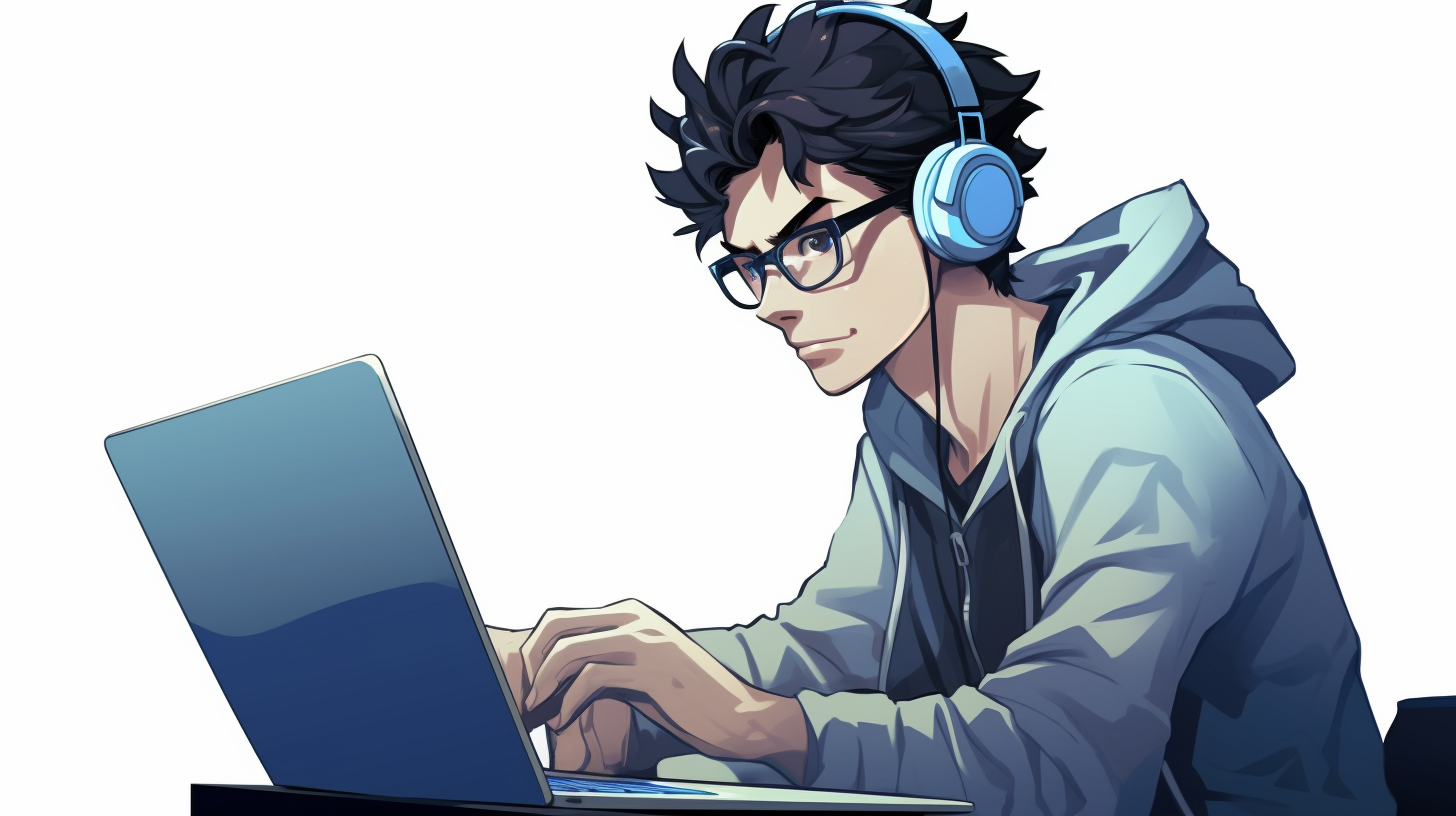
UI Testing in Swift
In the sphere of Swift development, UI testing plays an important role in ensuring that user interfaces function as intended across various scenarios. The primary framework for UI testing in Swift is the XCTest framework, which is integrated with Xcode. XCTest allows developers to write test cases that can automate the interaction with the user interface of their applications.
At its core, UI testing is about simulating user interactions. This involves tapping buttons, entering text into fields, and verifying that the expected elements are present on the screen. XCTest provides a rich set of APIs to interact with UI elements through the use of accessibility identifiers, which should be assigned to every UI element that needs to be tested.
One of the most powerful features of XCTest is its ability to run tests on actual devices or simulators, allowing developers to validate their UI under conditions that closely resemble real-world usage. Additionally, UI tests can be integrated into continuous integration (CI) pipelines, ensuring that UI regressions are caught early in the development cycle.
To get started with UI testing in Swift, you typically create a new target in your Xcode project specifically for UI tests. This involves selecting the “UI Testing Bundle” option when adding a new target. Once that’s set up, you can start writing your test cases.
Each UI test case is a subclass of XCTestCase
and can utilize methods like XCUIApplication
to launch your app and interact with it. Here’s a simple example that demonstrates how to tap a button and verify a label’s text:
import XCTest class MyAppUITests: XCTestCase { func testButtonTapChangesLabelText() { let app = XCUIApplication() app.launch() let button = app.buttons["Tap Me"] button.tap() let label = app.staticTexts["Hello, World!"] XCTAssertEqual(label.label, "Hello, World!") } }
When writing UI tests, it is essential to ensure that your tests are resilient and do not depend on specific timing. XCTest provides features like expectation
and waitForExpectations
to help with synchronization, allowing tests to wait for certain conditions before proceeding.
Setting Up a UI Testing Environment
Setting up a UI testing environment in Swift is a critical step that lays the groundwork for effective automated testing. The process begins with ensuring that your Xcode project is ready to support UI tests. You need to create a UI Testing target within your project. This is done by navigating to your project settings, selecting the “Targets” section, and then clicking the “+” button to add a new target. When prompted, choose “UI Testing Bundle” from the template options.
Once your UI Testing target is created, Xcode will automatically generate a basic structure, including a test class that subclasses XCTestCase. This setup allows you to start writing your tests immediately, using the XCTest framework’s capabilities.
Next, you must configure accessibility identifiers for your UI elements. Accessibility identifiers are crucial for enabling XCTest to locate and interact with these elements reliably. To add an accessibility identifier, simply set the accessibilityIdentifier
property on your UI components in your app’s code. For instance:
button.accessibilityIdentifier = "Tap Me" label.accessibilityIdentifier = "Hello, World!"
With the accessibility identifiers in place, your tests can interact with the app’s UI elements in a clear and maintainable way. For example, a test that taps the button can now easily locate it using its identifier:
let button = app.buttons["Tap Me"]
After the basic structure and identifiers are set, you need to ensure that your UI tests are run in a suitable environment. In Xcode, you can choose between running your tests on the simulator or a physical device. Running tests on the simulator is typically faster and more convenient for iterative development, while testing on actual devices can expose issues related to performance and device-specific behaviors.
Moreover, it’s essential to manage the state of your application before running tests. This includes ensuring that your app launches in a known state. You can achieve this by resetting the app’s state each time your tests run. XCTest provides a method called launchArguments
that lets you customize the launch behavior of your application:
app.launchArguments = ["-UITestMode"] app.launch()
By using the launch arguments, you can trigger specific behaviors in your app, such as clearing user data or enabling debugging features, ensuring that your tests are consistent and reliable.
Ensuring that your environment is correctly set up also involves addressing issues related to network conditions or external dependencies. If your app relies on network calls, ponder using mocks or stubs to simulate responses, enabling your UI tests to run without needing a live server.
Writing Effective UI Tests
Writing effective UI tests is a fundamental aspect of ensuring the robustness of your application. In order to write tests that are both reliable and maintainable, it’s vital to adhere to several best practices, which will enhance the clarity and effectiveness of your test cases.
First and foremost, tests should be descriptive and self-explanatory. This means that the names of your test methods should clearly indicate what the test intends to verify. For example, instead of naming a test method test1()
, opt for a more descriptive name that conveys the purpose of the test:
class MyAppUITests: XCTestCase { func testTapButtonChangesLabelTextToHelloWorld() { let app = XCUIApplication() app.launch() app.buttons["Tap Me"].tap() XCTAssertEqual(app.staticTexts["Hello, World!"].label, "Hello, World!") } }
This approach not only makes it easier for you to understand what the test does at a glance but also aids others who may read your code in the future.
Another important consideration is the order in which your tests are executed. Tests should be independent of one another, meaning that the result of one test should not affect the result of another. This can be achieved by resetting the state of your app between tests. XCTest provides a setUp()
method that can be overridden to configure the state before each test:
override func setUp() { super.setUp() let app = XCUIApplication() app.launchArguments = ["-UITestMode"] app.launch() }
By launching the app with the desired conditions, you ensure that each test starts from a known state.
When it comes to element interaction, make use of accessibility identifiers as previously mentioned. They not only facilitate easier access to UI elements but also allow for cleaner test code. Avoid using hard-coded values for button titles or static text that may change in the future. Instead, rely on identifiers that are explicitly assigned in your UI code:
app.buttons["Tap Me"].tap() XCTAssertTrue(app.staticTexts["Hello, World!"].exists)
This practice makes your tests more robust against UI changes, as the tests remain tied to the identifiers rather than specific text strings.
Timing is another critical aspect of UI testing. UI elements may take time to appear or become interactable, especially in complex applications. Failure to account for this can lead to flaky tests that fail inconsistently. To mitigate this, leverage expectations provided by XCTest:
let label = app.staticTexts["Hello, World!"] let exists = NSPredicate(format: "exists == true") expectation(for: exists, evaluatedWith: label, handler: nil) waitForExpectations(timeout: 5, handler: nil) XCTAssertTrue(label.exists)
By waiting for elements to exist before asserting their properties, you can reduce the likelihood of false negatives in your test results.
Finally, keep your tests focused. Each test should ideally verify a single aspect of your application’s behavior. This not only makes it easier to pinpoint failures but also simplifies the process of identifying the root cause of issues when they arise. If a test fails, it should be simpler to assess which functionality is impacted.
Best Practices for UI Testing in Swift
When it comes to best practices for UI testing in Swift, the goal is to write tests that are efficient, maintainable, and robust against changes in your application’s user interface. To achieve this, several strategies can be employed that not only enhance the effectiveness of your tests but also improve your overall development workflow.
1. Use Descriptive Test Names
Your test names should convey their purpose clearly. This makes it easier for you and other developers to understand what each test is checking. A descriptive test name can significantly improve the readability of your test suite. Here’s an example of a descriptive test:
class MyAppUITests: XCTestCase {
func testLoginButtonEnabledWhenUsernameAndPasswordAreProvided() {
let app = XCUIApplication()
app.launch()app.textFields["Username"].tap()
app.textFields["Username"].typeText("[email protected]")