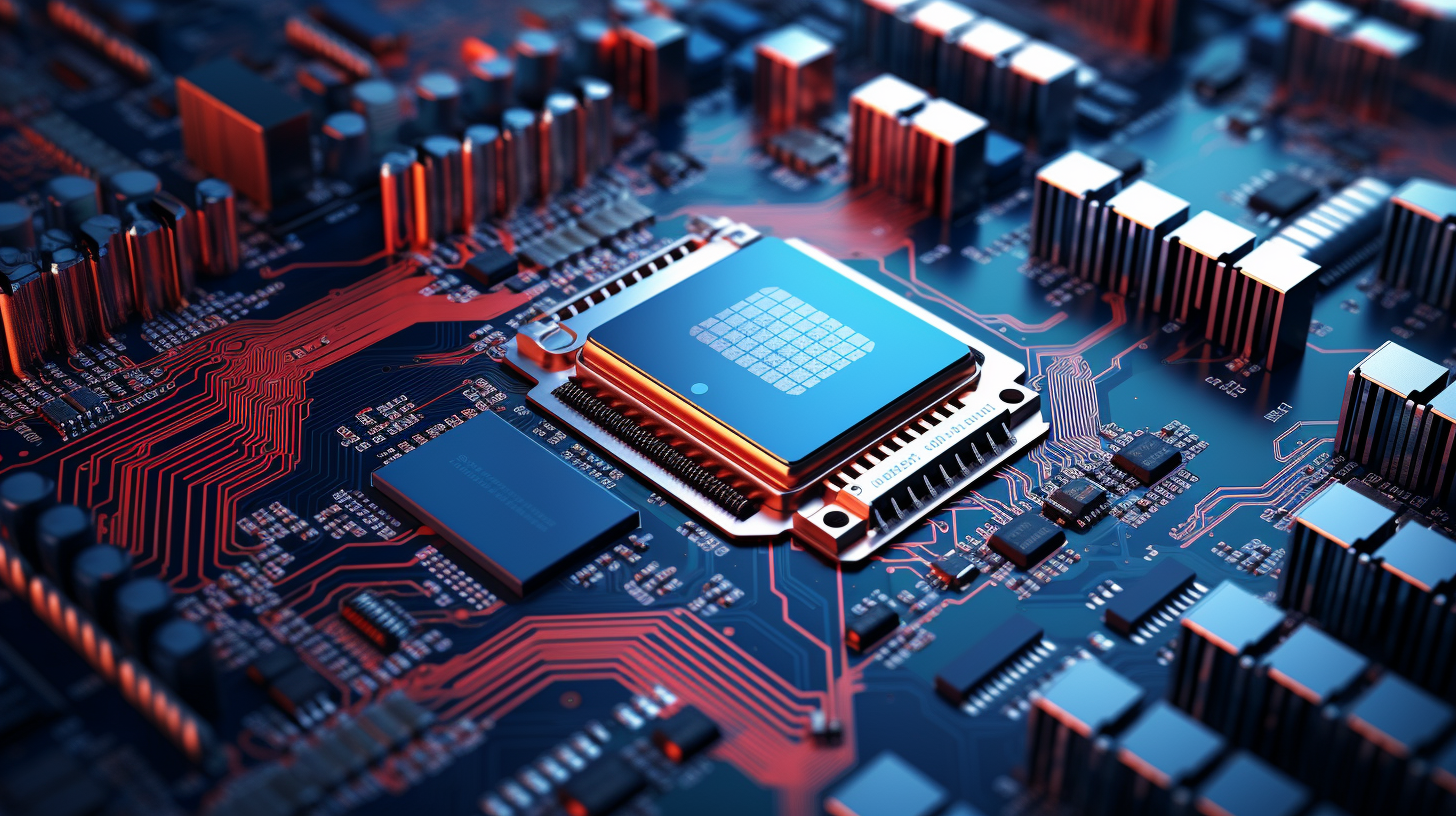
Python and Databases: SQL and NoSQL
At the core of many applications lies the need for structured data management, and that is where SQL databases come into play. SQL, or Structured Query Language, is a powerful tool for interacting with relational databases, which store data in a structured format using tables. Each table consists of rows and columns, allowing for organized data storage and retrieval. The relationships between these tables can be defined using foreign keys, enabling complex queries that can extract meaningful insights from the data.
SQL databases are ideally suited for applications where data integrity and transactional reliability are paramount. They enforce strict schemas, meaning that the structure of the data must be defined upfront. This characteristic allows for robust validation of data integrity, ensuring that only valid data is entered into the database. Examples of popular SQL databases include PostgreSQL, MySQL, and SQLite, each with its own strengths and use cases.
To interact with SQL databases in Python, the SQLite module provides a lightweight and easy-to-use interface. Here’s a simple example demonstrating how to create a database, define a table, and insert data using SQLite:
import sqlite3 # Connect to a database (or create it if it doesn't exist) conn = sqlite3.connect('example.db') # Create a cursor object to execute SQL commands cursor = conn.cursor() # Create a table cursor.execute(''' CREATE TABLE users ( id INTEGER PRIMARY KEY, name TEXT NOT NULL, email TEXT NOT NULL UNIQUE ) ''') # Insert a new user into the table cursor.execute(''' INSERT INTO users (name, email) VALUES (?, ?) ''', ('Alice', '[email protected]')) # Commit the changes and close the connection conn.commit() conn.close()
In this example, we first establish a connection to a SQLite database file named example.db. If the file does not exist, it will be created automatically. Next, we use a cursor to execute SQL commands. We define a table called users with three columns: id, name, and email. The id is an integer that serves as the primary key, while email is defined as unique to avoid duplicate entries.
Once the table is set up, we insert a new user, Alice, into the table. The use of placeholders (the ? in the SQL command) helps prevent SQL injection attacks, making the insertion process safer. Finally, changes are committed to the database, and the connection is closed cleanly.
This fundamental understanding of SQL databases and their operation in Python sets the stage for more complex interactions, such as querying data, updating records, and managing relationships between tables—all of which are essential skills for any Python developer working with relational databases.
Exploring NoSQL Databases
As we venture into the realm of NoSQL databases, we encounter a different paradigm of data management designed to address the limitations often associated with traditional SQL databases. NoSQL, which stands for “Not Only SQL,” encompasses a range of database technologies that prioritize flexibility, scalability, and performance, particularly for unstructured and semi-structured data.
NoSQL databases come in various types, each catering to specific use cases. The primary categories include:
- These databases store data in documents, typically JSON or BSON format. Each document is self-describing, which means it can contain varying fields and structures. Popular examples include MongoDB and CouchDB.
- As the simplest type of NoSQL database, key-value stores store data as a collection of key-value pairs. They provide high performance for read and write operations. Redis and DynamoDB are widely used key-value stores.
- These databases store data in columns rather than rows, which can be beneficial for analytical workloads. Apache Cassandra and HBase fall under this category.
- Designed to represent and navigate relationships between data points, graph databases excel in scenarios where relationships are deeply interconnected. Neo4j is a well-known graph database.
One of the defining features of NoSQL databases is their schema-less nature. Unlike SQL databases that require predefined schemas, NoSQL databases allow for dynamic structures, enabling developers to iterate rapidly without the need for extensive migrations or alterations to existing data models. This flexibility can significantly speed up the development cycle, especially in agile environments.
Moreover, NoSQL databases are designed to scale horizontally, meaning they can handle increased loads by adding more servers rather than upgrading existing ones. This characteristic makes them particularly suited for applications experiencing rapid growth or unpredictable traffic patterns.
To illustrate how to work with a NoSQL database in Python, let us think MongoDB, a popular document store. Below is a simple example demonstrating how to connect to a MongoDB database, create a collection, and insert a document:
from pymongo import MongoClient # Connect to the MongoDB server client = MongoClient('localhost', 27017) # Create or switch to a database db = client['example_db'] # Create or switch to a collection users_collection = db['users'] # Insert a new user document user = { 'name': 'Bob', 'email': '[email protected]', 'age': 30 } result = users_collection.insert_one(user) print(f'User inserted with id: {result.inserted_id}')
In this example, we utilize the pymongo
library to connect to a MongoDB server running on localhost. We create or switch to a database named example_db
and a collection called users
. The insert operation is straightforward; we define a user document and insert it into the collection. Finally, we print the ID of the newly inserted document.
This simple workflow highlights the ease of use and flexibility provided by NoSQL databases. As we continue exploring the capabilities of NoSQL, we will see how these systems can handle complex data structures and relationships, further expanding the toolbox available to Python developers.
Comparative Analysis: SQL vs NoSQL
When comparing SQL and NoSQL databases, it’s essential to understand the strengths and weaknesses of each approach, as well as the specific use cases where one may be more suitable than the other. SQL databases have a long history and are well-established in the industry. Their rigid structure and adherence to ACID (Atomicity, Consistency, Isolation, Durability) principles ensure data integrity and reliability, making them perfect for applications that require strong transactional support, such as financial systems or enterprise resource planning (ERP) software. The use of SQL as a query language allows developers to perform complex queries and joins, providing powerful data manipulation capabilities.
In contrast, NoSQL databases emerged from the need for flexibility and scalability in modern applications that deal with vast amounts of varied data. Their schema-less nature allows developers to store unstructured and semi-structured data seamlessly, adapting as the application evolves. This adaptability is particularly advantageous for startups and agile teams who need to iterate quickly without being constrained by rigid database schemas. However, this flexibility comes at the cost of some transactional guarantees, as many NoSQL databases embrace eventual consistency rather than strict ACID compliance.
Performance is another key factor in the SQL versus NoSQL debate. SQL databases excel in scenarios requiring complex transactions and joins across multiple tables, but they can struggle with high write loads or large-scale read operations. In contrast, NoSQL databases are designed for high throughput and horizontal scaling, enabling them to handle massive workloads by distributing data across multiple servers. This makes NoSQL an attractive choice for applications such as social networks, real-time analytics, and content management systems, where speed and scalability are critical.
Below is a performance comparison between SQL and NoSQL databases across several dimensions:
- SQL databases are structured and enforce schemas, while NoSQL databases are schema-less and can accommodate various data types.
- SQL databases ensure strong transactional support (ACID), whereas NoSQL databases often favor eventual consistency.
- NoSQL databases provide superior horizontal scalability, making it easier to manage large volumes of data and high traffic.
- SQL databases support complex queries with JOINs, while NoSQL databases typically rely on simpler querying methods.
When developing applications, the choice between SQL and NoSQL should be guided by the specific requirements of the project. For instance, if data integrity is paramount and the application requires complex queries, a SQL database would likely be the better option. Conversely, if the application needs to handle varying data formats and scale rapidly, a NoSQL solution could provide the necessary flexibility and performance.
To illustrate how each type of database can effectively manage data in different scenarios, consider the following examples:
# SQL example: Querying user data with a JOIN import sqlite3 # Connect to the SQLite database conn = sqlite3.connect('example.db') cursor = conn.cursor() # Query to fetch user data along with their email cursor.execute(''' SELECT name, email FROM users ''') # Fetch and print all results users = cursor.fetchall() for user in users: print(f'User: {user[0]}, Email: {user[1]}') conn.close()
# NoSQL example: Querying user data in MongoDB from pymongo import MongoClient # Connect to the MongoDB server client = MongoClient('localhost', 27017) db = client['example_db'] users_collection = db['users'] # Query to find users with a specific age age_query = {'age': 30} matching_users = users_collection.find(age_query) # Print matching users for user in matching_users: print(f'User: {user["name"]}, Email: {user["email"]}') client.close()
Ultimately, the decision between SQL and NoSQL is not merely about choosing one over the other; it can also involve a hybrid approach where both types of databases coexist within an application. This enables developers to leverage the strengths of each technology, optimizing for both data integrity and flexibility as needed. Understanding these nuances will empower Python developers to make informed decisions when architecting their applications, ensuring that they select the right tools for the specific challenges they face.
Best Practices for Database Integration in Python
When it comes to integrating databases with Python applications, following best practices very important for ensuring efficient, maintainable, and secure data handling. The choice of database, whether SQL or NoSQL, plays a significant role, but the way you interact with these databases in your Python code is equally important. Here are some key best practices to consider when integrating databases in Python.
1. Choose the Right ORM or Database Driver
Using an Object-Relational Mapping (ORM) library can simplify the interaction between your Python application and the database. Libraries like SQLAlchemy for SQL databases or MongoEngine for MongoDB can help abstract underlying database details and allow you to work with Python objects instead of raw SQL queries or document structures. This can lead to cleaner, more maintainable code.
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker # Create a new base class for declarative models Base = declarative_base() # Define a User model class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) email = Column(String, unique=True) # Create a database engine engine = create_engine('sqlite:///example.db') # Create the users table Base.metadata.create_all(engine) # Create a session Session = sessionmaker(bind=engine) session = Session() # Add a new user new_user = User(name='Charlie', email='[email protected]') session.add(new_user) session.commit() session.close()
2. Use Connection Pooling
Establishing a new connection to the database for every query can introduce significant overhead. Using connection pooling allows your application to reuse existing connections, improving performance and reducing latency. Libraries such as SQLAlchemy come with built-in support for connection pooling, which can be configured to match the expected workload.
3. Handle Exceptions Gracefully
Database operations can fail for various reasons, such as network issues, constraints violations, or unexpected data types. It is crucial to handle these exceptions proactively to prevent your application from crashing and to provide meaningful feedback to users. Use try-except blocks to catch exceptions and implement retry logic where appropriate.
try: session.add(new_user) session.commit() except Exception as e: session.rollback() print(f'Error occurred: {e}') finally: session.close()
4. Sanitize Inputs to Prevent SQL Injection
When working with SQL databases, it is vital to use parameterized queries or ORM methods to prevent SQL injection attacks. Raw SQL queries should be avoided whenever possible, especially when user input is involved. By using placeholders (like the ? in SQLite or :param in SQLAlchemy), you can protect your application from malicious input.
# Example of a parameterized query user_id = 1 cursor.execute('SELECT name, email FROM users WHERE id = ?', (user_id,))
5. Optimize Queries and Indexes
As your application grows, so does the volume of data in your database. It’s important to analyze and optimize queries for performance. Use database indexes to speed up data retrieval for frequently accessed columns. Profiling your queries can help identify bottlenecks, and tools like the EXPLAIN command in SQL can provide insights into query execution plans.
CREATE INDEX idx_email ON users(email);
6. Monitor and Log Database Activity
Monitoring database performance and logging activities can help identify issues before they escalate. Use logging libraries to record database interactions, errors, and performance metrics. This information can be invaluable when diagnosing problems and understanding how your application interacts with the database over time.
By adopting these best practices, developers can create Python applications that not only interact effectively with SQL and NoSQL databases but also maintain high standards of performance, security, and maintainability. The integration of robust database practices paves the way for scalable applications that meet user demands while minimizing the risk of data-related issues.