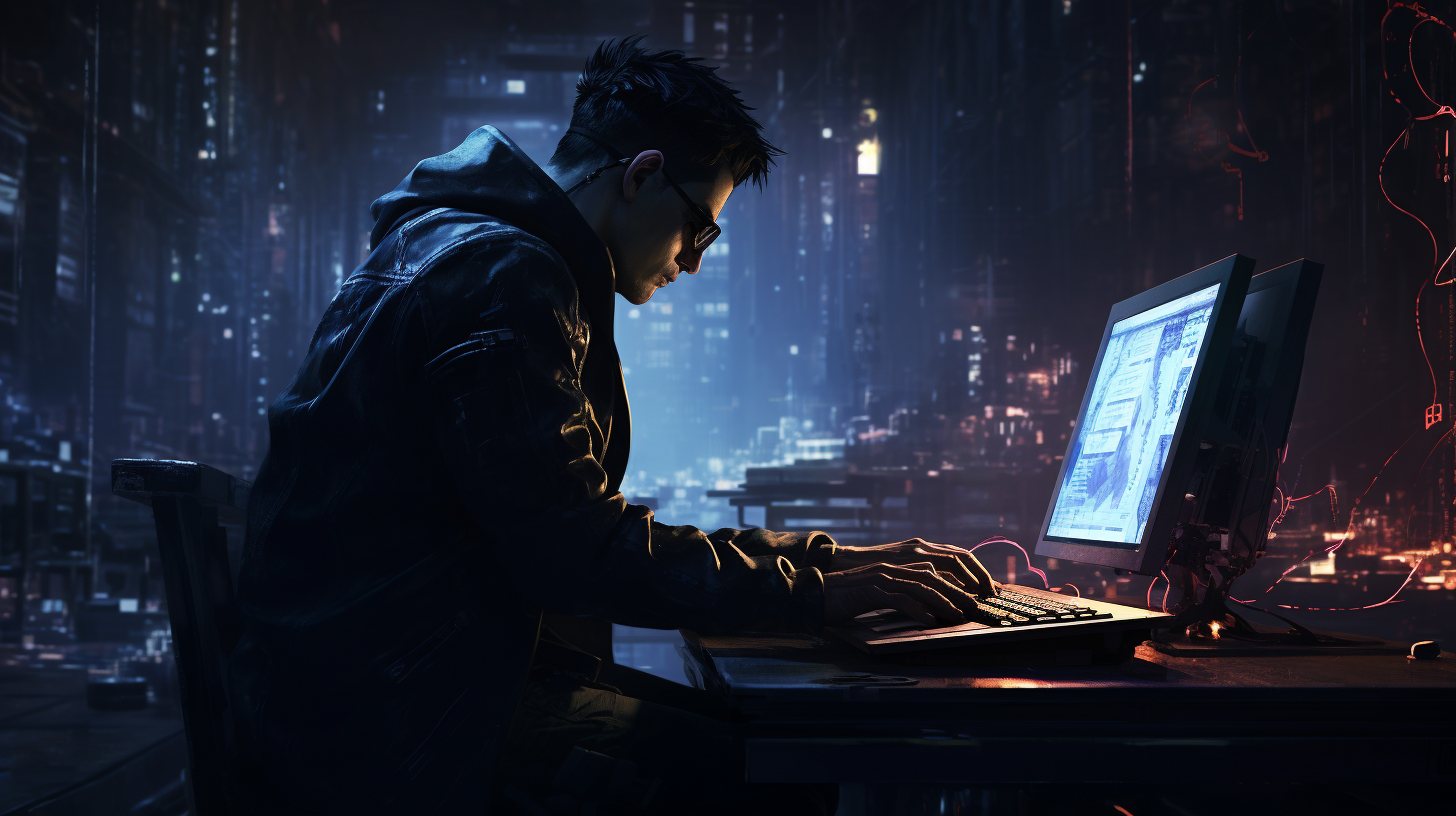
Managing Services and Daemons with Bash
In the Linux ecosystem, services and daemons play an important role in managing background processes and executing tasks without user intervention. Understanding how these entities operate can greatly enhance your ability to manage system resources effectively.
A service is a program that runs in the background, providing functionality to the system or other applications. It is typically tied to a particular function, such as a web server or database server, and is designed to be started and stopped by the system’s service manager.
A daemon, on the other hand, is a type of service specifically designed to run continuously, waiting to be activated by certain events or requests. Daemons are often started at boot time and operate independently of user sessions, allowing them to provide services like handling network requests, performing scheduled tasks, or managing hardware devices.
Both services and daemons are managed through various commands and scripts, which can be efficiently controlled using Bash. The service management system in Linux varies by distribution, with systemd and init being the most common systems. Understanding how to interact with these systems through Bash scripts is essential for effective service management.
To illustrate, consider a simple service management command using systemctl for a service called my_service
. Below is an example of how to start, stop, and check the status of this service using Bash:
# Start the service sudo systemctl start my_service # Stop the service sudo systemctl stop my_service # Check the status of the service sudo systemctl status my_service
Understanding the differences between services and daemons, as well as how to control them via the command line, is fundamental to managing a Linux environment effectively.
Creating Bash Scripts for Service Management
Creating Bash scripts for service management involves automating repetitive tasks associated with starting, stopping, and monitoring services and daemons. By using the power of Bash scripting, you can streamline these processes, reduce manual errors, and enhance your overall productivity.
First, let’s think the structure of a simple Bash script dedicated to managing a service. Below is a basic template that you can customize for any service:
#!/bin/bash SERVICE="my_service" ACTION=$1 case $ACTION in start) echo "Starting $SERVICE..." sudo systemctl start $SERVICE ;; stop) echo "Stopping $SERVICE..." sudo systemctl stop $SERVICE ;; status) echo "Checking status of $SERVICE..." sudo systemctl status $SERVICE ;; *) echo "Usage: $0 {start|stop|status}" exit 1 ;; esac exit 0
This script accepts a single argument that specifies the action to take: start, stop, or status. The case
statement handles each action appropriately, invoking the systemctl
command to manage the service. The script also includes basic usage instructions if an invalid argument is provided.
To create and run this script:
- Create a new file, for example
manage_service.sh
, and open it in your favorite text editor. - Copy the above script into the file.
chmod +x manage_service.sh
Now, you can execute the script to manage the specified service:
./manage_service.sh start
In addition to controlling services, you can enhance your script to log actions to a file, check for service dependency issues, or even notify you via email when a service fails to start. For logging, you might consider adding something like the following:
LOGFILE="/var/log/service_management.log" echo "$(date): $ACTION $SERVICE" >> $LOGFILE
Including logging not only helps you track actions but also aids in troubleshooting if an unexpected issue arises.
Ultimately, mastering the creation of Bash scripts for service management allows for a more controlled and efficient handling of services and daemons, helping to maintain system integrity and performance with minimal effort.
Starting and Stopping Services with Bash
Starting and Stopping Services with Bash Managing services through Bash scripts becomes particularly powerful when it comes to starting and stopping these processes. The ability to automate these tasks not only reduces manual overhead but also enhances reliability and consistency when services are required. Bash provides a simpler interface to execute system commands, including those necessary to control services. In modern Linux systems that utilize systemd, the commands `systemctl start` and `systemctl stop` are your primary tools. These commands control the lifecycle of a service effectively, allowing for seamless transitions from inactive to active states and vice versa. Think an example where you need to start the Apache web server, commonly known as httpd or apache2, depending on your distribution. The command would look like this:sudo systemctl start apache2Similarly, if you need to stop the service, you would use:
sudo systemctl stop apache2This functionality is critical for managing system resources, especially in environments where uptime very important. However, ensuring that services start or stop gracefully is equally important. Bash allows you to check the status of a service before taking action, helping to avoid unnecessary errors.
For instance, you can create a script to encapsulate this logic, ensuring that a service is only started if it isn't already running. Here’s how you can structure such a script:
#!/bin/bash SERVICE="apache2" ACTION=$1 # Function to check service status check_status() { systemctl is-active --quiet $SERVICE return $? } case $ACTION in start) if check_status; then echo "$SERVICE is already running." else echo "Starting $SERVICE..." sudo systemctl start $SERVICE fi ;; stop) if check_status; then echo "Stopping $SERVICE..." sudo systemctl stop $SERVICE else echo "$SERVICE is not running." fi ;; status) echo "Checking status of $SERVICE..." systemctl status $SERVICE ;; *) echo "Usage: $0 {start|stop|status}" exit 1 ;; esac exit 0This enhanced script not only starts and stops the service but also checks its current state before making changes. This preventive measure is vital in environments with stringent uptime requirements, particularly for production servers.
To execute this script, save it as `manage_apache.sh`, mark it as executable, and run the desired action:
chmod +x manage_apache.sh ./manage_apache.sh startBy using such scripts, you can automate the management of services, reduce the risk of human error, and enhance the overall reliability of your system. The blend of Bash scripting with service management commands allows system administrators to maintain control over their services without sacrificing efficiency or effectiveness. This mastery of Bash is an important skill in the toolkit of any proficient Linux administrator.
Checking the Status of Services and Daemons
Checking the status of services and daemons is a fundamental aspect of maintaining a healthy Linux system. Ensuring that essential services are running as expected can prevent potential disruptions and improve system reliability. The `systemctl` command offers a powerful interface for querying the current state of services managed by systemd, which is prevalent in many Linux distributions.
To check the status of a service, you can use the command:
sudo systemctl status my_serviceThis command provides detailed information about the service, including whether it's active (running), inactive (stopped), or failed. You will also receive logs regarding recent activity, which can be invaluable for troubleshooting issues.
To create a more automated approach, ponder writing a Bash script that checks the status of a specified service and provides a human-readable summary. Below is an example script that accomplishes this task:
#!/bin/bash SERVICE="my_service" # Function to check service status check_status() { if systemctl is-active --quiet $SERVICE; then echo "$SERVICE is currently running." else echo "$SERVICE is not running." fi if systemctl is-enabled --quiet $SERVICE; then echo "$SERVICE is enabled to start at boot." else echo "$SERVICE is not enabled to start at boot." fi echo "Current status information:" systemctl status $SERVICE } # Execute the function check_statusThis script defines a function called `check_status` that checks both the active state and whether the service is set to start automatically at boot time. It provides clear output to the user, indicating the current status of the service.
You can save this script as `check_service_status.sh`, make it executable, and run it by executing:
chmod +x check_service_status.sh ./check_service_status.shIn addition to using `systemctl`, you can also check the status of older init systems, such as SysVinit, with the `service` command. This command can serve a similar purpose, albeit with less detailed output. For example:
service my_service statusRegardless of the system initialization method, the ability to verify service status quickly is essential for effective system administration. Automating these checks with scripts not only saves time but also ensures that you can promptly respond to issues that may arise, thus maintaining optimal system performance.
As you delve deeper into managing services and daemons, consider integrating logs and notifications into your scripts. You can capture the output of your status checks into log files for historical analysis or send alerts via email or messaging platforms when a service fails to run as expected. This proactive approach can significantly enhance your ability to manage system health and performance.
Automating Service Management with Cron
Automating service management using Cron is an essential technique for maintaining system stability and reliability in a Linux environment. Cron is a time-based job scheduler that allows you to run scripts and commands at specified intervals, making it an ideal tool for automating the management of services and daemons. By using Cron, you can ensure that vital services are regularly checked, restarted if necessary, or even scheduled for maintenance tasks without manual intervention.
To get started with Cron, you first need to understand how to create and manage Cron jobs. Each user can have their own set of Cron jobs, which are defined in the crontab file. You can edit your user's crontab by running:
crontab -eIn the crontab editor, you will define your jobs using a specific syntax that includes the minute, hour, day of the month, month, day of the week, and the command to execute. Here’s a brief look at the syntax:
* * * * * command_to_executeEach asterisk symbolizes a time field, allowing you to specify when the command should be executed. For example, to run a script every day at 2:30 AM, you would write:
30 2 * * * /path/to/your/script.shNow, let’s create a Bash script that automatically checks the status of a service and restarts it if it is found to be inactive. Here’s a simple script that accomplishes this:
#!/bin/bash SERVICE="my_service" if ! systemctl is-active --quiet $SERVICE; then echo "$(date): $SERVICE is down. Attempting to restart..." >> /var/log/service_monitor.log sudo systemctl start $SERVICE if systemctl is-active --quiet $SERVICE; then echo "$(date): Successfully restarted $SERVICE." >> /var/log/service_monitor.log else echo "$(date): Failed to restart $SERVICE." >> /var/log/service_monitor.log fi else echo "$(date): $SERVICE is running." >> /var/log/service_monitor.log fiThis script checks whether the specified service is active. If it's not running, it attempts to restart the service and logs the outcome. You will want to route the output to a log file for future reference, which is particularly useful for troubleshooting and monitoring service health.
After saving this script (for example, as `monitor_service.sh`), make it executable:
chmod +x /path/to/monitor_service.shNext, you’ll schedule this script to run at regular intervals using cron. Suppose you want to check the status of your service every five minutes. You would add the following line to your crontab:
*/5 * * * * /path/to/monitor_service.shThis entry ensures that your `monitor_service.sh` script runs every five minutes, providing a proactive approach to service management. By automating these checks, you significantly reduce the risk of service downtime and maintain a more stable operating environment.
Additionally, you can enhance this automation by integrating email notifications. For instance, if the service fails to restart after attempts, you might want to receive an alert. You can utilize the `mail` command within the script to send an email notification in such instances:
if ! systemctl start $SERVICE; then echo "Failed to restart $SERVICE. Please check the system." | mail -s "$SERVICE Alert" [email protected] fiIncorporating these strategies allows you to leverage Cron effectively, creating a robust service management system that frees you from constant manual oversight while ensuring your services are always running as expected.
Troubleshooting Common Service Issues in Bash
Troubleshooting service issues in Linux can often feel like navigating a maze without a map. However, with the right Bash tools and techniques, you can streamline this process and gain valuable insights into the health of your services and daemons. When a service fails to start, unexpectedly stops, or behaves erratically, the first step in troubleshooting is to gather as much information as possible about the service’s current status, logs, and configuration.
Central to effective troubleshooting is the ability to check logs. Most services write their operational logs to specific files, often located in the /var/log directory. For systemd services, you can utilize the journalctl command to view logs. Here’s how you can check the logs for a service called my_service:
sudo journalctl -u my_serviceThis command will display all logs related to my_service, allowing you to pinpoint any error messages or warnings that might indicate why the service isn’t functioning as expected. If the service has been restarted multiple times, you can limit the output to the most recent logs by using the -n option followed by the number of lines you want to view:
sudo journalctl -u my_service -n 50In addition to logs, understanding the service’s configuration is important. Misconfigurations often lead to start failures or unexpected behavior. Access the configuration file for the service, typically found in /etc/systemd/system or /etc/init.d, depending on your system. You can use tools like nano or vim to inspect these files:
sudo nano /etc/systemd/system/my_service.serviceMake sure to validate the configuration settings. Look for common issues like incorrect paths, missing dependencies, or syntax errors. Once you’ve made any necessary changes, reload the systemd manager configuration to apply the updates:
sudo systemctl daemon-reloadAnother important aspect of troubleshooting is checking for dependency issues. Services often rely on other services to function correctly. If a dependent service isn’t running, it can cause failures. To check the dependencies of a service, you can use the following command:
systemctl list-dependencies my_serviceThis will provide you with a tree of dependencies, helping you identify if any required services are inactive. If you find that a dependency is down, you can use the previously mentioned service management commands to start it, which might resolve your primary service’s issues.
To encapsulate these troubleshooting steps in a Bash script can greatly enhance your productivity. Here’s a sample script that automates the process of checking the status, logs, and configuration of a specified service:
#!/bin/bash SERVICE="my_service" echo "Checking status of $SERVICE..." systemctl status $SERVICE echo "Fetching logs for $SERVICE..." sudo journalctl -u $SERVICE -n 50 echo "Checking dependencies for $SERVICE..." systemctl list-dependencies $SERVICE echo "Opening configuration file for $SERVICE..." sudo nano /etc/systemd/system/$SERVICE.serviceSave this script as check_service_troubleshoot.sh, make it executable with chmod +x check_service_troubleshoot.sh, and run it with ./check_service_troubleshoot.sh. This script will provide a quick overview of critical information and help you diagnose issues more efficiently.
Remember that troubleshooting is often iterative. You may need to repeatedly check logs, alter configurations, and restart services until you pinpoint the exact problem. By using Bash scripts, you can automate repetitive tasks, so that you can focus on solving the problem rather than spending time on manual checks.