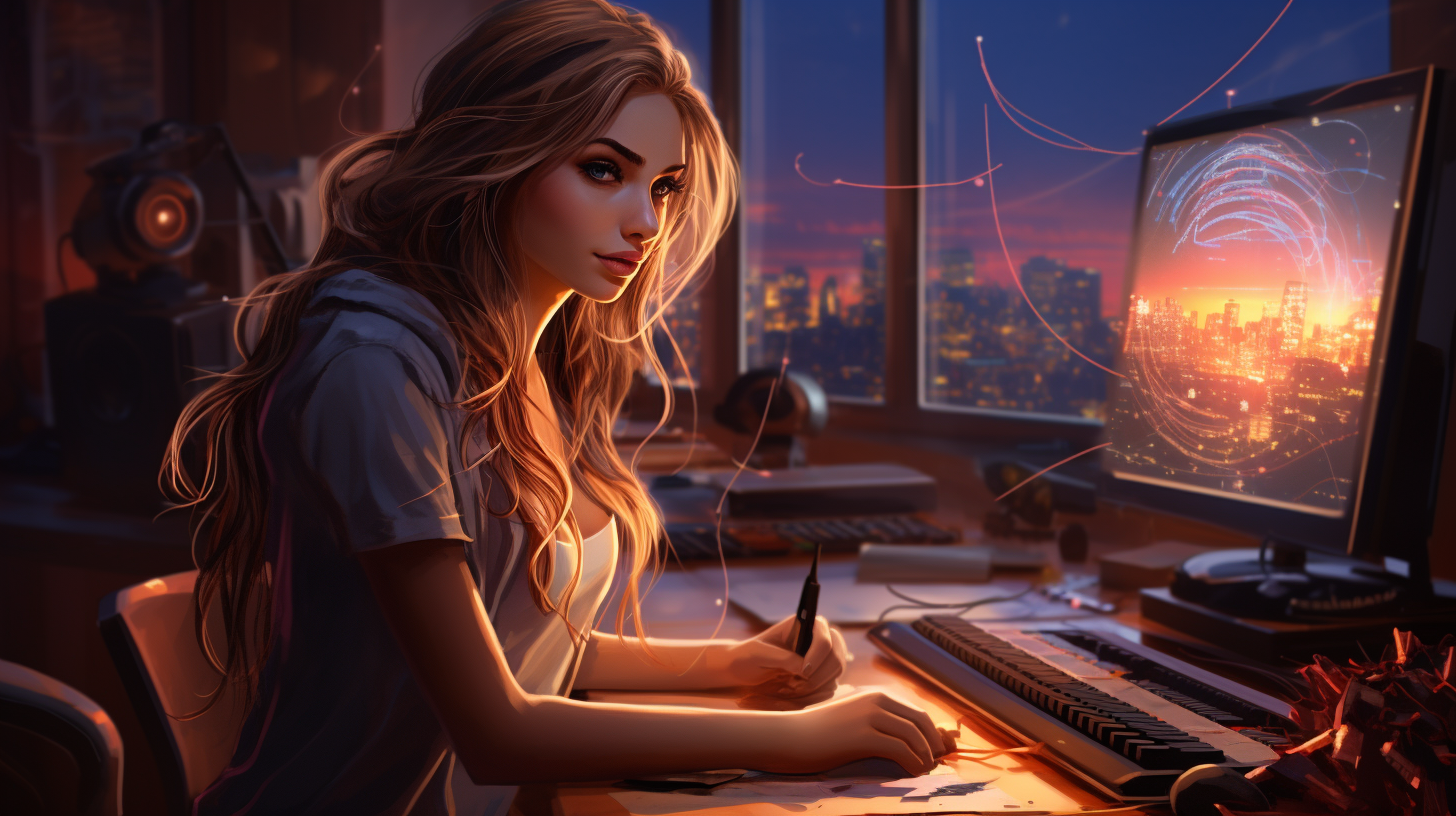
SQL and GraphQL: Data Retrieval Techniques
SQL (Structured Query Language) and GraphQL are both powerful tools for data retrieval, yet they operate on fundamentally different principles. Understanding the basics of each very important for choosing the right technology for your specific needs.
SQL is a domain-specific language used to manage and manipulate relational databases. It allows users to perform a variety of operations such as querying data, inserting records, updating information, and deleting records. SQL is built around the concept of tables, where data is organized into rows and columns. Each row represents a unique record, and each column represents a specific attribute of that record. A simple SQL query to retrieve data from a database might look like this:
SELECT first_name, last_name FROM users WHERE age > 21;
In this example, the SQL statement selects the first and last names of users older than 21 from the “users” table.
On the other hand, GraphQL is a query language for APIs and a runtime for executing those queries with your existing data. Unlike SQL, which is designed for relational databases, GraphQL is schema-based and allows clients to request only the data they need. This flexibility can help reduce over-fetching or under-fetching of data, which is common in traditional RESTful APIs. A GraphQL query might look like this:
{ user(id: "1") { firstName lastName } }
Here, the query requests the first and last name of the user with an ID of 1. The server responds with exactly the requested data structure, making it more efficient for clients that may have varying data needs.
Both SQL and GraphQL have their unique strengths and weaknesses. SQL excels in complex querying with joins and aggregations across multiple tables, which is a natural fit for relational databases. In contrast, GraphQL shines in scenarios where flexibility in data retrieval is paramount, allowing clients to specify exactly what they need.
Understanding these foundational concepts of SQL and GraphQL sets the stage for deeper exploration into their respective querying capabilities, performance considerations, and appropriate use cases.
Key Differences Between SQL and GraphQL
When comparing SQL and GraphQL, several key differences come to light that affect how each technology is applied in real-world scenarios. These differences can be categorized into areas such as data structure, query flexibility, error handling, and performance characteristics.
Data Structure and Organization
SQL databases are inherently relational, meaning they structure data into tables that have defined relationships with one another. This organization requires a well-thought-out schema that enforces data integrity through constraints like primary and foreign keys. In SQL, querying across these relationships is simpler, but it requires an understanding of the underlying schema.
SELECT users.first_name, orders.amount FROM users JOIN orders ON users.id = orders.user_id WHERE users.age > 21;
In contrast, GraphQL employs a hierarchical structure that allows clients to request specific fields from different types in a single query. The absence of rigid relationships means that GraphQL can return nested results in a more flexible manner, giving clients the power to dictate the shape of the data they receive.
{ users { firstName orders { amount } } }
Query Flexibility
One of the standout features of GraphQL is its ability to provide clients with the ability to specify exactly the data they require. This contrasts sharply with SQL, where the structure of the query is more static and defined by the schema. In SQL, retrieving related data often requires multiple queries or complex joins, while GraphQL enables efficient retrieval in a single request.
Error Handling
Error handling also differs significantly between the two. SQL typically returns an error response for invalid queries, which provides limited context regarding what went wrong. In contrast, GraphQL can return partial results along with error messages, allowing clients to understand which parts of their request were successfully processed. This feature can be particularly useful in applications that rely on fetching multiple resources concurrently.
Performance Characteristics
Performance can vary greatly depending on the nature of the data and the query patterns. SQL queries, when optimized with indexes and proper normalization, can perform exceptionally well, particularly for large datasets. However, poorly structured SQL queries can lead to performance bottlenecks, especially when multiple joins are involved.
CREATE INDEX idx_users_age ON users(age);
GraphQL, while flexible, can sometimes lead to performance issues as well, particularly when a single query requests a vast amount of nested data. Such scenarios can result in over-fetching, causing unnecessary load on the server. However, tools like batching and caching can help mitigate these issues.
// Example of batching in GraphQL const resolvers = { Query: { users: async () => { const users = await fetchUsers(); return users.map(user => ({ ...user, orders: await fetchUserOrders(user.id), })); }, }, };
Understanding these key differences between SQL and GraphQL allows developers to choose the right tool for the job based on the specific needs of their applications. Whether one favors the structured, relational nature of SQL or the flexible, client-driven architecture of GraphQL, each has its place in the landscape of state-of-the-art data retrieval techniques.
Data Querying in SQL: Techniques and Best Practices
Data querying in SQL involves a set of techniques and best practices that can significantly enhance the performance and maintainability of your database interactions. Understanding these techniques is essential for maximizing the efficiency of data retrieval operations.
One fundamental practice in SQL querying is the use of SELECT statements. A well-structured SELECT statement allows for precise data retrieval while minimizing the amount of data processed. For example, when retrieving data from a table, it’s advisable to specify only the necessary columns instead of using SELECT *.
SELECT first_name, last_name FROM users WHERE age > 21;
This query fetches only the required fields, reducing the amount of data transferred and processed.
Another technique to improve query efficiency is the implementation of indexes. Indexes enhance the speed of data retrieval operations, particularly with larger datasets. It’s important to index columns that are frequently used in WHERE clauses, JOIN conditions, or as sorting columns. For instance, creating an index on the “age” column can significantly accelerate queries filtering users by age:
CREATE INDEX idx_users_age ON users(age);
Using JOINs is another crucial aspect of SQL querying. JOINs enable you to combine rows from two or more tables based on related columns, facilitating the retrieval of complex datasets. However, using the correct type of JOIN (INNER JOIN, LEFT JOIN, etc.) based on your data needs is essential for optimal performance. For instance, a LEFT JOIN retains all records from the left table even if there are no matches in the right table:
SELECT users.first_name, orders.amount FROM users LEFT JOIN orders ON users.id = orders.user_id;
Additionally, employing filtering and grouping techniques in your queries can further streamline data retrieval. Using WHERE clauses helps narrow down results, while GROUP BY can be used to aggregate data effectively. Think the following example, which calculates the total order amounts for each user:
SELECT users.first_name, SUM(orders.amount) as total_amount FROM users JOIN orders ON users.id = orders.user_id GROUP BY users.first_name;
Furthermore, using subqueries allows for more complex querying capabilities. A subquery can be used to perform an additional query within the main query, helping to filter data efficiently. For instance, to find users whose total order amount exceeds a specific threshold, you can utilize a subquery:
SELECT first_name FROM users WHERE id IN (SELECT user_id FROM orders GROUP BY user_id HAVING SUM(amount) > 100);
Finally, it’s crucial to regularly monitor and optimize your queries. Employing the EXPLAIN command can help you analyze how your SQL queries are executed by the database, thereby identifying potential bottlenecks and optimization opportunities:
EXPLAIN SELECT first_name, last_name FROM users WHERE age > 21;
By applying these techniques and best practices, you can significantly enhance your SQL querying capabilities, ensuring efficient data retrieval and a better overall performance of your database applications.
Data Querying in GraphQL: Techniques and Best Practices
Data querying in GraphQL is characterized by its flexible and efficient approach to fetching data. Unlike traditional APIs, where the server dictates the structure of the response, GraphQL empowers clients to specify precisely what they need. This capability is particularly beneficial in scenarios where different clients may have varying data requirements. Here’s a closer look at some techniques and best practices for querying data in GraphQL.
One of the key techniques in GraphQL querying is the use of fragments. Fragments allow you to define reusable units of a query that can be included in multiple queries or parts of a query, helping to maintain consistency and reduce redundancy. For instance, if you often need to retrieve user details along with their orders, you can define a fragment for user details:
fragment UserDetails on User { id firstName lastName }
Then, when querying, you can include this fragment:
{ users { ...UserDetails orders { amount } } }
This not only makes your queries more concise but also improves maintainability, as any changes to the user details can be made in one place.
Another important aspect of GraphQL is its ability to handle nested queries efficiently. Clients can request complex data structures in a single query, which is a significant advantage over traditional REST APIs that typically require multiple round trips to the server. For example, if you want to retrieve user information along with their associated orders, you can structure your query like this:
{ users { id firstName orders { id amount } } }
This single query retrieves users and their orders in one go, minimizing network overhead and improving performance.
When it comes to performance considerations, it’s crucial to be aware of the potential pitfalls of overly complex queries, especially when fetching large amounts of nested data. To mitigate this risk, implementing depth limiting and complexity analysis can help control how deep and complex queries can go, thus preventing performance degradation on the server. For instance, you might limit queries to a maximum depth of 5 to prevent excessively deep nested structures.
Using caching strategies is also an excellent practice in GraphQL. Libraries like Apollo Client provide built-in caching mechanisms that can store previously fetched results, allowing for faster responses on subsequent queries. By caching responses, you can significantly reduce the load on your server and enhance the overall user experience.
Batching is another optimization technique often used in GraphQL. By grouping multiple requests into a single query, you can minimize the number of network calls. That is especially useful when a client needs to fetch data from multiple sources. Here’s a simple example of how batching might look:
{ users { id orders { id amount } } products { id name } }
In this example, both user orders and product data are fetched at the same time, reducing latency.
Finally, always ensure to handle errors gracefully in your GraphQL queries. Unlike SQL, where an error might halt execution, GraphQL can return partial results along with error messages. This means clients can still work with the data that was successfully retrieved while gaining insights into what went wrong. For example:
{ users { id firstName orders { id amount } __typename } }
In this query, if some user data is unavailable, the GraphQL response will still include the successful parts, allowing applications to handle missing data more effectively.
By embracing these querying techniques and best practices in GraphQL, developers can create more efficient, flexible, and maintainable applications that cater to the unique data needs of various clients.
Performance Considerations: SQL vs GraphQL
When considering the performance of SQL versus GraphQL, it is essential to acknowledge the distinct nature of their architectures and the implications these differences have on data retrieval efficiency. SQL, being a query language designed for relational databases, benefits from optimizations that leverage the database’s relational structure. In contrast, GraphQL, with its flexible querying capabilities, introduces its own set of performance considerations that developers must manage.
In SQL, performance is often contingent on how well queries are structured and how effectively the database is indexed. Efficient SQL queries can benefit immensely from the use of indexes, which facilitate faster data access. For example, if you are frequently searching for users based on their age, creating an index on the age column can substantially reduce query execution time:
CREATE INDEX idx_users_age ON users(age);
Moreover, SQL databases can utilize various optimization techniques, such as query caching, execution plans, and parallel processing, to improve performance further. Using the EXPLAIN
command allows developers to analyze query plans, identifying any performance bottlenecks:
EXPLAIN SELECT first_name FROM users WHERE age > 21;
In contrast, GraphQL’s performance challenges often arise from its flexibility. While the ability to request specific fields can reduce over-fetching, complex queries that request deeply nested data can lead to performance degradation. A single GraphQL query can spawn multiple database requests, especially if resolvers are not optimized. For example, fetching a user’s data along with their orders and products in one request may result in multiple database calls if not handled correctly:
const resolvers = { Query: { users: async () => { const users = await fetchUsers(); // Might fetch all users return users.map(user => ({ ...user, orders: await fetchUserOrders(user.id), // Fetch orders per user })); }, }, };
To mitigate these issues in GraphQL, developers can implement batching techniques, where multiple requests are combined into a single database call. This reduces the number of trips to the server and can significantly improve performance:
const resolvers = { Query: { users: async () => { const users = await fetchUsers(); const orders = await fetchUserOrders(); // Fetch all orders at once return users.map(user => ({ ...user, orders: orders.filter(order => order.userId === user.id), })); }, }, };
Another important consideration in GraphQL is the implementation of depth limiting and complexity analysis to prevent excessively deep or complex queries that could strain server resources. By setting limits, developers can ensure that queries remain performant while balancing flexibility:
const depthLimit = 5; // Set a maximum query depth
Furthermore, caching strategies play a pivotal role in enhancing GraphQL performance. By using tools such as Apollo Client, previously fetched results can be stored and reused, thus reducing the load on the server and improving response times:
const client = new ApolloClient({ cache: new InMemoryCache(), // Using in-memory cache });
Ultimately, both SQL and GraphQL demand careful consideration of performance characteristics to draw out their strengths effectively. SQL benefits from a well-structured, indexed relational database, while GraphQL requires a thoughtful approach to querying, batching, and caching to maintain efficiency in dynamic data retrieval environments. By understanding these performance considerations, developers can build robust applications that leverage the best of both worlds.
Use Cases: When to Choose SQL or GraphQL
When it comes to choosing between SQL and GraphQL for data retrieval, understanding specific use cases can greatly inform your decision. Each technology offers unique advantages that can cater to different application needs, making it essential to analyze the context in which they will be deployed.
SQL is particularly well-suited for applications that rely on structured data and require complex queries involving multiple relationships. For instance, traditional business applications that manage sales, inventory, and customer data often benefit from SQL’s powerful JOIN capabilities. The relational model ensures that data integrity is maintained and allows for rigorous data analysis through aggregate functions. Ponder an e-commerce application where you need to retrieve user information along with their order history. SQL handles this efficiently through structured queries:
SELECT users.first_name, COUNT(orders.id) as order_count FROM users LEFT JOIN orders ON users.id = orders.user_id GROUP BY users.first_name;
This SQL query effectively counts the number of orders placed by each user, a task that would require more complex nesting and restructuring in GraphQL.
On the other hand, GraphQL shines in scenarios where client requirements are dynamic and diverse. Its ability to allow clients to specify exactly what data is needed makes it perfect for applications with varying endpoints or platforms, such as mobile apps that may require different data sets based on user actions. For example, if a mobile application needs to display user profiles with optional details like addresses or recent orders, a GraphQL query can elegantly accommodate this variability:
{ user(id: "1") { firstName lastName orders { amount } address { street city } } }
This query retrieves user details along with either orders or address information, depending on what the client requests, thereby minimizing over-fetching.
GraphQL is particularly advantageous in microservices architectures, where different services may handle various aspects of data. A single GraphQL endpoint can consolidate these diverse data sources, providing a unified interface for the client. This capability makes GraphQL an excellent choice for applications that aggregate data from multiple services, enabling rapid development and iteration.
Despite their strengths, both technologies have scenarios where they are less effective. SQL may not be the best fit for applications requiring extreme flexibility or those that involve rapid changes in data structure. Conversely, GraphQL may present performance challenges in cases where extensive nested queries lead to increased server load or latency. In these instances, optimizing GraphQL resolvers or employing pagination strategies can help mitigate performance concerns.
Ultimately, the choice between SQL and GraphQL should be driven by the specific data requirements of your application, the complexity of the data relationships, and the expected client interaction patterns. Carefully evaluating these factors will help you select the most suitable technology for your data retrieval needs.