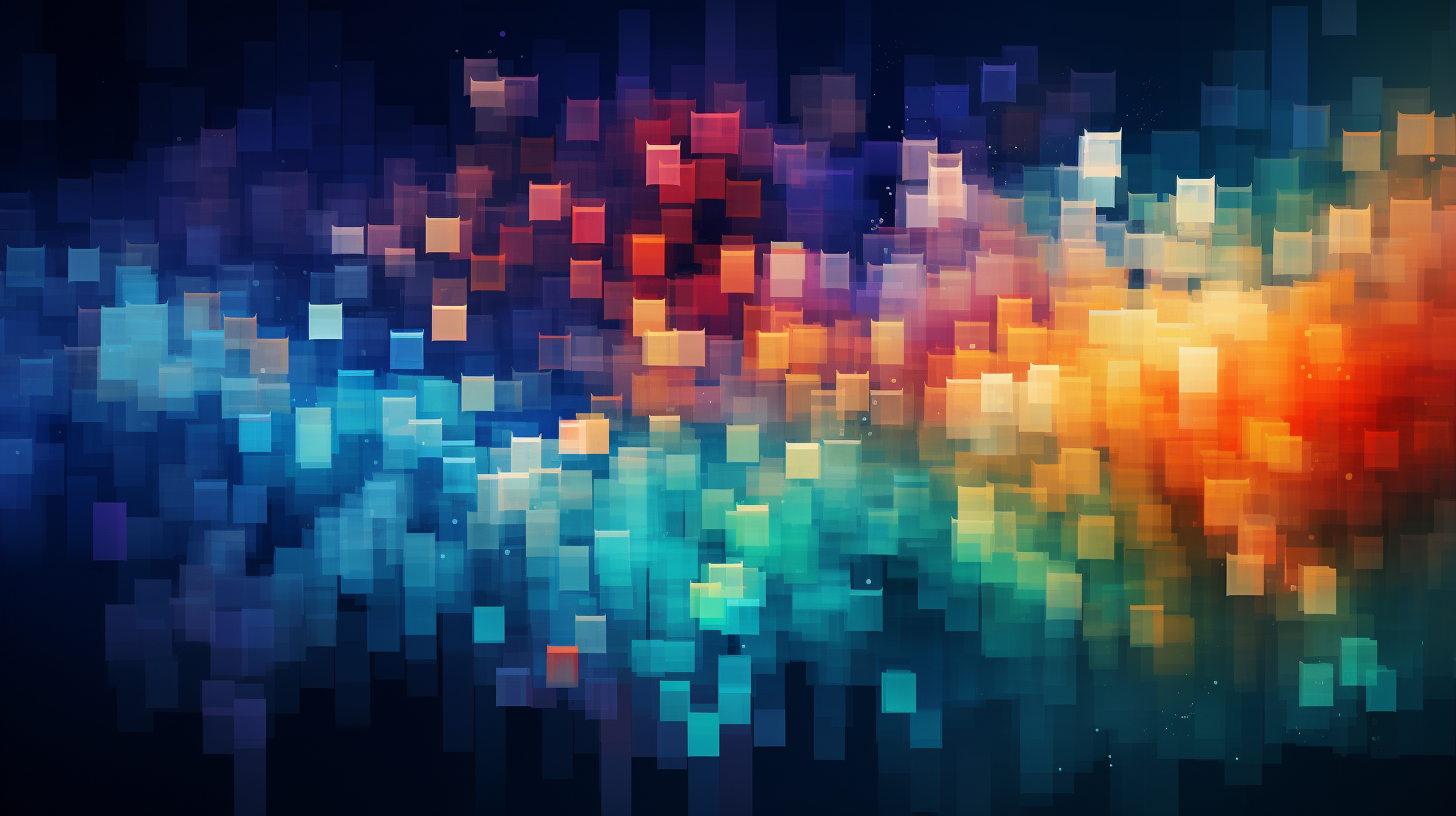
SQL for Data Integrity Checks
Data integrity in SQL is a critical aspect that ensures the accuracy and reliability of data within a database. It refers to the maintenance of, and the assurance of, the accuracy and consistency of data over its entire lifecycle. The importance of data integrity cannot be overstated; it affects all aspects of data management, from the way applications interact with the database to how data is reported and analyzed.
When we talk about data integrity, we are really addressing several key points:
- Data must be correct and precisely reflect the real-world entities they represent. Incorrect data can lead to flawed analysis and decisions.
- Data should remain consistent throughout its lifecycle, meaning it must not contradict itself within a database or across multiple databases.
- All required data must be present. Missing information can severely limit the usefulness of data.
- Data must adhere to defined formats, ranges, or other rules. This ensures that only acceptable data can be entered into the database.
- In many cases, certain data entries must be unique across the dataset, preventing duplicates which can skew analysis.
To effectively manage and enforce data integrity, SQL provides various constraints and mechanisms. The integrity of data can be ensured through:
- Defining constraints that prevent invalid data from being inserted or updated.
- Implementing proper database normalization to minimize redundancy and dependency.
- Using transactions to maintain consistency, ensuring that all operations within a transaction are completed successfully before committing to the database.
For example, when creating a table, you can implement constraints like so:
CREATE TABLE Employees ( EmployeeID INT PRIMARY KEY, FirstName VARCHAR(100) NOT NULL, LastName VARCHAR(100) NOT NULL, Email VARCHAR(100) UNIQUE, HireDate DATE NOT NULL CHECK (HireDate >= '2000-01-01') );
In this SQL statement, several aspects of data integrity are enforced:
- The
PRIMARY KEY
constraint ensures that eachEmployeeID
is unique. - The
NOT NULL
constraint onFirstName
,LastName
, andHireDate
guarantees that these fields cannot be empty. - The
UNIQUE
constraint onEmail
ensures that no two employees can have the same email address. - The
CHECK
constraint onHireDate
enforces that the hire date must be valid and not earlier than January 1, 2000.
By understanding and implementing these principles of data integrity within SQL, developers can ensure that the data being managed is both reliable and actionable.
Types of Data Integrity Constraints
When discussing the types of data integrity constraints in SQL, we delve into specific mechanisms that help maintain the inherent accuracy, consistency, and reliability of data. Various constraints can be applied to enforce data integrity effectively. These constraints can be broadly categorized into several types: primary keys, foreign keys, unique constraints, not null constraints, and check constraints.
Primary Key Constraints
The primary key constraint is pivotal in relational databases as it uniquely identifies each record in a table. A primary key must contain unique values, and it cannot contain NULL values. By defining a primary key, we ensure that each entry in a table is distinct, thereby preventing any ambiguity in data retrieval.
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100) NOT NULL, Price DECIMAL(10, 2) NOT NULL );
Foreign Key Constraints
Foreign key constraints establish a relationship between two tables. A foreign key in one table points to a primary key in another table, ensuring referential integrity. This relationship prevents actions that would leave orphaned records in the database. When a foreign key constraint is defined, any attempt to insert a record in the child table that does not have a corresponding record in the parent table will fail.
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, ProductID INT, OrderDate DATE NOT NULL, FOREIGN KEY (ProductID) REFERENCES Products(ProductID) );
Unique Constraints
A unique constraint ensures that all values in a column are distinct from one another. Unlike primary keys, a table can have multiple unique constraints, and these constraints can also accept NULL values (though only one NULL per unique column). Unique constraints are particularly useful for enforcing business rules, such as ensuring that usernames or emails are unique across user accounts.
CREATE TABLE Users ( UserID INT PRIMARY KEY, Username VARCHAR(50) UNIQUE, Email VARCHAR(100) UNIQUE );
Not Null Constraints
The not null constraint ensures that a column cannot contain NULL values. This constraint is essential for fields that are critical for the business logic of the application, as it mandates that essential data must always be present. Not null constraints help maintain the completeness of the data by preventing incomplete records.
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, FirstName VARCHAR(50) NOT NULL, LastName VARCHAR(50) NOT NULL );
Check Constraints
Check constraints enforce a specific condition on the values in one or more columns. This allows for validation of data based on business rules at the time of data entry. For example, a check constraint could ensure that an age column only contains values greater than or equal to 18, thus enforcing the business requirement that all customers must be adults.
CREATE TABLE Employees ( EmployeeID INT PRIMARY KEY, Age INT CHECK (Age >= 18) );
By using these types of data integrity constraints, database designers and developers can enforce rules that ensure data remains accurate and reliable throughout its lifecycle. Constraints effectively serve as guards against erroneous data entry, helping to maintain the quality of the database while providing a structural framework for data management.
Implementing Primary and Foreign Keys
Implementing primary and foreign keys is essential for enforcing data integrity within relational databases. The primary key serves as a unique identifier for each record in a table, while foreign keys establish relationships between tables, ensuring that these relationships are maintained correctly. This subsection will delve into how to implement these keys effectively, illustrating their significance through practical SQL examples.
To begin with, defining a primary key is a critical step in table creation. A primary key must be unique and cannot contain NULL values, thus guaranteeing that every record can be uniquely referenced. Consider the following SQL statement for creating a table with a primary key:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, FirstName VARCHAR(50) NOT NULL, LastName VARCHAR(50) NOT NULL, Email VARCHAR(100) UNIQUE );
In this example, the CustomerID serves as the primary key. This ensures that each customer can be uniquely identified, preventing any ambiguity in data operations.
Next, foreign keys are utilized to establish relationships between tables, thereby enforcing referential integrity. A foreign key in one table references a primary key in another table, ensuring that relationships between records remain valid. For instance, when creating an Orders table that references the Customers table, the SQL statement would look like this:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, OrderDate DATE NOT NULL, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
Here, CustomerID in the Orders table acts as a foreign key that links each order to the corresponding customer. This relationship ensures that every order is associated with a valid customer, preventing orphaned records.
When implementing these keys, it’s essential to think what happens during deletion or updates. By default, if a record referenced by a foreign key is deleted, it can lead to orphan records unless proper actions are defined. SQL allows for the specification of cascading actions using ON DELETE CASCADE or ON UPDATE CASCADE. Here’s how to implement these actions:
CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, OrderDate DATE NOT NULL, FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) ON DELETE CASCADE ON UPDATE CASCADE );
In this scenario, if a customer is deleted, all associated orders will automatically be removed, maintaining the integrity of the data. Similarly, if the CustomerID is updated in the Customers table, the changes will propagate to the Orders table, ensuring consistency across related records.
Implementing primary and foreign keys not only ensures data integrity but also enhances the relational model of the database, allowing for complex queries and data retrievals that respect the inherent relationships between tables. By carefully designing these keys and their constraints, database administrators can maintain a robust, reliable system that accurately reflects business rules and operational needs.
Using CHECK Constraints for Data Validation
CHECK constraints are a powerful feature in SQL that allow developers to enforce specific conditions on the data being entered into a table. By defining these constraints, you can ensure that only valid data that meets certain criteria can be stored in the database. This is particularly important for maintaining data integrity, as it prevents erroneous or out-of-range values from being recorded, which could otherwise compromise the reliability of the dataset.
The syntax for creating a CHECK constraint is quite simpler. It can be specified directly in the table definition during the creation phase or added later using an ALTER TABLE statement. The condition specified in a CHECK constraint can reference one or more columns within the table.
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100) NOT NULL, Quantity INT CHECK (Quantity >= 0), Price DECIMAL(10, 2) CHECK (Price > 0) );
In this example, the CHECK constraints on the Quantity
and Price
columns ensure that no product can have a negative quantity or a price less than or equal to zero. Such validations are vital for maintaining logical consistency in product data, as negative quantities or zero prices do not make sense in a typical product inventory context.
CHECK constraints can also be used to enforce more complex rules. For instance, you might want to ensure that a date column reflects a certain range based on business logic. In the following example, we ensure that the ExpirationDate
of a product must always be later than the ManufactureDate
:
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100) NOT NULL, ManufactureDate DATE NOT NULL, ExpirationDate DATE NOT NULL, CHECK (ExpirationDate > ManufactureDate) );
In this case, the CHECK constraint actively prevents the entry of any product where the expiration date is earlier than the manufacture date, thereby preserving the integrity of this product lifecycle data.
Moreover, CHECK constraints can be applied to string data as well. For instance, if you have an employee table, and you want to ensure that the JobTitle
column only contains certain predefined titles, you can use a CHECK constraint to enforce this rule:
CREATE TABLE Employees ( EmployeeID INT PRIMARY KEY, FirstName VARCHAR(50) NOT NULL, LastName VARCHAR(50) NOT NULL, JobTitle VARCHAR(50) CHECK (JobTitle IN ('Manager', 'Developer', 'Analyst')), Salary DECIMAL(10, 2) CHECK (Salary > 0) );
This constraint restricts the JobTitle
entries to only the specified values, preventing invalid job titles from being recorded and ensuring that salary values are positive.
However, while CHECK constraints are powerful, they should be used judiciously. Overly complex CHECK conditions can lead to performance issues, especially when dealing with large datasets, as they require additional evaluations during insert and update operations. Thus, it’s essential to strike a balance between data validation needs and performance considerations.
Implementing CHECK constraints in your SQL tables is a vital part of ensuring data integrity. By enforcing rules on data entry, you significantly reduce the risk of invalid data being recorded, which in turn enhances the quality and reliability of your database.
Monitoring Data Integrity with Triggers
Monitoring data integrity with triggers is an advanced technique that allows developers to enforce and maintain the rules of data integrity dynamically as data is manipulated within the database. Triggers are special types of stored procedures that automatically execute in response to specific events on a particular table, such as INSERT, UPDATE, or DELETE operations. By using triggers, you can implement complex business rules and validations that might not be directly feasible through standard constraints alone.
To create a trigger, you first define the conditions under which the trigger should fire. This expression can be quite flexible, allowing for the execution of custom logic every time relevant data changes. For instance, think a scenario where you want to ensure that no employee can be removed from the database if they have any associated records in the ‘Projects’ table. You can implement a trigger like this:
CREATE TRIGGER prevent_employee_deletion BEFORE DELETE ON Employees FOR EACH ROW BEGIN DECLARE project_count INT; SELECT COUNT(*) INTO project_count FROM Projects WHERE EmployeeID = OLD.EmployeeID; IF project_count > 0 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Cannot delete employee with active projects.'; END IF; END;
In this example, the trigger, named prevent_employee_deletion, is set to execute before any DELETE operation on the Employees table. It checks how many projects are associated with the employee being deleted. If the count is greater than zero, the trigger raises an error, preventing the deletion and maintaining data integrity by ensuring that no orphaned records exist in the Projects table.
Triggers can also be used to automate the maintenance of related data. For instance, you might want to automatically update a last_modified timestamp column in your table whenever a row is updated. This can be accomplished with a simple trigger like this:
CREATE TRIGGER update_last_modified BEFORE UPDATE ON Employees FOR EACH ROW BEGIN SET NEW.last_modified = NOW(); END;
Here, the update_last_modified trigger updates the last_modified column to the current timestamp whenever an employee’s record is updated. This implicit tracking of changes can be extremely useful for auditing purposes and ensures that the data reflects the most recent modifications.
However, one must tread carefully when using triggers. They can introduce complexity into the database management process, especially if multiple triggers interact in unexpected ways. Additionally, triggers can impact performance, as they introduce additional processing overhead for each qualifying data manipulation event. Therefore, it is essential to use triggers judiciously and to document their behavior clearly to avoid confusion for future maintainers of the code.
Triggers are a powerful tool for monitoring and enforcing data integrity in SQL databases. They allow for dynamic enforcement of business rules and maintain referential integrity by automatically responding to data changes. When applied correctly, triggers can significantly enhance the robustness and reliability of your database management practices.
Best Practices for Maintaining Data Integrity
When it comes to maintaining data integrity within SQL databases, adhering to best practices is essential. These practices not only help in upholding the accuracy and reliability of data but also aid in preventing errors before they occur. Here are key strategies that database administrators and developers can follow to ensure data integrity remains intact.
1. Use Constraints Wisely: Constraints are the first line of defense in maintaining data integrity. Make sure to use primary keys, foreign keys, unique constraints, not null constraints, and check constraints appropriately. Each constraint serves a specific purpose, and their correct implementation can prevent invalid data entries from occurring. For instance, always define NOT NULL constraints for critical fields to avoid incomplete records.
CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100) NOT NULL, Quantity INT CHECK (Quantity >= 0) NOT NULL, Price DECIMAL(10, 2) CHECK (Price > 0) NOT NULL );
2. Regularly Audit Data: Frequent audits of the data can help uncover inconsistencies and errors that may have slipped through during data entry. Implement scripts or stored procedures that periodically check for anomalies, such as duplicate entries or records that violate check constraints. Early detection of data integrity violations can save time and resources in the long run.
SELECT ProductName, COUNT(*) FROM Products GROUP BY ProductName HAVING COUNT(*) > 1;
3. Employ Transactions: Whenever making changes to the database, especially for multiple related operations, use transactions. Transactions ensure that the database remains in a consistent state by which will allow you to commit or roll back changes as a single unit of work. This approach helps prevent partial updates that could lead to data integrity issues.
BEGIN TRANSACTION; UPDATE Inventory SET Quantity = Quantity - 1 WHERE ProductID = 1; INSERT INTO Sales (ProductID, SaleDate) VALUES (1, NOW()); COMMIT;
4. Utilize Triggers for Complex Validations: While triggers can complicate the database logic, they offer a powerful mechanism for enforcing business rules. Use triggers to automatically check conditions and maintain integrity during data modifications. Make sure to craft triggers that are simpler and well-documented to facilitate future modifications and troubleshooting.
CREATE TRIGGER check_product_quantity BEFORE INSERT ON Sales FOR EACH ROW BEGIN DECLARE available_quantity INT; SELECT Quantity INTO available_quantity FROM Inventory WHERE ProductID = NEW.ProductID; IF available_quantity < 1 THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Insufficient quantity for sale.'; END IF; END;
5. Adopt Database Normalization: Normalize your database to reduce redundancy and improve data integrity. Proper normalization involves structuring the database in a way that minimizes duplication and dependencies, which in turn helps maintain consistency across related tables.
6. Backup Data Regularly: Regular data backups are essential to safeguard against data loss. By having consistent backups, you can restore data to a previous state in case of corruption or integrity violations. Automated backup schedules can help ensure that this practice becomes part of your routine without additional effort.
7. Educate Users and Developers: Finally, educating everyone who interacts with the database—whether developers, users, or administrators—about the importance of data integrity is important. Encourage best practices in data entry and manipulation, and provide training on how to use the constraints and transactions effectively.
By following these best practices, you can create a robust environment that not only maintains data integrity but also enhances the overall performance and reliability of your SQL databases. The proactive management of data integrity challenges will pay dividends in the form of cleaner, more reliable data that accurately reflects your business operations.