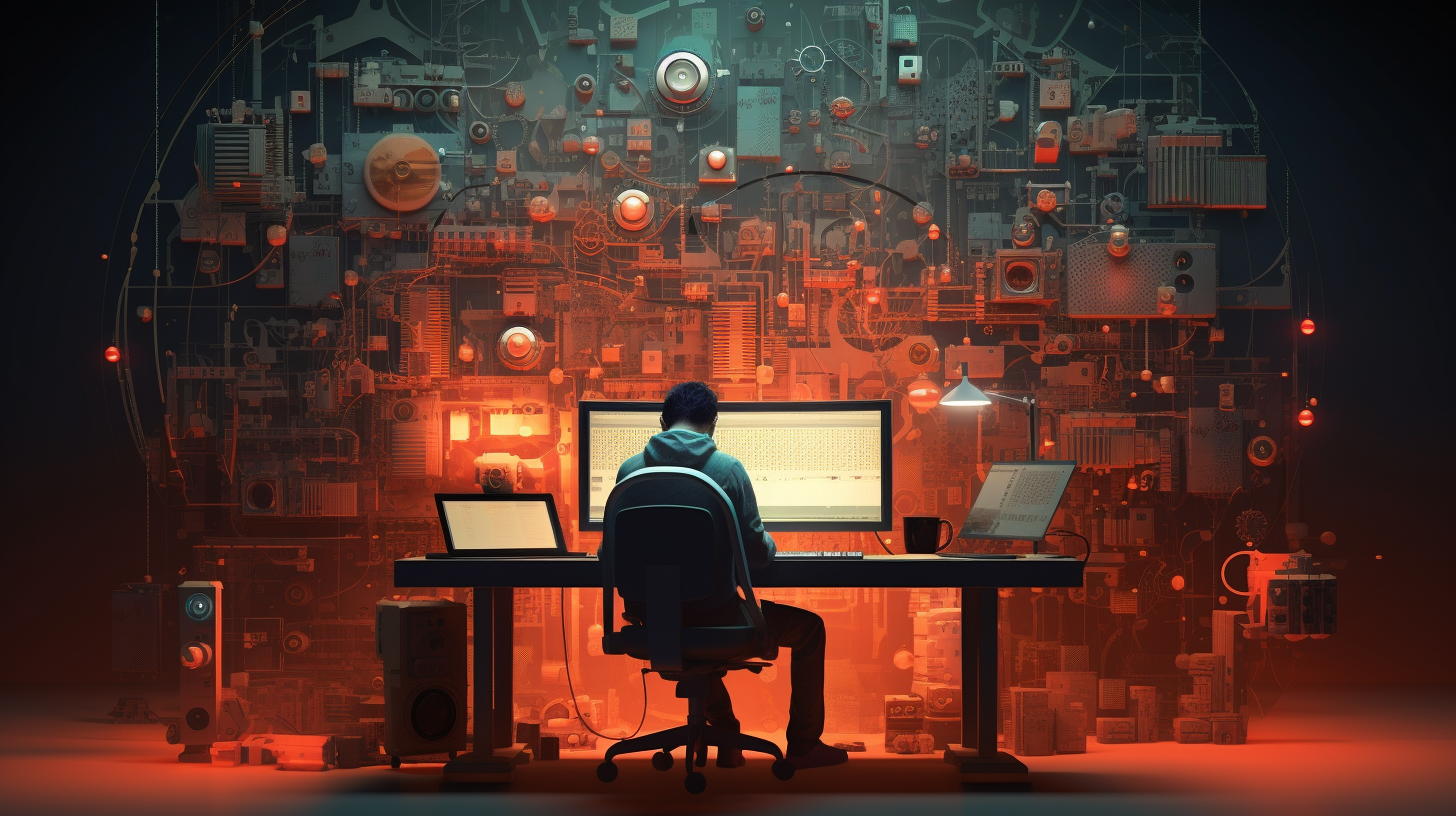
Python Lambda Functions
In Python, a lambda function is a small, anonymous function that is defined using the lambda
keyword. Unlike regular functions defined using the def
keyword, lambda functions can take any number of arguments but can only have one expression. This makes them particularly useful for short, throwaway functions that are not intended to be reused elsewhere in your code.
Lambda functions are often used in conjunction with functions like map()
, filter()
, and sorted()
, allowing for concise and readable code. The syntax for a lambda function is as follows:
lambda arguments: expression
Here, arguments represent the parameters that the function receives, and expression is the single expression that the function evaluates and returns.
Think the following example, where a lambda function is created to add two numbers:
add = lambda x, y: x + y result = add(5, 3) # result is 8
In this snippet, the lambda function add
takes two parameters, x
and y
, and returns their sum. The beauty of lambda functions lies in their simplicity and ability to be defined inline, making your code cleaner and more elegant.
Lambda functions can also be utilized for sorting, as shown in the following example:
points = [(1, 2), (3, 1), (5, 0)] sorted_points = sorted(points, key=lambda point: point[1]) # Sort by the second element # sorted_points is [(5, 0), (3, 1), (1, 2)]
In this case, the lambda function serves as a key function for sorted()
, allowing us to sort a list of tuples based on the second element of each tuple. The use of lambda functions in such contexts can greatly reduce the amount of boilerplate code needed.
While lambda functions provide an elegant solution for small, simple functions, it’s crucial to recognize their limitations. They’re designed to be used for simpler expressions and are not suited for complex operations that require multiple statements or extensive logic. For such scenarios, standard function definitions using def
are more appropriate.
Syntax and Usage of Lambda Functions
To further illustrate the syntax and usage of lambda functions, let us explore a few more practical examples that demonstrate their versatility in different contexts.
One common use case is in the context of filtering data. The filter()
function can be employed alongside a lambda function to eliminate elements from a list based on a condition. For instance, suppose you have a list of numbers and you want to filter out all numbers that are not even:
numbers = [1, 2, 3, 4, 5, 6, 7, 8] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) # Filters even numbers # even_numbers is [2, 4, 6, 8]
In this example, the lambda function lambda x: x % 2 == 0
checks whether each number is even, and filter()
uses this function to construct a new list containing only the even numbers. This succinctly captures the logic without the need for explicitly defining a separate function.
Another powerful application of lambda functions is within the scope of applying transformations to data structures. The map()
function can be utilized to apply a lambda function across a sequence. Ponder the following example where we want to square each number in a list:
numbers = [1, 2, 3, 4] squared_numbers = list(map(lambda x: x ** 2, numbers)) # Squares each number # squared_numbers is [1, 4, 9, 16]
The lambda function here, lambda x: x ** 2
, takes each element from the numbers
list and returns its square, resulting in a new list that holds the squared values.
Moreover, lambda functions shine in scenarios where quick, inline sorting is required. For example, if you have a list of dictionaries and you wish to sort them based on a specific key, you can seamlessly integrate a lambda function as follows:
data = [{'name': 'Alice', 'age': 30}, {'name': 'Bob', 'age': 25}, {'name': 'Charlie', 'age': 35}] sorted_data = sorted(data, key=lambda x: x['age']) # Sort by age # sorted_data is [{'name': 'Bob', 'age': 25}, {'name': 'Alice', 'age': 30}, {'name': 'Charlie', 'age': 35}]
In this case, the lambda function lambda x: x['age']
extracts the age attribute from each dictionary, allowing the sorted()
function to order the list accordingly. This exemplifies how lambda functions can provide clarity and brevity when working with complex data structures.
Ultimately, the effective use of lambda functions hinges on understanding their strengths and limitations. They excel in scenarios demanding simplicity and conciseness, streamlining code and enhancing readability. As you integrate lambda functions into your Python programming toolkit, ponder the context of usage to leverage their capabilities fully while maintaining the clarity of your code.
Common Use Cases for Lambda Functions
Lambda functions are a powerful tool in Python, particularly when it comes to applying functional programming techniques. Their ability to be used inline simplifies many operations that would otherwise require the overhead of formal function definitions. Let’s explore some common use cases where lambda functions truly shine, making your code not only more efficient but also more readable.
One of the most frequent applications of lambda functions is in the map()
function, where they allow for data transformation. For example, if you have a list of strings and you want to convert them to uppercase, you can elegantly achieve this through a lambda function as follows:
words = ['hello', 'world', 'python'] uppercase_words = list(map(lambda word: word.upper(), words)) # Convert to uppercase # uppercase_words is ['HELLO', 'WORLD', 'PYTHON']
Here, the lambda function lambda word: word.upper()
applies the upper()
method to each string in the words
list, producing a new list with all uppercase strings.
Another area where lambda functions excel is in filtering datasets, particularly using the filter()
function. Ponder a scenario where you have a collection of employee ages and need to select those who are eligible for retirement, which is typically defined as 65 years or older:
ages = [22, 45, 67, 34, 75, 55] retirement_eligible = list(filter(lambda age: age >= 65, ages)) # Select ages 65 and above # retirement_eligible is [67, 75]
In this example, the lambda function checks whether each age meets the retirement criterion, filtering the original list down to those who qualify.
Sorting data based on specific criteria is yet another common use case. When dealing with lists of tuples, for instance, you might need to sort them by the first element. Here’s how you can do that with a lambda function:
data = [(2, 'two'), (1, 'one'), (3, 'three')] sorted_data = sorted(data, key=lambda x: x[0]) # Sort by the first element # sorted_data is [(1, 'one'), (2, 'two'), (3, 'three')]
The lambda function lambda x: x[0]
extracts the first element of each tuple, allowing the sorted()
function to arrange the tuples in ascending order based on that element.
Lambda functions also provide an efficient way to implement custom sorting logic. Imagine you have a list of dictionaries representing products, and you want to sort them by price:
products = [{'name': 'Widget', 'price': 25}, {'name': 'Gadget', 'price': 15}, {'name': 'Doodad', 'price': 22}] sorted_products = sorted(products, key=lambda p: p['price']) # Sort by price # sorted_products is [{'name': 'Gadget', 'price': 15}, {'name': 'Doodad', 'price': 22}, {'name': 'Widget', 'price': 25}]
This example showcases the use of a lambda function to access the price of each product, achieving a simpler sorting mechanism without the need for additional function definitions.
Moreover, lambda functions can be extremely useful when working with advanced data structures and functional programming paradigms. For instance, they can be used for group-by operations, where you need to categorize data based on certain attributes. This flexibility in handling collections efficiently highlights the power of lambda functions.
In scenarios where you need to perform operations like these, lambda functions offer a succinct and readable approach that enhances your coding efficiency. Whether transforming data, filtering lists, or sorting collections, the ability to use lambda functions effectively can significantly streamline your Python programming efforts.
Limitations and Best Practices for Lambda Functions
When using the power of lambda functions in Python, it’s essential to remain cognizant of their inherent limitations and best practices to ensure your code remains both maintainable and efficient. Despite their elegance and succinctness, lambda functions are not a panacea; they come with trade-offs that can affect readability and debugging.
Limitations of Lambda Functions
Firstly, lambda functions are restricted to a single expression. While this constraint is what allows them to be compact, it also means that any complex logic requiring multiple statements or conditions cannot be encapsulated within a lambda. For example, if you need to handle multiple operations or include error handling, a traditional function defined with def
is more suitable:
def process_number(x): if x < 0: return "Negative number" return x ** 2
In this case, the function process_number
is capable of executing multiple statements, making it more versatile than a lambda function.
Another limitation is the potential for reduced readability. While lambda functions can make code more concise, they can also obscure intent, especially for readers unfamiliar with them. For instance, think using a lambda function for complex operations:
result = list(map(lambda x: x * 2 + 1 if x > 0 else 0, numbers))
This line performs a transformation that may not be immediately clear to someone reading the code. In such cases, defining a named function can clarify intent:
def transform(x): return x * 2 + 1 if x > 0 else 0 result = list(map(transform, numbers))
Here, the function transform
makes the operation clear, enhancing code readability.
Best Practices for Using Lambda Functions
To effectively incorporate lambda functions into your code, think the following best practices:
- Use lambda functions for simpler operations. If a lambda is becoming complex, refactor it into a named function.
- When using lambda functions, especially in higher-order functions like
map
orfilter
, ensure the context makes it clear what the function is doing. In some cases, assigning a lambda to a variable can help clarify its purpose:
double = lambda x: x * 2 result = list(map(double, numbers))
- Lambda functions should ideally be pure functions without side effects. This aligns with functional programming principles and makes your functions easier to test and reason about.
- If a lambda function is essential but not self-explanatory, don’t hesitate to add comments to describe its purpose and expected behavior.
By adhering to these practices, you can leverage the concise nature of lambda functions while maintaining clear, maintainable code. Ultimately, the key is to use lambda functions where they enhance code readability and efficiency, without compromising the clarity of your logic.