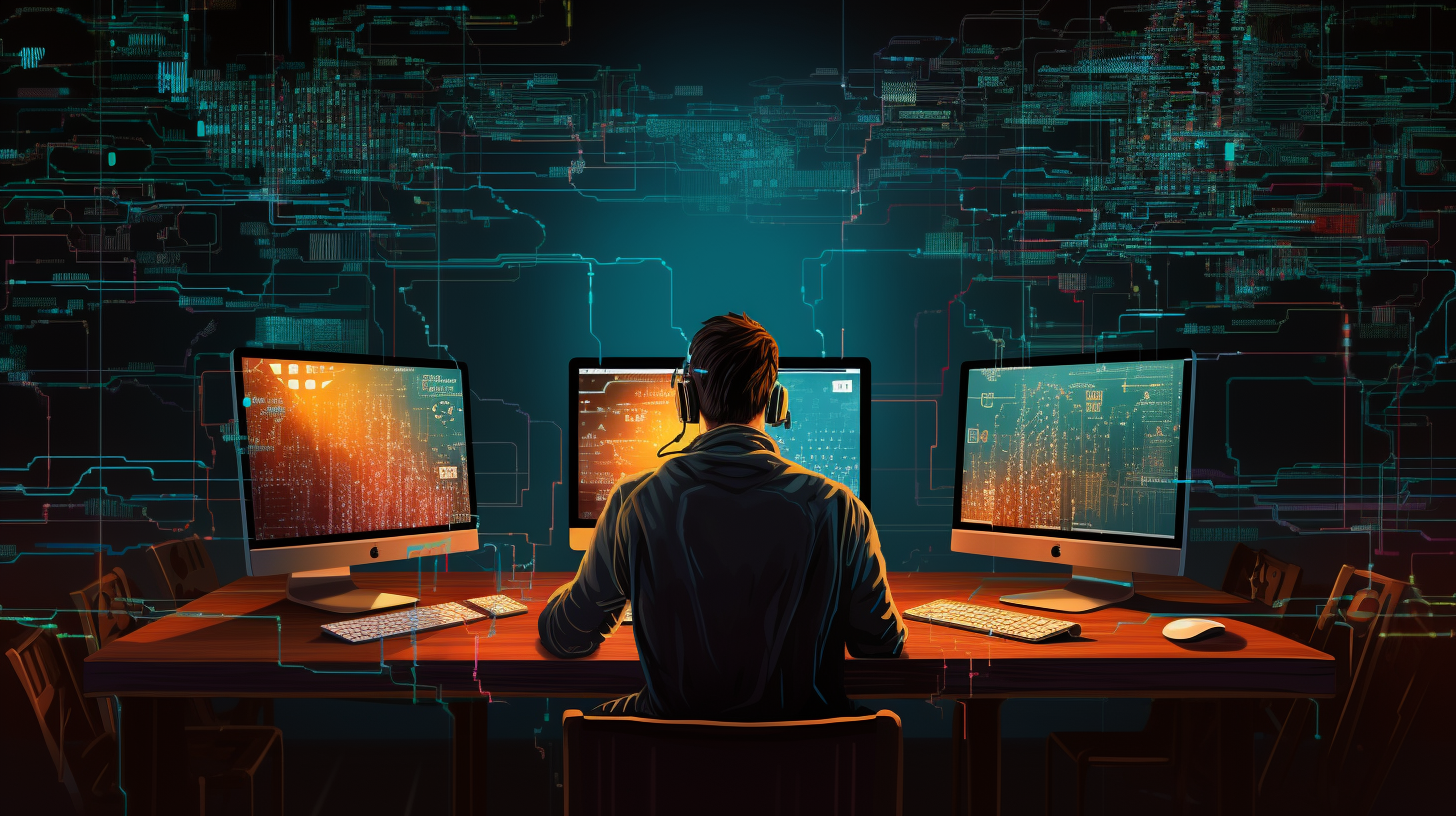
Java and Game Development: Basics and Frameworks
Java has long been a popular choice for game development due to its platform independence, robust libraries, and active community support. At its core, Java enables developers to write code once and run it anywhere, thanks to the Java Virtual Machine (JVM). This feature is particularly appealing in the gaming industry, where a wide variety of platforms such as PCs, consoles, and mobile devices demand flexibility.
One of the primary advantages of Java in game development is its rich ecosystem of libraries and frameworks. Libraries such as JavaFX for 2D graphics and LibGDX for cross-platform development provide developers with powerful tools to create visually engaging and interactive games. These libraries abstract much of the complexity involved in graphics rendering and input handling, allowing developers to focus on the game logic rather than the underlying details.
Another vital aspect of Java that benefits game developers is its memory management. Java’s automatic garbage collection helps manage memory allocation and deallocation, reducing the risk of memory leaks and improving overall game stability. This feature is particularly important for games that require extensive resource management, such as those with large asset loads or complex world states.
Moreover, Java’s strong typing system contributes to writing more reliable and maintainable code. The ability to catch errors at compile time rather than at runtime minimizes the occurrence of bugs during gameplay, enhancing the overall player experience. This especially important in game development, where performance and reliability can significantly impact player satisfaction.
Java also boasts a vast community that continuously contributes to its development. This community support translates into a plethora of resources, tutorials, and forums where developers can seek help or share their knowledge. Whether it’s learning about the latest game development techniques or finding solutions to specific problems, the Java community is an invaluable asset for game developers.
In practical terms, here’s a simple example of a basic game loop written in Java, which is fundamental in most game applications:
public class SimpleGameLoop { private boolean running; public void start() { running = true; runGameLoop(); } private void runGameLoop() { while (running) { updateGame(); // Update game logic renderGame(); // Render graphics sleep(16); // Approximate 60 FPS } } private void updateGame() { // Game logic updates (e.g., moving characters) } private void renderGame() { // Graphics rendering logic } private void sleep(int milliseconds) { try { Thread.sleep(milliseconds); } catch (InterruptedException e) { e.printStackTrace(); } } }
This code snippet illustrates the structure of a game loop, which especially important for updating the game state and rendering graphics at a consistent frame rate. By using Java’s capabilities, developers can create engaging experiences while managing the complexities of game logic and rendering.
Java’s role in game development is multifaceted. Its platform independence, strong ecosystem, built-in garbage collection, robust community support, and ease of use make it an attractive option for developers. As game complexity continues to grow, Java provides the tools and features necessary to tackle these challenges effectively.
Core Concepts of Game Programming in Java
Understanding the core concepts of game programming in Java is essential for aspiring developers who wish to create immersive gaming experiences. At its heart, game programming involves three primary components: the game loop, game state management, and input handling. Mastering these elements will empower developers to design games that are not only functional but also engaging for players.
The game loop is the backbone of any game. It controls the flow of the game by continuously updating the game state and rendering graphics in a cycle. A well-structured game loop ensures that the game runs smoothly, maintaining a consistent frame rate and responding promptly to player inputs. In Java, the game loop can be implemented using a simple while loop, as demonstrated in the previous example.
public class SimpleGameLoop { private boolean running; public void start() { running = true; runGameLoop(); } private void runGameLoop() { while (running) { updateGame(); // Update game logic renderGame(); // Render graphics sleep(16); // Approximate 60 FPS } } // Additional methods... }
Within the game loop, two critical methods are called: updateGame()
and renderGame()
. The updateGame()
method is where game logic takes place, such as moving characters, checking collisions, and managing game physics. The renderGame()
method handles the drawing of game objects on the screen, ensuring players see the latest game state.
Another fundamental aspect of game programming is managing the game state. The game state represents the current condition of the game, including player scores, levels, and any active entities. Efficiently managing the game state is vital for creating a seamless player experience. Java’s object-oriented design allows developers to encapsulate game state within classes, making it easier to manage and update as the game progresses.
public class GameState { private int playerScore; private int level; public GameState() { playerScore = 0; level = 1; } public void increaseScore(int points) { playerScore += points; } public void advanceLevel() { level++; } // Getters and other methods... }
This GameState
class exemplifies how to encapsulate the current score and level of the game. By managing the game state through dedicated classes, developers can easily update, retrieve, or reset values as needed, fostering cleaner code and improved readability.
Input handling is another critical component in game development. Players interact with games through various input devices such as keyboards, mice, or gamepads. Java provides several ways to handle user input, such as using the KeyListener
interface or libraries like LibGDX which abstract the input handling process. Detecting and responding to player input in real-time is essential for providing an intuitive and responsive gaming experience.
import java.awt.event.KeyEvent; import java.awt.event.KeyListener; public class InputHandler implements KeyListener { @Override public void keyPressed(KeyEvent e) { switch (e.getKeyCode()) { case KeyEvent.VK_UP: // Move player up break; case KeyEvent.VK_DOWN: // Move player down break; // Other key events... } } @Override public void keyReleased(KeyEvent e) { // Handle key release } @Override public void keyTyped(KeyEvent e) { // Handle key typed } }
In this example, the InputHandler
class listens for keyboard events and responds accordingly. Using a structured approach to input handling allows developers to create dynamic and interactive gameplay, which is important for player engagement.
By grasping these core concepts—game loop, game state management, and input handling—developers can build a solid foundation for their game projects in Java. Each piece plays a vital role in creating a cohesive and enjoyable gaming experience, while Java’s features enable developers to implement these concepts efficiently and effectively.
Popular Java Game Development Frameworks
As we delve into the game development frameworks available in Java, it’s crucial to highlight several prominent libraries that can significantly streamline the development process. These frameworks not only reduce the complexity of creating games but also enhance productivity and allow developers to focus on crafting engaging gameplay rather than getting caught up in the intricacies of low-level programming.
One of the most widely used frameworks is LibGDX. This open-source framework provides a comprehensive set of tools for developing cross-platform games. It supports 2D and 3D graphics, physics, audio, and input handling. With its architecture designed for performance, LibGDX facilitates the creation of games that can run on various platforms such as Windows, Linux, Android, and iOS. One of the standout features of LibGDX is its ability to use the same codebase across all platforms, which significantly reduces development time.
Here’s a simple example of creating a basic LibGDX application:
import com.badlogic.gdx.ApplicationAdapter; import com.badlogic.gdx.Gdx; import com.badlogic.gdx.graphics.GL20; import com.badlogic.gdx.graphics.Texture; import com.badlogic.gdx.graphics.g2d.SpriteBatch; public class MyGdxGame extends ApplicationAdapter { private SpriteBatch batch; private Texture img; @Override public void create() { batch = new SpriteBatch(); img = new Texture("badlogic.jpg"); } @Override public void render() { Gdx.gl.glClearColor(1, 0, 0, 1); Gdx.gl.glClear(GL20.GL_COLOR_BUFFER_BIT); batch.begin(); batch.draw(img, 0, 0); batch.end(); } @Override public void dispose() { batch.dispose(); img.dispose(); } }
In this code, we set up a simple LibGDX application that displays a texture on the screen. The create method initializes the resources, while the render method clears the screen and draws the image. The dispose method ensures that resources are cleaned up properly, which is vital for managing memory effectively.
Another notable framework is JavaFX, which is intended primarily for building rich graphical user interfaces. While often overlooked in game development, JavaFX provides powerful capabilities for creating 2D games. Its scene graph architecture simplifies the rendering of shapes, images, and animations, making it easier to implement visually appealing game elements. JavaFX’s built-in support for animations allows developers to create dynamic and responsive gameplay without needing extensive additional libraries.
For example, here’s how to create a simple JavaFX application with an animated circle:
import javafx.animation.Animation; import javafx.animation.KeyFrame; import javafx.animation.Timeline; import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.paint.Color; import javafx.scene.shape.Circle; import javafx.stage.Stage; import javafx.util.Duration; public class SimpleAnimation extends Application { @Override public void start(Stage primaryStage) { Circle circle = new Circle(50, Color.BLUE); circle.setTranslateX(100); circle.setTranslateY(100); Timeline timeline = new Timeline(new KeyFrame(Duration.seconds(1), e -> { circle.setTranslateX(circle.getTranslateX() + 10); })); timeline.setCycleCount(Animation.INDEFINITE); timeline.play(); Scene scene = new Scene(new Group(circle), 400, 400); primaryStage.setScene(scene); primaryStage.show(); } public static void main(String[] args) { launch(args); } }
This application creates a blue circle that moves horizontally across the screen. The Timeline class is used to define the animation, which updates the position of the circle every second. This showcases how JavaFX can be effectively utilized for animations in a game-like setting.
Lastly, Pure Java Game Engine (PJGE) and JMonkeyEngine are excellent choices for more complex game development, particularly if you are looking into 3D gaming. JMonkeyEngine is a powerful, open-source game engine that provides a comprehensive suite of tools for creating 3D games in Java. It supports features like scene graph management, physics simulations, and asset loading, which are essential for high-quality 3D game development.
Choosing the right framework depends on the specific requirements of your game project, including the target platform, desired graphics capabilities, and the complexity of gameplay. By using these frameworks, developers can harness the full potential of Java for game development, ensuring that their creations are both performant and compelling. As Java continues to evolve, so too will the frameworks that support game development, enabling a new wave of innovative and exciting games.
Best Practices for Java Game Development
When embarking on game development with Java, adhering to best practices is essential for ensuring your project is not only efficient but also maintainable and scalable. These practices encompass coding standards, architectural design, and performance optimization techniques, each playing an important role in successful game development.
1. Code Organization and Modularity
One of the cornerstones of maintainable code is modularity. This involves breaking down your code into smaller, reusable components. Java’s object-oriented nature facilitates this by allowing developers to encapsulate related functionalities within classes. For instance, you might create separate classes for different game entities, such as players, enemies, and items, each responsible for their behaviors and attributes:
class Player { private int health; private int score; public Player() { this.health = 100; this.score = 0; } public void takeDamage(int damage) { this.health -= damage; } public void increaseScore(int points) { this.score += points; } // Getters and other methods... } class Enemy { private int health; public Enemy(int health) { this.health = health; } public void attack(Player player) { player.takeDamage(10); } // Other enemy behaviors... }
This separation allows for easier debugging and testing, as you can focus on individual components without needing to navigate through a monolithic codebase.
2. Resource Management
In game development, managing resources such as textures, sounds, and other assets is vital. Loading all resources at once can lead to high memory usage and long loading times. A common best practice is to use a resource manager that loads assets on demand rather than all at startup. Here’s a simple example of a resource manager:
import java.util.HashMap; import java.util.Map; class ResourceManager { private Map textures = new HashMap(); public Texture getTexture(String path) { if (!textures.containsKey(path)) { textures.put(path, new Texture(path)); // Load the texture } return textures.get(path); } public void dispose() { for (Texture texture : textures.values()) { texture.dispose(); // Clean up resources } textures.clear(); } }
This code keeps track of loaded textures, only loading them when they’re first requested and providing a method to dispose of them when no longer needed, thus managing memory effectively.
3. Performance Optimization
Performance is critical in game development. Inefficient code can lead to frame drops and poor user experience. One common optimization technique is to minimize object creation within the game loop. Instead of creating new objects every frame, consider reusing existing instances:
class Bullet { private boolean active; public void activate() { active = true; } public void deactivate() { active = false; } public boolean isActive() { return active; } // Other bullet methods... } class BulletPool { private List bullets = new ArrayList(); public Bullet acquireBullet() { for (Bullet bullet : bullets) { if (!bullet.isActive()) { bullet.activate(); return bullet; } } Bullet newBullet = new Bullet(); bullets.add(newBullet); newBullet.activate(); return newBullet; } public void releaseBullet(Bullet bullet) { bullet.deactivate(); } }
This approach, known as object pooling, significantly reduces the overhead caused by frequent object creation and destruction, thus improving performance.
4. Utilize the Java Collections Framework
Java’s Collections Framework offers powerful data structures that can help optimize your game’s performance and organization. For example, using a HashMap to manage game entities allows for efficient lookups and updates, which is particularly useful in games where entities frequently change state or position:
import java.util.HashMap; class GameWorld { private HashMap entities = new HashMap(); public void addEntity(String id, GameEntity entity) { entities.put(id, entity); } public GameEntity getEntity(String id) { return entities.get(id); } public void updateEntities() { for (GameEntity entity : entities.values()) { entity.update(); // Update each entity } } }
Using the right data structures can yield better performance and enhanced readability, allowing developers to focus more on game design rather than on the underlying mechanics of data storage.
5. Testing and Debugging
Regular testing especially important in game development to identify and fix bugs early in the process. Automated unit tests can be invaluable, especially for game logic. Java’s JUnit framework is an excellent tool for this purpose. Think writing tests for critical game functions, ensuring they behave as expected:
import org.junit.Test; import static org.junit.Assert.*; public class PlayerTest { @Test public void testScoreIncrease() { Player player = new Player(); player.increaseScore(10); assertEquals(10, player.getScore()); } }
Incorporating testing into your development cycle not only enhances code quality but also boosts confidence when making changes or adding new features.
By adhering to these best practices—modularity, resource management, performance optimization, effective use of collections, and rigorous testing—Java game developers can create high-quality, engaging games while mitigating common pitfalls associated with game development. As you progress in your development journey, continually refining these practices will serve you well, ensuring that your projects are not just functional, but also a pleasure to work on.