routes for different HTTP methods. For instance, you can create a POST route to handle form submissions. Updates to your server can be made with more complex routing and middleware for handling requests efficiently. Middleware functions are a powerful feature of Express; they can process requests before they reach your route handlers. Here’s how you can add a simple middleware function:
app.use((req, res, next) => { console.log(`${req.method} ${req.url}`); next(); });
This middleware logs the HTTP method and URL of incoming requests to the console. The next function is called to pass control to the next middleware or route handler in the stack.
Handling Asynchronous Programming
Asynchronous programming is a cornerstone of Node.js, allowing developers to write efficient code that handles multiple tasks concurrently without blocking the execution thread. In a typical server scenario, such as handling HTTP requests, if a function performs a long-running operation—like reading a file or querying a database—it can halt other operations if executed synchronously. Instead, using asynchronous patterns ensures that the server remains responsive while operations are processed in the background.
Node.js primarily utilizes callbacks, but the syntax can quickly become convoluted when dealing with multiple asynchronous operations, leading to what’s commonly referred to as “callback hell.” To mitigate this, JavaScript introduced promises and, more recently, the async/await syntax, which allows for writing asynchronous code in a more readable, synchronous-like manner.
Here’s a quick breakdown of how these different methods work:
Callbacks
Callbacks are functions that are passed as arguments to another function and are executed after the completion of that function. Here’s an example of using a callback to read a file:
const fs = require('fs'); fs.readFile('example.txt', 'utf8', (err, data) => { if (err) { return console.error('Error reading the file:', err); } console.log(data); });
While this works, nesting multiple callbacks can create deeply indented code that is difficult to read and maintain.
Promises
Promises provide a cleaner alternative to callbacks. A promise represents a value that may be available now, or in the future, or never. You can chain promises using the .then() and .catch() methods, which keeps your code more organized. Here’s the previous example refactored to use promises:
const fs = require('fs').promises; fs.readFile('example.txt', 'utf8') .then(data => { console.log(data); }) .catch(err => { console.error('Error reading the file:', err); });
With promises, you can handle errors in a centralized manner using .catch(), making your code less error-prone and more readable.
Async/Await
The async/await syntax, introduced in ES2017, allows developers to write asynchronous code that looks synchronous. You mark a function as async, and inside it, you can use the await keyword to pause execution until the promise is resolved. This makes the code much easier to follow. Here’s how you would rewrite the previous example using async/await:
const fs = require('fs').promises; async function readFile() { try { const data = await fs.readFile('example.txt', 'utf8'); console.log(data); } catch (err) { console.error('Error reading the file:', err); } } readFile();
In this example, the async function readFile() is defined to handle reading the file. The await keyword is used to pause execution until fs.readFile resolves, making it clear where the program is waiting for asynchronous work to complete.
Understanding these asynchronous patterns very important for effectively using Node.js. By mastering callbacks, promises, and async/await, you can write clean, efficient code that harnesses the full potential of Node.js’s non-blocking architecture. This mastery allows for the development of robust applications capable of handling a high number of concurrent requests without sacrificing performance or readability.
Interfacing with Databases Using JavaScript
When it comes to interfacing with databases using JavaScript in a Node.js environment, the choice of database often depends on the application’s requirements. While you can use traditional SQL databases like MySQL or PostgreSQL, NoSQL databases such as MongoDB have gained immense popularity due to their flexibility and scalability. Each has its own libraries and methodologies for integration, but all provide the means to perform CRUD (Create, Read, Update, Delete) operations effectively.
To kick things off, let’s explore how to connect to a MongoDB database using the popular Mongoose library, which provides a simpler way to interact with MongoDB through a schema-based solution. First, you need to install the Mongoose package:
npm install mongoose
Once Mongoose is installed, you can establish a connection to your MongoDB instance. The following example demonstrates how to connect to a local MongoDB server and define a schema for a simple user model:
const mongoose = require('mongoose'); // Connect to MongoDB mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true }) .then(() => console.log('MongoDB connected...')) .catch(err => console.error('Could not connect to MongoDB:', err)); // Define a user schema const userSchema = new mongoose.Schema({ name: { type: String, required: true }, email: { type: String, required: true, unique: true }, password: { type: String, required: true } }); // Create a user model const User = mongoose.model('User', userSchema);
In this snippet, we first establish a connection to a MongoDB database named “mydatabase.” We utilize Mongoose’s connection promise to log whether the connection was successful or not. Next, we define a simple user schema with fields for name, email, and password. Finally, we create a User model based on this schema, which we can use to interact with the users’ collection in the database.
Now that we have set up our database connection and defined a model, we can perform CRUD operations. Below is an example of how to create a new user and save it in the database:
async function createUser(name, email, password) { const user = new User({ name, email, password }); try { const savedUser = await user.save(); console.log('User created:', savedUser); } catch (error) { console.error('Error creating user:', error); } } createUser('Alex Stein', '[email protected]', 'securepassword');
In this function, we create a new instance of the User model and call the save()
method to persist the user to the database. Using async/await simplifies error handling and keeps the code readable.
For reading user data, you can use the find()
method provided by Mongoose. Here’s how to retrieve all users from the database:
async function getUsers() { try { const users = await User.find(); console.log('Users:', users); } catch (error) { console.error('Error retrieving users:', error); } } getUsers();
This function fetches all user documents from the database and logs them to the console. Mongoose also supports querying the database with various conditions, which allows you to filter results effectively.
Updating a user’s information is equally simpler. Below is a function to update a user’s email based on their ID:
async function updateUser(id, newEmail) { try { const updatedUser = await User.findByIdAndUpdate(id, { email: newEmail }, { new: true }); console.log('Updated User:', updatedUser); } catch (error) { console.error('Error updating user:', error); } } // Assume we have a user ID to update updateUser('60f5b8d2bfc0b557bc2f0a2e', '[email protected]');
In the updateUser
function, we use findByIdAndUpdate
to locate the user and update their email. The option { new: true }
ensures that the function returns the modified document.
Finally, deleting a user can be accomplished with the findByIdAndDelete
method:
async function deleteUser(id) { try { await User.findByIdAndDelete(id); console.log('User deleted successfully'); } catch (error) { console.error('Error deleting user:', error); } } // Assume we have a user ID to delete deleteUser('60f5b8d2bfc0b557bc2f0a2e');
Using the deleteUser
function, we can remove a user from the database. This illustrates how easily Node.js can handle database operations using Mongoose, providing a powerful yet flexible interface for data management.
It would be beneficial to include a mention of security best practices when working with Node.js, particularly regarding database interactions. For instance, discussing the importance of validating user input, using environment variables for sensitive information, implementing proper authentication and authorization methods, and using libraries like dotenv for managing environment variables could significantly enhance the article. Additionally, emphasizing the need for securely handling passwords (e.g., using bcrypt for hashing) would provide a well-rounded view of best practices in developing secure applications with Node.js.