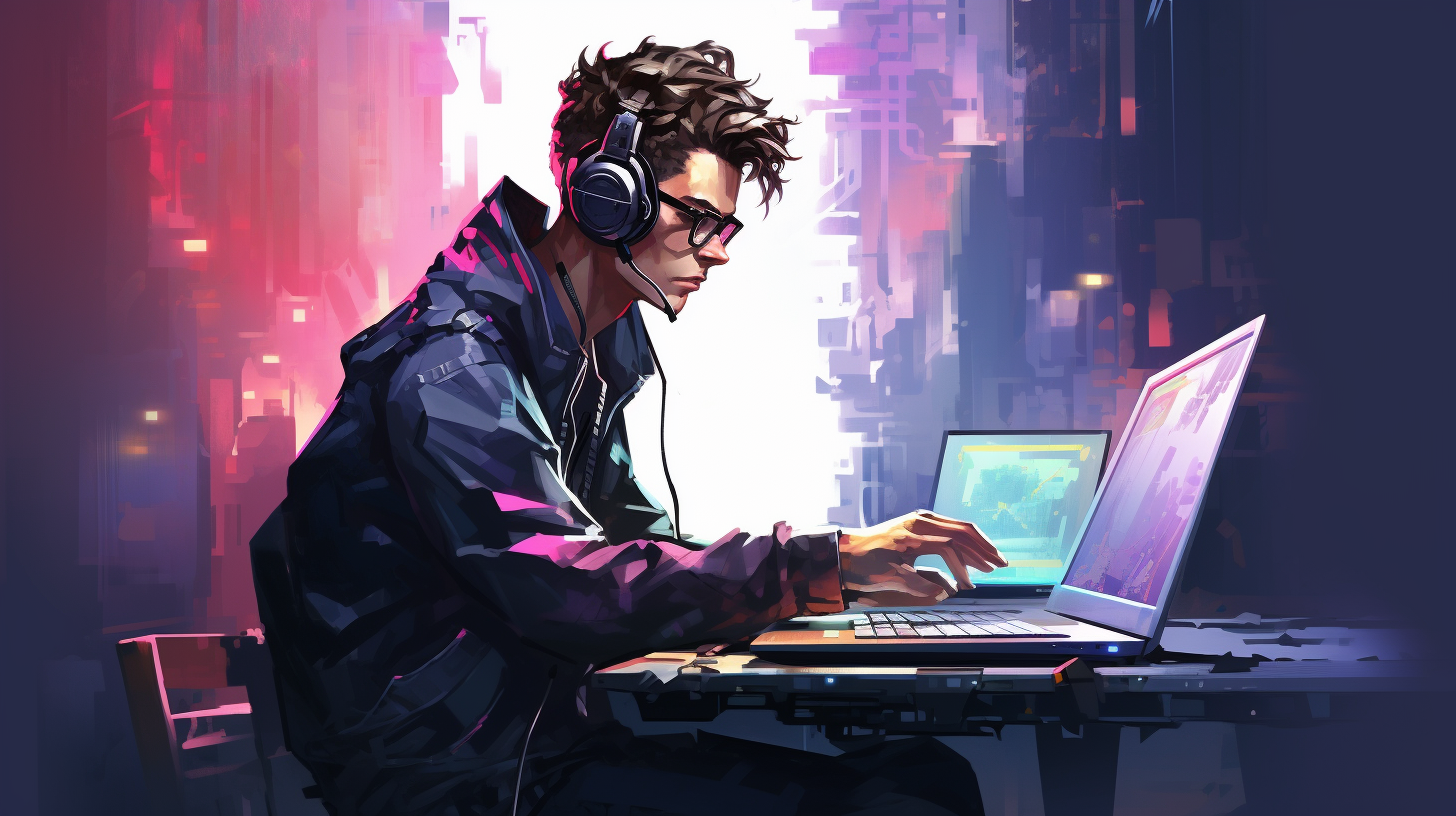
SQL and User-Defined Functions for Data Processing
User-Defined Functions (UDFs) in SQL offer a powerful way to encapsulate logic that can be reused throughout your database queries. They allow you to create custom functions that extend SQL’s built-in capabilities, enabling more complex operations to be performed directly within your queries. Understanding how UDFs work especially important for efficient database management and data processing.
A UDF is essentially a piece of code that you write to perform a specific task, returning a single value or a table. There are two main types of UDFs: scalar functions and table-valued functions. Scalar functions return a single value, whereas table-valued functions return a table. This distinction is important as it determines how these functions can be utilized within your SQL statements.
When defining a UDF, you declare its name, input parameters, and the return type. The logic of the function is encapsulated within the body of the function. Here’s a simple example of a scalar UDF that calculates the square of a number:
CREATE FUNCTION dbo.SquareNumber (@Number INT) RETURNS INT AS BEGIN RETURN @Number * @Number; END;
In this example, the function SquareNumber takes an integer parameter @Number and returns its square. Once a UDF is created, it can be called in SQL queries just like any built-in function:
SELECT dbo.SquareNumber(5) AS SquareValue;
This will return a result set with SquareValue equal to 25.
Table-valued functions are slightly more complex. They can return a result set that you can treat like a table. Here’s an example of a simple table-valued function that returns all employees with a specified department ID:
CREATE FUNCTION dbo.GetEmployeesByDepartment (@DepartmentID INT) RETURNS TABLE AS RETURN ( SELECT EmployeeID, FirstName, LastName FROM Employees WHERE DepartmentID = @DepartmentID );
Once this function is created, you can utilize it in a query as follows:
SELECT * FROM dbo.GetEmployeesByDepartment(1);
This will return a set of all employees that belong to the department with ID 1.
Understanding UDFs allows for better abstraction of code and improved maintainability in your SQL queries. They can encapsulate complex calculations, business logic, or data transformations that might otherwise clutter your SQL code. However, it’s essential to be mindful of their impact on performance, particularly when used in large datasets or within frequently executed queries.
Benefits of Using User-Defined Functions for Data Processing
Using User-Defined Functions (UDFs) in SQL comes with a myriad of benefits that can significantly enhance data processing capabilities. First and foremost, UDFs promote code reusability. By encapsulating complex logic into functions, you can call the same function from multiple places in your SQL code without having to re-write the logic each time. This not only reduces redundancy but also makes your SQL scripts cleaner and easier to maintain.
Another advantage is that UDFs can improve the readability of your SQL queries. Instead of embedding intricate logic directly into the query, which can make it difficult to understand at a glance, you can call a UDF with a meaningful name that conveys the purpose of the logic being executed. This abstraction simplifies the overall structure of your SQL statements, making it easier for others (and your future self) to comprehend the intent behind the queries.
Moreover, UDFs can encapsulate business logic that’s specific to your application or domain. Whether it’s calculating a discount based on business rules or transforming data formats, encapsulating this logic within a UDF ensures consistency across different parts of your application. For instance, if the discount rules change, you only need to update the logic in one place, rather than hunting down every occurrence of that logic throughout your SQL code.
Here’s a practical example of how a UDF can encapsulate business logic. Think a scenario where you want to apply a specific discount based on customer loyalty:
CREATE FUNCTION dbo.CalculateDiscount (@CustomerID INT, @PurchaseAmount DECIMAL(10, 2)) RETURNS DECIMAL(10, 2) AS BEGIN DECLARE @DiscountRate DECIMAL(5, 2); -- Sample logic for determining the discount rate IF EXISTS (SELECT * FROM Customers WHERE CustomerID = @CustomerID AND IsLoyal = 1) SET @DiscountRate = 0.10; -- 10% discount for loyal customers ELSE SET @DiscountRate = 0.00; -- No discount for non-loyal customers RETURN @PurchaseAmount * @DiscountRate; END;
In this example, the function CalculateDiscount takes a customer ID and a purchase amount as inputs and returns the discount amount. You can leverage this function in your queries that deal with customer purchases to apply consistent discount logic across the board:
SELECT OrderID, PurchaseAmount, dbo.CalculateDiscount(CustomerID, PurchaseAmount) AS DiscountAmount FROM Orders;
This returns each order with the calculated discount amount, ensuring that the discount logic is consistently applied.
Additionally, UDFs help in enhancing modularity in your SQL code. By breaking down complex operations into smaller, manageable functions, you can troubleshoot and test individual components more easily. This modular approach also facilitates parallel development, allowing different team members to work on different functions without stepping on each other’s toes.
One of the often-overlooked benefits of UDFs is the ability to simplify maintenance tasks. As databases evolve, changes in logic or business rules can necessitate updates to your SQL queries. With UDFs, you can minimize the impact of these changes. Updating a UDF ensures that all queries using that function automatically reflect the new logic, thereby reducing the time and effort required to maintain your SQL code.
The use of User-Defined Functions in SQL can lead to improved code reusability, enhanced readability, encapsulation of business logic, better modularity, and simplified maintenance. These benefits collectively contribute to a more efficient and manageable SQL environment, enabling developers to focus on crafting high-quality data solutions rather than getting bogged down in repetitive coding tasks.
Creating and Implementing User-Defined Functions
Creating and implementing User-Defined Functions (UDFs) in SQL involves a systematic approach that ensures your functions not only perform the intended tasks but also integrate seamlessly into your existing database environment. Once you are familiar with the syntax and conceptual framework for UDFs, the next step is to actually write and deploy them in your SQL database.
The first step is to define the function. You must clearly specify the function’s name, input parameters, return type, and the logic encapsulated within its body. Think a scenario where you need to compute the total price of products including tax. You can create a scalar UDF for this purpose:
CREATE FUNCTION dbo.CalculateTotalPrice (@Price DECIMAL(10, 2), @TaxRate DECIMAL(5, 2)) RETURNS DECIMAL(10, 2) AS BEGIN RETURN @Price + (@Price * @TaxRate); END;
This function, CalculateTotalPrice
, takes the price of a product and a tax rate as inputs and returns the total price after tax. This function can be seamlessly integrated into your SELECT statements:
SELECT ProductID, ProductName, dbo.CalculateTotalPrice(Price, 0.07) AS TotalPrice FROM Products;
In this example, you retrieve products along with their total price, using the UDF to perform the necessary calculation. This keeps the SQL query clean while ensuring that the business logic is encapsulated within the function itself.
When implementing UDFs, it is also crucial to consider error handling. Like any other piece of programming, SQL functions can encounter unexpected inputs or conditions. Using TRY...CATCH
blocks allows you to manage errors gracefully. Here’s an example of how you could enhance the CalculateTotalPrice
function with error handling:
CREATE FUNCTION dbo.CalculateTotalPrice (@Price DECIMAL(10, 2), @TaxRate DECIMAL(5, 2)) RETURNS DECIMAL(10, 2) AS BEGIN DECLARE @TotalPrice DECIMAL(10, 2); BEGIN TRY SET @TotalPrice = @Price + (@Price * @TaxRate); END TRY BEGIN CATCH RETURN NULL; -- or handle the error as needed END CATCH RETURN @TotalPrice; END;
In this modified function, if an error occurs during the calculation, it returns NULL, which will allow you to manage errors outside the function as necessary. This adds robustness to your UDFs, ensuring reliability in data processing.
When it comes to table-valued functions, the process is similar but requires returning a result set instead of a single value. For example, suppose you want to create a function that returns products in a specific category:
CREATE FUNCTION dbo.GetProductsByCategory (@CategoryID INT) RETURNS TABLE AS RETURN ( SELECT ProductID, ProductName, Price FROM Products WHERE CategoryID = @CategoryID );
To use this function, you can easily join it with other tables in your queries:
SELECT p.ProductID, p.ProductName, c.CategoryName FROM dbo.GetProductsByCategory(3) AS p JOIN Categories AS c ON p.CategoryID = c.CategoryID;
This example demonstrates how UDFs can return a table, allowing for complex queries that draw from multiple sources of data while maintaining clean and readable SQL code.
Finally, once you have created your UDFs, it is essential to test them thoroughly. Ensure that they handle edge cases and unexpected inputs gracefully. Performance testing is also important, especially for larger datasets, as poorly optimized functions can lead to degraded query performance.
By following these steps for creating and implementing UDFs, you can leverage their full potential in your SQL environment. UDFs not only enhance code clarity and maintainability but also empower developers to encapsulate complex logic effectively, making data processing tasks more intuitive and efficient.
Best Practices for Performance Optimization in SQL Functions
When it comes to optimizing the performance of User-Defined Functions (UDFs) in SQL, there are several best practices that can significantly enhance the efficiency and speed of your database operations. Following these practices can lead to more responsive applications and a better user experience, particularly when dealing with complex data processing tasks.
1. Choose the Right Type of Function
Understanding when to use scalar functions versus table-valued functions is critical. Scalar functions process each row individually, which can lead to performance degradation when applied to large datasets. In contrast, table-valued functions can return results in a set-based manner, which is generally more efficient. If you find that your UDF can be rewritten as a set-based operation, consider doing so.
CREATE FUNCTION dbo.GetEmployeeCountByDepartment (@DepartmentID INT) RETURNS INT AS BEGIN RETURN (SELECT COUNT(*) FROM Employees WHERE DepartmentID = @DepartmentID); END;
In this example, the function counts employees in a department. Instead of executing a row-by-row operation, it is advisable to leverage set-based logic within your function.
2. Minimize Side Effects
UDFs should be designed to be deterministic whenever possible. A deterministic function will always produce the same result for the same input parameters. Avoid using UDFs that modify database state or have side effects, as these can lead to unpredictable behavior and complicate performance tuning.
3. Keep Logic Simple
Complex logic within UDFs can lead to slower performance. Strive to keep your functions as simple as possible. If a function is doing too much, consider breaking it down into smaller, more manageable functions. This not only improves performance but also enhances readability and maintainability.
CREATE FUNCTION dbo.GetFullEmployeeName (@EmployeeID INT) RETURNS NVARCHAR(100) AS BEGIN DECLARE @FullName NVARCHAR(100); SELECT @FullName = FirstName + ' ' + LastName FROM Employees WHERE EmployeeID = @EmployeeID; RETURN @FullName; END;
This simple function retrieves an employee’s full name. It avoids unnecessary complexity, making it efficient for use in queries.
4. Avoid Using UDFs in SELECT Statements
Using UDFs directly in SELECT statements can lead to poor performance, especially in large datasets. SQL Server processes these functions row-by-row, which can severely impact execution time. Instead, use joins, subqueries, or computed columns where applicable to avoid this pitfall.
SELECT e.EmployeeID, e.FirstName, e.LastName, c.DepartmentName FROM Employees e JOIN Departments c ON e.DepartmentID = c.DepartmentID WHERE e.EmployeeID = 1;
In this example, a join is used instead of a UDF to retrieve the department name, which is more efficient.
5. Utilize Inline Table-Valued Functions
When you must create a table-valued function, ponder using inline table-valued functions instead of multi-statement table-valued functions. Inline functions are more efficient because they are essentially treated as views, allowing for better optimization by the SQL Server query engine.
CREATE FUNCTION dbo.GetDepartmentEmployees (@DepartmentID INT) RETURNS TABLE AS RETURN ( SELECT EmployeeID, FirstName, LastName FROM Employees WHERE DepartmentID = @DepartmentID );
By defining your function this way, you allow SQL to optimize the execution plan, leading to better performance.
6. Monitor and Analyze Performance
Finally, continuous monitoring and analysis of your UDFs in production are vital. Use SQL Server’s built-in tools, such as Query Store or SQL Profiler, to identify performance bottlenecks. Analyze how often your UDFs are being called and under what conditions to determine if further optimization is needed.
By integrating these best practices into your SQL development process, you can ensure that your User-Defined Functions are not only functional but also optimized for performance. That is essential for maintaining a responsive and efficient database environment, particularly as data volumes grow and application demands increase.